Struggling with JavaFX Responsive Design? Here’s How!
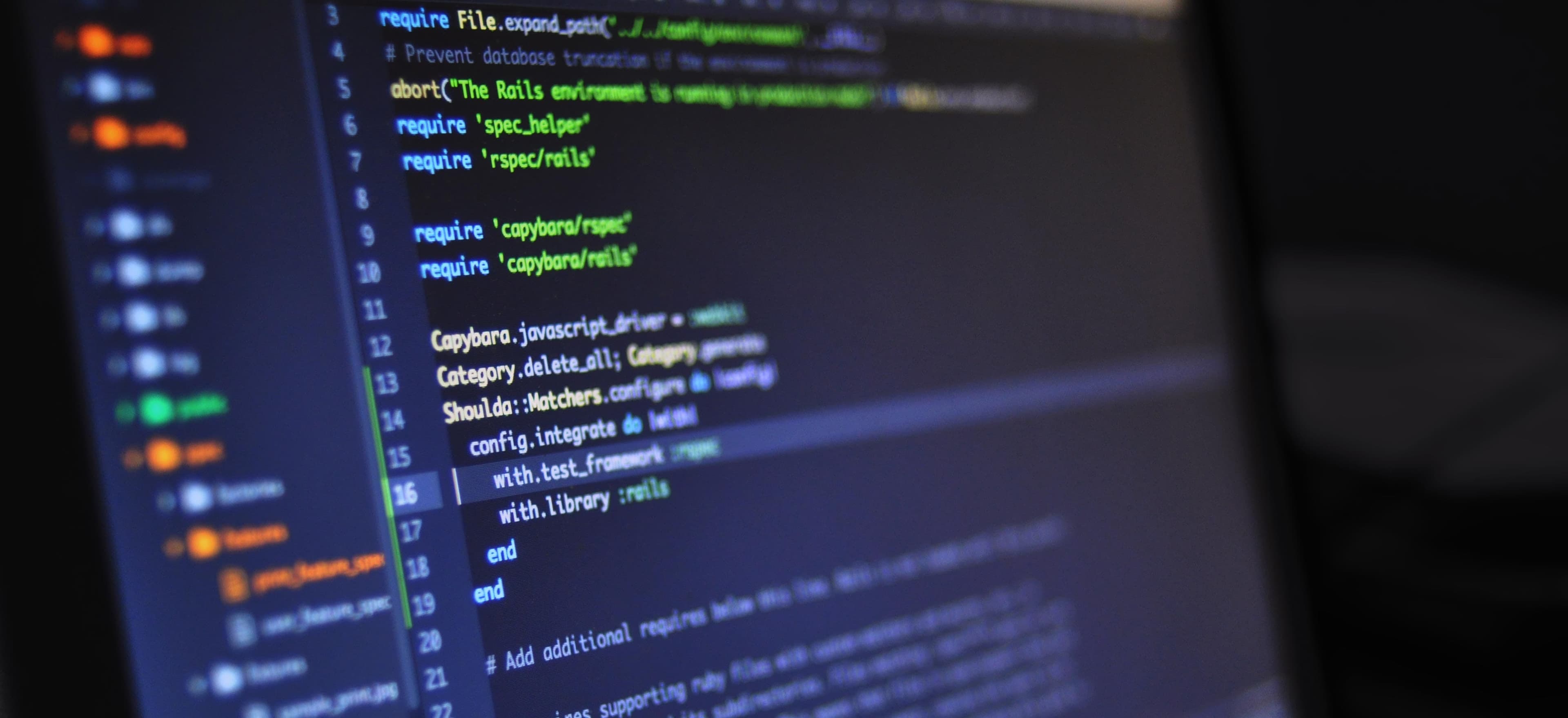
- Published on
Struggling with JavaFX Responsive Design? Here’s How!
Creating responsive applications is essential in today’s multi-device world. Users expect applications to adapt to various screen sizes and orientations seamlessly. JavaFX is a powerful framework for building Java applications, and while it does offer a rich set of components, developing responsive layouts can be challenging. In this post, we’ll delve into effective strategies for creating responsive designs in JavaFX.
Table of Contents
- Understanding JavaFX Layouts
- Utilizing Layout Pane for Responsiveness
- Exploring CSS in JavaFX
- Making Use of Anchors and Bindings
- Handling Resizing Events
- Conclusion and Best Practices
1. Understanding JavaFX Layouts
Before diving into responsive design strategies, it's essential to understand the JavaFX layout system. JavaFX offers various layout panes—VBox
, HBox
, GridPane
, and BorderPane
, among others. These layouts organize nodes (UI components) in a specific arrangement and manage their resizing behavior.
Example: Basic Layouts
Here's a simple example of how VBox
and HBox
operate:
VBox vbox = new VBox(); // Vertical box
HBox hbox = new HBox(); // Horizontal box
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
vbox.getChildren().addAll(button1, hbox);
hbox.getChildren().add(button2);
In this snippet, we create a vertical layout containing two buttons. It’s important to know how these layouts behave since they serve as the foundation for responsive design.
2. Utilizing Layout Pane for Responsiveness
To ensure your application looks good on any screen, utilizing a combination of layout panes is key. For example, wrapping multiple panes inside a BorderPane
is a common practice that allows the whole application to maintain structure.
Example: Combining Layouts
Here’s how you could structure a layout using BorderPane
:
BorderPane borderPane = new BorderPane();
VBox leftPane = new VBox(); // Left section
HBox topPane = new HBox(); // Top section
BorderPane centerPane = new BorderPane();
borderPane.setLeft(leftPane);
borderPane.setTop(topPane);
borderPane.setCenter(centerPane);
This structure allows the UI to grow and shrink responsively. The elements placed in the BorderPane
will adjust according to the window size.
3. Exploring CSS in JavaFX
JavaFX also supports CSS, enabling you to apply styles dynamically. With responsive design, you can change the CSS properties based on screen size.
Example: CSS Styles
.button {
-fx-font-size: 14px;
}
@media screen and (max-width: 600px) {
.button {
-fx-font-size: 12px; /* Smaller font for small screens */
}
}
In the above CSS, we've defined a base style for a button and adjusted the font size for screens smaller than 600 pixels. This is crucial for maintaining usability on smaller devices.
4. Making Use of Anchors and Bindings
JavaFX introduces bindings, which are a powerful tool for creating responsive UI components. By binding properties of UI elements to the properties of their parent containers, you can ensure that changes are reflected instantly.
Example: Property Binding
Consider the following code snippet:
Label label = new Label("Resizable label");
label.prefWidthProperty().bind(borderPane.widthProperty().multiply(0.75)); // 75% of parent's width
In this example, the label's preferred width automatically adjusts to 75% of the BorderPane
width. This kind of binding ensures that the UI components react dynamically to any size changes, making it a critical aspect of responsive design.
5. Handling Resizing Events
Another strategy for responsive design is to watch for resizing events and adjust your layout accordingly. This is especially useful in more complex UIs where components must reposition or resize based on available space.
Example: Resize Listener
primaryStage.widthProperty().addListener((obs, oldVal, newVal) -> {
if (newVal.doubleValue() < 500) {
// Logic for changing layout for small sizes
} else {
// Logic for larger sizes
}
});
In this snippet, we utilize a listener on the primary stage’s width property to define behavior depending on the screen size. This allows you to programmatically adjust layouts based on conditions you specify.
6. Conclusion and Best Practices
Designing responsive JavaFX applications requires a thoughtful approach to layout management, CSS usage, bindings, and event handling. Here are some best practices to keep in mind:
- Use the Right Layouts: Choose layouts that fit your needs.
GridPane
is useful for grid-like designs, whileStackPane
can help overlay elements. - Leverage Bindings: Utilize property bindings to ensure elements scale and adjust automatically with the window size.
- Test on Multiple Screens: Always run your application on various screen sizes to ensure it looks and performs as expected.
- Optimize CSS: Use media queries in your CSS to adapt styles based on screen resolutions. This enhances usability and aesthetics.
- Listen to Resizing Events: Implement logic to adjust the interface based on dynamic content or resizing events.
By mastering these techniques, you can create engaging, responsive JavaFX applications that provide a seamless experience across different devices. For more in-depth information on JavaFX, check out the official JavaFX documentation.
Happy coding!
Checkout our other articles