Overcoming the Complexity of RDD Fault Tolerance in Spark
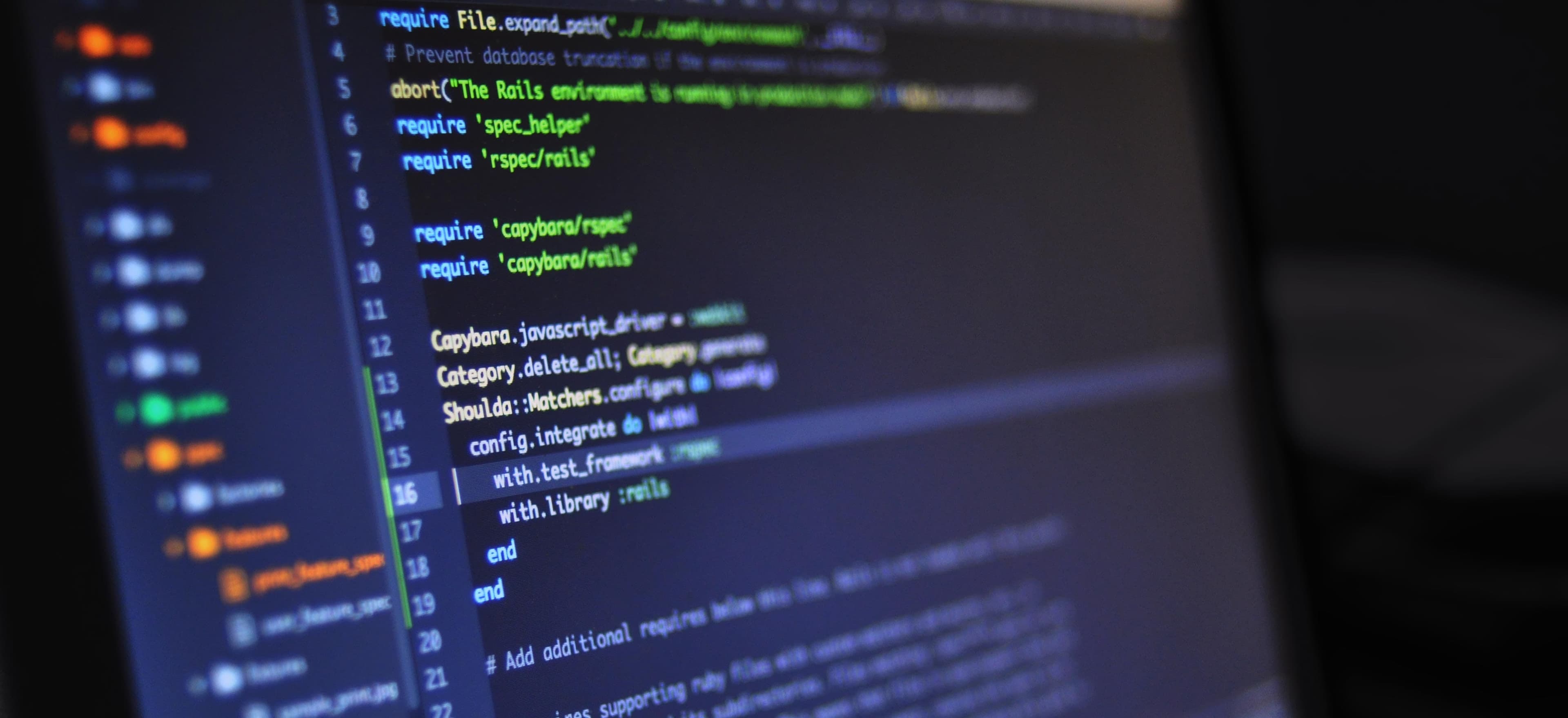
- Published on
Overcoming the Complexity of RDD Fault Tolerance in Spark
Apache Spark revolutionized the way we handle data processing. Its impressive capabilities in handling large datasets quickly and efficiently stem, in no small part, from the resilient distributed dataset (RDD) abstraction. While RDDs are instrumental for distributed computation, their inherent complexity—specifically related to fault tolerance—can impose challenges. In this blog post, we will navigate through the intricacies of RDD fault tolerance, explore how it works, and discuss strategies to effectively manage and utilize it in your Spark applications.
What is RDD?
RDD is the core abstraction in Spark that enables fault-tolerant distributed data processing. It represents a collection of objects that can be partitioned across a cluster, allowing for parallel processing. Perhaps the most significant feature of RDDs is their ability to recover from failures, which is vital in a distributed computing environment.
Key Characteristics of RDDs
- Immutable: Once created, RDDs cannot be changed. This immutability helps track lineage and supports fault tolerance.
- Lazy Evaluation: RDD transformations are not computed until an action is triggered. This allows Spark to optimize the execution plan and potentially avoid unnecessary computations.
- Partitioned: RDDs can be split into partitions, which are distributed across the nodes in a cluster, allowing parallel processing.
Understanding Fault Tolerance with RDDs
Fault tolerance in Spark is guaranteed through RDD lineage. When an RDD is modified, the new RDD does not alter the original but instead represents a logical transformation applied to the previous RDD. This lineage graph keeps track of the transformation sequence, allowing Spark to recompute lost partitions when a failure occurs.
How Does RDD Lineage Work?
Each RDD maintains a ‘lineage’ or a record of its parent RDDs and the transformations applied to them. If a partition of an RDD is lost, Spark can efficiently recover it by re-running the necessary transformations on its parent RDDs.
Example Code Snippet
JavaRDD<String> inputRDD = sparkContext.textFile("hdfs://data/input.txt");
JavaRDD<String> transformedRDD = inputRDD
.filter(line -> line.length() > 0)
.map(line -> line.toUpperCase());
In this snippet:
- The
inputRDD
reads data from HDFS. - The
transformedRDD
filters out empty lines and converts the text to uppercase. - In case of a failure while computing
transformedRDD
, Spark can go back to theinputRDD
and apply the same transformations to reconstruct what was lost.
Why Choose Lineage for Fault Tolerance?
Using lineage for fault tolerance provides several advantages:
- Low Overhead: Lineage allows Spark to only recompute lost data instead of storing duplicates across the cluster.
- Simplicity: The lineage model aligns naturally with the functional programming paradigm, simplifying reasoning about data transformations.
- Scalability: As applications grow, lineage graphs grow proportionately, offering a straightforward path to scaling up.
Challenges in RDD Fault Tolerance
Despite its powerful fault tolerance mechanism, RDDs face specific challenges:
- Complex Transformations: When using multiple transformations, lineage graphs can become complex and large, potentially leading to inefficiencies during recovery.
- Memory Consumption: Storing long lineage histories can consume memory, making it challenging for long-running applications.
- Performance Implications: Too many transformations can affect performance, as Spark needs to re-compute stages from distant parents in the lineage chain.
Strategies to Overcome Fault Tolerance Challenges
Fortunately, there are effective strategies to minimize complexity and enhance RDD fault tolerance.
Use Checkpointing
Checkpointing is a way to truncate the lineage graph. It involves saving the RDD to a reliable persistent storage (like HDFS or S3) so that if data needs to be recomputed, Spark can start from the checkpoint rather than traversing back through the entire lineage.
sparkContext.setCheckpointDir("hdfs://data/checkpoints");
JavaRDD<String> checkpointedRDD = transformedRDD.checkpoint();
In this code:
- The
setCheckpointDir
method specifies where to save checkpoints. - The checkpoint operation marks
transformedRDD
for storage, which can be used to recover if issues arise.
Use Caching Carefully
Caching RDDs can speed up access and reduce recomputation time. However, be mindful of when and what to cache. Only cache RDDs used multiple times to prevent unnecessary memory consumption.
transformedRDD.cache();
This one-line cache invocation ensures that transformedRDD
is stored in memory for future actions.
Leverage Broadcast Variables
Often, RDD transformations involve shared data. Using broadcast variables can distribute this data efficiently across the cluster, reducing data transfer costs.
Broadcast<Map<String, String>> broadcastVar = sparkContext.broadcast(myMap);
JavaRDD<String> enrichedRDD = transformedRDD.map(line -> {
// Access broadcast variable
String value = broadcastVar.value().get(line);
return line + " enriched with " + value;
});
In this example, broadcastVar
is shared across tasks, enabling fast access without multiple data transfers.
Wrapping Up
Navigating RDD fault tolerance in Spark can initially seem daunting, given the complex transformations and memory considerations. However, understanding the underlying principles, primarily focusing on lineage, checkpointing, caching, and proper variable broadcasting, can help overcome these obstacles.
The powerful combination of RDDs and Spark’s fault tolerance ensures that your data processing applications are not just robust but also optimized for performance.
For more details on RDDs, refer to the Apache Spark Documentation. To get a deeper understanding of Spark’s architecture, check out the Spark: The Definitive Guide by Bill Chambers and Matei Zaharia.
As you venture into the world of Apache Spark, leverage these strategies to enhance your applications and effectively manage dataset reliability. Happy coding!