Common Pitfalls When Using Java's Random in Java 8
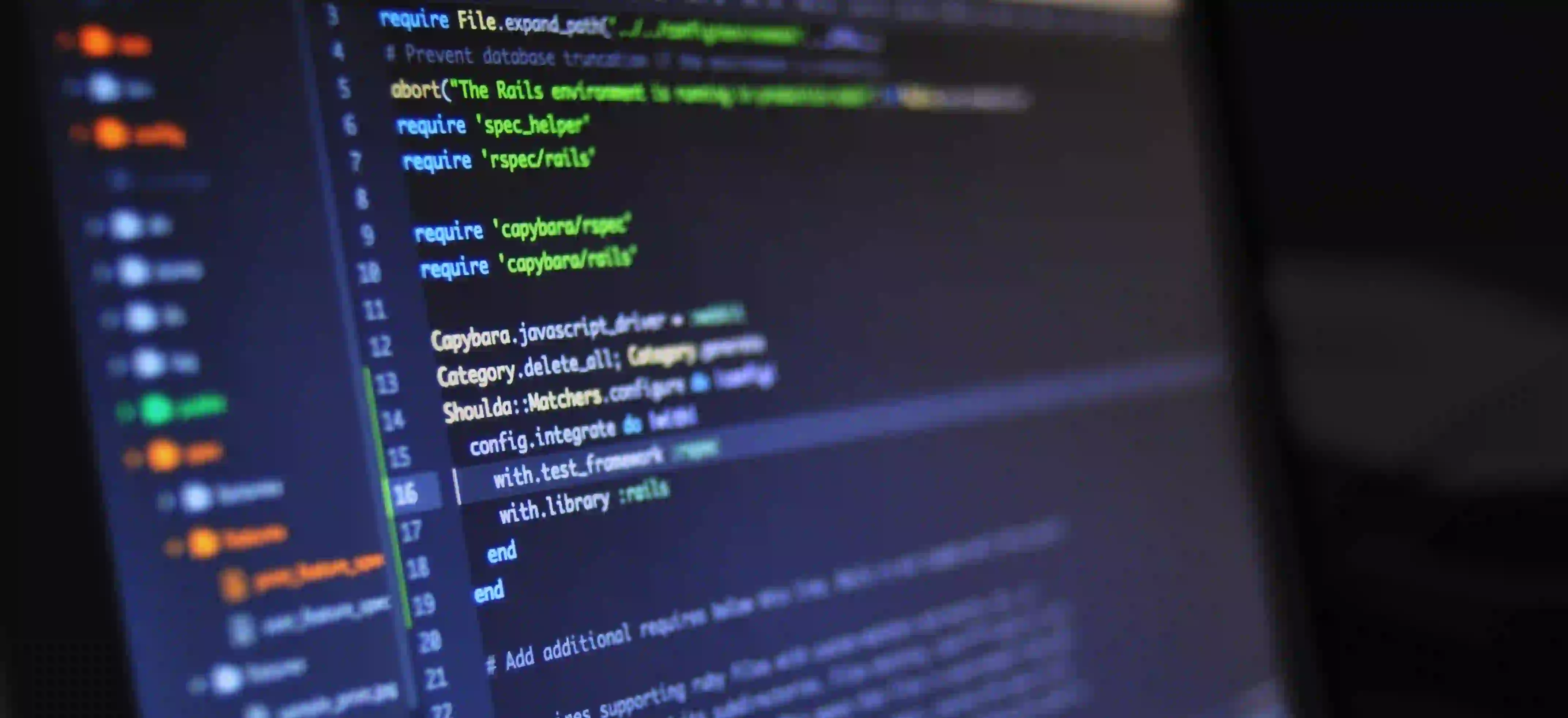
Common Pitfalls When Using Java's Random in Java 8
Java has a robust ecosystem, and since being added in Java 1.0, the java.util.Random
class has been a widely used utility for generating random numbers. However, there are some common pitfalls when using it, especially with the features introduced in Java 8. This blog post will explore these pitfalls, how to avoid them, and best practices for effectively using the Random
class.
The Basics of Java's Random Class
Before diving into pitfalls, let's clarify what the Random
class does. It provides methods to generate random numbers in various integer types, floats, and even booleans.
For instance, you can create an instance of Random
as follows:
import java.util.Random;
public class RandomDemo {
public static void main(String[] args) {
Random rand = new Random();
int randomInt = rand.nextInt(100); // Generates an integer between 0 (inclusive) and 100 (exclusive)
System.out.println("Random Integer: " + randomInt);
}
}
Why Use Random?
Using Random
is crucial, especially in applications that require unpredictability, such as games, simulations, or cryptography. However, care must be taken to use it properly.
Common Pitfalls
1. Not Using a Seed to Ensure Reproducibility
One of the most common pitfalls when using Random
is overlooking the importance of seeding. If you run the same program multiple times without a seed, you could end up with the same sequence of random numbers.
Random rand1 = new Random(); // Implicitly seeded based on current time
Random rand2 = new Random(); // Another instance with the "same" seed if invoked closely in time
Solution: Always provide an explicit seed, especially during testing.
Random rand = new Random(42); // Makes generated values reproducible
System.out.println("Random Integer with Seed: " + rand.nextInt(100));
2. Thread Safety Issues
Java's Random
is not thread-safe, which poses a problem when used in concurrent environments. Multiple threads using the same Random
object can lead to race conditions, resulting in duplicate or missing values.
Alternative: Use ThreadLocalRandom
, which is thread-safe and designed specifically for concurrent use.
import java.util.concurrent.ThreadLocalRandom;
public class ThreadSafeRandom {
public static void main(String[] args) {
// Each thread can safely use its own instance of ThreadLocalRandom
int randomInt = ThreadLocalRandom.current().nextInt(100);
System.out.println("Thread-safe Random Integer: " + randomInt);
}
}
3. Poor Distribution of Values
Another point of concern is that naive implementations of Random
might generate overlapping values or fail to provide a sufficient distribution of numbers in certain ranges.
Example of Poor Range Usage:
Random rand = new Random();
for (int i = 0; i < 10; i++) {
System.out.println(rand.nextInt(50)); // Limited range
}
Better Practice: Ensure that your intended distribution aligns with your application's needs.
int low = 1; // minimum
int high = 50; // maximum
int randomNumberInRange = low + rand.nextInt(high - low);
System.out.println("Better Distributed Random Number: " + randomNumberInRange);
4. Implicitly Understanding Pseudo-Randomness
Random
generates pseudo-random numbers, which means that if you know the seed, you can predict the numbers generated thereafter. This can be a security risk in applications that require high randomness, such as cryptographic applications.
Best Practice: For cryptographic applications, use SecureRandom
instead.
import java.security.SecureRandom;
public class SecureRandomExample {
public static void main(String[] args) {
SecureRandom secureRand = new SecureRandom();
int secureRandomNumber = secureRand.nextInt(100);
System.out.println("Secure Random Integer: " + secureRandomNumber);
}
}
5. Overnoising With Randomization
Another common pitfall is overusing randomization in an attempt to increase unpredictability. Sometimes, using randomness excessively can introduce more noise than intended into the application.
Instead of using random values carelessly, it is advisable to have a clear strategy for randomness, such as controlling when and how often to generate random values.
// Example of overzealous random number generation
for (int i = 0; i < 10; i++) {
System.out.println(Math.random()); // Generates a random number each loop
}
More Controlled Usage: Only generate random numbers when necessary.
int eventCounter = 0;
for (int i = 0; i < 100; i++) {
if (shouldGenerateRandom()) {
System.out.println(Math.random());
eventCounter++;
}
}
System.out.println("Total Random Events: " + eventCounter);
Bringing It All Together
Java's Random
class is a powerful tool when used properly. By being aware of common pitfalls, you can ensure that its use enhances your application rather than detracting from its functionality.
For further reading, consider checking out the official Java documentation on java.util.Random and SecureRandom.
Always remember: in the world of randomness, being deliberate about your choices makes all the difference. Happy coding!