The Hidden Costs of Overusing Threads in Programming
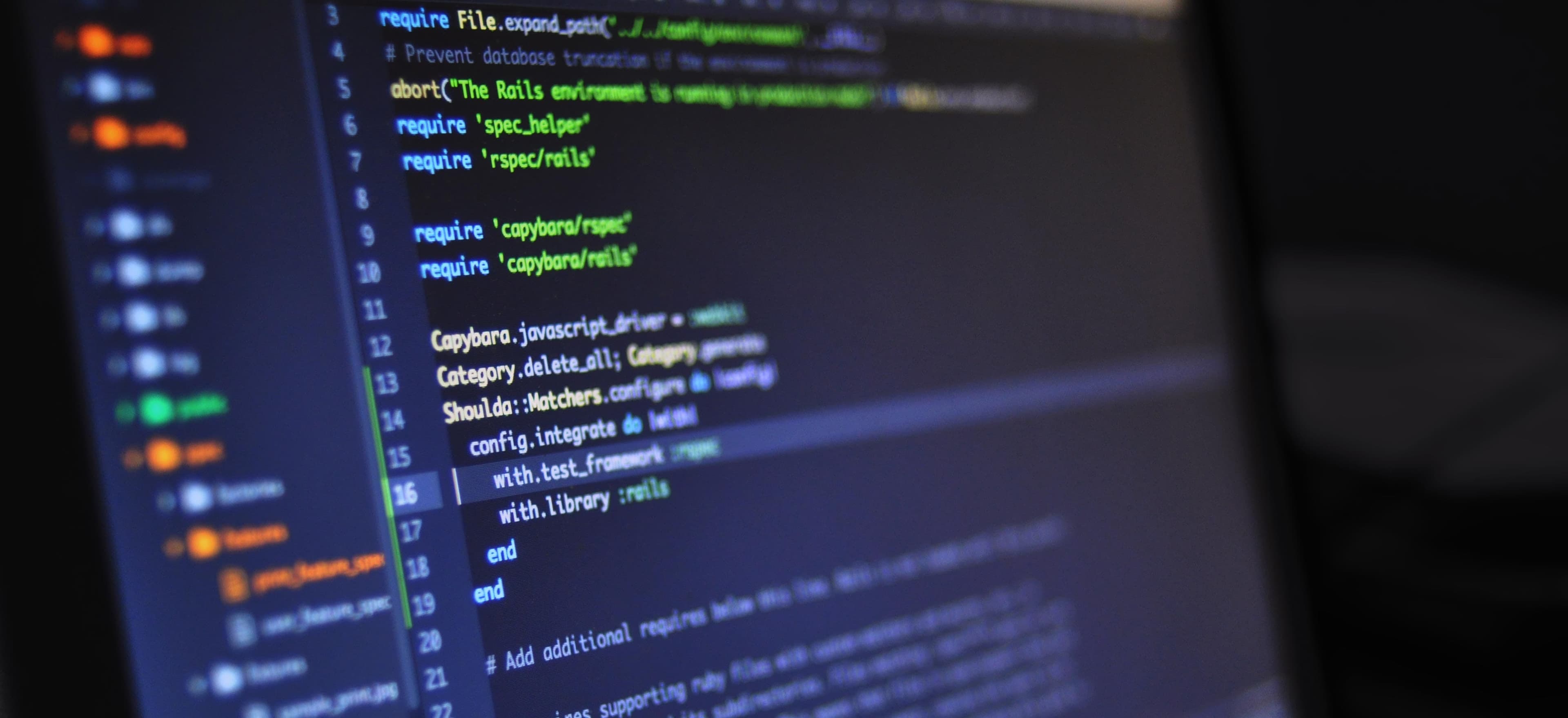
- Published on
The Hidden Costs of Overusing Threads in Programming
In recent years, multithreading has gained significant traction in software development. It is a powerful way to improve application performance by allowing multiple threads to execute concurrently. However, what many developers overlook are the hidden costs associated with overusing threads in programming. In this article, we will explore these costs and present a balanced perspective on when to use threading effectively.
Understanding Threads
A thread is the smallest unit of processing that can be scheduled by an operating system. Each process may contain multiple threads that share the same resources and memory space, enabling them to communicate with each other more efficiently than if they were separate processes. While multithreading can enhance performance, overusing threads can lead to increased complexity and resource contention, ultimately causing performance degradation.
Why Use Threads?
Before diving into the hidden costs, let's understand the scenarios where threading yields benefits:
-
Responsive User Interfaces: In applications with user interfaces, threads help keep the application responsive while executing long-running tasks in the background. This keeps the user experience smooth.
-
Parallel Processing: Tasks that can be executed concurrently can leverage multiple cores to complete in a fraction of the time, effectively reducing computational overhead.
-
I/O Operations: Threads can be particularly beneficial for handling I/O-bound operations, as they can continue executing while waiting for I/O processes to complete.
The Hidden Costs of Overusing Threads
Increased Complexity
One of the most pressing issues with multithreading is its inherent complexity. Debugging multithreaded applications can be quite challenging due to issues such as race conditions, deadlocks, and thread contention.
Example: Consider the following snippet demonstrating a simple banking application:
public class BankAccount {
private int balance;
public BankAccount(int initialBalance) {
this.balance = initialBalance;
}
public synchronized void deposit(int amount) {
balance += amount;
}
public synchronized void withdraw(int amount) {
balance -= amount;
}
public int getBalance() {
return balance;
}
}
In this code, we use the synchronized
keyword to ensure that concurrent access to the balance
variable is managed properly. However, this approach can introduce performance bottlenecks. If too many threads try to access these methods simultaneously, other threads will be blocked, waiting for their turn.
Memory Overhead
Each thread consumes system memory for its own stack. When too many threads are created, the memory consumption can escalate quickly. Each thread has its own thread stack, context data, and scheduling information, which can lead to increased memory usage and, subsequently, a risk of triggering OutOfMemoryError.
Context Switching
The operating system manages threads through a process known as context switching. This is the act of saving the state of a currently running thread and loading the state of the next thread to run. Context switching consumes CPU cycles and can diminish application performance if too many threads are active simultaneously.
For example, consider a Java application with 200 threads when the system can only efficiently handle 20 threads. The overhead introduced by managing these additional threads can impact overall performance negatively.
Thread Contention
When multiple threads attempt to acquire the same resource, they must wait. This situation—known as thread contention—can lead to suboptimal performance. High contention will require the threads to spend more time waiting than executing, significantly reducing the benefits of concurrent processing.
Example of Thread Contention:
public class Printer {
public void printJob(String document) {
System.out.println("Printing: " + document);
}
}
public class User implements Runnable {
private Printer printer;
public User(Printer printer) {
this.printer = printer;
}
public void run() {
synchronized (printer) {
printer.printJob("My Document");
}
}
}
In this example, user threads must wait to access the Printer
object's printJob
method, which can lead to a bottleneck in a highly threaded environment.
Alternatives to Overusing Threads
-
Executor Framework: Instead of manually managing threads, consider using the Executor framework, which provides a high-level abstraction over thread management.
ExecutorService executor = Executors.newFixedThreadPool(10); executor.submit(() -> { // Task goes here }); executor.shutdown();
-
Fork-Join Framework: For computational tasks that can be broken into smaller tasks, consider using the Fork-Join framework for efficient parallel computation.
-
Reactive Programming: In recent years, reactive programming using libraries like RxJava or Project Reactor has become increasingly popular. They enable developers to write non-blocking applications that can handle a large number of tasks efficiently.
To Wrap Things Up
While threads can significantly improve the performance of applications, overusing them can lead to hidden costs that often outweigh the benefits. Increased complexity, memory overhead, context-switching inefficiencies, and thread contention are just a few of the challenges faced in multithreaded programming.
To write efficient and maintainable code, focus on using threads judiciously, opting for frameworks that abstract complexity when possible.
If you would like to dive deeper into best practices in multithreading, consider visiting the Java Documentation on Concurrency or Effective Java's item on Threading.
By understanding both the benefits and costs of threading, developers can create more efficient applications while minimizing headaches associated with complex multithreaded programming. Always measure, analyze, and adjust accordingly, and remember—sometimes less is more when it comes to threads in your applications.
Checkout our other articles