Unlocking the Complexity: Regex Performance Issues in Java 9
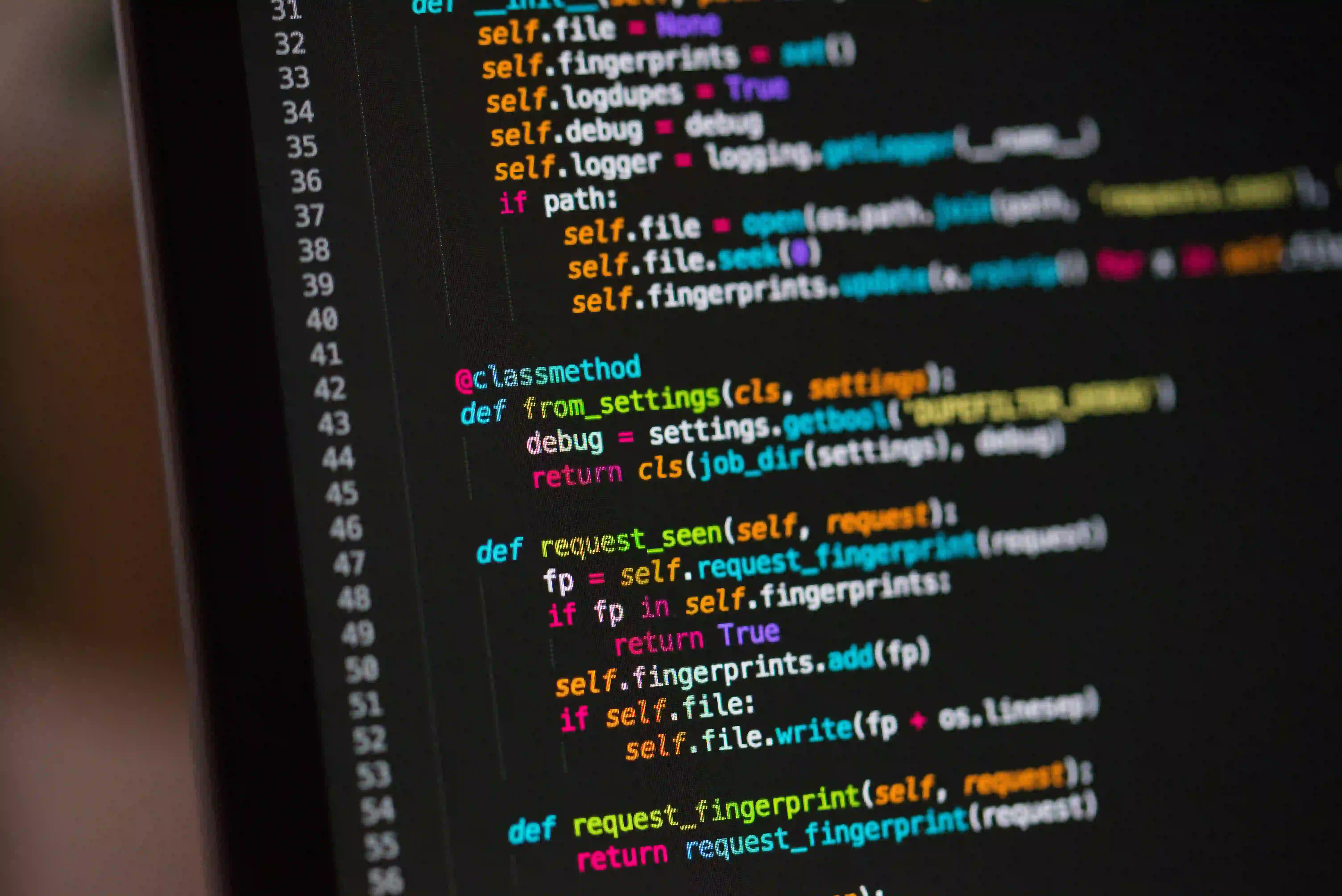
Unlocking the Complexity: Regex Performance Issues in Java 9
Regular expressions (regex) are a powerful tool for string manipulation, data validation, and data extraction. However, when it comes to performance, regex can often unveil complexities that developers might not anticipate, especially in Java 9. In this article, we'll explore the fundamental aspects of regex performance issues in Java 9, how to identify these problems, and the best practices to mitigate them.
Understanding Regex in Java
Before diving into performance issues, let’s quickly recap how Java handles regex. The Java regex engine is part of the java.util.regex
package, allowing for powerful string matching capabilities through patterns.
Example of a Simple Regex Match
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class RegexExample {
public static void main(String[] args) {
String text = "The quick brown fox jumps over the lazy dog.";
String regex = "\\bfox\\b";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(text);
if (matcher.find()) {
System.out.println("Found: " + matcher.group());
} else {
System.out.println("Not found");
}
}
}
In the example above, we compile a regex to find the word 'fox' within the provided text. The Pattern.compile()
method optimizes the regex for performance, making it reusable.
Performance Issues in Java 9
While the regex engine in Java is efficient, certain scenarios can lead to performance bottlenecks. Here, we'll discuss some typical regex performance issues in Java 9.
1. Catastrophic Backtracking
Catastrophic backtracking occurs when a regex pattern uses many quantifiers (e.g., *
, +
, ?
) or alternations (|
). In such cases, the regex engine may try a vast number of combinations to find a match, leading to significant slowdowns.
Example of Catastrophic Backtracking
String regex = "(a+)+b";
String text = "aaaaaaaaaaaaaaaaaaaaaaab"; // Many 'a's followed by one 'b'
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(text);
System.out.println("Matches: " + matcher.find()); // This can take a long time
Why This Happens: The nested quantifiers can generate exponential possibilities, and if the input string is large, the matching process can take a considerable amount of time.
2. Unanchored Patterns
Unanchored patterns (patterns that do not specify start or end positions) can potentially lead to significant performance issues. The engine checks every possible position in the string rather than narrowing its search space.
Example of Unanchored Pattern
String regex = "fox";
String text = "The quick brown fox jumps over the lazy dog. fox is fast.";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(text);
while (matcher.find()) {
System.out.println("Found: " + matcher.group());
}
Mitigation: If you know your text starts or ends with a specific boundary, utilize the ^
and $
anchors to limit the search space.
3. Greedy vs. Lazy Quantifiers
Using greedy quantifiers (e.g., .*
) can cause performance problems because they attempt to match as much input as possible, leading to backtracking.
Greedy Example
String regex = ".*bc";
String text = "aaaaaaabc";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(text);
if (matcher.find()) {
System.out.println("Greedy Match: " + matcher.group());
}
Lazy quantifiers (e.g., .*?
) can be used to improve performance when you want a match that consumes the least amount of input before moving on.
Lazy Example
String regex = ".*?bc";
String text = "aaaaaaabc";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(text);
if (matcher.find()) {
System.out.println("Lazy Match: " + matcher.group());
}
4. Using Character Classes Wisely
Regex patterns using character classes (e.g., [a-zA-Z]
) are generally faster than using alternations. Prefer character classes over multiple alternatives.
Example of Character Class Usage
String regex = "[a-zA-Z]+";
String text = "123abc456";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(text);
while (matcher.find()) {
System.out.println("Found: " + matcher.group());
}
Best Practices for Regex Performance
To ensure your regex is efficient in Java 9, consider these best practices:
1. Compile Regex Patterns
Always compile your regex patterns into a Pattern
object. Reusing a compiled pattern is much faster than recompiling it each time.
2. Limit the Use of Wildcards and Quantifiers
Avoid excessive use of .*
, .+
, and nested quantifiers. Instead, be specific with your regex.
3. Use Anchors
Where possible, use ^
and $
to specify the start and end of your string, limiting the search space.
4. Opt for Lazy Matches
Use lazy quantifiers when you want to minimize the amount of input processed at once.
5. Profile and Test
Use tools like Java's built-in java.util.regex
profiling and external libraries like JMH (Java Microbenchmark Harness) to assess performance and optimize.
Lessons Learned
Regex is a powerful tool in Java 9, but it comes with complexity. Understanding and addressing regex performance issues can save you from unexpected slowdowns and inefficiencies. By applying best practices such as compiling patterns, avoiding catastrophic backtracking, and choosing greedy versus lazy quantifiers wisely, you can maximize the efficiency of your regex operations.
For more details on regex patterns and their usage, check the official Java documentation.
By taking these insights into account, you'll harness the full power of regex in your Java applications, while maintaining optimal performance. Happy coding!