Sorting Lists in Java 8: Common Lambda Expression Pitfalls
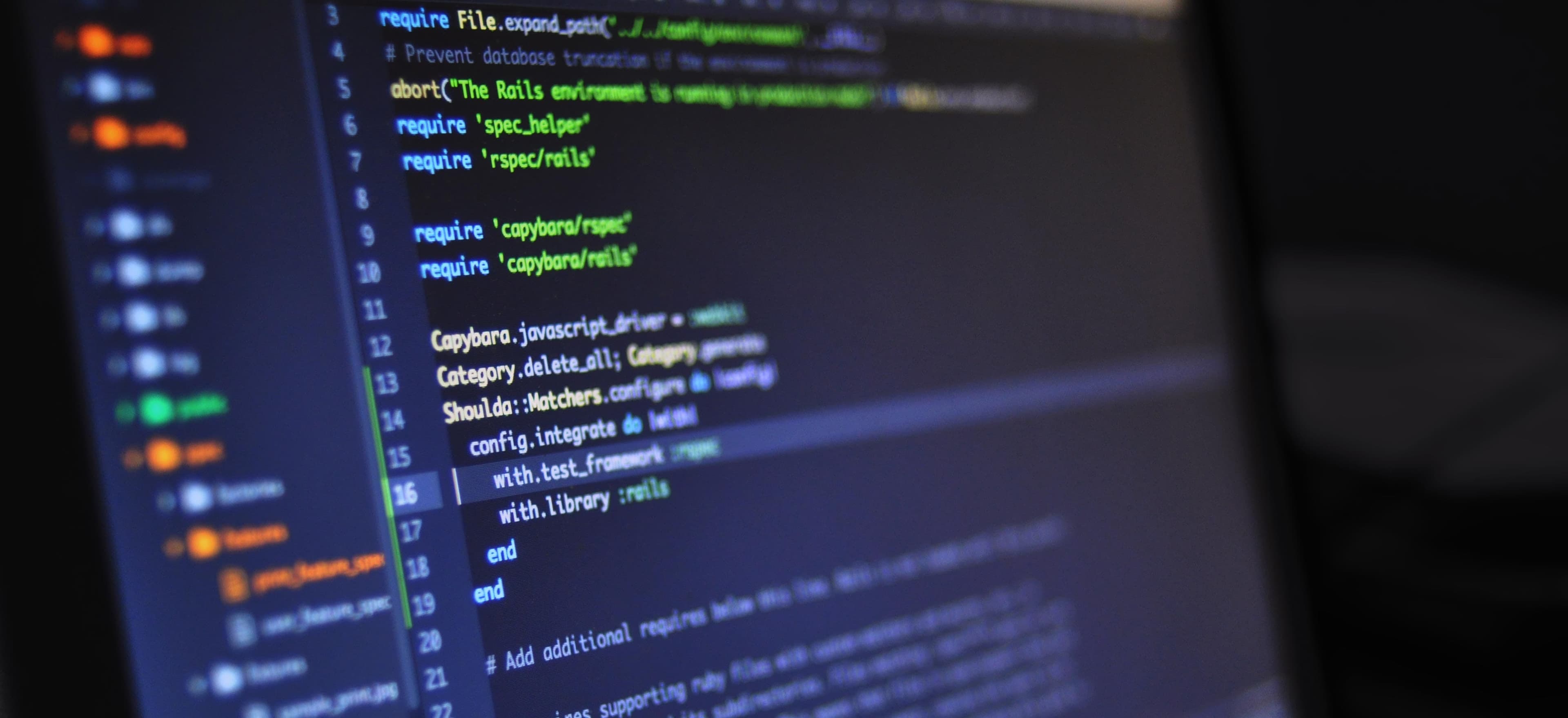
- Published on
Sorting Lists in Java 8: Common Lambda Expression Pitfalls
Java 8 brought a significant shift to the language with the introduction of lambda expressions and the Stream API, offering more concise and functional programming styles. Sorting collections, especially lists, became easier with these new tools. However, while lambda expressions streamline code, they also come with their own set of challenges and pitfalls. In this blog post, we’ll explore how to sort lists in Java 8, focusing on common errors developers encounter when using lambda expressions.
Overview of Sorting in Java 8
Before diving into the pitfalls, let's quickly cover how to sort lists in Java 8 using lambda expressions. The List
interface provides a built-in method called sort()
which can take a Comparator
. With lambda expressions, we can define comparators succinctly.
Here’s a simple example of sorting a list of strings in natural order:
List<String> names = Arrays.asList("Sarah", "John", "Claire", "David");
names.sort((s1, s2) -> s1.compareTo(s2));
System.out.println(names);
Explanation
In the code snippet above, we define a comparator using a lambda expression:
- The lambda
(s1, s2) -> s1.compareTo(s2)
tells Java how to compare two strings. compareTo
returns a negative integer ifs1
precedess2
lexicographically, zero if they are equal, and a positive integer ifs1
followss2
.
This simplicity is one of the remarkable advantages of using lambda expressions for sorting.
Avoiding Common Pitfalls
While using lambda expressions, developers often encounter a few common pitfalls. Here are some critical considerations that can save you from headaches later on.
1. Not Handling Null Values
When sorting lists, developers often forget to handle null values in the list. Attempting to sort a list that contains null elements will throw a NullPointerException
.
Example of the Pitfall
List<String> names = Arrays.asList("Sarah", null, "Claire", "David");
names.sort((s1, s2) -> s1.compareTo(s2)); // This will throw NullPointerException
Solution
Use Comparator.nullsFirst()
or Comparator.nullsLast()
to handle nulls gracefully.
names.sort(Comparator.nullsFirst((s1, s2) -> s1.compareTo(s2)));
System.out.println(names);
Explanation
Using Comparator.nullsFirst()
allows you to place any null values at the beginning of the sorted list. This ensures that the comparator does not encounter nulls, thus avoiding the exception.
2. Confusing Order of Sorting
Lambda expressions can sometimes lead to confusion about the sorting order. Particularly when sorting in descending order, developers may forget to adjust their comparison logic.
Example of the Pitfall
List<Integer> numbers = Arrays.asList(5, 3, 8, 1);
numbers.sort((n1, n2) -> n1.compareTo(n2));
System.out.println(numbers); // This sorts in ascending order
Solution
For descending order, ensure you reverse the logic in your comparator.
numbers.sort((n1, n2) -> n2.compareTo(n1));
System.out.println(numbers); // Output: [8, 5, 3, 1]
Explanation
In the modified example, we swapped n1
and n2
, which reverses the order, enabling the sort to work in descending mode.
3. Using the Wrong Data Type for Comparison
Another pitfall arises when developers don't properly account for the data types they are sorting. For instance, trying to sort a list of different object types without a proper comparator can lead to runtime exceptions.
Example of the Pitfall
List<Object> mixedList = Arrays.asList("John", 25, "Alice");
mixedList.sort((o1, o2) -> o1.toString().compareTo(o2.toString())); // Runtime Exception
Solution
Make sure that you only sort elements of the same type or implement a sufficient comparator for mixed types.
List<String> names = Arrays.asList("John", "Alice", "Daniel");
names.sort((o1, o2) -> o1.length() - o2.length()); // Sort by length
System.out.println(names); // Output: [John, Alice, Daniel]
Explanation
In this example, we compare the lengths of the names rather than the names themselves, allowing for a coherent sort.
4. Forgetting to Use the List.sort()
Method
One major oversight can be forgetting the distinction between List.sort()
and the Collections.sort()
method. Although they may seem interchangeable, they behave differently with lambda expressions.
Example of the Pitfall
Using Collections.sort()
with a lambda may lead to confusion:
List<String> names = Arrays.asList("Emma", "Olivia", "Ava", "Isabella");
Collections.sort(names, (s1, s2) -> s1.compareTo(s2)); // Works, but can be less preferable.
Solution
Prefer using the sort()
method available on the List
interface, which allows for a cleaner syntax.
names.sort((s1, s2) -> s1.compareTo(s2));
System.out.println(names);
Explanation
The List.sort
method is more direct and is the preferred choice when working with lambda expressions, enhancing readability and maintainability.
Advanced Sorting Techniques
As you get comfortable with basic sorting, you might want to delve deeper into more advanced sorting strategies, such as sorting objects with multiple fields.
Example of Multi-Field Sorting
Consider you have a Person
class that holds name
and age
. You can sort first by name
, then by age
.
class Person {
private String name;
private int age;
// Constructor, getters, and toString() omitted for brevity
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
List<Person> people = Arrays.asList(
new Person("Alice", 30),
new Person("Bob", 25),
new Person("Alice", 25)
);
people.sort(Comparator.comparing(Person::getName)
.thenComparing(Person::getAge));
people.forEach(System.out::println);
Explanation
In this example, Comparator.comparing()
is used to sort first by name
and then by age
. This flexibility is part of what makes Java 8 powerful.
To Wrap Things Up
Java 8 introduces exciting features that significantly improve how we write code, but they also require users to be mindful of potential pitfalls. Understanding these pitfalls, including handling nulls, ensuring the correct order, and correctly using data types, will make your sorting operations more effective and your code more robust.
As you learn and adapt to newer programming paradigms, ensure you keep practicing and refining your approach. For more tips on Java 8 features, consider exploring Oracle's official Java documentation.
Further Reading
For additional insights on lambda expressions and their intricacies, check out this article on Lambda Expressions in Java.
By being aware of these common pitfalls and strategies, you can leverage Java 8's sorting capabilities with confidence and clarity. Happy coding!