Troubleshooting UTF-8 Encoding Issues in Eclipse
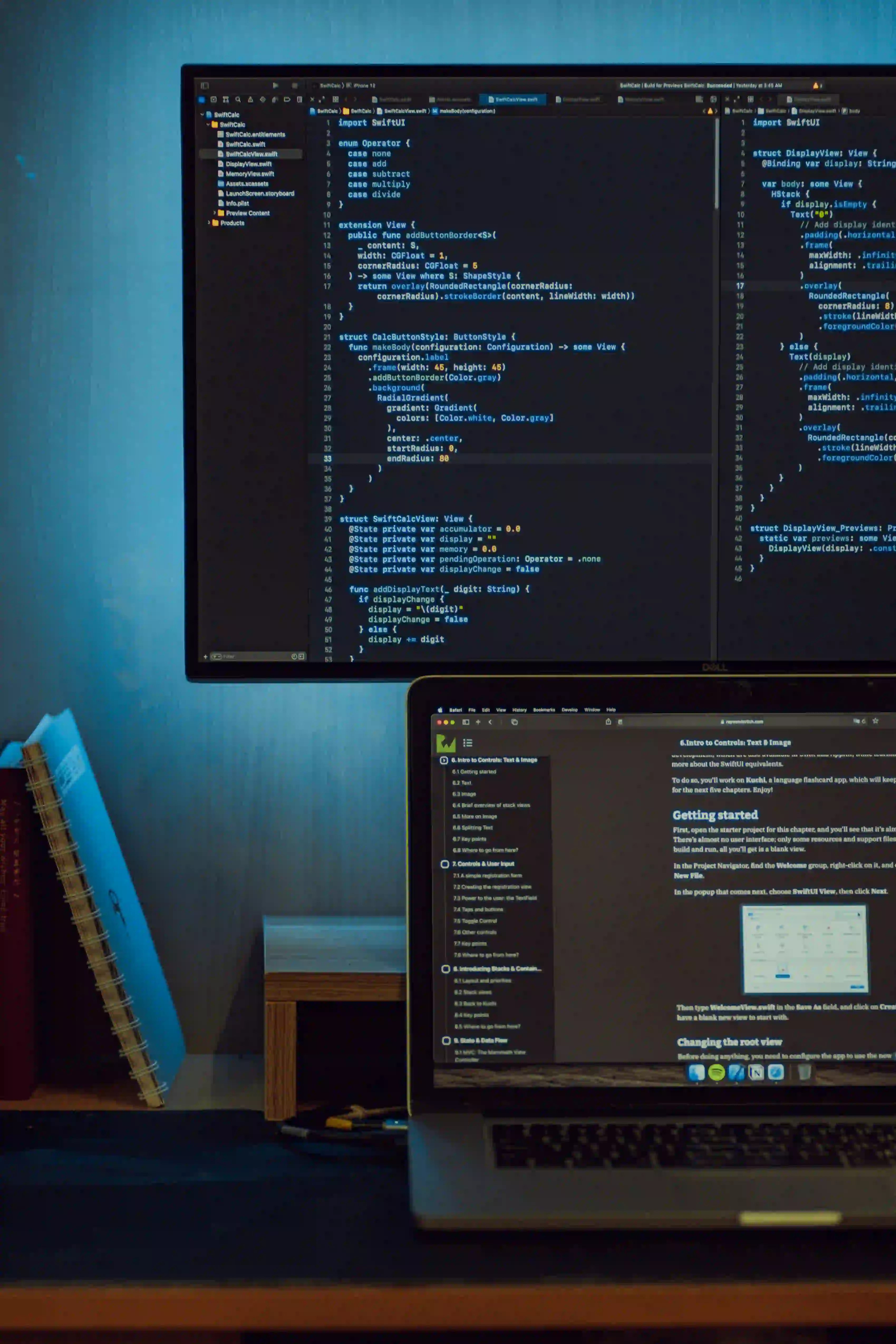
Troubleshooting UTF-8 Encoding Issues in Eclipse
When developing software, one of the common challenges you might encounter is character encoding, particularly when you're dealing with internationalization or special characters. UTF-8 is the most widely-used encoding for web content, ensuring that text is represented accurately across different systems. However, when using an IDE like Eclipse, you may face issues caused by misconfigured settings. This blog post will walk you through troubleshooting UTF-8 encoding issues in Eclipse, providing solutions and examples to help you maintain character integrity in your Java applications.
Understanding UTF-8 Encoding
Before diving into troubleshooting, it is crucial to understand what UTF-8 encoding is and why it matters.
- UTF-8: A variable-width character encoding that can represent every character in the Unicode character set. This allows for the correct representation of characters from various languages and symbols.
If your Java application handles text (especially that includes international characters), ensuring UTF-8 encoding is critical to avoid problems such as garbled text, missing characters, or runtime exceptions.
Common Signs of UTF-8 Encoding Issues
- Garbled Characters: When reading from or writing to files, characters may not display as intended.
- Compile Errors: Some characters may trigger unexpected compile errors.
- Database Issues: Characters stored in databases may not display correctly when retrieved.
Configuring Eclipse for UTF-8 Support
Global Workspace Encoding
To resolve encoding issues, you should configure your workspace to use UTF-8 by default.
- Open Eclipse.
- Navigate to Window > Preferences.
- Expand the General section.
- Click on Workspace.
- Set the Text file encoding to UTF-8.
Here’s a quick visual walkthrough:
!Eclipse Preferences.
Project-Specific Encoding
Each Eclipse project can maintain its encoding settings, which may override the workspace encoding.
- Right-click the project in the Package Explorer.
- Choose Properties.
- Click on Resource in the left panel.
- Set Text file encoding to UTF-8.
Editor Settings
Ensure your editors are also configured to handle UTF-8:
- Open Window > Preferences.
- Go to General > Editors > Text Editors.
- Check the Encoding setting and select UTF-8.
Handling Source Code Files
If your source code files themselves possess a different encoding, it is essential to convert them.
Converting Existing Files to UTF-8
- Open the file in Eclipse.
- Right-click on the file in the Package Explorer and select Properties.
- Under Resources, change the Text file encoding to UTF-8.
- Confirm any prompts to convert the file.
Code Example: Handling UTF-8 in Java
When reading from or writing to files in Java, specify the encoding explicitly. Here’s how to read a UTF-8 encoded file:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.nio.charset.StandardCharsets;
import java.io.IOException;
public class ReadUtf8File {
public static void main(String[] args) {
String filePath = "example.txt";
try {
// Read all lines from the UTF-8 encoded file
List<String> lines = Files.readAllLines(Paths.get(filePath), StandardCharsets.UTF_8);
for (String line : lines) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why Use StandardCharsets.UTF_8
?
Using StandardCharsets.UTF_8
guarantees that you read the file with the correct encoding. It prevents issues related to platform default encoding and ensures that characters are loaded accurately.
Dealing with Databases
Character encoding issues often arise when inserting or retrieving data from databases. Ensure that your database is configured to use UTF-8 encoding.
Configuring MySQL
If you're using MySQL, make sure the character set is set to UTF-8. Use the following SQL commands:
SET NAMES 'utf8mb4';
SET character_set_connection=utf8mb4;
SET character_set_results=utf8mb4;
Also, ensure your JDBC URL includes useUnicode=true&characterEncoding=utf8
.
JDBC Example
Using JDBC to connect to a MySQL database with UTF-8:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase?useUnicode=true&characterEncoding=utf8";
String username = "root";
String password = "password";
try (Connection conn = DriverManager.getConnection(url, username, password)) {
// Connection established
System.out.println("Database connected!");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
IDE Plugins and Tools
To further enhance your experience when dealing with character encodings in Eclipse, consider using plugins.
- Eclipse Code Recommenders provides suggestions for fixing issues, including encoding problems.
- Eclipse Marketplace offers a variety of tools to help manage and visualize encodings effectively.
Final Considerations
UTF-8 encoding issues can derail even the best of projects. Thankfully, by properly configuring Eclipse and ensuring that your code and data interactions follow UTF-8 standards, you can mitigate these issues effectively.
If you follow the steps outlined in this blog post, you should be on the right track to resolving any UTF-8 encoding problems you might face in Eclipse. For further reading, check out the Oracle Java Documentation and W3C's character encoding guide.
By addressing these settings, your application will better support internationalization, leading to a smoother experience for users across the globe. Happy coding!