Why Your JavaFX Layouts Ignore Invisible Nodes
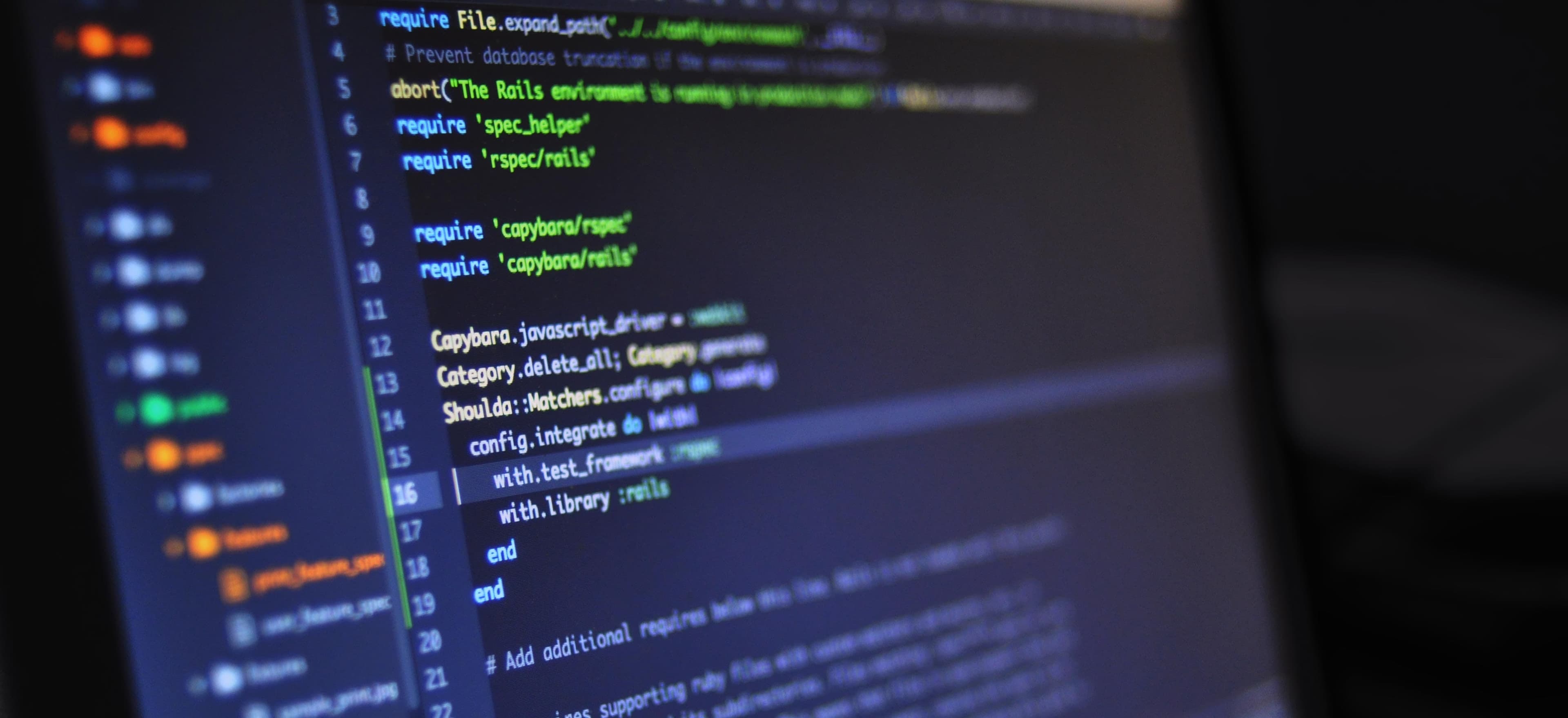
- Published on
Why Your JavaFX Layouts Ignore Invisible Nodes
JavaFX is a fantastic toolkit for building rich client applications in Java. It allows developers to create visually appealing user interfaces with ease. However, one common source of confusion arises when working with layouts: why do JavaFX layouts ignore invisible nodes? This blog post will delve into this issue, exploring the mechanics behind it and providing tips to manage your layouts more effectively.
Understanding the Layout System in JavaFX
Before we address the behavior of invisible nodes, it's essential to understand how the JavaFX layout system functions. JavaFX layouts manage the way nodes (UI components) are arranged on the screen.
The Layout Hierarchy
JavaFX provides several types of layout containers, each catering to specific layout needs:
- VBox: A vertical box layout that stacks children vertically.
- HBox: A horizontal box layout that arranges children side by side.
- GridPane: Organizes nodes in a grid format using rows and columns.
When these layout managers perform their calculations, they consider only the nodes that are visible. The reason is rooted in performance and design.
The Behavior of Invisible Nodes
When a node is set to invisible (using the setVisible(false)
method) or not added to a scene graph, JavaFX layouts skip these nodes during their size and layout calculations. Let's explore a practical example to illustrate this.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class InvisibleNodeExample extends Application {
@Override
public void start(Stage primaryStage) {
VBox layout = new VBox();
Button visibleButton = new Button("I am visible");
Button invisibleButton = new Button("I am invisible");
invisibleButton.setVisible(false);
layout.getChildren().addAll(visibleButton, invisibleButton);
Scene scene = new Scene(layout, 300, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("Invisible Node Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Commentary
In this example, we create a simple VBox
layout containing two buttons. The second button is invisible. When the layout is rendered, you will notice that the visible button occupies space, and the invisible button does not affect the layout at all. The invisible button is omitted from the layout's calculations, making it seem as if it doesn’t exist.
This feature of ignoring invisible nodes helps improve performance. Layout calculations can become intensive, especially with complex layouts, so skipping non-visible nodes can save both computational resources and rendering time.
Practical Implications
Understanding how invisible nodes are treated in JavaFX can influence your application design. For instance:
-
Dynamic UI Elements: If your application allows users to toggle elements (like a settings panel), you might expect that invisible elements would still maintain their layout space. However, they won’t, leading to potential layout shifts when they are made visible.
-
Performance Optimization: If you know certain nodes will not be displayed, setting them to invisible instead of removing them from the scene graph can be an effective way to maintain state without incurring re-initialization costs.
Alternatives: Using Node.setOpacity
Another way to manage the visibility of nodes without skipping layout calculations is by altering the opacity of nodes using the setOpacity(double value)
method. Setting a node's opacity to 0
makes it fully transparent while still retaining its space within the layout.
invisibleButton.setOpacity(0);
While this does keep the node included in layout calculations, it also means that it will respond to events, which can be undesirable. Therefore, the choice between using setVisible(false)
and altering opacity should be carefully considered based on your application's needs.
Working with Advanced Layouts
When building more complex interfaces, you may find that certain layouts require the visibility of nodes to maintain their aspect. In cases where you want to conditionally show content but not disrupt the layout, consider using AnchorPane
, which allows positioning nodes explicitly with respect to their anchors.
Example: Using AnchorPane
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
public class AnchorPaneExample extends Application {
@Override
public void start(Stage primaryStage) {
AnchorPane layout = new AnchorPane();
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
button2.setVisible(false); // still ignored in layout
AnchorPane.setTopAnchor(button1, 50.0);
AnchorPane.setLeftAnchor(button1, 50.0);
AnchorPane.setTopAnchor(button2, 100.0);
AnchorPane.setLeftAnchor(button2, 50.0);
layout.getChildren().addAll(button1, button2);
Scene scene = new Scene(layout, 300, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("AnchorPane Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Final Considerations
In JavaFX, invisible nodes are not merely hidden; they are completely omitted from layout calculations. This characteristic poses both advantages and challenges, particularly when creating dynamic user interfaces. By understanding these behaviors, developers can design and implement their applications more effectively.
For those interested in diving deeper into JavaFX layouts, I recommend checking out the official documentation on JavaFX Layouts which provides further insights into the intricacies of layout management.
Overall, mastering the intricacies of JavaFX layouts, including the handling of invisible nodes, is critical for building polished and responsive applications. With the right knowledge and practices, you can achieve a high level of control over your UI design and functionality. Happy coding!