Improving Signal-to-Noise Ratio in Your Codebase
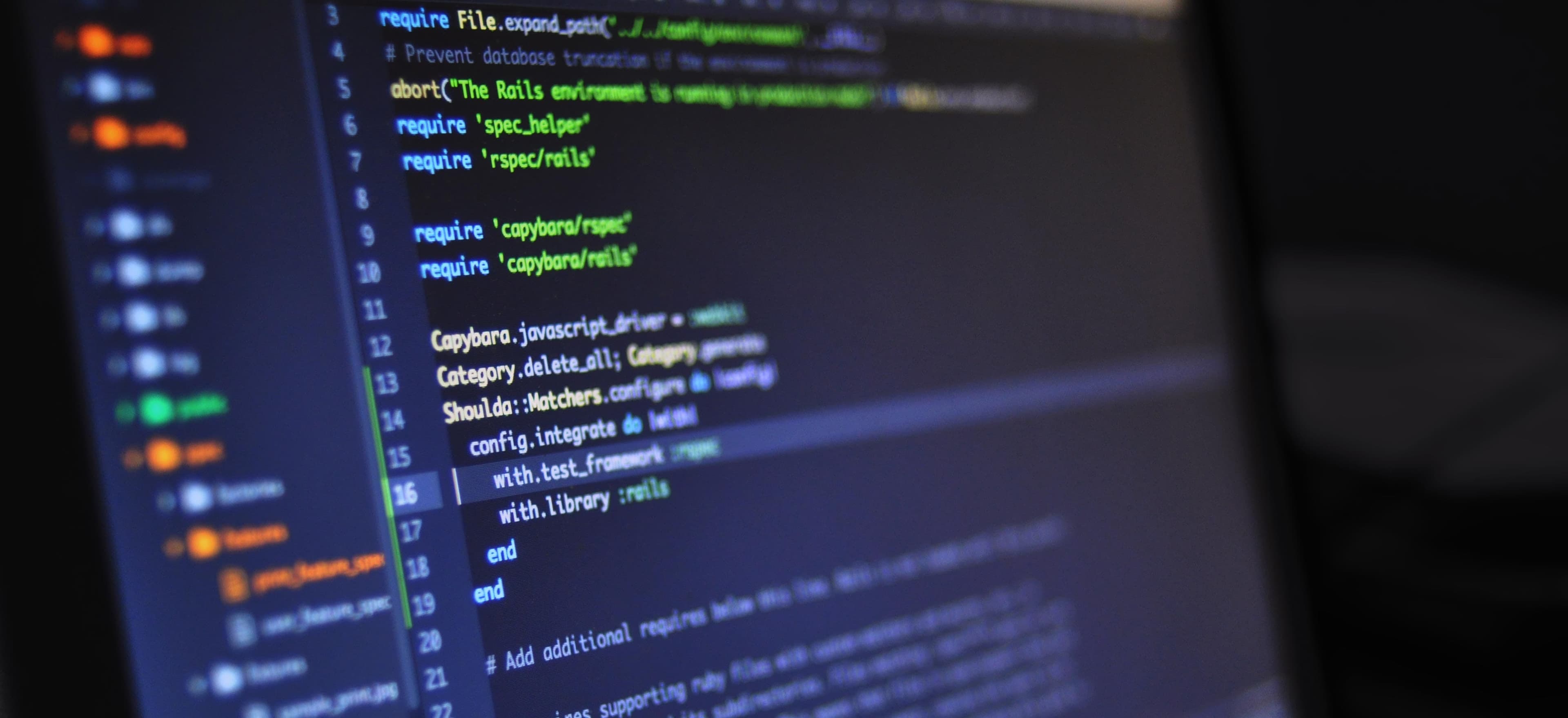
- Published on
Improving Signal-to-Noise Ratio in Your Codebase
In software development, the term Signal-to-Noise Ratio (SNR) refers to the clarity and quality of the essential information (signal) within a codebase relative to the irrelevant, redundant, or distracting content (noise). A high SNR is crucial as it facilitates better understanding, maintainability, and scalability of software projects. This blog post delves into techniques for improving the SNR in your codebase, making it cleaner, more readable, and easier to work with.
Understanding Signal and Noise in Code
Signal in programming represents the valuable, useful parts of the code—functions, classes, and libraries that directly contribute to the application's core functionalities. Noise, on the other hand, often includes:
- Unused variables and functions
- Overly complex algorithms
- Redundant code
- Poorly structured comments
- Inconsistent naming conventions
A high ratio of signal to noise means that the valuable information stands out clearly to anyone reading the code.
Why is a High SNR Important?
- Enhanced Readability: Clear and concise code is easier to read, thereby reducing the cognitive load on developers.
- Easier Maintenance: When the noise is minimized, maintaining the codebase becomes more straightforward. It's easier to track down bugs and understand complex sections of code.
- Improved Collaborations: Team members can work more effectively when the SNR is high, as they can quickly grasp what each section of the code does.
- Faster Onboarding: New developers can get up to speed more quickly with a well-structured codebase.
Techniques to Improve SNR
1. Refactor Regularly
Refactoring is the process of restructuring existing code without changing its external behavior. It’s a vital practice for enhancing readibility and removing noise.
For instance, consider the following example of a cluttered function:
public void process(int[] data) {
for (int i = 0; i < data.length; i++) {
if (data[i] > 0) {
// Do something
System.out.println(data[i] * 2);
}
}
}
Improved Version
public void processPositiveData(int[] data) {
for (int number : data) {
if (isPositive(number)) {
printProcessedData(number);
}
}
}
private boolean isPositive(int number) {
return number > 0;
}
private void printProcessedData(int number) {
System.out.println(number * 2);
}
Why This Matters: The improved version breaks down the logic into smaller, more focused methods, enhancing readability and easing maintenance.
2. Embrace the DRY Principle
The DRY (Don't Repeat Yourself) principle encourages avoiding code duplication. Redundant code constitutes noise because it can lead to inconsistencies and make code harder to maintain.
Here's an example of code that violates the DRY principle:
public void drawCircle() {
System.out.println("Drawing Circle");
// Code to draw Circle
}
public void drawSquare() {
System.out.println("Drawing Square");
// Code to draw Square
}
// Lots of similar drawing methods...
DRY Implementation
public void drawShape(Shape shape) {
if (shape instanceof Circle) {
System.out.println("Drawing Circle");
// Code to draw Circle
} else if (shape instanceof Square) {
System.out.println("Drawing Square");
// Code to draw Square
}
}
Why This Matters: This approach reduces the number of lines in your codebase, lower redundancy, and structures your code to enhance clarity.
3. Use Meaningful Naming Conventions
Choosing descriptive and meaningful names for variables, methods, and classes can significantly improve SNR. Avoid cryptic names as they contribute to noise.
Consider:
public void a() {
// Some processing
}
Improved Version
public void calculateMonthlyRevenue() {
// Some processing related to revenue
}
Why This Matters: Meaningful names reflect the purpose of elements in your code, leading to better self-documentation and ease for anyone reading the code.
4. Write Comprehensive Comments
Comments should tell the story behind the implementation of the code. However, they should never serve as a substitute for clear, well-structured code. Comments lose their value when they are redundant or vague.
Bad Comment Example:
int x = 5; // Set x to five
Good Comment Example:
int maxRetries = 5; // Maximum number of retry attempts for API calls
Why This Matters: Clear comments that explain the motivation behind the logic add value and reduce the potential for misunderstanding.
5. Apply Consistent Formatting
Consistent formatting makes code easier to read. Gobbling up space with inconsistent indentation or variable positioning can confuse any reader. Java doesn’t enforce formatting, but you can adopt tools like Google Java Style Guide for reference.
An example of inconsistent formatting:
public void example() {
int a = 1;int b = 2;System.out.println(a+b);
}
Consistently Formatted Version
public void displaySum() {
int a = 1;
int b = 2;
System.out.println(a + b);
}
Why This Matters: Consistency in formatting leads to better readability and helps focus attention on the signal in your code.
6. Automated Testing
Implementing automated tests allows developers to verify the functionality of code segments without human intervention. Tests are part of the signal as they confirm that the code behaves as expected. Here's a basic example using JUnit:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class MathUtilTest {
@Test
public void testAdd() {
MathUtil mathUtil = new MathUtil();
assertEquals(5, mathUtil.add(2, 3));
}
}
Why This Matters: Automated testing helps mitigate noise by ensuring that any code changes don’t introduce unexpected behavior, thus enhancing the reliability and stability of your codebase.
Closing Remarks
Improving the Signal-to-Noise Ratio in your codebase is a practical and achievable goal. By refactoring regularly, embracing DRY principles, using meaningful naming conventions, writing valuable comments, applying consistent formatting, and implementing automated tests, you can significantly enhance the clarity and maintainability of your code.
Striving for a higher SNR not only contributes to better individual performance but also fosters a more efficient collaborative environment in your software development team. Start implementing these practices today, and watch your codebase transform into a cleaner, more efficient resource.
For more information on best practices in Java development, check out resources like Oracle's Java Documentation and Effective Java by Joshua Bloch. Happy coding!
Checkout our other articles