Overcoming Common Pitfalls in Object-Oriented Programming
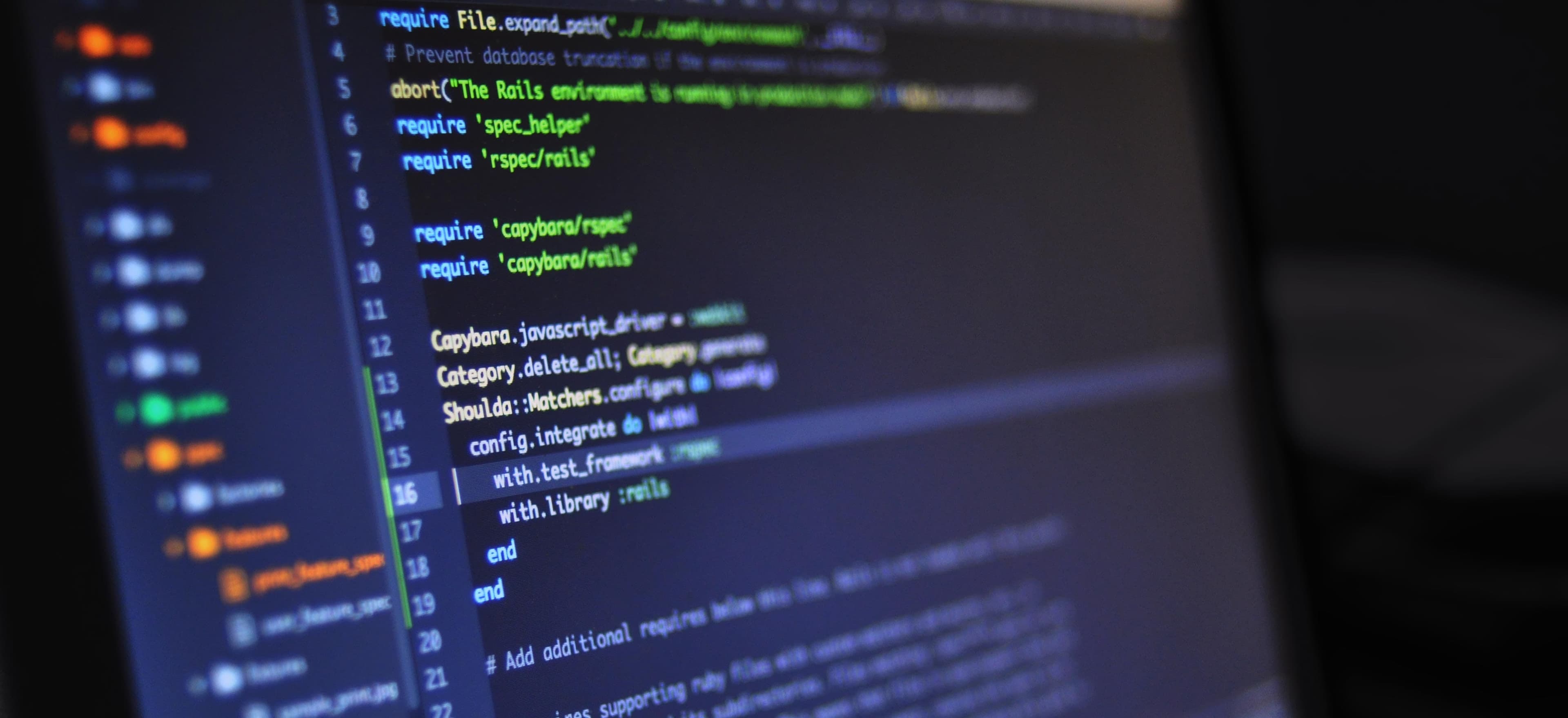
- Published on
Overcoming Common Pitfalls in Object-Oriented Programming
Object-Oriented Programming (OOP) is a powerful paradigm that encourages organized and reusable code. However, many developers, especially those new to OOP, can stumble upon common pitfalls. This blog post will explore these issues and provide strategies for overcoming them, ensuring you harness the full potential of OOP in Java.
Understanding Object-Oriented Programming Concepts
Before diving into pitfalls, let's briefly revisit the core concepts of OOP:
-
Encapsulation: Bundling data with the methods that operate on that data. This hides the internal state and requires all interaction to occur through an object's methods.
-
Inheritance: Mechanism where a new class inherits properties and behavior from an existing class, promoting code reusability.
-
Polymorphism: Ability of different classes to be treated as instances of the same class through a common interface. This allows methods to do different things based on the object it is acting upon.
-
Abstraction: Hiding complex implementation details and exposing only the necessary parts of an object.
Understanding these concepts forms a strong foundation for exploring their pitfalls.
Common Pitfalls in Object-Oriented Programming
1. Over-Engineering
One of the most frequent mistakes in OOP is over-engineering a solution. New developers often feel compelled to implement multiple classes and interfaces, even when a simpler solution suffices.
Solution
Start simple. Embrace the YAGNI principle ("You Aren't Gonna Need It"). Begin with a straightforward implementation, and refactor only when necessary to avoid complexity. For instance:
// A simple class instead of multiple classes implementing complicated patterns
public class User {
private String username;
private String email;
public User(String username, String email) {
this.username = username;
this.email = email;
}
// Getter methods
public String getUsername() { return username; }
public String getEmail() { return email; }
}
This approach promotes code readability. You can always refactor if your requirements evolve.
2. Misusing Inheritance
Inheritance is a powerful tool but also a double-edged sword. Misusing inheritance can lead to fragile code and tight coupling between classes.
Solution
Prefer composition over inheritance. This means instead of inheriting behavior from a parent class, use it as a member of another class. For example:
// Instead of inheritance, we use composition
public class Order {
private PaymentStrategy paymentStrategy;
public Order(PaymentStrategy paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
public void processPayment() {
paymentStrategy.pay();
}
}
// PaymentStrategy can be an interface, and we can have multiple implementations
public interface PaymentStrategy {
void pay();
}
public class CreditCardPayment implements PaymentStrategy {
public void pay() {
// Implementation for credit card payment
}
}
In this example, Order
does not extend PaymentStrategy
, reducing tight coupling and allowing for greater flexibility.
3. Ignoring Encapsulation
Encapsulation is essential for building robust applications. By exposing internal state directly, you risk external modification that can lead to inconsistent states.
Solution
Use access modifiers wisely. Keep fields private and expose them through public methods. For instance:
public class Student {
private String id;
public Student(String id) {
this.id = id;
}
public String getId() { return id; }
}
By making id
private, you control access and modifications, enhancing security and data integrity.
4. Abusing Polymorphism
Polymorphism can simplify code but can also lead to misunderstandings when overused or used inappropriately. When developers heavily rely on dynamic polymorphism, code readability can suffer.
Solution
Limit the use of polymorphism where appropriate. For example, do not force a class hierarchy when it adds no value. Instead, favor interfaces for shared behavior where it enhances clarity:
public interface Shape {
double area();
}
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double area() {
return Math.PI * radius * radius;
}
}
public class Rectangle implements Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double area() {
return width * height;
}
}
Here, both Circle
and Rectangle
implement the Shape
interface, adhering to the Open/Closed principle. It enhances maintainability and clarity.
5. Tight Coupling
Tight coupling among classes makes your codebase rigid, complicating testing and enhancing the chance for errors during changes or updates.
Solution
Use the Dependency Injection (DI) pattern. DI promotes loose coupling and increases testability by injecting dependencies rather than hard-coding them.
public class EmailService {
public void sendEmail(String message) {
// Implementation
}
}
public class Notification {
private final EmailService emailService;
public Notification(EmailService emailService) {
this.emailService = emailService;
}
public void notifyUser(String message) {
emailService.sendEmail(message);
}
}
In this setup, the Notification
class is not responsible for creating the EmailService
. Instead, it receives it, allowing easier testing and modification.
6. Failing to Implement SOLID Principles
The SOLID principles are five design principles intended to make software designs more understandable, flexible, and maintainable. Ignoring these principles can lead to complicated and rigid systems.
Solution
Familiarize yourself with and apply SOLID principles:
- Single Responsibility Principle (SRP): A class should have only one reason to change.
- Open-Closed Principle (OCP): Software entities should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): Subtypes must be substitutable for their base types.
- Interface Segregation Principle (ISP): No client should be forced to depend on methods it does not use.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules. Both should depend on abstractions.
By adhering to these principles, your projects will become easier to manage and extend.
The Bottom Line
Object-Oriented Programming is a robust paradigm that, when used correctly, can dramatically improve the quality of software design. By being cognizant of common pitfalls such as over-engineering, misuse of inheritance, failure to encapsulate data, abusing polymorphism, maintaining tight coupling, and neglecting SOLID principles, you can create clean, maintainable, and efficient Java code.
For further reading on OOP principles, consider exploring Java Programming by Oracle and Effective Java by Joshua Bloch. The journey to mastering OOP in Java is ongoing, but with mindful practice and a clear understanding of common pitfalls, you are well on your way to becoming an exceptional Java developer.
Happy coding!
Checkout our other articles