Unlocking the Hidden CLOB and BLOB Methods in JDBC 4.0
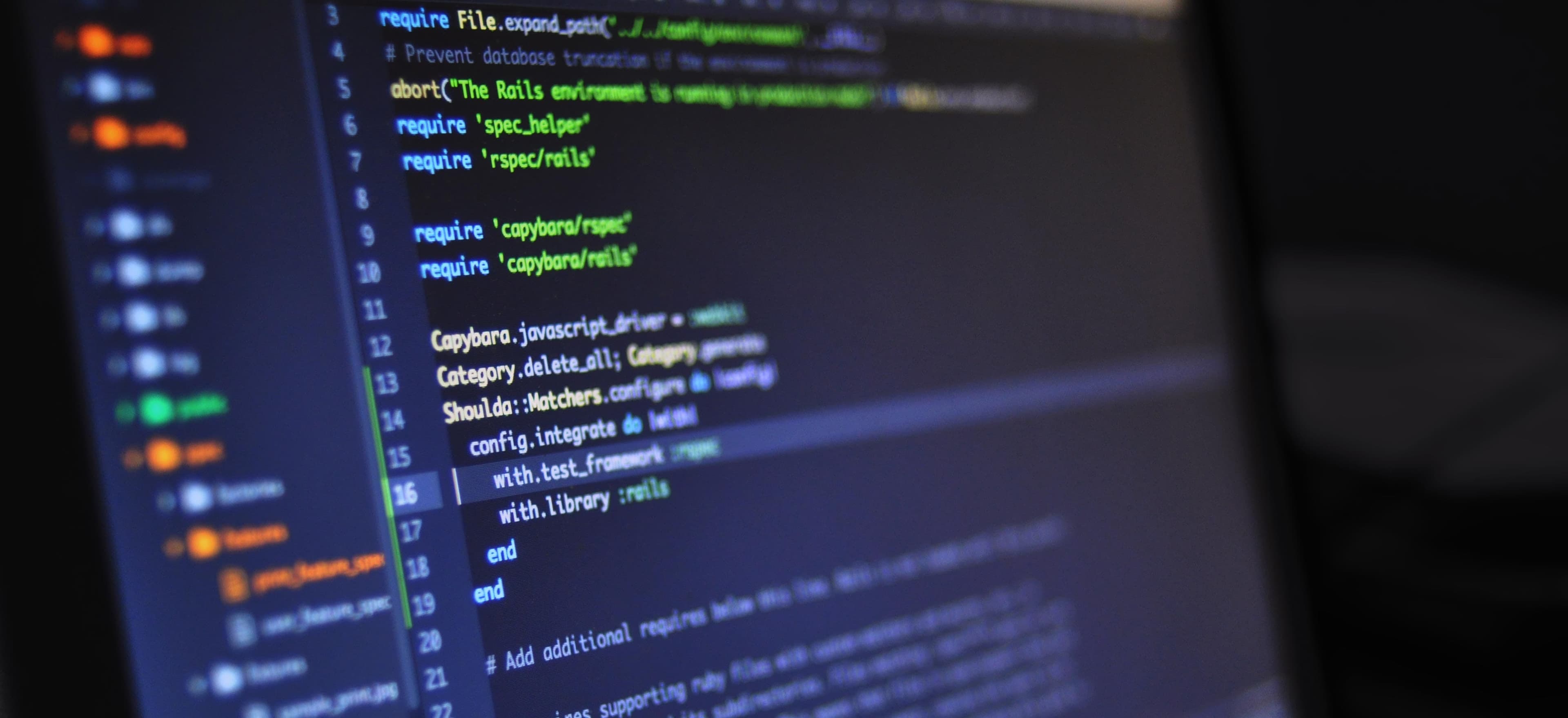
- Published on
Unlocking the Hidden CLOB and BLOB Methods in JDBC 4.0
Java Database Connectivity (JDBC) provides developers with a powerful framework for interacting with relational databases. Among its various features, JDBC 4.0 has introduced efficient methods to manage Character Large OBjects (CLOB) and Binary Large OBjects (BLOB). In this post, we will explore how to use these methods effectively, discussing their significance, providing illustrative code snippets, and revealing best practices.
Understanding CLOB and BLOB
Before diving into the specifics, let's clarify what CLOB and BLOB are.
- CLOB: A Character Large Object, primarily used for storing large text data. Databases may utilize CLOB for text strings exceeding 4,000 characters.
- BLOB: A Binary Large Object, intended for storing binary data such as images, audio, or multimedia files, which can exceed 64 KB in size.
With JDBC 4.0, the handling of these data types became more straightforward. Now, let's examine the new methods and how they can be leveraged.
Setup: JDBC 4.0 Environment
First, make sure you have the JDBC driver for your database. In this example, we will focus on MySQL, but the principles can apply to various database environments.
Maven Dependency for MySQL Connector
If you are using Maven, include the following dependency in your pom.xml
:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.27</version> <!-- Check for the latest version -->
</dependency>
Sample Database Preparation
Ensure that your database has tables designed to handle CLOB and BLOB data types. For example, use the following SQL to create tables.
CREATE TABLE documents (
id INT AUTO_INCREMENT PRIMARY KEY,
doc_data CLOB,
image_data BLOB
);
Inserting CLOB Data
We'll begin with inserting a CLOB. The following code snippet demonstrates how to handle the insertion of large text data into the database.
Code Snippet: Inserting CLOB
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class InsertCLOB {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourdatabase";
String user = "root";
String password = "yourpassword";
String longText = "This is a long text..."; // Assume this is longer than 4,000 characters
try (Connection con = DriverManager.getConnection(url, user, password)) {
String sql = "INSERT INTO documents (doc_data) VALUES (?)";
PreparedStatement pstmt = con.prepareStatement(sql);
pstmt.setCharacterStream(1, new java.io.StringReader(longText), longText.length());
pstmt.executeUpdate();
System.out.println("CLOB Inserted Successfully.");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Why This Code Works
- StringReader: We used
StringReader
to wrap the long text. This allows JDBC to read the text data as a stream, which is crucial for handling large datasets. - setCharacterStream: This method is pivotal for CLOB data types as it helps manage larger text sizes efficiently.
Retrieving CLOB Data
Retrieving CLOB data is just as simple. Here is how you can read CLOB from the database.
Code Snippet: Retrieving CLOB
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class RetrieveCLOB {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourdatabase";
String user = "root";
String password = "yourpassword";
try (Connection con = DriverManager.getConnection(url, user, password)) {
String sql = "SELECT doc_data FROM documents WHERE id = 1";
Statement stmt = con.createStatement();
ResultSet rs = stmt.executeQuery(sql);
if (rs.next()) {
java.io.Reader reader = rs.getCharacterStream("doc_data");
StringBuilder sb = new StringBuilder();
int ch;
while ((ch = reader.read()) != -1) {
sb.append((char) ch);
}
System.out.println("CLOB Retrieved: " + sb.toString());
}
} catch (SQLException | IOException e) {
e.printStackTrace();
}
}
}
Why This Code Works
- getCharacterStream: This method retrieves the CLOB data as a character stream, allowing us to read the data efficiently.
- StringBuilder: Useful for collecting characters into a string, especially when reading from a stream.
Inserting BLOB Data
Now, let's tackle how to insert binary data into a BLOB column.
Code Snippet: Inserting BLOB
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.File;
public class InsertBLOB {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourdatabase";
String user = "root";
String password = "yourpassword";
String filePath = "path/to/your/image.png"; // Specify your image file path
try (Connection con = DriverManager.getConnection(url, user, password);
FileInputStream fis = new FileInputStream(new File(filePath))) {
String sql = "INSERT INTO documents (image_data) VALUES (?)";
PreparedStatement pstmt = con.prepareStatement(sql);
pstmt.setBinaryStream(1, fis, (int) new File(filePath).length());
pstmt.executeUpdate();
System.out.println("BLOB Inserted Successfully.");
} catch (SQLException | IOException e) {
e.printStackTrace();
}
}
}
Why This Code Works
- FileInputStream: This allows for reading the binary data from a file efficiently.
- setBinaryStream: It maps the binary stream to the SQL BLOB type.
Retrieving BLOB Data
Finally, let’s look at retrieving BLOB data from the database.
Code Snippet: Retrieving BLOB
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.io.FileOutputStream;
import java.io.IOException;
public class RetrieveBLOB {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourdatabase";
String user = "root";
String password = "yourpassword";
try (Connection con = DriverManager.getConnection(url, user, password)) {
String sql = "SELECT image_data FROM documents WHERE id = 1";
Statement stmt = con.createStatement();
ResultSet rs = stmt.executeQuery(sql);
if (rs.next()) {
java.sql.Blob blob = rs.getBlob("image_data");
byte[] imageBytes = blob.getBytes(1, (int) blob.length());
try (FileOutputStream fos = new FileOutputStream("output_image.png")) {
fos.write(imageBytes);
}
System.out.println("BLOB Retrieved Successfully.");
}
} catch (SQLException | IOException e) {
e.printStackTrace();
}
}
}
Why This Code Works
- getBlob: This retrieves the BLOB data from the database.
- getBytes: Converts the BLOB to a byte array for easy manipulation or saving to a file.
Bringing It All Together
In this post, we've thoroughly explored how to manage CLOB and BLOB data types using JDBC 4.0. From inserting large text or binary files to retrieving them back, the principles and examples demonstrate the ease and effectiveness of these operations.
By leveraging setCharacterStream
, setBinaryStream
, and their corresponding retrieval methods, developers can handle large volumes of data seamlessly. Always keep performance in mind; streaming data helps prevent memory overflow when dealing with vast amounts of information.
For further reading on JDBC and database handling, explore the following links:
Incorporate this knowledge into your projects and unlock the full potential of your Java applications!