Building Camel Web Apps Solo: Ditch Spring for Simplicity
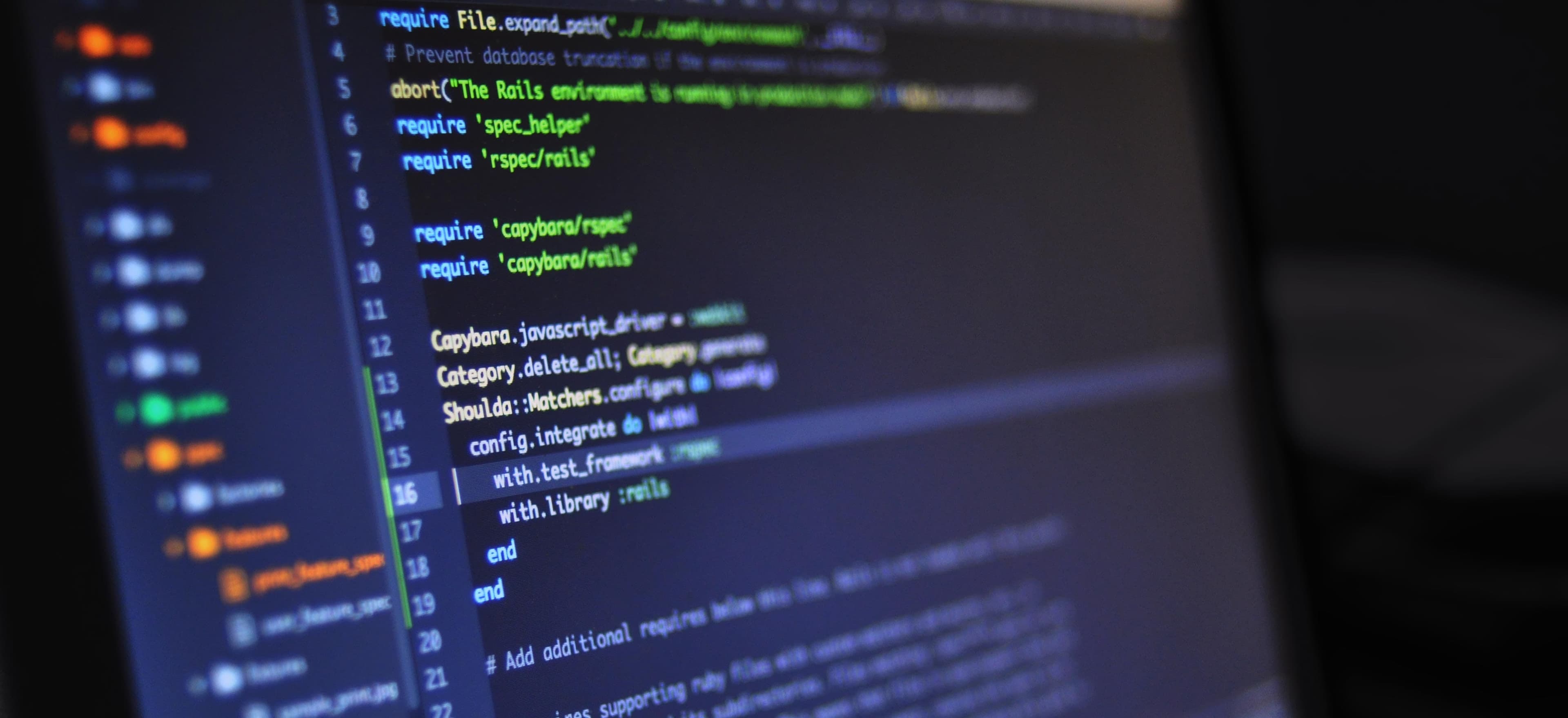
- Published on
Building Camel Web Apps Solo: Ditch Spring for Simplicity
In a world where modern web application development often gravitates toward complex frameworks like Spring, it's easy to overlook simpler alternatives. One such alternative is Apache Camel, a powerful open-source integration framework that allows developers to create robust applications without the need for excessive boilerplate code. In this post, we will explore how to build web applications using Apache Camel by utilizing its capabilities to simplify development—without relying on the entanglements of Spring.
What is Apache Camel?
Apache Camel is an integration framework that is designed to provide a rule-based routing and mediation engine. It allows developers to define routing and mediation rules in a variety of domain-specific languages (DSLs), including a Java DSL, XML, and YAML. Camel's architecture is based on the Enterprise Integration Patterns (EIP), making it a powerful choice for building integrations.
For more information about Apache Camel, you can refer to the official documentation here.
Why Choose Camel Over Spring?
1. Reduced Boilerplate Code
One of the key advantages of using Camel is the significant reduction in boilerplate code. Unlike Spring, which requires a plethora of annotations and configuration settings, Camel allows you to concentrate on the business logic.
2. Flexibility and Modularity
Camel enables you to build applications in a modular fashion. This modularity results in applications that are much easier to maintain and test.
3. Ease of Use
With its intuitive DSL, developing Camel routes can be a more straightforward process. Developers can write less code while achieving the same functionality.
4. Lightweight
Camel can be embedded into existing applications or used as a standalone service. This lightweight nature helps reduce the complexity of the application as a whole.
Setting Up Your Development Environment
Before we get started with Camel, let's set up the required tools and technologies:
- Java Development Kit (JDK): Ensure you have JDK version 8 or higher installed.
- Apache Camel Libraries: You can download the latest version of Apache Camel from here.
- Maven: Apache Camel projects often use Maven for dependency management. Ensure it is installed and configured.
Step 1: Create a New Maven Project
To create a new Maven project, use the following directory structure:
my-camel-app
│
├── pom.xml
└── src
└── main
├── java
└── resources
In the pom.xml
, include the necessary dependencies for Camel:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-camel-app</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-core</artifactId>
<version>3.x.x</version> <!-- Replace with the latest version -->
</dependency>
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-http</artifactId>
<version>3.x.x</version> <!-- Replace with the latest version -->
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
<version>1.7.30</version>
</dependency>
</dependencies>
</project>
Step 2: Create Your First Camel Route
In Apache Camel, a route is a fundamental concept that describes how messages move from one endpoint to another. Let's create a simple route that takes an HTTP request and returns a "Hello World" response.
Create a Java class named MyCamelApp.java
in src/main/java/com/example
:
package com.example;
import org.apache.camel.CamelContext;
import org.apache.camel.builder.RouteBuilder;
import org.apache.camel.impl.DefaultCamelContext;
public class MyCamelApp {
public static void main(String[] args) throws Exception {
// Create a Camel context
CamelContext context = new DefaultCamelContext();
// Add routes to the context
context.addRoutes(new RouteBuilder() {
@Override
public void configure() throws Exception {
// Define a simple route
from("jetty:http://0.0.0.0:8080/hello")
.setBody(constant("Hello World from Apache Camel!"));
}
});
// Start the Camel context
context.start();
// Keep the application running
Thread.sleep(5000);
// Stop the context
context.stop();
}
}
Code Explanation
-
CamelContext: The
CamelContext
is the runtime system of Camel where all configurations and routes are initialized. -
RouteBuilder: The
RouteBuilder
provides a fluent API for defining routes easily. -
from(): The
from()
method specifies an endpoint where messages originate. In this case, it opens an HTTP endpoint on port8080
. -
setBody(): The
setBody()
method defines the body of the message. Here we are simply returning "Hello World." -
Thread.sleep(): This keeps the application running for a specified duration.
Step 3: Run Your Application
To run your application, execute:
mvn clean compile exec:java -Dexec.mainClass="com.example.MyCamelApp"
After executing the above command, you can test your application by visiting http://localhost:8080/hello
in a web browser. You should see the response, "Hello World from Apache Camel!"
More Functionality with Camel
Now that you have created a basic Camel application, you can continue expanding its functionality. Here are some ideas you might consider:
Integration with Databases
Apache Camel allows you to connect to a variety of databases seamlessly. For instance, if you wish to fetch data from a MySQL database, you can simply add the necessary dependencies and define a new route to interact with the database.
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-sql</artifactId>
<version>3.x.x</version> <!-- Replace with the latest version -->
</dependency>
Example Route for Fetching Data
from("timer:myTimer?period=60000") // Triggers every 60 seconds
.to("jdbc:dataSource") // Assume a DataSource bean is configured
.log("Result: ${body}");
Handle JSON Data
You can also create routes that handle JSON payloads. For example, you can use Camel's JSON support to parse incoming JSON from HTTP requests and transform it as needed.
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-jackson</artifactId>
<version>3.x.x</version> <!-- Replace with the latest version -->
</dependency>
Example Route for JSON Processing
from("jetty:http://0.0.0.0:8080/processJson")
.unmarshal().json(MyJsonObject.class)
.to("bean:myBean?method=process");
Testing Your Routes
Apache Camel makes it easy to test your routes with the help of the Camel Test Kit. You can utilize tools like JUnit to write unit tests for your routes, ensuring that they behave as expected.
Here’s a simple example of how to test the Hello World
route:
import org.apache.camel.test.junit4.CamelTestSupport;
import org.junit.Test;
public class MyCamelAppTest extends CamelTestSupport {
@Test
public void testHelloWorldRoute() throws Exception {
getMockEndpoint("mock:jetty:http://0.0.0.0:8080/hello").expectedBodiesReceived("Hello World from Apache Camel!");
template.sendBody("jetty:http://0.0.0.0:8080/hello", "");
assertMockEndpointsSatisfied();
}
}
Closing the Chapter
Building web applications with Apache Camel provides developers with a wonderful opportunity to create lightweight and maintainable applications without the complexities brought on by conventional frameworks like Spring. The simplicity, flexibility, and modular design of Apache Camel can enhance your development process.
Whether you're creating simple HTTP endpoints, integrating with databases, or processing JSON, Camel provides a unified approach to application development. By focusing on integrating your systems and processes effortlessly, you can streamline your work and deliver value faster.
Additional Resources
With the rise of microservices and the need for integrations, Apache Camel offers a straightforward pathway toward achieving your goals with minimal fuss. By ditching Spring for this powerful alternative, you can craft web applications that are both efficient and easier to manage. Happy coding!