Optimizing Performance: JIT Compiler Pitfalls in HotSpot
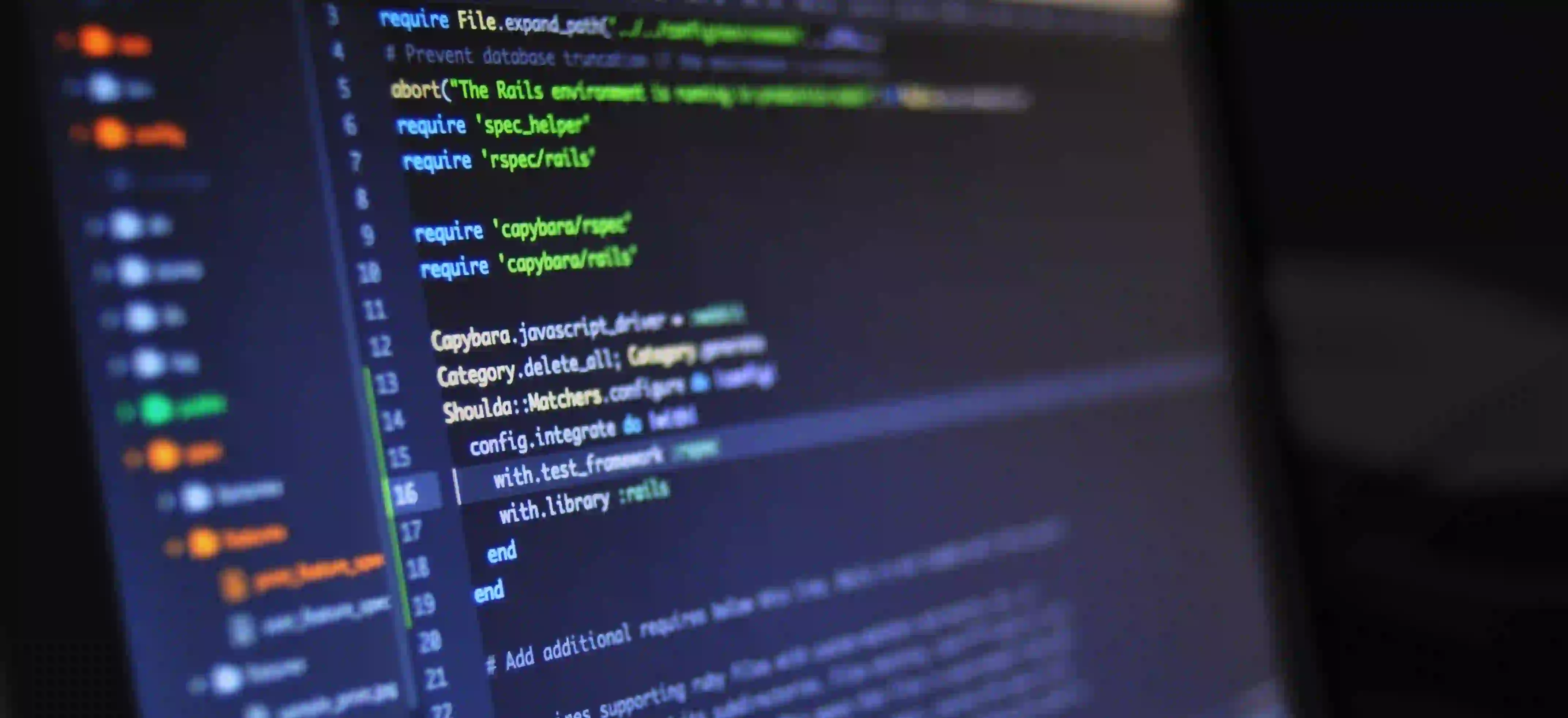
Optimizing Performance: JIT Compiler Pitfalls in HotSpot
The Java Virtual Machine (JVM) has its own mechanisms to enhance performance through Just-In-Time (JIT) compilation. HotSpot is the most widely used JVM and offers remarkable features to optimize execution speed. However, there are pitfalls associated with JIT compilation that can affect performance. In this blog post, we’ll delve into what HotSpot JIT compilation is, discuss its potential pitfalls, and provide solutions to mitigate these issues.
Understanding JIT Compilation
JIT compilation is a feature of the JVM that translates Java bytecode into native machine code at runtime. This approach allows frequently executed code paths to achieve performance akin to that of compiled languages like C or C++.
How Does It Work?
When a Java program runs, the JVM interprets bytecode initially, which is relatively slow. As the application executes, the JVM monitors the code paths that are executed most frequently. The JIT compiler steps in to optimize these "hot" methods by converting them into native code.
Example
public class JITDemo {
public static void main(String[] args) {
for (int i = 0; i < 10_000_000; i++) {
System.out.println(compute(i));
}
}
public static int compute(int value) {
return value * value;
}
}
In this example, the compute
method could be identified as a hot method if it is called frequently, allowing JIT compilation to take over, optimizing its execution.
Pitfalls of JIT Compilation
While JIT can greatly optimize performance, certain pitfalls can arise, negatively impacting execution speed and resource consumption. Let’s examine these pitfalls in detail.
1. Warm-Up Time
The most notable pitfall of JIT compilation is the warm-up time. When an application begins execution, the JIT compiler has to collect profiling data to identify hot spots, which means that the application might run slower initially.
Solution
To overcome this issue, applications can utilize benchmarks to measure performance over time. Techniques like pre-warming the JVM by running key methods can also be beneficial.
2. JIT Inlining Limitations
JIT compilers often utilize inlining — substituting a method call with the body of the method itself. This can improve performance but can also lead to excessive memory usage if overdone.
Example
public class InliningDemo {
public static void main(String[] args) {
for (int i = 0; i < 5_000_000; i++) {
new InliningDemo().performOperation(i);
}
}
public void performOperation(int value) {
System.out.println(complexOperation(value));
}
private int complexOperation(int value) {
// Perform an expensive operation
return value * value + value * 10;
}
}
In this case, if complexOperation
is marked for inlining, it leads to a significant memory footprint due to the increased size of native code.
Solution
Developers can manage inlining through JVM flags, for instance, adjusting -XX:MaxInlineSize
to avoid excessive memory use without losing the performance benefits from inlining.
3. Profiling Overhead
JIT compilation introduces profiling overhead, which can consume CPU cycles. The overhead occurs due to the need for the JVM to keeps track of method invocations and compile hot spots.
Solution
Choose performance tuning wisely. Always profile the application to understand where the bottlenecks lie. Tools like VisualVM can help visualize performance metrics without much overhead on the application.
4. Deoptimization: A Hidden Cost
The JIT compiler can decide to deoptimize code, reverting it back to interpreted state when runtime conditions change, for example, if assumptions about the execution context are invalidated.
Example
Consider a method that was optimized across certain conditions:
public class DeoptDemo {
public static void method(String arg) {
// Assuming arg is always a specific value
if ("optimized".equals(arg)) {
optimize();
}
}
public static void optimize() {
// Some optimized code execution
System.out.println("Optimized execution!");
}
}
If the method is later called with a different argument, it may trigger deoptimization, incurring a performance cost.
Solution
Utilize profiling data judiciously. Keeping the codebase simple and maintaining predictable path conditions will reduce the likelihood of deoptimization.
5. Garbage Collection Pressure
HotSpot's sophisticated garbage collection (GC) differs based on memory allocation patterns, which can interfere with JIT-compiled code's execution efficiency. Larger heap sizes can lead to more frequent GCs.
Solution
Monitor memory usage to ensure your application isn’t allocating unnecessary memory. Tuning JVM garbage collection parameters via flags like -Xmx
and -Xms
can help strike a balance between performance and memory overhead.
In Conclusion, Here is What Matters
JIT compilation in HotSpot enhances Java performance but comes with its set of pitfalls, including warm-up time, inlining issues, profiling overhead, deoptimization, and garbage collection pressure.
By understanding these challenges and ensuring proper optimization techniques, Java developers can unlock the full power of the JIT compiler while mitigating its downsides. Continuous benchmarking and tuning during development will lead to improved Java application performance.
For more detailed strategies on JVM tuning and performance optimization, visit the Java Performance Tuning Documentation and explore Common Performance Pitfalls.
By being mindful of these pitfalls and adopting solid coding practices, your Java applications can achieve exceptional performance, paving the way for a responsive and efficient user experience. Happy coding!