Mastering StringJoiner: Avoid Common Pitfalls in Java 8
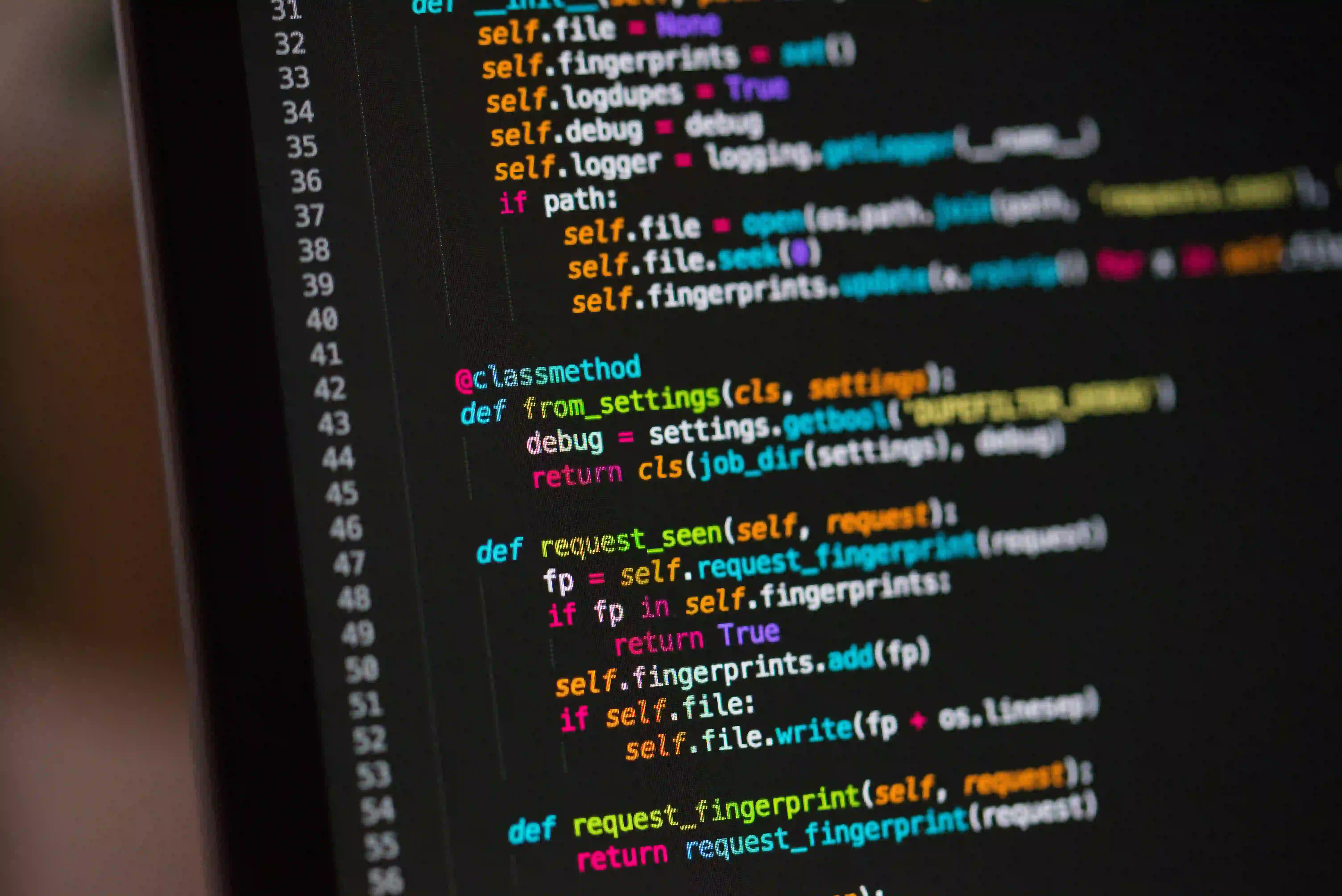
Mastering StringJoiner: Avoid Common Pitfalls in Java 8
In the Java programming landscape, manipulating strings effectively is a fundamental skill that every developer should master. Java 8 introduced many features that revolutionized the way we work with data. One of these invaluable features is StringJoiner
. In this blog post, we will explore the uses of StringJoiner
, discuss common pitfalls, and provide practical examples to give you a comprehensive understanding of this powerful utility.
What is StringJoiner?
StringJoiner
is available in the java.util
package and is designed to construct strings by combining multiple strings with a specified delimiter, prefix, and suffix. This feature is particularly beneficial when you need to format the output of a collection or an array in a readable or specific manner.
Basic Structure of StringJoiner
import java.util.StringJoiner;
public class StringJoinerExample {
public static void main(String[] args) {
StringJoiner joiner = new StringJoiner(", ", "[", "]");
joiner.add("Apple").add("Banana").add("Cherry");
System.out.println(joiner); // Output: [Apple, Banana, Cherry]
}
}
In this example, we create a StringJoiner
that joins elements with a comma delimiter, prepended by a "[" and appended by a "]". This results in a well-structured output.
Key Features of StringJoiner
- Delimiter: It defines what separates the joined strings.
- Prefix: It is an optional string that will be added at the beginning.
- Suffix: It is an optional string that will be added at the end.
These features allow developers to format strings elegantly without cumbersome concatenation, particularly valuable when dealing with collections or arrays.
Benefits of Using StringJoiner
- Readability: Code using
StringJoiner
is simpler and easier to read. - Less Error-Prone: By using object-oriented construction, the potential for errors is reduced compared to manual concatenation.
- Performance: For concatenating many strings,
StringJoiner
can be more efficient than traditional methods like usingStringBuilder
.
Common Pitfalls When Using StringJoiner
Even though StringJoiner
simplifies string manipulation, there are still common pitfalls that developers may encounter. Let's look at some of these and how to avoid them.
1. Forgetting the Prefix and Suffix
A common mistake is neglecting to specify a prefix or suffix when necessary. This can lead to output that may not meet your formatting goals.
Example of This Pitfall:
StringJoiner joiner = new StringJoiner(", "); // No prefix or suffix
joiner.add("One").add("Two").add("Three");
System.out.println(joiner); // Output: One, Two, Three
In scenarios where you need output for JSON or array strings, forgetting the prefix and suffix could create a less readable format.
Resolution:
Always specify the prefix and suffix when the context requires it. For instance:
StringJoiner joiner = new StringJoiner(", ", "[", "]");
2. Changing the Delimiter After Initialization
Once a StringJoiner
is created, attempting to change its delimiter is not possible. This can lead to unexpected behavior if any alteration is mistakenly invoked.
Example of This Pitfall:
StringJoiner joiner = new StringJoiner(", ");
joiner.setEmptyValue("No Elements"); // Incorrectly assumes it can change the delimiter
Resolution:
Create a new instance of StringJoiner
if a different delimiter is needed, such as:
StringJoiner joinerWithSemiColon = new StringJoiner("; ");
3. Using add() Method Without Prior Initialization
Before using the add()
method, you must ensure that the StringJoiner
is properly initialized. Attempting to add elements without specifying the delimiter, prefix, or suffix can lead to unformatted results.
Example of This Pitfall:
StringJoiner joiner = new StringJoiner(", ");
joiner.add("One").add("Two");
System.out.println(joiner); // Output: One, Two
Suppose you mistakenly assume it’s initialized with a prefix and suffix. In that case, your output will be unadorned when you require formatted data.
Resolution:
Always double-check that you are initializing the StringJoiner
correctly:
StringJoiner joiner = new StringJoiner(", ", "[", "]");
4. Ignoring setEmptyValue()
StringJoiner
has a method called setEmptyValue()
that sets a string to be used when no elements are added to the joiner. Failing to use this can lead to outputs that default to an empty string, which might be confusing or undesirable.
Example of This Pitfall:
StringJoiner joiner = new StringJoiner(", ");
String result = joiner.toString(); // Output: ""
Resolution:
Implement the setEmptyValue()
method to provide meaningful output:
StringJoiner joiner = new StringJoiner(", ");
joiner.setEmptyValue("No Elements");
System.out.println(joiner); // Output: No Elements
Practical Use-Cases of StringJoiner
- Building CSV Strings: Create a string for CSV output.
- Combining URL Parameters: Concatenate key-value pairs for queries.
- Formatted Data Outputs: Generate formatted strings for logging or user interfaces.
Example: Building a CSV String
import java.util.StringJoiner;
public class CsvBuilder {
public static void main(String[] args) {
StringJoiner csvJoiner = new StringJoiner(", ");
csvJoiner.add("Name").add("Age").add("Country");
System.out.println(csvJoiner.toString()); // Output: Name, Age, Country
}
}
Example: Creating URL Parameters
import java.util.StringJoiner;
import java.util.Map;
public class UrlParameterExample {
public static void main(String[] args) {
StringJoiner urlJoiner = new StringJoiner("&", "?", "");
Map<String, String> params = Map.of("name", "John", "age", "30", "city", "NewYork");
params.forEach((key, value) -> urlJoiner.add(key + "=" + value));
System.out.println(urlJoiner); // Output: ?name=John&age=30&city=NewYork
}
}
Lessons Learned
Java's StringJoiner
is an elegant solution for managing string concatenation with clear parameters for delimiters, prefixes, and suffixes. By understanding and avoiding common pitfalls—like forgetting to set formats or alter properties improperly—you can leverage the full potential of this utility.
Mastering StringJoiner
not only enhances code clarity but also improves performance. Consider incorporating this into your toolkit, especially for tasks involving complex string manipulations.
Further Reading
For more information about managing strings in Java, check out these resources:
By embracing the tools and techniques discussed here, you'll be well on your way to mastering string manipulation in Java!