Mastering File Permissions: Make Java Files Read-Only
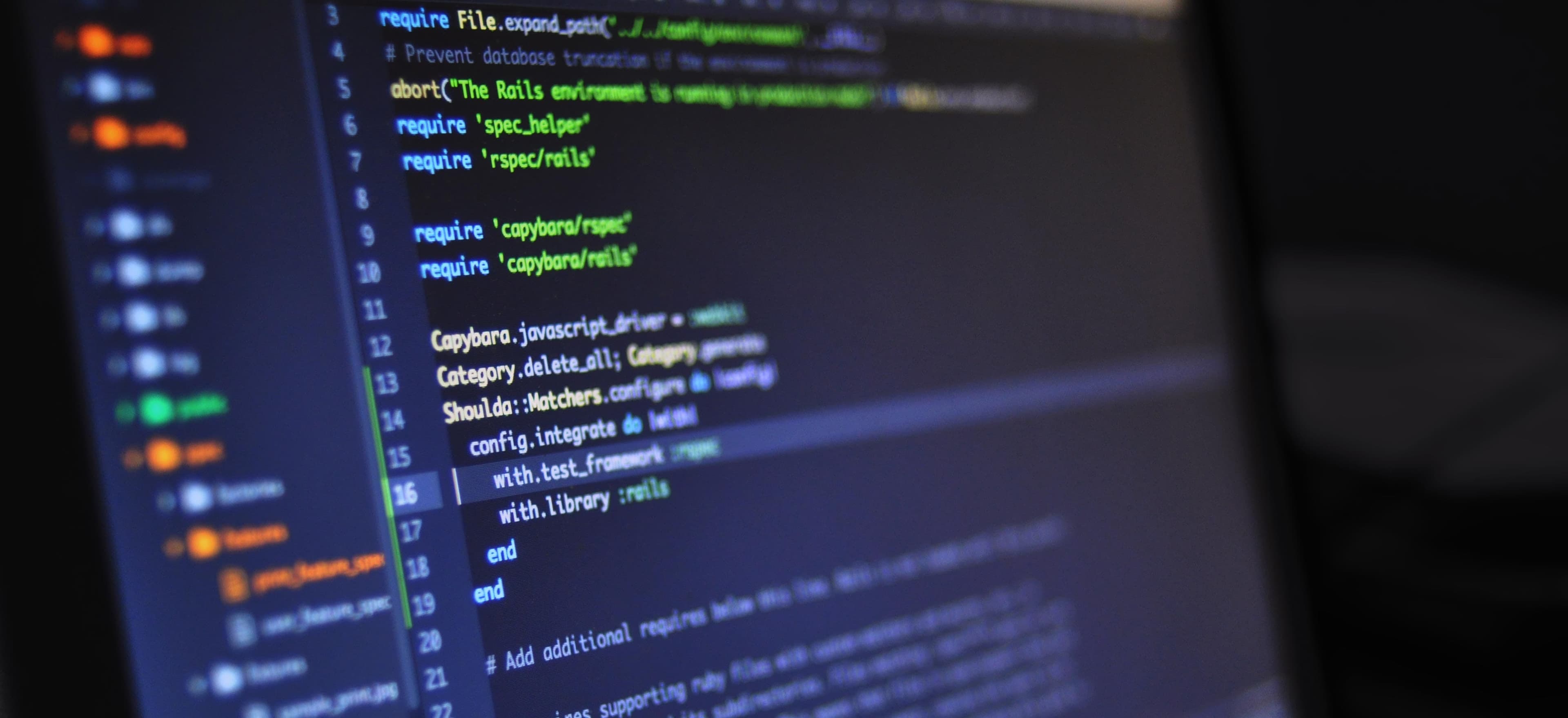
- Published on
Mastering File Permissions: Make Java Files Read-Only
When dealing with file systems in Java, you may often find a need to manage permissions, particularly when you wish to set a file as read-only. This control not only helps maintain data integrity but also protects sensitive information. In this blog post, we'll outline best practices for making Java files read-only, discuss the significance of file permissions, and provide code snippets with clear explanations.
Why File Permissions Matter
File permissions define how files can be accessed and modified. They serve as a critical security feature that helps:
- Protect sensitive data from unauthorized access and modifications.
- Preserve the integrity of configuration files and application data.
- Help manage user permissions in multi-user environments.
Consider a scenario where your Java application writes sensitive configuration files. If these files are not set to read-only, malicious users or unintentional processes could overwrite or alter these files, causing potential disruptions to your application.
Understanding File Attributes in Java
Java provides a File
class that allows developers to interact with the file system. However, not all file handling or permission management can be done with the File
class alone. From Java 7 onward, the new NIO file system package (java.nio.file
) introduced more advanced file operations, including changing file attributes.
To set a file to read-only, we primarily work with the Files
class from java.nio.file
package. This class provides methods for manipulating file attributes conveniently.
Making a File Read-Only
To make a file read-only in Java, you can use the Files
class as follows:
- Import Necessary Packages
First, you'll need to import the required classes:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
- Set the File Attribute
The setAttribute
method of the Files
class allows you to change the file property. Here’s how you can make a file read-only:
public class FilePermissionExample {
public static void main(String[] args) {
// Specify the path to your file
String filePath = "example.txt";
// Create a Path object
Path path = Paths.get(filePath);
try {
// Set the file to read-only
Files.setAttribute(path, "dos:readonly", true);
System.out.println("File " + filePath + " is now read-only.");
} catch (IOException e) {
System.err.println("An error occurred while setting the file permission: " + e.getMessage());
}
}
}
Code Explanation
- Path Object: We create a
Path
object representing the file location. - setAttribute Method: We invoke
Files.setAttribute
, specifying the target attribute ("dos:readonly"
for Windows, which makes a file read-only). - Error Handling: Using a try-catch block ensures that we can handle any IOException that may occur when attempting to set the attribute.
Important Note on File Attributes
File attributes can vary based on the operating system. The "dos:readonly"
attribute specifically works for files in a DOS filesystem (primarily Windows). For UNIX-like systems, a different approach is required.
Cross-Platform Considerations
If you are developing a Java application that is expected to run on multiple platforms, you should be cautious of how file permissions are managed. For example:
- Windows: Uses a DOS-like permission model.
- Unix/Linux: Uses owner, group, and other permissions which can be altered using
chmod
. Setting read-only in Unix would typically mean changing the permission to444
.
Here's a simple example of how you could use command-line interactions to change file permissions in a Unix-like system:
public class UnixFilePermissionExample {
public static void main(String[] args) {
String filePath = "example.txt";
try {
Process process = Runtime.getRuntime().exec("chmod 444 " + filePath);
process.waitFor();
System.out.println("File " + filePath + " is now set to read-only on Unix.");
} catch (IOException | InterruptedException e) {
System.err.println("An error occurred: " + e.getMessage());
}
}
}
Explanation of the Unix Example
- Runtime.exec(): This method allows execution of system commands. We invoke
chmod 444 <filename>
which sets the file's permission to read-only for everyone. - Process.waitFor(): It's good practice to wait for the process to complete before moving on to ensure that the command was successful.
Checking File Permissions
Before attempting to change a file's status, it's often useful to check its current permission settings. Here's how you can do it:
public class CheckFilePermission {
public static void main(String[] args) {
String filePath = "example.txt";
Path path = Paths.get(filePath);
try {
boolean isReadable = Files.isReadable(path);
boolean isWritable = Files.isWritable(path);
boolean isExecutable = Files.isExecutable(path);
System.out.println("Read permission: " + isReadable);
System.out.println("Write permission: " + isWritable);
System.out.println("Execute permission: " + isExecutable);
} catch (IOException e) {
System.err.println("An error occurred while checking file permissions: " + e.getMessage());
}
}
}
Explanation of Permission Check
- isReadable, isWritable, isExecutable: These methods return boolean values indicating whether the file has the respective permissions.
Wrapping Up
Managing file permissions is essential when developing robust Java applications. Whether you are setting a file to read-only for security reasons or managing permissions in a multi-user environment, understanding how to manipulate file attributes programmatically is crucial.
For more on Java File I/O, check out the official Java Documentation and consider exploring the NIO package further for more advanced file handling capabilities.
Engage with file permission management today and ensure your application handles sensitive data securely. Whether you are modifying files, enforcing read-only status, or checking permission settings, you now have a solid foundation to tackle file permissions in Java.
Feel free to leave your comments below or share any additional tips about managing file permissions effectively. Happy coding!
By providing a clear and structured approach along with practical code samples, we’ve equipped you with the knowledge needed to make Java files read-only across different platforms. Whether you're a seasoned developer or just started exploring Java, mastering file permissions will enhance the integrity and security of your applications.
Checkout our other articles