Overcoming Ceylon's Jigsaw Module System Challenges
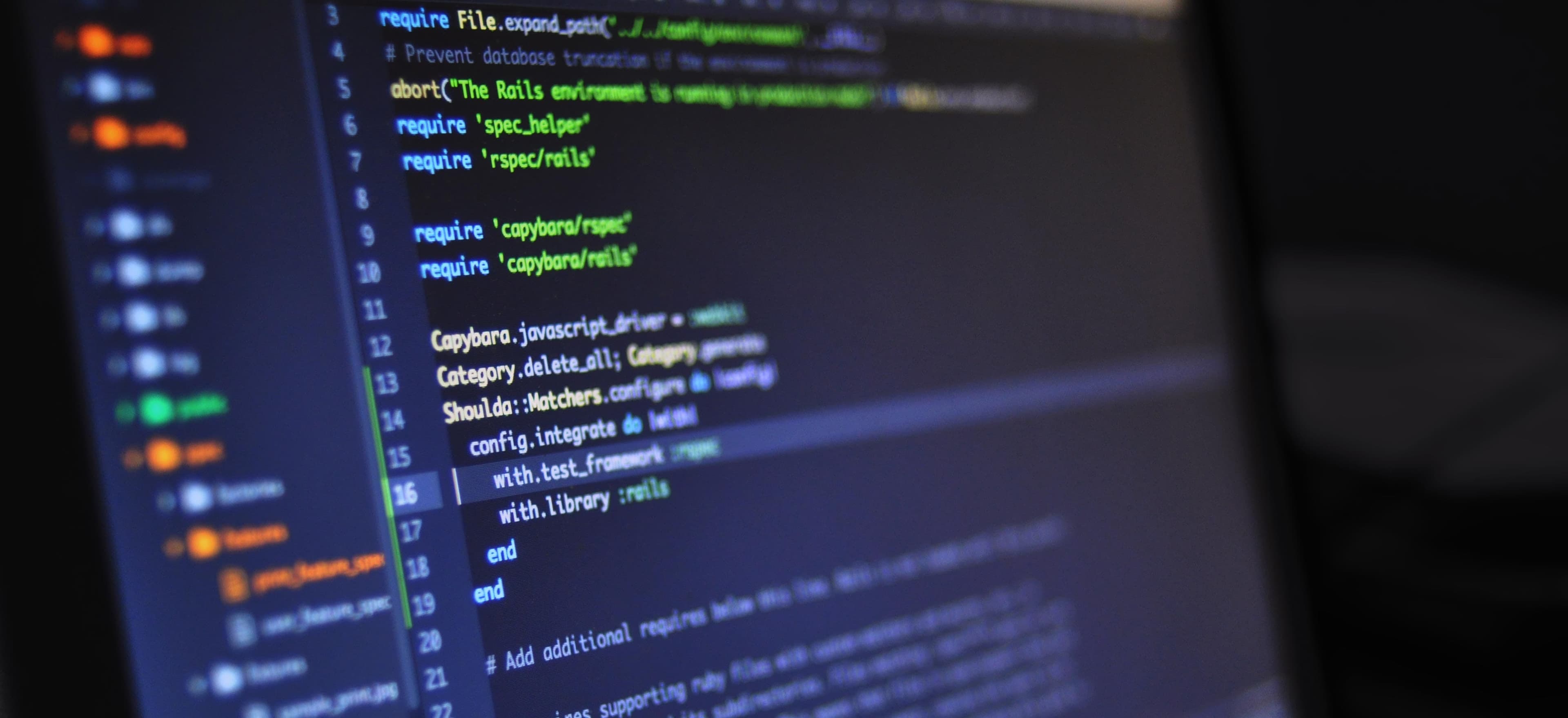
- Published on
Overcoming Ceylon's Jigsaw Module System Challenges
Before We Begin
Ceylon, a programming language designed for modularity, is a powerful tool that offers a fresh perspective on software development. However, its introduction of a unique module system posed specific challenges in compatibility and integration, especially when compared to other Java-based environments. This blog post dives into the intricacies of Ceylon’s Jigsaw module system while offering solutions to these challenges.
By the end of this article, you will have a comprehensive understanding of the primary issues and strategies to navigate through them effectively.
What is Ceylon?
Before we delve into overcoming challenges, let’s briefly define Ceylon. Ceylon is a modern programming language that emphasizes a modular architecture, aiming to enhance reusability and manageability of code. For more information on the Ceylon language, check out the official documentation.
Understanding the Jigsaw Module System
The term "Jigsaw" refers to the Java Platform Module System (JPMS) introduced in Java 9. It revolutionizes Java's project structure by offering a modular framework. But how does Ceylon relate to Jigsaw?
In Ceylon, modules are defined distinctly, and they serve as a foundation for encapsulation, versioning, and dependency management. However, implementing Jigsaw’s concepts in Ceylon can lead to challenges that developers need to be aware of.
Challenges Faced by Ceylon's Jigsaw Module System
1. Compatibility Issues
One of the most significant challenges is compatibility with existing Java libraries. Many Java libraries were not designed with modules in mind, making direct integration difficult.
Solution: Use Ceylon's Java interoperability features, which allow smooth interaction between Ceylon modules and Java code.
Example Code
import java.util { List }
shared void main() {
List<Integer> list = new arrayList<Integer>();
list.add(5);
print(list);
}
Why? This code showcases how we can utilize Java's ArrayList
within Ceylon code while taking advantage of the seamless interaction between the two languages.
2. Naming Conflicts
The rigid naming conventions enforced by Jigsaw can lead to conflicts in large projects, especially when various modules inadvertently define the same package structure.
Solution: Adopt a clear and convention-based naming strategy for your modules to avoid conflicts.
Example Code
module myapp.graphics {
// Graphics related code
}
module myapp.network {
// Network related code
}
Why? By prefixing the module names with a common namespace (e.g., myapp
), you can reduce the chances of naming conflicts significantly.
3. Complexity in Dependency Management
The modular architecture can add an additional layer of complexity in managing dependencies, especially when different modules require disparate versions of a library.
Solution: Use Ceylon's powerful dependency resolution capabilities, which can help ensure that the correct version of libraries is used across modules.
Example Dependency Configuration
module myapp.data {
import myapp.graphics { GraphicsModule }
import myapp.network { NetworkModule }
}
module myapp.graphics {
import myapp.shared { CommonResources }
}
Why? This modular approach lays out clear relationships and dependencies, making it easier to manage and update as your project evolves.
Best Practices for Overcoming Challenges
Embrace Modularity
Use Ceylon's module philosophy. This allows you to segment your code logically, making it easier to locate and manage files.
Utilize Ceylon’s Built-In Tools
Ceylon offers several built-in tools to manage modules effectively. Utilize them to resolve conflicts, manage dependencies, and ensure compatibility.
Maintain Updated Documentation
Keeping an updated documentation is crucial in modular projects. It helps in clarifying the purpose and functionality of each module.
The Closing Argument
Navigating the complexities of Ceylon’s Jigsaw module system doesn’t have to be a daunting task. With a clear understanding of the challenges and a set of effective solutions, developers can leverage this versatile language to build robust and maintainable applications.
By embracing the concept of modularity, utilizing Ceylon's built-in tools, and maintaining thorough documentation, you stand a better chance of overcoming the hurdles posed by the Jigsaw module system.
For further readings and resources, consider exploring the Ceylon language site and the official Java Jigsaw documentation.
Call to Action
Are you currently using Ceylon in your projects? Or do you have experiences overcoming similar challenges? Share your thoughts in the comments below.
Happy Coding!