Common Mistakes When Using Decorators in Python
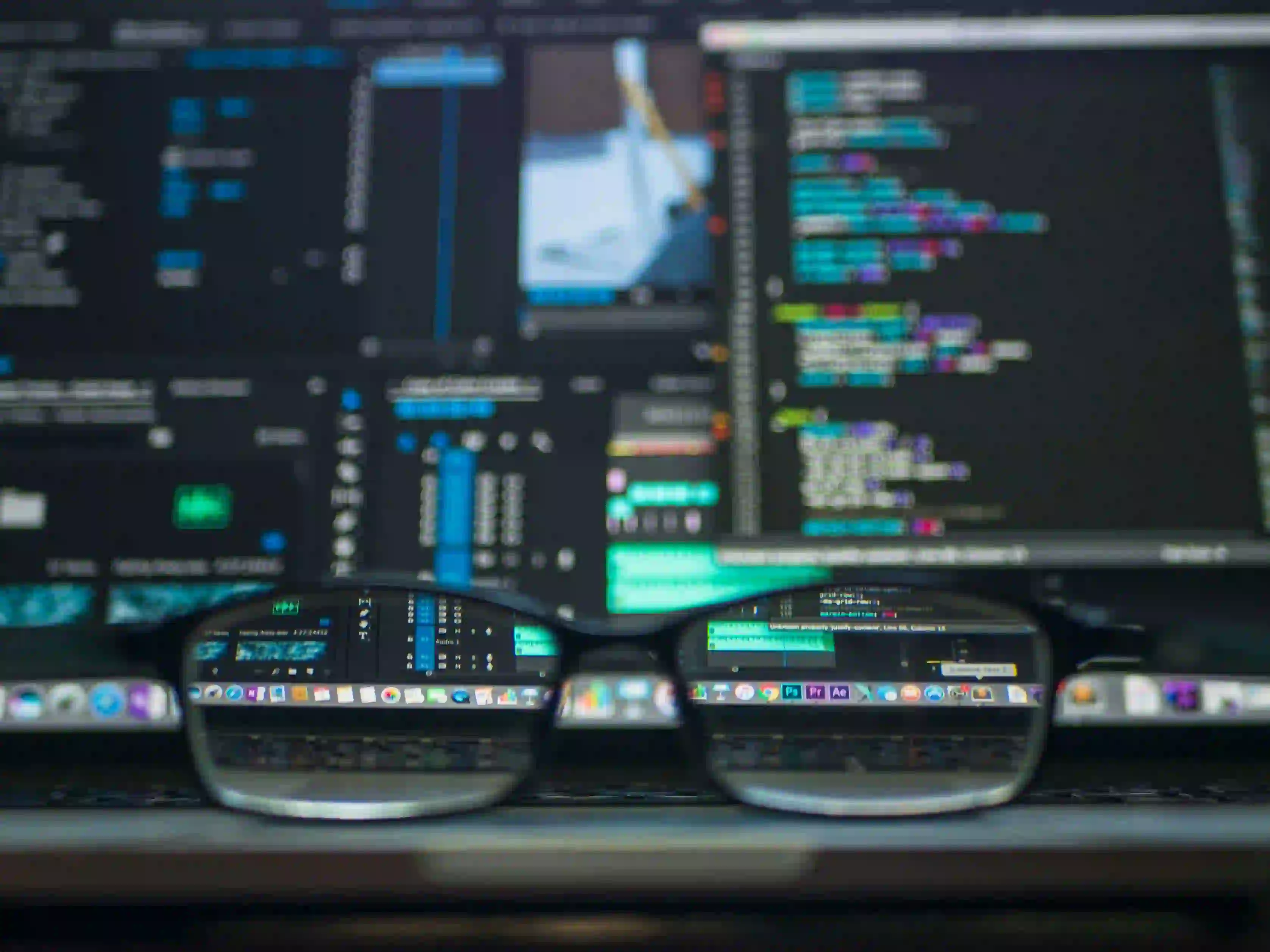
Common Mistakes When Using Decorators in Python
Python decorators are a powerful feature that allows you to modify the behavior of a function or a method. They can be incredibly useful in many scenarios, from logging and authentication to enforcing function signatures. However, when not used carefully, decorators can introduce subtle bugs and unexpected behavior. In this blog post, we'll explore some common mistakes developers make when using decorators in Python and how to avoid them.
What is a Decorator?
A decorator in Python is a function that takes another function and extends the behavior of the latter function without explicitly modifying it. The decorator pattern is a great way to implement the Single Responsibility Principle. This principle states that every function should have one reason to change.
Here's a simple example of a decorator:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
When you run the above code, you'll see:
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
In the example above, my_decorator
modifies the behavior of the say_hello
function without changing it directly.
Common Mistakes
1. Forgetting to Use functools.wraps
One of the most common mistakes when creating a decorator is forgetting to use functools.wraps
. This utility updates the wrapper function to look like the original function, preserving its metadata.
from functools import wraps
def my_decorator(func):
@wraps(func) # This line is crucial
def wrapper(*args, **kwargs):
print("Something before")
result = func(*args, **kwargs)
print("Something after")
return result
return wrapper
@my_decorator
def say_hello():
"""This function greets you."""
print("Hello!")
print(say_hello.__name__) # Outputs: say_hello
print(say_hello.__doc__) # Outputs: This function greets you.
Without @wraps(func)
, calling say_hello.__name__
would return wrapper
instead of say_hello
.
2. Not Accepting Arguments in the Decorator
If your decorator is supposed to accept arguments, omitting that feature will lead to confusion. The decorator itself should accept parameters and return a function that takes the target function.
Hereβs an example of a properly designed decorator that accepts arguments:
def repeat(num_times):
def decorator_repeat(func):
@wraps(func)
def wrapper(*args, **kwargs):
for _ in range(num_times):
func(*args, **kwargs)
return wrapper
return decorator_repeat
@repeat(num_times=3)
def say_hello():
print("Hello!")
say_hello()
Why this matters:
This allows decorators to be more flexible and reusable. Always ensure that you design decorators with the possibility for parameters when necessary.
3. Ignoring Function Signatures
Another common mistake is not preserving the signature of the original function. This can make debugging difficult, as third-party tools like inspector
might not work correctly with such wrappers.
You can solve this issue with functools.wraps
, as shown above, or by using the inspect
module to create a decorator that preserves the function signature manually.
import inspect
def my_decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
return func(*args, **kwargs)
return wrapper
4. Overusing Decorators
While decorators can clean up code and make it more manageable, overusing them can lead to "decorator hell." This means stacking too many decorators on a single function, making it hard to follow the control flow.
Instead of piling up decorators, consider extracting complex functionalities into dedicated functions or classes:
def logging_decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
print(f"Calling {func.__name__} with {args} and {kwargs}")
return func(*args, **kwargs)
return wrapper
def authentication_decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
# Logic for authentication
return func(*args, **kwargs)
return wrapper
@logging_decorator
@authentication_decorator
def secured_function(arg1):
print("Executing secured function")
# Consider applying only one decorator to avoid confusion
5. Modifying Input Arguments Unintentionally
Another issue arises when decorators modify the input arguments of the function they decorate without such behavior being clearly defined. This can lead to unexpected consequences.
To avoid this, always handle parameters cleanly:
def modify_argument_decorator(func):
@wraps(func)
def wrapper(arg):
new_arg = arg + 1 # Modifying the argument
return func(new_arg) # Passes the modified argument
return wrapper
@modify_argument_decorator
def print_number(num):
print(num)
print_number(5) # Outputs: 6
In this case, it would be advisable to clearly document the behavior of the original function to prevent any misuse.
6. Not Testing Your Decorators
Like any code, decorators should also be thoroughly tested. Every variation in input and every potential edge case should be verified. This is especially important in decorators that incorporate business logic.
You can use testing frameworks such as unittest
or pytest
to ensure your decorators are working as expected.
The Closing Argument
Decorators in Python are a robust programming construct. They can greatly improve code maintenance and readability when used properly. However, they come with their own set of common pitfalls.
To recap:
- Always use
functools.wraps
to preserve metadata. - Design decorators to accept parameters if needed.
- Preserve function signatures to facilitate debugging.
- Avoid stacking too many decorators on one function.
- Be cautious while modifying input arguments.
- Test your decorators thoroughly.
By avoiding these common mistakes, you can harness the power of decorators effectively in your Python projects.
For more knowledge on Python decorators, consider exploring these Python Docs on Decorators and Real Python's Guide on Decorators.
Happy coding!