Don't Miss Out: Upgrade to Java 8 for Better Performance!
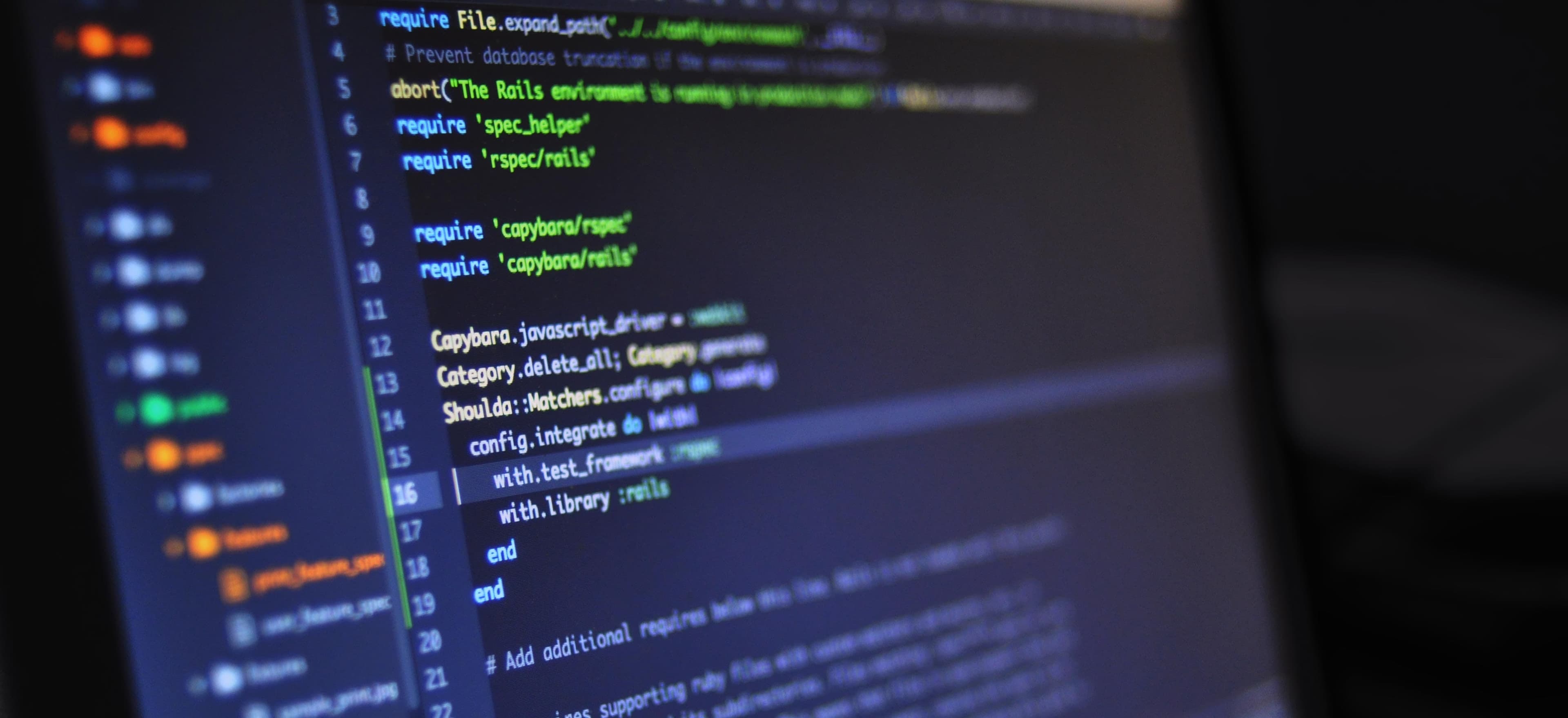
- Published on
Don't Miss Out: Upgrade to Java 8 for Better Performance!
Java has been one of the leading programming languages for decades, powering a vast array of applications and platforms. However, with the release of Java 8, developers worldwide have been prompted to take a closer look at their Java applications and consider the significant benefits of upgrading. This blog post explores why upgrading to Java 8 is crucial, focusing on performance improvements, new features, and practical implementations.
The Importance of Upgrading
When a new version of Java is released, it often comes with performance enhancements and pivotal features that can make development easier and more efficient. Upgrading to Java 8 is especially beneficial because it introduces features that not only enhance performance but also improve code quality and developer productivity.
Key Performance Improvements in Java 8
Java 8 introduced several improvements that can lead to better performance:
-
Lambda Expressions: These allow you to write clearer and more concise code. More importantly, they can result in performance improvements particularly in scenarios involving functional programming, as they enable optimally parallel operations.
-
Stream API: This powerful tool is designed to process sequences of elements—collections, arrays, etc.—in a functional style. It allows for parallel processing of data, thus making your applications much faster when dealing with large collections.
-
Default Methods: Default methods enable developers to add new methods to interfaces without breaking existing implementations. This simplifies code evolution and can enhance performance by optimizing method calls.
Let’s dive deeper into these features, exploring how they practically enhance performance.
Lambda Expressions: Simplicity and Efficiency
Lambda expressions allow you to express instances of single-method interfaces (functional interfaces) in a much more readable and less verbose manner. Here’s a simple example to illustrate their power.
import java.util.Arrays;
import java.util.List;
public class LambdaExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Lambda expression to print names
names.forEach(name -> System.out.println(name));
}
}
Why Use Lambda Expressions?
- Conciseness: The code is shorter and easier to read compared to traditional anonymous classes.
- Enhanced Readability: By removing boilerplate code, lambda expressions clarify the intent.
- Facilitates Parallel Processing: They can easily be used with the Stream API.
The Stream API: A Paradigm Shift
The Stream API empowers developers to process collections of objects using a functional approach. It allows for bulk operations on collections and is particularly significant in handling large datasets efficiently.
Here's a practical example.
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
// Using Stream API to filter even numbers and print them
numbers.stream()
.filter(n -> n % 2 == 0)
.forEach(System.out::println);
}
}
Why Stream API Matters
-
Parallel Processing: Create parallel streams to utilize multicore processors.
numbers.parallelStream() .filter(n -> n % 2 == 0) .forEach(System.out::println);
-
Less Boilerplate Code: The Stream API reduces the amount of code required to perform common operations.
-
Code Reusability: It allows developers to define common behaviors in one place, promoting reuse.
Default Methods: Flexibility and Forward Compatibility
Default methods enable developers to add new functionalities to interfaces without breaking existing implementations. This is crucial for maintaining a backward-compatible evolution of APIs.
Here's an example of a default method in an interface:
interface Drawable {
void draw();
default void print() {
System.out.println("Drawing...");
}
}
class Circle implements Drawable {
public void draw() {
System.out.println("Drawing Circle");
}
}
public class DefaultMethodExample {
public static void main(String[] args) {
Circle circle = new Circle();
circle.draw(); // Output: Drawing Circle
circle.print(); // Output: Drawing...
}
}
Why Default Methods?
- Easier API Evolution: Add methods to interfaces with existing classes still intact.
- Improves Code Organization: Reduce the need for helper classes to provide common functionality.
- Save Development Time: You can quickly implement new functionalities without worrying about breaking existing code.
Enhanced Type Inference
Java 8 also provides improved type inference in generic types. The compiler can infer the expected type better thanks to lambda expressions and the Stream API, resulting in cleaner code.
For example, instead of:
List<String> names = new ArrayList<String>();
You can simply use:
List<String> names = new ArrayList<>();
The Bottom Line: The Takeaway
Upgrading to Java 8 is not merely about keeping pace with technological advancements; it represents a commitment to harnessing the most efficient, clean, and robust coding practices. The enhanced performance features, combined with powerful tools like Lambda expressions, Stream API, and default methods, provide a compelling case for upgrading.
Java's continual evolution promises to make your code faster, clearer, and more efficient. If you haven’t transitioned to Java 8 yet, it’s time to make that leap.
For more detailed guidance on upgrading, visit the Oracle Java 8 Documentation.
If you found this article helpful, stay tuned for more insights and tips on Java programming! Keep coding and enjoy the journey!