Common Pitfalls When Getting Started with TestNG Framework
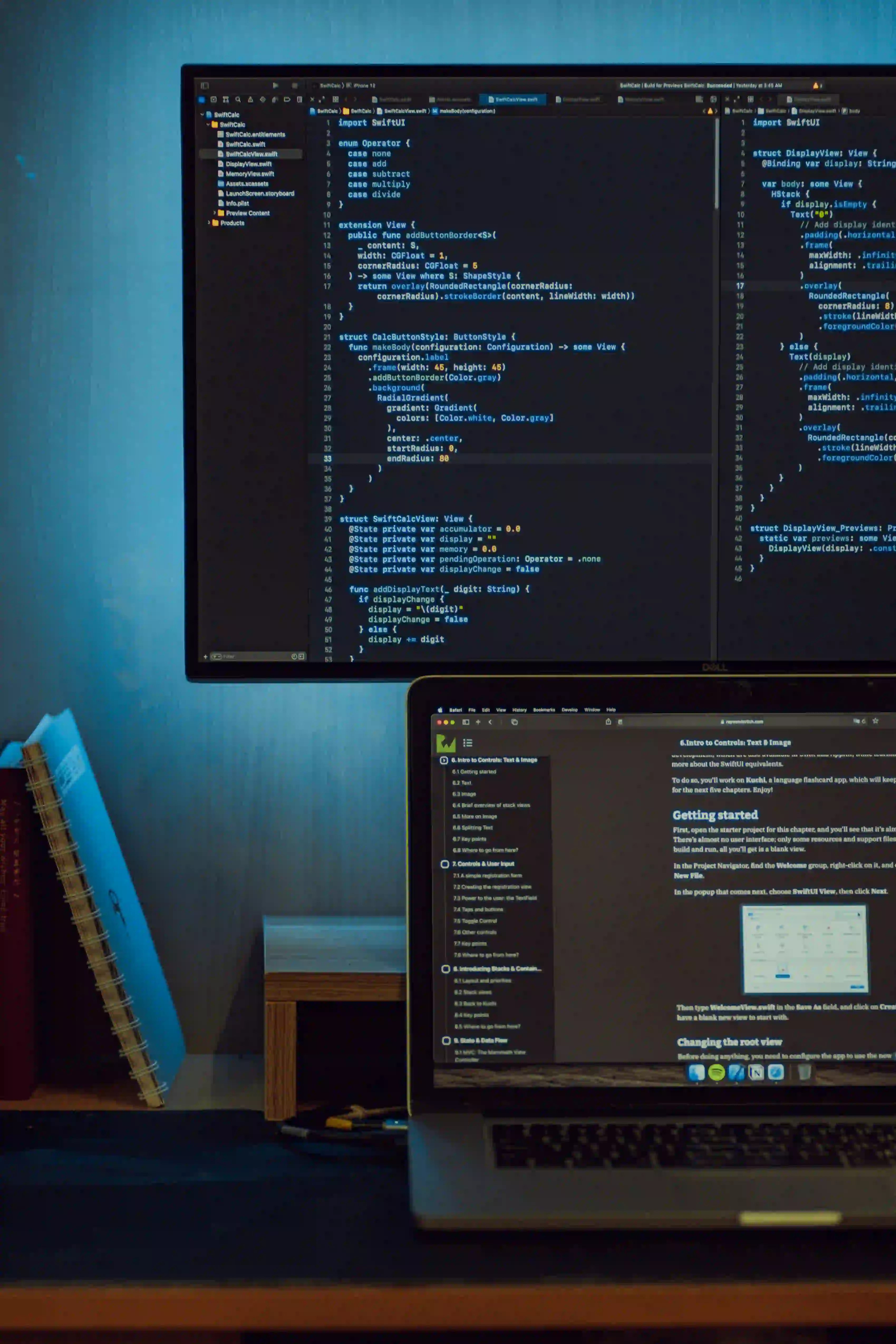
Common Pitfalls When Getting Started with TestNG Framework
When diving into test automation, especially in Java-based projects, it’s crucial to leverage robust testing frameworks. One of the standout frameworks in this domain is TestNG. TestNG offers an innovative approach with annotations, allowing for more flexible testing than its predecessors. However, beginners often encounter various pitfalls that can derail their testing efforts. In this blog post, we'll discuss these common pitfalls while also offering proactive solutions to help streamline your journey with TestNG.
What is TestNG?
Before we get into the pitfalls, it’s important to clarify what TestNG is. TestNG (Test Next Generation) is a testing framework inspired by JUnit and NUnit but introduces some unique functionalities to simplify the testing process. It supports annotations, supports flexible test configurations, enables parallel execution, and is highly extensible.
Key Features of TestNG:
- Annotations: Simplifies writing test cases.
- Parameterization: Allows passing parameters to test methods.
- Parallel Execution: Enables running tests concurrently, enhancing test efficiency.
- Data Providers: Facilitates data-driven testing.
Pitfall 1: Ignoring Annotations
Understanding TestNG Annotations
TestNG relies heavily on annotations to organize and control your testing execution. Some widely used annotations include:
@Test
: Marks a method as a test method.@BeforeMethod
: Runs before each test method.@AfterMethod
: Runs after each test method.@BeforeClass
: Runs once before the first method in the current class.@AfterClass
: Runs once after all the methods in the current class.
Example Code
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.Test;
public class SampleTest {
@BeforeClass
public void setUp() {
System.out.println("Setting up before tests...");
}
@Test
public void testAddition() {
int result = add(2, 3);
assert result == 5 : "Addition failed";
System.out.println("Test Passed: " + result);
}
@AfterClass
public void tearDown() {
System.out.println("Cleaning up after tests...");
}
private int add(int a, int b) {
return a + b;
}
}
Why This Matters
Ignoring annotations may lead you to believe that your tests are executed in a logical sequence, which they may not be. Mismanagement of test sequences can lead to misleading results and complicated debugging.
Pitfall 2: Misconfiguring TestNG XML File
The Role of TestNG XML
The TestNG XML file is essential for running tests. It defines how tests will be executed, their configurations, and related settings.
Example XML Configuration
<!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd" >
<suite name="Suite1">
<test name="Test1">
<classes>
<class name="SampleTest"/>
</classes>
</test>
</suite>
Why This Matters
Misconfiguring the TestNG XML file can lead to parts of your test suite not running as expected. Always ensure that you specify the correct test class names and methods.
Pitfall 3: Overcomplicating Test Structure
Keeping It Simple
Many beginners attempt to create excessively complex test structures with various layers of abstraction. While it's important to have organized tests, excessive complexity can be counterproductive.
Example of Simplified Testing
Having a flat structure with focused tests is generally more maintainable.
import org.testng.annotations.Test;
public class UserServiceTest {
@Test
public void testUserCreation() {
UserService userService = new UserService();
User user = userService.createUser("Alice");
assertNotNull(user);
}
@Test
public void testUserDeletion() {
UserService userService = new UserService();
userService.deleteUser("Alice");
assertNull(userService.getUser("Alice"));
}
}
Why This Matters
A simpler test structure aids in maintainability, readability, and can significantly speed up debugging. Follow the KISS principle: Keep It Simple, Stupid.
Pitfall 4: Not Leveraging Data Providers
The Need for Data Providers
Data providers in TestNG are a powerful means of running the same test with multiple sets of data. Neglecting to use them is a common oversight.
Example of a Data Provider
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class CalculatorTest {
@DataProvider(name = "additionData")
public Object[][] provideData() {
return new Object[][] {
{1, 1, 2},
{2, 3, 5},
{5, 5, 10}
};
}
@Test(dataProvider = "additionData")
public void testAddition(int a, int b, int expectedSum) {
assert add(a, b) == expectedSum;
}
private int add(int a, int b) {
return a + b;
}
}
Why This Matters
Using data providers can significantly reduce code duplication. Instead of writing multiple test cases for different inputs, you can consolidate tests, increasing efficiency.
Pitfall 5: Poor Exception Handling
Understanding Test Failure Reasons
Not managing exceptions properly can lead to misleading test reports, making it difficult to identify the root cause of failure.
Tips for Exception Handling
- Use assertions wisely.
- Log meaningful messages.
- Catch specific exceptions.
Example of Exception Handling in Tests
import org.testng.Assert;
import org.testng.annotations.Test;
public class ExceptionHandlingTest {
@Test(expectedExceptions = ArithmeticException.class)
public void testDivisionByZero() {
int result = divide(10, 0);
}
private int divide(int a, int b) {
return a / b;
}
}
Why This Matters
Effective exception handling not only improves report quality but also streamlines the debugging process. Implementing clear exception rules makes it easier to understand test results.
Pitfall 6: Ignoring Test Annotations Timing
Testing Setup and Teardown
Understanding when your setup and teardown methods are invoked is essential. Misplaced annotations may lead to tests that are not properly isolated from each other, resulting in flaky tests.
Example Code for Timing Visualization
import org.testng.annotations.*;
public class UserTest {
@BeforeMethod
public void init() {
System.out.println("Initializing before each test method...");
}
@Test
public void testUserUpdate() {
System.out.println("Testing user update...");
}
@AfterMethod
public void clean() {
System.out.println("Cleaning up after each test method...");
}
}
Why This Matters
Understanding the lifecycle of your tests helps in isolating test contexts, thereby ensuring they do not interfere with one another.
The Closing Argument
While TestNG offers a robust framework for testing, newcomers often stumble when getting started. By recognizing these common pitfalls—incorrect usage of annotations, misconfiguring TestNG XML, complicating the test structure, not leveraging data providers, poor exception handling, and misunderstanding timing—you can avoid many of the headaches associated with beginning your automation journey.
By following best practices, you'll not only streamline your learning process but also create a basis for efficient and robust test writing.
For more elaborate discussions on advanced TestNG features, check out the official TestNG documentation.
Happy testing!