Understanding Immutable vs Mutable Objects: A Common Pitfall
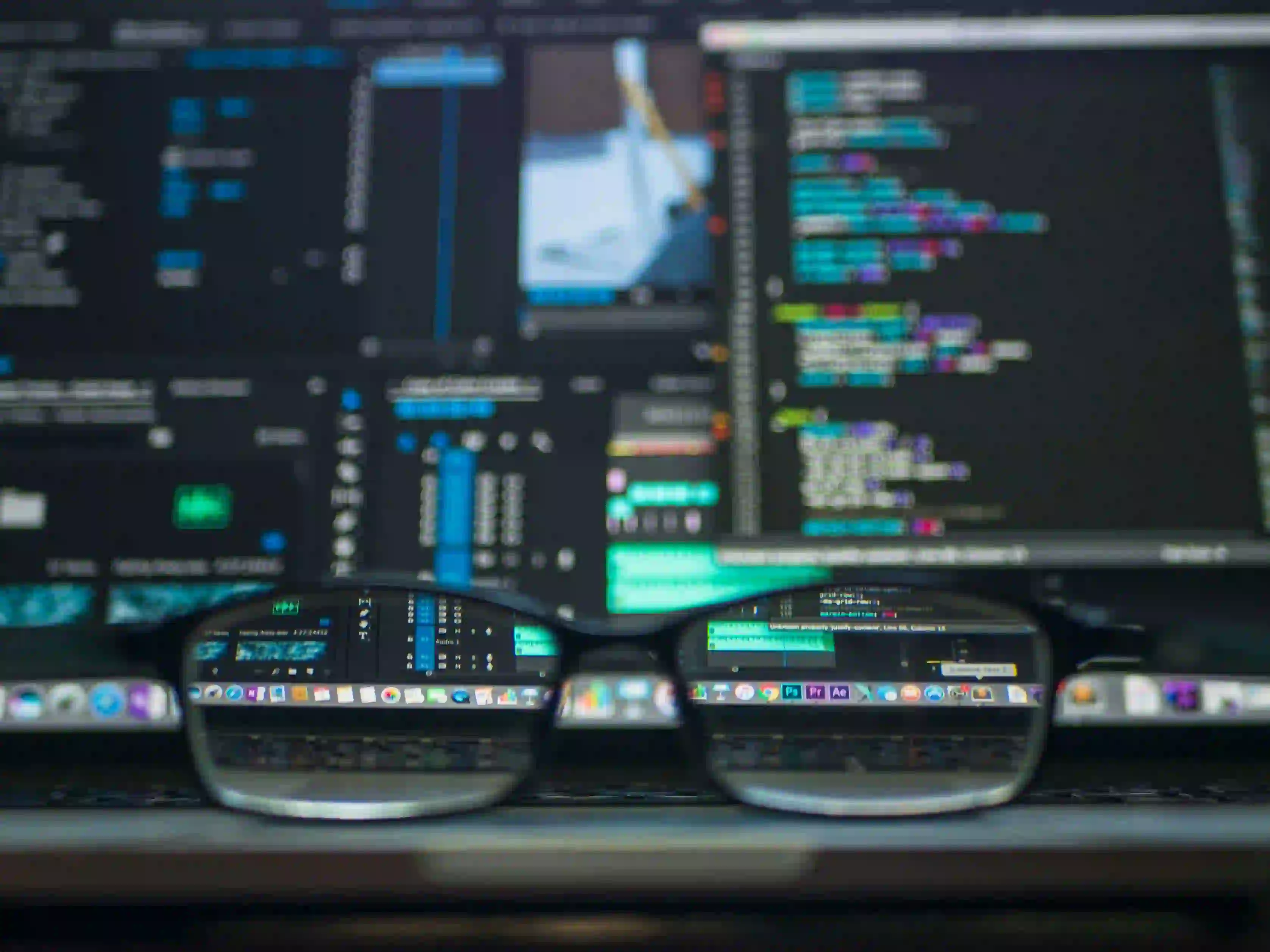
Understanding Immutable vs Mutable Objects: A Common Pitfall
In the vast world of Java programming, understanding the distinction between immutable and mutable objects is crucial. As developers, the decisions we make about object mutability can impact performance, security, and overall design. In this blog post, we'll dive deep into these concepts, explore the advantages and disadvantages, and highlight the common pitfalls arising from misuse.
What Are Mutable and Immutable Objects?
Before we go further, let's clarify what we mean by mutable and immutable objects.
Mutable Objects: These are objects whose state can be modified after they are created. For example, in Java, an instance of an ArrayList
is mutable because you can add or remove items from it.
Immutable Objects: In contrast, immutable objects cannot be changed once they are created. The Java String
class is a prime example. Once you create a string, you cannot alter its contents; any modification will result in the creation of a new string.
Why It Matters
The choice of using mutable or immutable objects can directly affect your code’s predictability and behavior. Here are some key considerations:
-
Thread Safety: Immutable objects are inherently thread-safe. You can share them across multiple threads without synchronizing access. This avoids the complexities often associated with mutable objects, where changes could affect shared state.
-
Hashing and Caching: Immutable objects make excellent keys in hash-based collections like
HashMap
. Since their state doesn’t change, they will consistently produce the same hash code throughout their lifecycle. -
Ease of Use: Working with immutable objects can lead to cleaner, more understandable code. You don’t have to manage the object’s state, making debugging considerably easier.
Mutable Objects: The Pitfall
Despite the advantages of immutable objects, mutable ones still play a crucial role in Java programming, especially when performance and memory use are top priorities. However, reliance on mutable objects comes with risks, often leading to common pitfalls.
Example of Mutable Objects
Here's a simple example of a mutable class:
public class MutablePoint {
private int x;
private int y;
public MutablePoint(int x, int y) {
this.x = x;
this.y = y;
}
public void move(int deltaX, int deltaY) {
this.x += deltaX;
this.y += deltaY;
}
@Override
public String toString() {
return "Point(" + x + ", " + y + ")";
}
}
In the above code:
MutablePoint
is mutable because you can change itsx
andy
values.- The
move
method allows external components to modify the state of an object.
This flexibility can lead to unintentional side effects. Multiple parts of a program may change the same MutablePoint
object, leading to unpredictable behavior.
Immutable Objects: A Safer Alternative
Let’s now contrast this with an immutable object:
public final class ImmutablePoint {
private final int x;
private final int y;
public ImmutablePoint(int x, int y) {
this.x = x;
this.y = y;
}
public ImmutablePoint move(int deltaX, int deltaY) {
return new ImmutablePoint(this.x + deltaX, this.y + deltaY);
}
@Override
public String toString() {
return "Point(" + x + ", " + y + ")";
}
}
In this code:
ImmutablePoint
is immutable because it doesn't allow any changes to itsx
andy
values after creation.- The
move
method doesn’t modify the current object. Instead, it returns a new instance with the updated coordinates.
The Trade-Offs
While immutable objects offer advantages, they are not without their own trade-offs:
-
Performance Cost: Creating new instances can incur performance overhead, particularly in high-frequency operations where many changes are required. This is, however, often mitigated through clever design patterns, such as the use of builders.
-
Memory Consumption: Immutable objects can lead to higher memory usage in situations where many instances are created for minor changes. This can be a concern in limited resource environments.
When to Use Which
Choosing between mutable and immutable objects depends on the specific requirements and constraints of your application.
When to Use Mutable Objects:
- When you need to perform a lot of modifications on an object.
- For performance-sensitive applications where object creation is costly.
When to Use Immutable Objects:
- When thread safety is paramount.
- When you want to create API designs that are easier to use and reason about.
- When working with collections where object keys should remain stable, such as in
HashMap
.
Best Practices
-
Favor Immutability: As a general rule, aim for immutable objects unless mutability is explicitly required.
-
Document Changes: If a mutable object is necessary, ensure you document all modifications clearly. In larger systems, clarity helps avoid confusion.
-
Use Defensive Copies: When exposing mutable objects through methods, consider returning a copy instead of the original. This practice avoids unwanted side effects.
public class SafePoint {
private final int[] coordinates;
public SafePoint(int x, int y) {
this.coordinates = new int[]{x, y};
}
public int[] getCoordinates() {
return coordinates.clone(); // Return a copy
}
}
In this example, SafePoint
offers an array through a defensive approach, allowing the user to interact without risking state changes.
Lessons Learned
In summary, understanding the differences between mutable and immutable objects in Java is not just an academic exercise; it directly impacts application design and behavior. By balancing between these two paradigms, developers can craft optimal solutions tailored to program requirements.
For further reading, don't miss these valuable resources:
- Java Documentation on String Immutability
- Effective Java by Joshua Bloch - A must-read for Java developers.
By understanding, applying, and knowing when to shift between visibility and mutability, developers can steer clear of common pitfalls, ensuring the robustness and reliability of their code. Happy coding!