Mastering Non-Linear Data Structures: Common Pitfalls
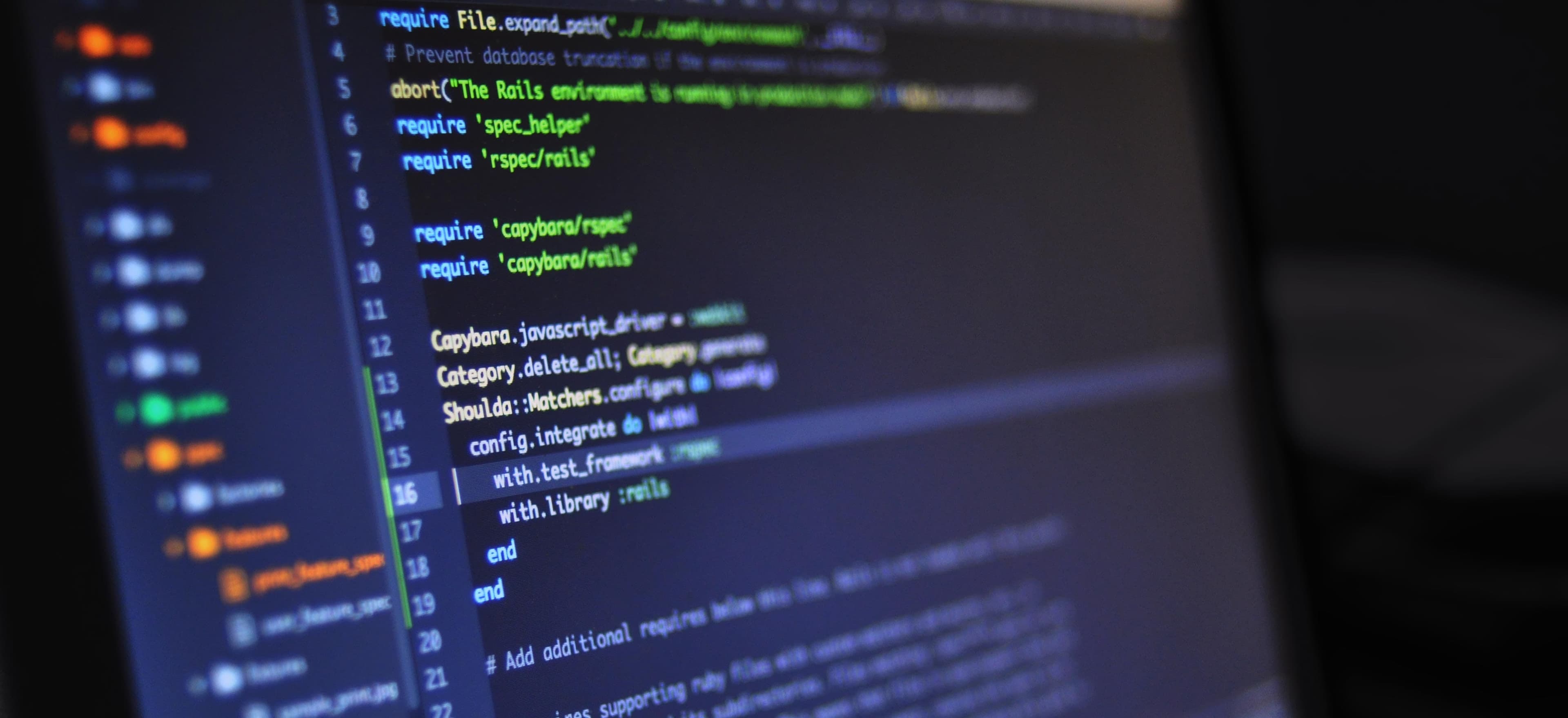
- Published on
Mastering Non-Linear Data Structures: Common Pitfalls
Non-linear data structures play an essential role in optimizing complex data interactions and relationships. Unlike linear structures, such as arrays and linked lists, non-linear structures like trees and graphs can manage data in a more hierarchical and interconnected way. In this blog post, we will explore some common pitfalls when working with non-linear data structures, particularly focusing on trees and graphs, supplemented by relevant Java code snippets.
Understanding Non-Linear Data Structures
Before diving into pitfalls, it's crucial to understand what we mean by non-linear data structures. Non-linear structures do not arrange data in a sequential manner. Instead, they allow for more intricate relationships, often leading to more efficient data handling.
Trees
A tree is a hierarchical structure composed of nodes, where each node is connected by edges. It presents a parent-child relationship, making it perfect for organizing sorted data and representing hierarchical relationships (like file directories).
Graphs
Graphs are even more flexible, forming connections (edges) between nodes (vertices) in various ways, leading to a multitude of paths and relationships. They are ideal for scenarios such as social networks, transportation systems, and more.
Common Pitfalls
1. Ignoring the Choice of Data Structure
One of the most frequent mistakes developers make is not choosing the right data structure for the given problem. When dealing with relationships, a graph is often more suited than a tree.
Example: When to Use a Graph
If your application requires tracking relationships between entities, such as in social network applications, using a graph is more effective than a tree.
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
class Graph {
private final Map<String, Set<String>> adjacencyList;
public Graph() {
adjacencyList = new HashMap<>();
}
public void addVertex(String vertex) {
adjacencyList.putIfAbsent(vertex, new HashSet<>());
}
public void addEdge(String from, String to) {
adjacencyList.get(from).add(to);
adjacencyList.get(to).add(from); // If it's an undirected graph
}
}
In this code, each vertex manages its connections through an adjacency list. This structure allows for efficient traversal and manipulation of relationships. Misusing structures can lead to slower performance and increased complexity.
2. Not Implementing Proper Traversal
Traversal methods like Breadth-First Search (BFS) and Depth-First Search (DFS) are critical for efficiently exploring data in trees and graphs. Not implementing these correctly can lead to sluggish performance and even stack overflow errors in the case of DFS when working with deep structures.
Example: DFS Implementation
import java.util.HashSet;
import java.util.Stack;
class GraphDFS {
private final Map<String, Set<String>> adjacencyList;
public GraphDFS(Map<String, Set<String>> adjacencyList) {
this.adjacencyList = adjacencyList;
}
public void depthFirstSearch(String start) {
Set<String> visited = new HashSet<>();
Stack<String> stack = new Stack<>();
stack.push(start);
while (!stack.isEmpty()) {
String vertex = stack.pop();
if (!visited.contains(vertex)) {
System.out.println(vertex);
visited.add(vertex);
stack.addAll(adjacencyList.get(vertex));
}
}
}
}
In this context, if one does not manage the visited nodes properly, it may cause infinite loops, particularly in cyclic graphs. The key takeaway here is the necessity for careful traversal management.
3. Neglecting Memory Management
Transforming from linear to non-linear data structures often introduces the complexity of increased memory usage. Particularly in recursive structures like trees, failure to analyze or manage memory could cause your application to crash.
Example: Managing Node Memory
class TreeNode {
int value;
TreeNode left;
TreeNode right;
public TreeNode(int value) {
this.value = value;
this.left = null;
this.right = null;
}
// Memory optimization could involve various strategies
public void clear() {
this.left = null;
this.right = null;
}
}
In this code, the clear
method provides a simple way to nullify references, which can aid the garbage collector in reclaiming memory. Always keep memory management in mind, especially in larger applications.
4. Failing to Consider Edge Cases
In the realm of non-linear data structures, edge cases often arise which developers overlook. Understanding how your structure behaves with extreme inputs is necessary for robust application development.
Example: Handling Empty Structures
public void printTree(TreeNode node) {
if (node == null) {
System.out.println("The tree is empty.");
return;
}
// Continue with the tree traversal
}
Not handling these conditions can lead to unhandled exceptions and negatively impact user experience. Rigorous testing of diverse scenarios is a fundamental aspect of developing reliable applications.
5. Overcomplicating the Code
Another error is over-engineering your data structures. Often, developers add layers of complexity unnecessarily, which can make code harder to read and maintain.
Example: Minimizing Complexity
class SimpleTree {
int value;
List<SimpleTree> children;
public SimpleTree(int value) {
this.value = value;
this.children = new ArrayList<>();
}
public void addChild(SimpleTree child) {
this.children.add(child);
}
}
This straightforward implementation offers clarity while maintaining functionality. Simple designs are generally more effective than convoluted class structures.
Key Takeaways
Navigating the complexities of non-linear data structures is a challenging yet rewarding journey. Understanding common pitfalls, such as failing to choose the appropriate structure, neglecting traversal techniques, and poor memory management, can significantly enhance your coding proficiency.
For more in-depth knowledge, you might want to check GeeksforGeeks on Data Structures and The Java Tutorials which cover various data structures in Java comprehensively.
By being mindful of these challenges and equipping yourself with the right strategies, you can master non-linear data structures and enhance your Java programming skills significantly. Happy coding!
Checkout our other articles