Common Pitfalls in Spring AOP You Should Avoid
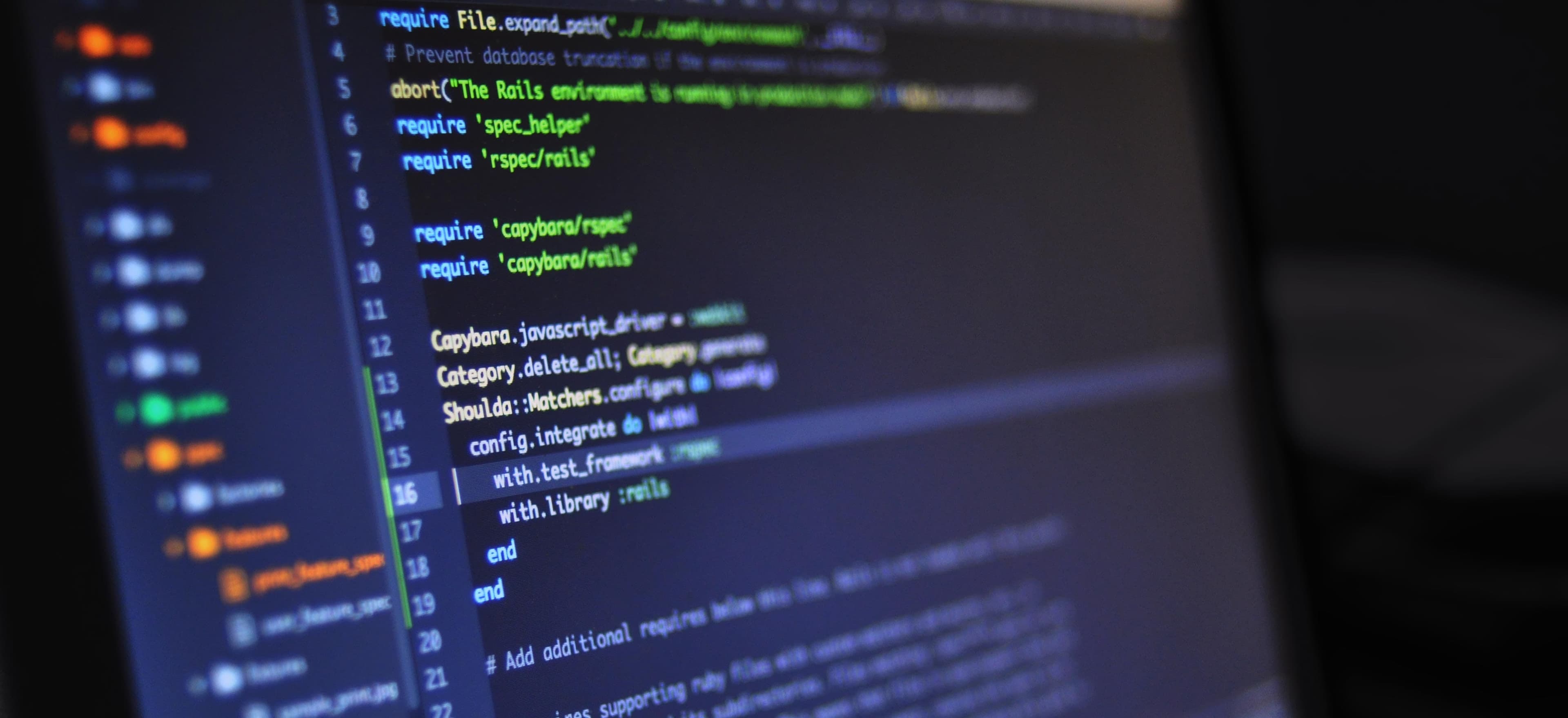
- Published on
Common Pitfalls in Spring AOP You Should Avoid
Aspect-Oriented Programming (AOP) is a powerful paradigm in programming that separates cross-cutting concerns, allowing developers to write cleaner, more maintainable code. The Spring Framework leverages AOP extensively, providing developers with tools to weave aspects seamlessly into their applications. However, while AOP offers numerous benefits, pitfalls may hinder its effectiveness. In this post, we’ll explore common mistakes developers make when working with Spring AOP and how to avoid them.
Understanding Spring AOP
Before diving into pitfalls, it’s crucial to understand the fundamentals of Spring AOP. AOP enables developers to define "aspects" - reusable modules that can intercept method calls, manage transactions, handle logging, and more. This approach helps keep business logic cleaner and more focused.
Core Concepts of Spring AOP
- Aspect: A class containing advice (code that is executed) and pointcut definitions.
- Join Point: A point during the execution of a program, such as a method call.
- Pointcut: An expression that matches join points, determining where advice should be applied.
- Advice: The code that is executed when a join point is reached.
Here’s a simple example of a Spring AOP aspect:
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBeforeMethodExecution() {
System.out.println("Method execution started");
}
}
Why It Matters
This aspect, LoggingAspect
, will log a message before any method in the com.example.service
package is executed. Launching AOP in this manner allows for cleaner service classes and centralized logging functionality.
Pitfall 1: Not Using Proper Annotations
The Issue
One common mistake is neglecting to use the appropriate AOP annotations. Using annotations incorrectly can lead to misunderstandings in how aspects are applied.
The Solution
Always make sure to use the correct AOP annotations in your aspect classes, e.g., @Aspect
, @Before
, and @Around
. Moreover, familiarize yourself with the scope of these annotations.
Here's an example of a missing @Component
annotation:
@Aspect
public class LoggingAspect {
// Missing @Component might prevent Spring from detecting this aspect
}
Why It Matters
Without the @Component
annotation, Spring’s component scanning will ignore the aspect, resulting in no logging being performed. Always ensure your aspects are registered as Spring beans.
Pitfall 2: Using Pointcuts Too Broadly
The Issue
A broad pointcut can lead to performance issues and unexpected behaviors. Applying aspects to excessively large scopes means that advice may run more often than necessary.
The Solution
Define pointcuts as narrowly as possible.
For example, instead of this:
@Before("execution(* com.example..*.*(..))") // Too broad
Use:
@Before("execution(* com.example.service.UserService.*(..))") // More targeted
Why It Matters
Using targeted pointcuts enhances application performance and makes debugging easier. Specificity allows you to control when advice is applied, reducing overhead.
Pitfall 3: Misunderstanding Proxy Behavior
The Issue
Spring AOP operates through proxies, either JDK dynamic proxies or CGLIB proxies. Many developers mistakenly believe that AOP can intercept calls to self methods (internal method calls), which it cannot.
The Solution
Be aware of proxy settings in your Spring configuration and understand that internal method calls bypass AOP.
For example, if you have:
@Aspect
@Component
public class SecurityAspect {
public void secureMethod() {
// Security checks
}
public void applySecurity() {
secureMethod(); // This will not trigger the security aspect!
}
}
Why It Matters
Understanding this limitation helps in structuring your code better. If you require cross-cutting concerns for self-invocations, consider refactoring to use a delegate pattern, where the method being advised is invoked from another bean.
Pitfall 4: Failing to Handle Exceptions Properly
The Issue
When using AOP, it's essential to handle exceptions, especially in the context of advice. Neglecting exception handling may lead to incomplete transactions or unexpected behavior.
The Solution
Use @Around
advice when you want to wrap method executions, allowing you to handle exceptions effectively.
Here’s an example:
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
@Aspect
@Component
public class ExceptionHandlingAspect {
@Around("execution(* com.example.service.*.*(..))")
public Object handleExceptions(ProceedingJoinPoint joinPoint) throws Throwable {
try {
return joinPoint.proceed();
} catch (Exception e) {
// Handle or log the exception
System.out.println("Exception caught in aspect: " + e.getMessage());
throw e; // Re-throwing might be important for other layers
}
}
}
Why It Matters
With @Around
, you gain control over both the execution and exceptions. Proper exception handling prevents your application from failing silently and enables logging or other recovery measures.
Pitfall 5: Ignoring Aspect Order
The Issue
When you have multiple aspects that may apply to the same join points, the order in which they execute can lead to unexpected side effects unless specified correctly.
The Solution
Utilize @Order
on your aspect classes to define their execution order explicitly.
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.order.annotation.Order;
@Aspect
@Order(1) // Ensures this aspect runs first
@Component
public class FirstAspect {
// Your code
}
Why It Matters
Defining execution order ensures predictable behavior in complex systems where multiple aspects interact. It’s crucial for ensuring that high-priority concerns execute at the desired stage in the application lifecycle.
The Closing Argument
Spring AOP can be incredibly powerful when used correctly, but common pitfalls can hinder its effectiveness. By understanding the nuances of Spring AOP and applying best practices, you can leverage its capabilities to craft a cleaner, more maintainable codebase. Always remember to focus on proper annotations, targeted pointcuts, proxy behavior, exception handling, and aspect order to avoid these pitfalls.
For a deeper understanding of Spring AOP, consider checking out the Spring documentation for more information and examples.
By steering clear of these common mistakes, you can maximize the benefits of Spring AOP in your applications. Happy coding!