Optimize JavaFX: Save Memory with Shadow Fields
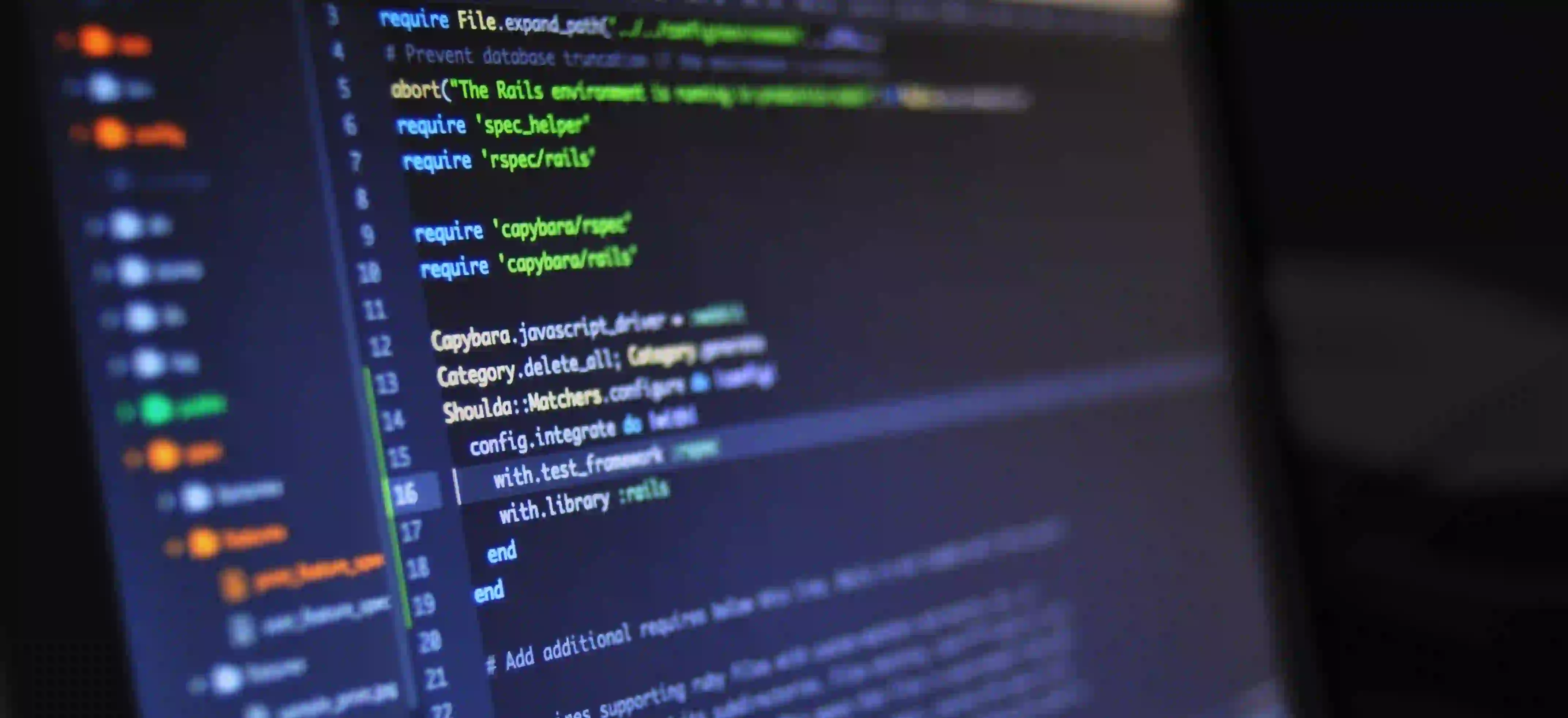
Optimize JavaFX: Save Memory with Shadow Fields
JavaFX is a powerful framework for building rich client applications in Java. One of the common challenges developers face when using JavaFX is managing memory efficiently. As applications grow in size and complexity, finding ways to optimize memory usage becomes crucial. One effective technique is the use of shadow fields.
In this blog post, we will explore what shadow fields are, how they can be used effectively in JavaFX applications, and the benefits they provide in terms of memory optimization. Additionally, we will provide you with practical code snippets to illustrate how shadow fields can be implemented.
What Are Shadow Fields?
Shadow fields are essentially private fields in a class that serve as storage for data, while allowing the public interface of that class to expose a different representation of that data. They enable developers to manage the state without unnecessarily consuming memory or exposing the class's internal structure to the outside world.
Example: Shadow Field in Java
Here’s a simple example of a class using a shadow field.
public class User {
private String shadowName;
public String getName() {
return shadowName;
}
public void setName(String name) {
this.shadowName = name.trim();
}
}
In this example, shadowName
is a shadow field. The getName
method exposes shadowName
to the outside world, while the setName
method manages how the data is set, ensuring it is trimmed before being stored. The use of shadow fields here keeps User
's internal state consistent.
Benefits of Using Shadow Fields
-
Memory Efficiency: By using shadow fields, you can avoid creating unnecessary objects that consume memory. This is particularly useful for large applications where memory usage matters.
-
Encapsulation: Shadow fields allow you to control how and when your data is accessed or modified. This means you can enforce validation and constraints conveniently.
-
Performance: By reducing the number and size of the objects created during runtime, you improve performance. Less memory allocation translates to faster garbage collection.
-
Code Clarity: Using well-named shadow fields can make your code easier to understand. They help separate internal representation from external exposure, making maintenance easier.
Implementation of Shadow Fields in JavaFX
Let’s see how shadow fields can improve memory usage in a JavaFX application. We’ll create a simple UI that displays user data. This will allow us to demonstrate the importance of memory optimizations through shadow fields.
Full Example: User Registration Form
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class UserRegistration extends Application {
private String shadowUsername;
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("User Registration");
Label label = new Label("Enter username:");
TextField usernameInput = new TextField();
Button submitButton = new Button("Submit");
submitButton.setOnAction(event -> setUsername(usernameInput.getText()));
VBox vbox = new VBox(label, usernameInput, submitButton);
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
public void setUsername(String username) {
this.shadowUsername = username != null ? username.trim() : "";
System.out.println("Username set to: " + shadowUsername);
}
public String getUsername() {
return shadowUsername;
}
}
Code Explanation
-
Memory Optimization: The
shadowUsername
field retains the username. This prevents the need for additional objects and hence saves memory. -
UI Interaction: When the button is clicked, it invokes the
setUsername
method, ensuring the username is trimmed of excess whitespace before it's assigned to the shadow field. -
Encapsulation: By controlling the access to
shadowUsername
through thesetUsername
andgetUsername
, we protect the integrity of our data.
When to Use Shadow Fields
While using shadow fields can help in various situations, consider using them when:
- You have complex objects with multiple fields to manage but wish to provide a simplified interface to your public API.
- Performance measurements indicate memory consumption is high or close to limits.
- You need to maintain a clean separation between the internal representation of your data and its external presentation.
Though using shadow fields carries benefits, like any tool, it ought to be used wisely. Over-complicating your object models with unnecessary shadow fields can lead to an unmanageable codebase.
The Bottom Line
Optimizing your JavaFX applications for better memory efficiency can significantly improve performance and usability. Shadow fields are a valuable technique in achieving this. Not only do they reduce memory overhead, but they also provide a cleaner, more manageable way to handle your data by promoting encapsulation.
For further reading on JavaFX and performance optimization techniques, consider checking out the official JavaFX documentation or delve into a more detailed coverage of Java performance with Java SE Performance.
By implementing techniques like shadow fields thoughtfully, you can ensure that your JavaFX applications are not only feature-rich but also lightweight and efficient.