Common Issues After Upgrading to JDK 11: Solutions Inside
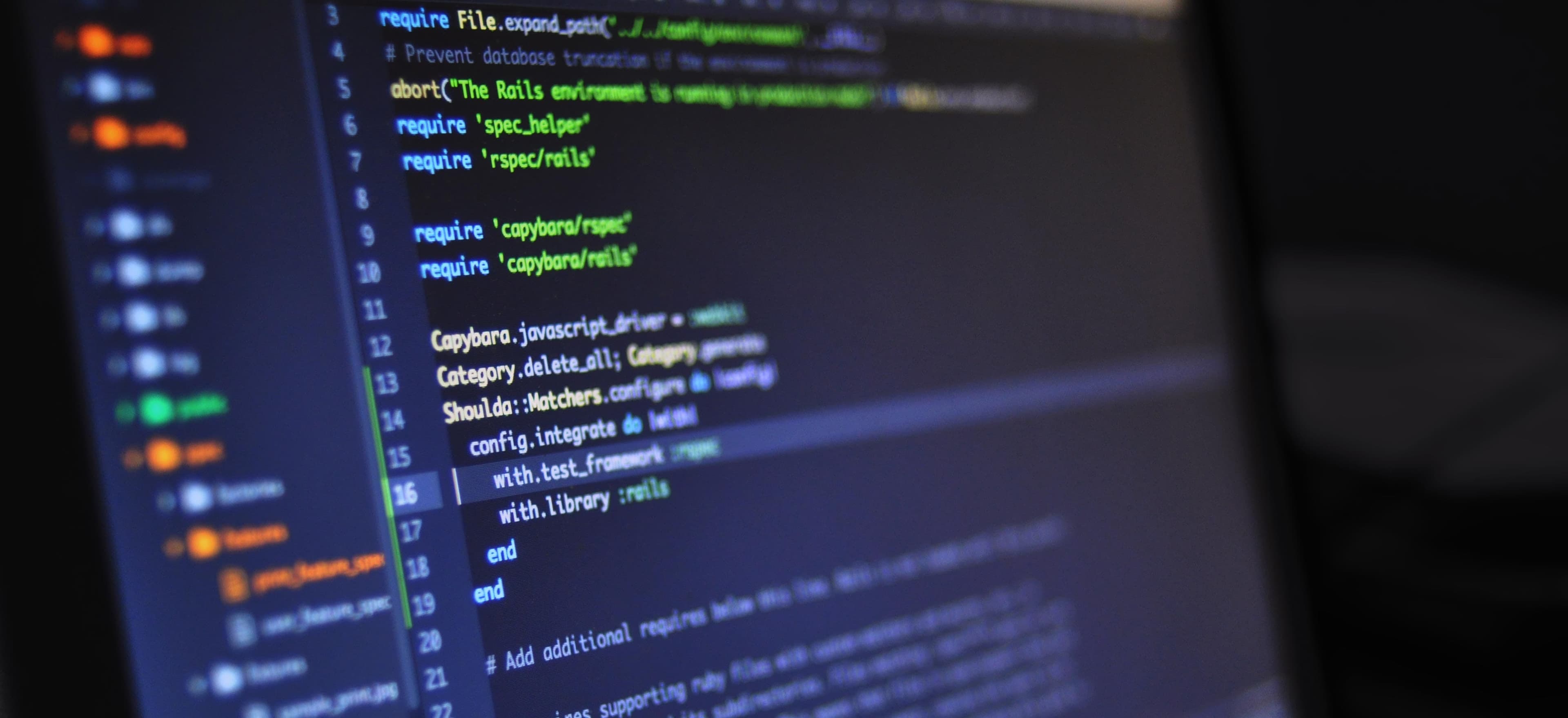
- Published on
Common Issues After Upgrading to JDK 11: Solutions Inside
Upgrading to JDK 11 offers numerous benefits, including new language features, performance improvements, and long-term support. However, it can also introduce various challenges if you are not prepared. In this post, we explore common problems developers encounter after upgrading to JDK 11 and provide solutions to address them.
Why Upgrade to JDK 11?
JDK 11 introduces several key features such as:
- Local-Variable Syntax for Lambda Parameters: This allows the use of
var
in lambda expressions. - New String Methods: Methods like
isBlank()
,lines()
, andstrip()
enhance string manipulation. - Removed Deprecated APIs: Some old APIs have been deprecated or removed, which might affect legacy code.
The reasons to upgrade far outweigh the challenges, but being prepared can save you time and headaches.
Common Issues After Upgrading to JDK 11
1. Changes in JDK Modules
With the introduction of the Java Platform Module System (JPMS) in JDK 9, JDK 11 further emphasizes modularization. A common issue is related to module access.
Symptoms
You may encounter IllegalAccessError
or java.lang.module.ResolutionException
indicating that a specific module cannot access another.
Solution
Ensure that your module-info.java
files are correctly configured. Explicitly require any modules your application uses.
Example
module my.module {
requires java.logging;
exports com.mypackage;
}
In this example, my.module
declares a dependency on the java.logging
module, allowing it to use logging functionalities.
For those using existing libraries, consider using the --add-modules
option when running your application to include necessary modules.
2. Removed Java EE and CORBA Modules
JDK 11 removed several Java EE modules and the CORBA module. If your application relies on them, you will see compilation and runtime errors.
Symptoms
Compilation errors stating that packages or classes cannot be found.
Solution
You need to replace removed modules with appropriate dependencies. For instance, you can use the Jakarta EE libraries in place of Java EE.
Example (using Maven)
<dependency>
<groupId>jakarta.persistence</groupId>
<artifactId>jakarta.persistence-api</artifactId>
<version>3.0.0</version>
</dependency>
3. Compilation Errors for Deprecated Methods
JDK 11 has removed many deprecated methods, leading to compilation issues in legacy codebases.
Symptoms
The message "method is not found" or "cannot access" may arise for deprecated methods.
Solution
Identify deprecated methods by checking the official Java API documentation. Replace the usage of deprecated methods with their recommended alternatives.
Example
If you were using Thread.stop()
, which has been deprecated, you should stop threads more safely using flag variables.
class MyRunnable implements Runnable {
private volatile boolean running = true;
public void run() {
while (running) {
// thread logic here
}
}
public void stop() {
running = false;
}
}
4. Changes in Garbage Collection
JDK 11 has introduced improvements and changes in garbage collection. The default garbage collector is the G1 collector, which may not fit all applications perfectly.
Symptoms
Performance degradation can occur, or applications may hang unexpectedly.
Solution
Profile your application’s memory usage to assess if G1 is appropriate. You can also switch to different collectors using JVM flags.
Example
To switch to the Parallel GC, use:
java -XX:+UseParallelGC -jar myapplication.jar
5. Default Character Set Changed
The default character set has changed to UTF-8 on some operating systems. This can lead to issues if your applications expect a different encoding.
Symptoms
Text data may not read correctly, and file operations can fail.
Solution
Specify the encoding explicitly in your application or configuration.
Example
BufferedReader reader = new BufferedReader(
new InputStreamReader(
new FileInputStream("file.txt"),
StandardCharsets.UTF_8));
6. Error With JavaFX Dependency
If you’re using JavaFX, you’ll encounter complications since it is no longer bundled with JDK 11.
Symptoms
Runtime errors indicating that JavaFX libraries cannot be found.
Solution
Add JavaFX as external dependencies in your build file.
Example (using Maven)
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>11.0.2</version>
</dependency>
7. New Module Path Concept
JDK 11 introduces the module path, which can lead to complications when distinguishing between classpath and module path, especially for libraries that might not be modularized.
Symptoms
ClassNotFoundExceptions and other similar issues occur when libraries are not accessible.
Solution
Use the --class-path
or --module-path
properly based on whether your libraries are in a modular format.
My Closing Thoughts on the Matter
Upgrading to JDK 11 can seem daunting due to these common issues, but understanding and preparing for them can significantly ease the process. By addressing problems related to modules, deprecated APIs, garbage collection, character sets, and external libraries, you position your application for success.
For a deeper understanding, consider exploring these additional resources:
- Java Platform Module System
- Removing Java EE Modules
- Garbage Collection Tuning
Stay up-to-date with future Java releases and best practices to ensure smooth transitions. Embrace the changes, and happy coding!