How to Choose the Right Package for Your Needs
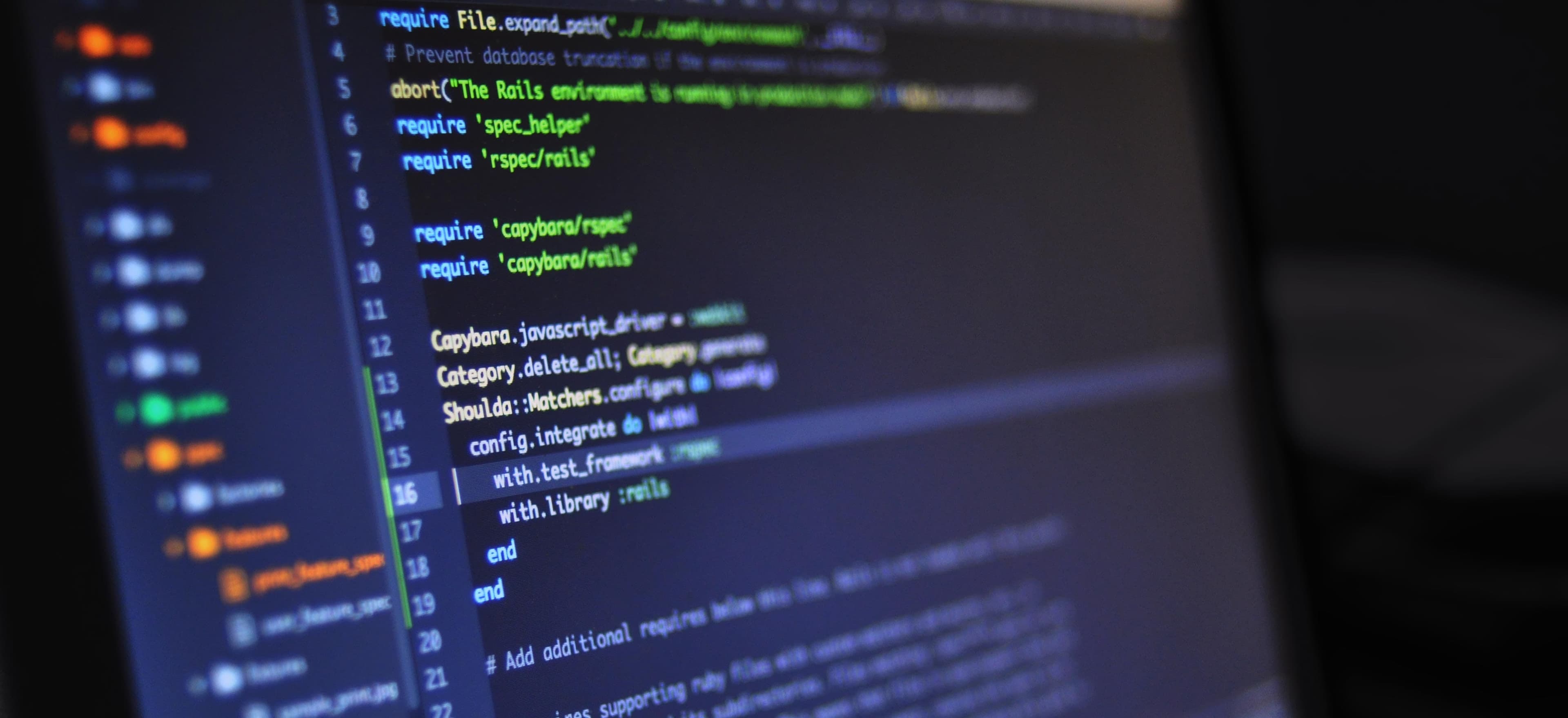
- Published on
How to Choose the Right Package for Your Needs in Java Development
Choosing the right package in Java can often feel overwhelming. With an array of libraries and frameworks available, the decision-making process can be cumbersome. However, understanding a few key principles can simplify your choice and ensure you select the best package for your project's needs. In this blog post, we will guide you through the essential factors to consider when picking the right package and illustrate with code examples.
Understanding Java Packages
In Java, packages are used to group related classes and interfaces together. This organization makes it easier to manage large applications. By utilizing packages, you can structure your code more efficiently, avoid name conflicts, and control access with visibility modifiers.
Factors to Consider When Choosing a Package
-
Functionality
The first and foremost factor is the functionality offered by the package. Ensure it meets the specific requirements of your project. This comes down to understanding the features and capabilities of the package.
For instance, if you're working on a web application, you might consider using Spring Framework for its robust features in managing web applications. Conversely, for lightweight applications, Java’s built-in libraries might suffice.
Example:
import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class HelloController { @GetMapping("/hello") public String sayHello() { return "Hello, World!"; } }
In this case, choosing the Spring package allows you to easily manage RESTful web services with minimal overhead due to its built-in annotations and structure.
-
Peer Recommendations and Community Support
Before settling on a package, look for feedback from peers and the development community. Libraries and frameworks with a strong community and user base are generally more reliable. You'll find many support threads, tutorials, and documentation to help you.
Consider the following points:
- Popularity: Check platforms like GitHub or Stack Overflow for usage metrics and community feedback.
- Activity: Frequent updates indicate that the package is actively maintained. Look for the latest releases.
- Documentation: High-quality documentation is a hallmark of a good package. It should clearly describe how to implement features and troubleshoot issues.
-
Performance
Performance matters, especially when developing large-scale applications. Before incorporating a library, evaluate its performance metrics. Review benchmarks and conduct your tests to ensure it meets your needs.
For example, consider Apache Commons Collections for data manipulation tasks. Here’s how to use a few of its utility classes:
import org.apache.commons.collections4.CollectionUtils; import java.util.ArrayList; import java.util.List; public class CollectionExample { public static void main(String[] args) { List<String> list1 = new ArrayList<>(); list1.add("Java"); list1.add("Python"); List<String> list2 = new ArrayList<>(); list2.add("C++"); // Merging two collections List<String> mergedList = new ArrayList<>(list1); mergedList.addAll(list2); System.out.println("Merged List: " + mergedList); // Checking if two collections are equal boolean areEqual = CollectionUtils.isEqualCollection(list1, list2); System.out.println("Are the collections equal? " + areEqual); } }
By using Apache Commons Collections, complex data manipulations become straightforward without compromising performance.
-
Compatibility and Licensing
Ensure the chosen package is compatible with your existing system. Check for compatibility with your Java version, and any other frameworks or libraries in use.
Licensing is equally crucial. Some packages come with restrictive licenses, while others are open-source. Familiarize yourself with the license terms to avoid future legal issues. For instance, the MIT License is popular for open-source projects due to its permissiveness.
-
Ease of Use and Learning Curve
Consider how easy the package is to learn and use. For newer developers, a steep learning curve can lead to frustration and delayed development. Look for packages that are straightforward and come with good starter examples.
Frameworks like Spring Boot are excellent choices due to their focus on simplicity and ease of use. Here’s how you can quickly create a REST service using Spring Boot:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } @RestController public class MyController { @GetMapping("/api") public String getApiResponse() { return "API Response"; } } }
With just a few lines of code, you have a fully functional REST API, demonstrating the simplicity of using Spring Boot.
-
Cost
If your package choice involves costs (like paid libraries or commercial frameworks), thoroughly assess your budget. Weigh the expenses against the expected benefits. Are you paying for advanced features that you might not need?
Moreover, consider the total cost of ownership; this includes warranty, future licensing fees, and support services.
Closing the Chapter
Choosing the right package for your needs is paramount in Java development. By focusing on functionality, community support, performance, compatibility, ease of use, and cost, you can make an informed decision.
As a final tip, never hesitate to try out a package before making it a core part of your project. Experimentation can provide valuable insights into its practical application and fit with your needs. Remember, the goal is to enhance productivity and maintainability.
For additional context and resources, you might find these links helpful:
- Spring Framework Documentation
- Apache Commons Documentation
- Java Collections Framework
With these guidelines in mind, you'll be well on your way to selecting the right Java packages for your projects. Happy coding!