Solving Common Issues with Android NotificationListenerService
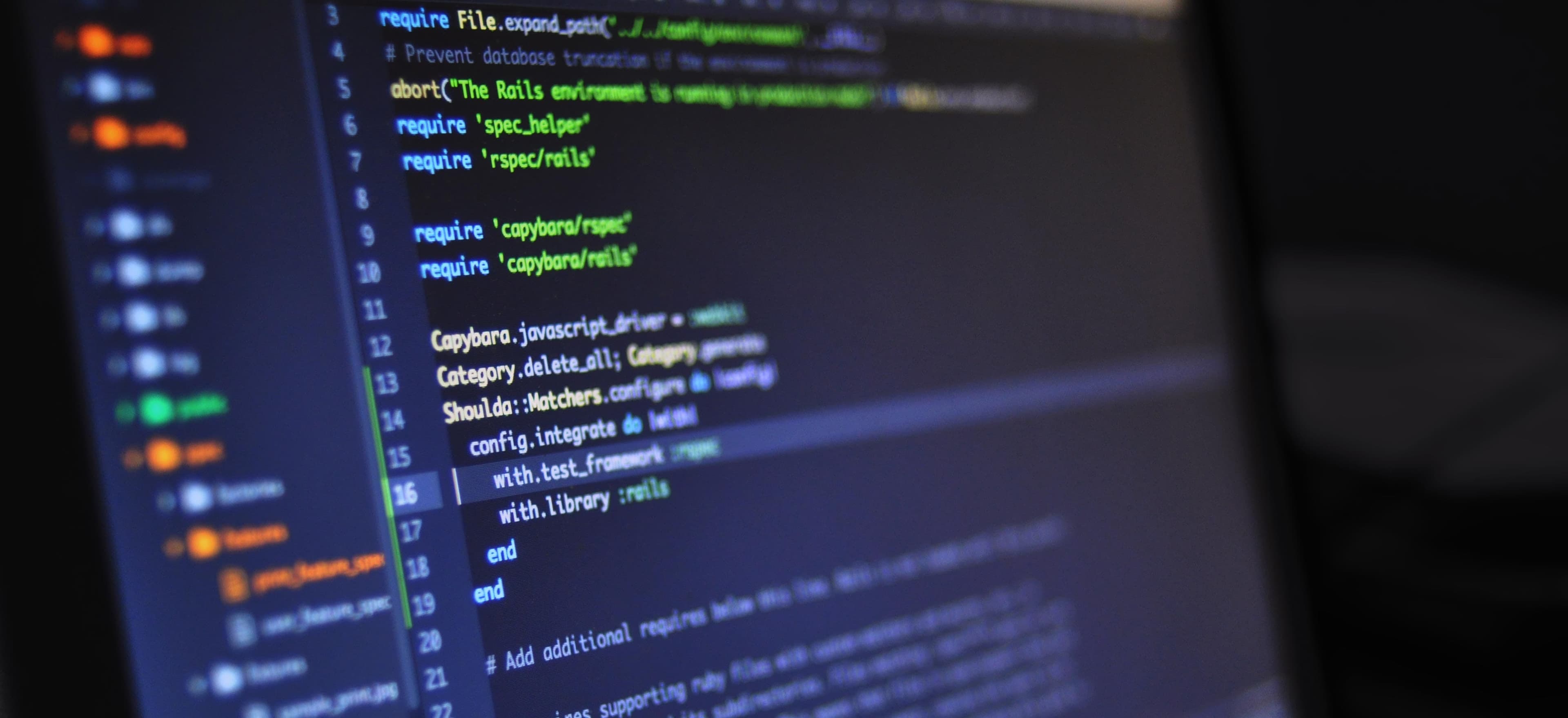
- Published on
Solving Common Issues with Android NotificationListenerService
The Android NotificationListenerService
is a powerful tool that allows your application to listen to notifications posted by the system or other apps. It can enhance user experience by providing timely and contextual information. However, it can also present some challenges during implementation. In this blog post, we will explore common issues developers frequently encounter with NotificationListenerService
and how to solve them, all while providing practical code examples and insightful commentary.
What is NotificationListenerService?
Before diving into troubleshooting, let’s understand what NotificationListenerService
is and how it works. This service allows you to receive and respond to notifications in real time. You can access notifications as they are posted, dismissed, or updated. You can also interact with them, providing context-aware functionality.
Here's a brief overview of how to declare it in your AndroidManifest.xml
:
<service
android:name=".YourNotificationListenerService"
android:permission="android.permission.BIND_NOTIFICATION_LISTENER_SERVICE">
<intent-filter>
<action android:name="android.service.notification.NotificationListenerService"/>
</intent-filter>
</service>
Make sure to replace .YourNotificationListenerService
with your own service implementation. The android.permission.BIND_NOTIFICATION_LISTENER_SERVICE
permission is essential for your service to operate correctly.
Common Issues and Solutions
1. Service Not Being Called
Issue: One of the frequent issues developers encounter is that the service is not being called or invoked as expected.
Solution: Ensure that your service is correctly registered in the manifest. Verify the following:
- Confirm that the package name is correct.
- Make sure you have the right permissions declared, particularly the
BIND_NOTIFICATION_LISTENER_SERVICE
. - Check that your device runs a version of Android that supports
NotificationListenerService
(Android 4.3 Jelly Bean and above).
2. Missing Notifications
Issue: Sometimes, notifications might not be captured even though they are posted.
Solution: This usually points to filtering issues. Add logs in your onNotificationPosted()
method to check if the notifications are being received:
@Override
public void onNotificationPosted(StatusBarNotification sbn) {
Log.d("NotificationListener", "Notification Posted: " + sbn.getPackageName());
}
If you see the logs but not the notifications, it means the service is working but the notifications may not be processed correctly.
3. Overriding Methods Incorrectly
Issue: Another common mistake involves overriding the incorrect methods in your service.
Solution: Ensure that you override only the methods relevant to your functionality. Here’s a basic structure for a NotificationListenerService
:
public class YourNotificationListenerService extends NotificationListenerService {
@Override
public void onNotificationPosted(StatusBarNotification sbn) {
// Handle the notification
}
@Override
public void onNotificationRemoved(StatusBarNotification sbn) {
// Handle notification removal
}
}
Make sure to use the correct notifications’ package names and IDs to track them accurately.
4. Permissions Not Granted
Issue: Users may unintentionally not grant the necessary permissions.
Solution: Always include an inline explanation for users if they have not granted notification access. You can redirect them to the notification settings.
if (!isNotificationServiceEnabled()) {
// Show a dialog or a Toast to direct users to Settings
Intent intent = new Intent();
intent.setAction(Settings.ACTION_NOTIFICATION_LISTENER_SETTINGS);
startActivity(intent);
}
You can check if your service is enabled with this method:
private boolean isNotificationServiceEnabled() {
String pkgName = getPackageName();
String flat = Settings.Secure.getString(getContentResolver(), "enabled_notification_listeners");
return flat != null && flat.contains(pkgName);
}
5. Handling Different Notification Types
Issue: Notifications can come from various sources, and handling all of them might be tricky.
Solution: You can categorize notifications by their types and take appropriate actions. Use the getCategory()
method on StatusBarNotification
:
@Override
public void onNotificationPosted(StatusBarNotification sbn) {
String category = sbn.getNotification().category;
switch (category) {
case Notification.CATEGORY_MESSAGE:
// Handle messaging notifications
break;
case Notification.CATEGORY_CALL:
// Handle call notifications
break;
default:
break;
}
}
This approach allows you to create different flows for various types of notifications, enhancing user engagement.
6. Testing Difficulties
Issue: Testing notification services can sometimes seem daunting.
Solution: Use emulators or create testing scenarios in a controlled environment. The adb
(Android Debug Bridge) can simulate notifications as well:
adb shell am broadcast -a android.intent.action.NEW_OUTGOING_CALL --ez is_outgoing false --es number "1234567890"
This command will help you ensure that your service reacts correctly to different notification scenarios.
Best Practices for Using NotificationListenerService
Here are some best practices to follow while working with NotificationListenerService
:
-
Optimize Notifications Handling: Only process notifications you need. This can enhance performance and reduce unnecessary complexity.
-
Respect User Privacy: Ensure you handle sensitive data responsibly. Avoid logging sensitive information.
-
User Settings for Configurations: Allow users to enable/disable certain notifications in your app settings. This not only respects user choices but also refines the user experience.
-
Testing Across Multiple Devices: Notifications and their behavior can vary between devices and OS versions. It is crucial to test your implementation on multiple devices.
-
User Education: Teach users how to enable notification access if they aren't aware of it. Providing links to documentation can be immensely helpful.
My Closing Thoughts on the Matter
The NotificationListenerService
is a powerful feature of Android that can significantly elevate the interactivity and user experience of your application. However, as with any powerful tool, it can present challenges. By addressing common issues and adhering to best practices, you can implement an effective notification listener.
By understanding your audience’s needs and actively managing notifications, you can provide contextual interactions that truly resonate. For additional resources, check the Android Developers Documentation.
If you have more questions or need further assistance, feel free to leave your comments below! Happy coding!
Checkout our other articles