Ensuring Test Reliability: The Key to Effective Testing
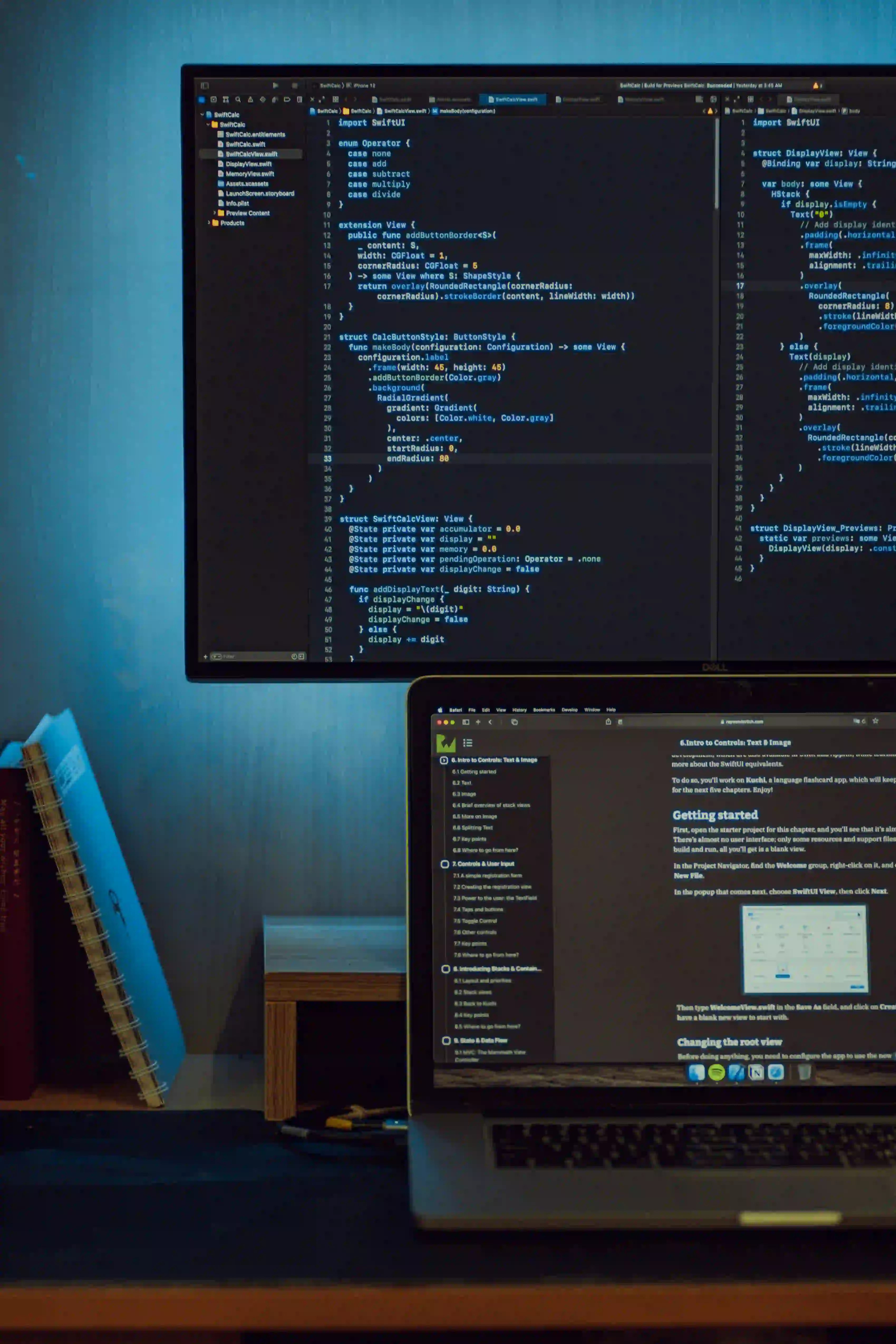
Ensuring Test Reliability: The Key to Effective Testing in Java
In today's fast-paced software development world, ensuring the reliability of your tests is paramount. Testing is not just a step in the software development lifecycle; it’s the backbone that upholds the quality of your applications. In this blog post, we delve into the significance of test reliability, discuss strategies for achieving it, and provide practical implementations in Java.
What is Test Reliability?
Test reliability refers to the consistency and stability of test results over time. Reliable tests yield the same outcomes under consistent conditions, allowing developers to trust that any failure in a test corresponds to a true defect in the code rather than an artifact of the testing process.
Why is Test Reliability Important?
-
Trustworthy Results: Reliable tests help in making informed decisions regarding code changes or releases. If a test is unreliable, it may produce false positives or negatives, leading to misguided efforts.
-
Reduced Debugging Time: When testing is reliable, developers can focus their time on resolving real issues rather than deciphering why tests are failing.
-
Improved Collaboration: A consistent testing framework fosters teamwork. All team members can confidently rely on tests, facilitating better collaboration among developers and testers.
Strategies for Ensuring Test Reliability
1. Isolate Tests
Ensuring that each test operates independently of others is crucial. Tests should not rely on the outcome or state left by previous tests.
Example:
@Test
public void testAddition() {
int result = add(2, 3);
assertEquals(5, result);
}
@Test
public void testSubtraction() {
int result = subtract(5, 2);
assertEquals(3, result);
}
Why? By structuring your tests this way, you ensure that the addition test does not accidentally get influenced by the result of the subtraction test.
2. Use Mocking
Mock objects are a powerful feature when testing, especially when dealing with external systems like databases or web services. Mocks allow you to simulate the behavior of complex objects while isolating the functionality being tested.
Example:
import static org.mockito.Mockito.*;
@Test
public void testUserServiceWithMock() {
UserRepository mockRepo = mock(UserRepository.class);
when(mockRepo.findUserById(1)).thenReturn(new User("Jane Doe"));
UserService userService = new UserService(mockRepo);
User user = userService.getUserById(1);
assertEquals("Jane Doe", user.getName());
}
Why? This approach ensures that the UserService
tests are not dependent on an actual database, which could introduce variability and external factors into tests.
3. Maintain Test Data Consistency
It’s critical to ensure test data is set up in a predictable manner. Use fixtures or setup methods to prepare the state before each test.
Example:
@Before
public void setUp() {
// Initialize test data
database.add(new User(1, "Alice"));
database.add(new User(2, "Bob"));
}
@Test
public void testFindUserById() {
User user = userService.findUserById(1);
assertEquals("Alice", user.getName());
}
Why? The @Before
annotation sets up a consistent state before every test runs, eliminating inconsistencies from data modifications made by previous tests.
4. Use Parameterized Tests
To further enhance reliability, consider using parameterized tests that allow you to run the same test logic with different inputs. This avoids redundancy in your test cases and ensures more exhaustive coverage.
Example:
@RunWith(Parameterized.class)
public class CalculatorTest {
private int a;
private int b;
private int expectedResult;
public CalculatorTest(int a, int b, int expectedResult) {
this.a = a;
this.b = b;
this.expectedResult = expectedResult;
}
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][]{
{1, 1, 2},
{2, 3, 5},
{3, 5, 8}
});
}
@Test
public void testAdd() {
assertEquals(expectedResult, add(a, b));
}
}
Why? This approach reduces the boilerplate code by grouping multiple similar cases under a single test method while ensuring that all scenarios are covered.
5. Continuous Integration
Implement a Continuous Integration (CI) system that runs your tests automatically on different environments. CI serves to further validate the reliability of your tests through regular execution and monitoring.
Why? Regular execution in varied environments can prevent tests from becoming stale and ensures compatibility across different platforms and setups.
Bringing It All Together
Ensuring test reliability is integral to maintaining a robust software application. By employing strategies such as isolating tests, effective use of mocking, maintaining test data, using parameterized tests, and integrating CI practices, Java developers can build a trustworthy testing landscape.
The road to reliable testing requires diligence and continuous improvement. As you refine these practices, you'll not only enhance the quality of your tests but also empower your team and overall project success.
For further reading on reliable testing practices, consider exploring the resources listed below:
By prioritizing test reliability, you ensure that every build is a step towards producing not only functional software but also a stress-free development environment for your team. Happy coding!