Effortlessly Manage Checked Checkboxes in Java Modal Forms
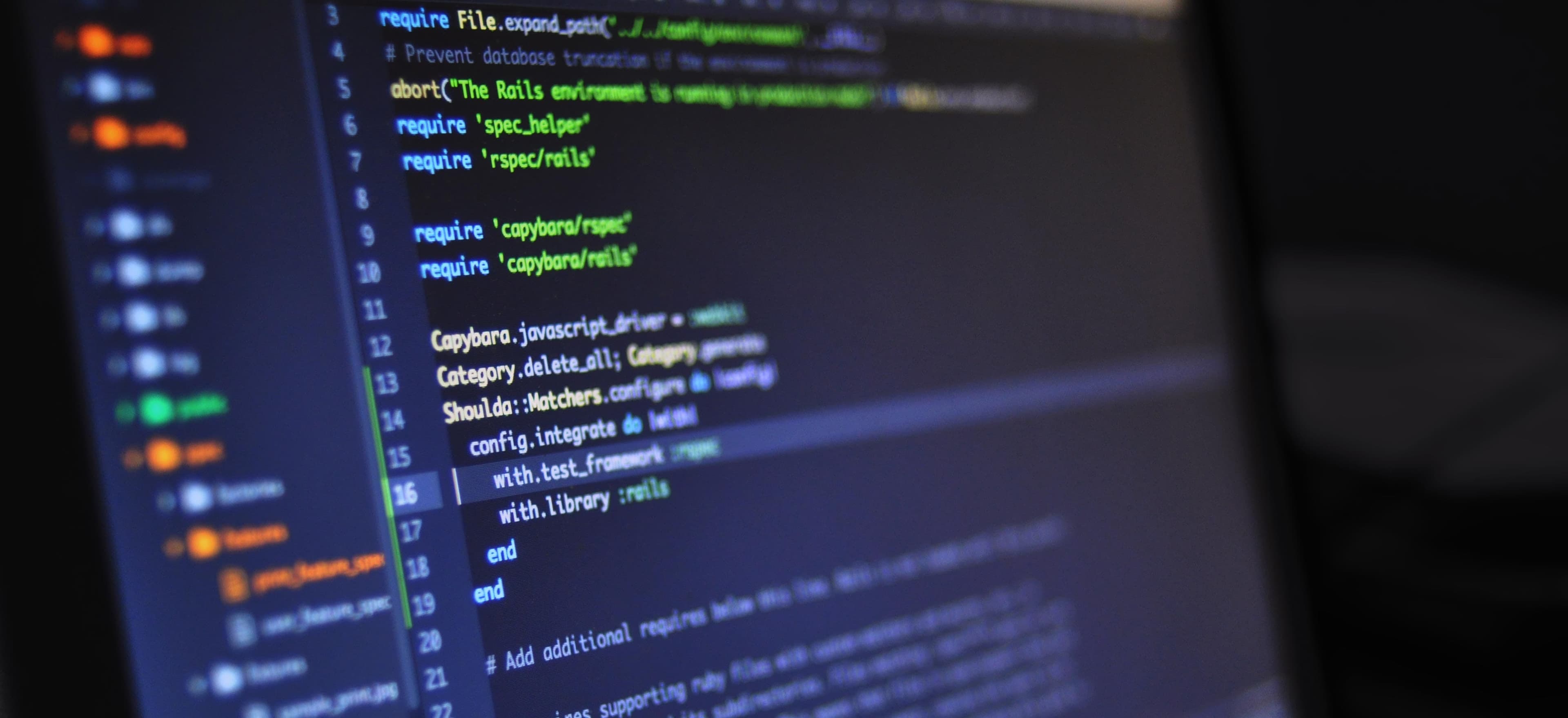
- Published on
Effortlessly Manage Checked Checkboxes in Java Modal Forms
Checkboxes are common UI components used for collecting user preferences in forms. In Java applications, especially those utilizing Java Swing or JavaFX, managing the state of these checkboxes can be pivotal for ensuring a smooth user experience. This article will explore how to effectively manage checked checkboxes, particularly when dealing with modal forms—a feature that’s often utilized to capture user input without navigating away from the main application.
In this post, we will:
- Discuss the importance of checkbox management in modal forms.
- Provide step-by-step guidance on implementing a checkbox feature in a Java Swing modal dialog.
- Illustrate best practices through code snippets.
- Reference the article Displaying Checked Checkbox Elements in Modal for further insights.
Understanding Checkbox State Management
Checkboxes allow users to select one or multiple options from a set of choices. They can be either checked (true) or unchecked (false). Managing their state effectively is crucial in scenarios where users expect seamless interaction. When using modals, it becomes necessary to remember the state of each checkbox even if the dialog is opened multiple times.
Benefits of Managing Checkbox States
- User Experience: A well-managed checkbox state reduces user frustration, as they won't have to reselect options every time a modal is opened.
- Data Integrity: Ensures that the correct data is passed back to the application when the user closes the modal.
Creating a Modal Dialog with Checkboxes in Java Swing
Let's create a simple example of a modal dialog that contains several checkboxes.
Step 1: Setup Your Java Swing Environment
Ensure you have a Java development environment set up. You can use IDEs like IntelliJ IDEA, Eclipse, or even a simple text editor with command-line tools.
Step 2: Create the Main Application Frame
We'll start by creating the main application window. Below is a simple outline:
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class MainApp extends JFrame {
public MainApp() {
setTitle("Checkbox Modal Example");
setSize(400, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
JButton openModalButton = new JButton("Open Modal");
openModalButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
CheckboxModal dialog = new CheckboxModal(MainApp.this);
dialog.setVisible(true);
}
});
add(openModalButton, BorderLayout.CENTER);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
MainApp app = new MainApp();
app.setVisible(true);
});
}
}
Code Explanation
- The
MainApp
class extendsJFrame
, which is the main window of our application. - We create a button that initializes the
CheckboxModal
class, which we will define next.
Step 3: Creating the Modal with Checkboxes
Now, let's define the CheckboxModal
class, which will handle the checkbox states.
class CheckboxModal extends JDialog {
private JCheckBox option1;
private JCheckBox option2;
private JCheckBox option3;
public CheckboxModal(JFrame parent) {
super(parent, "Select Options", true);
setLayout(new FlowLayout());
setSize(300, 200);
setLocationRelativeTo(parent);
// Initializing checkboxes
option1 = new JCheckBox("Option 1");
option2 = new JCheckBox("Option 2");
option3 = new JCheckBox("Option 3");
// Load previous state (if needed)
loadPreviousState();
JButton submitButton = new JButton("Submit");
submitButton.addActionListener(e -> {
// Save the state when submitted
saveCheckboxStates();
dispose(); // Close the modal
});
add(option1);
add(option2);
add(option3);
add(submitButton);
}
private void loadPreviousState() {
// Implementation to load previous checkbox states
// This could come from a database, configuration, etc.
}
private void saveCheckboxStates() {
boolean isChecked1 = option1.isSelected();
boolean isChecked2 = option2.isSelected();
boolean isChecked3 = option3.isSelected();
// Logic to handle the saved states
System.out.println("Checkbox 1: " + isChecked1);
System.out.println("Checkbox 2: " + isChecked2);
System.out.println("Checkbox 3: " + isChecked3);
}
}
Code Explanation
- The
CheckboxModal
class extendsJDialog
, making it a modal window. - We initialize three
JCheckBox
components. - The
loadPreviousState
method (which you can implement to retrieve the previously checked states) is called to ensure users see their last selections. - The
saveCheckboxStates
method captures the current state of each checkbox and prints it out. You can replace this with logic to persist data (e.g., saving to a database).
Simulating Checkbox State Persistence
For a real-world application, you might want to persist the checkbox states. This could be done using various methods like serialization or storing values in a database. Here's an example of how to use a simple properties file to save and load states.
State Persistence Code Snippet
To implement state persistence, consider the following methods:
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Properties;
private void loadPreviousState() {
Properties properties = new Properties();
try (FileInputStream input = new FileInputStream("checkbox_states.properties")) {
properties.load(input);
option1.setSelected(Boolean.parseBoolean(properties.getProperty("option1", "false")));
option2.setSelected(Boolean.parseBoolean(properties.getProperty("option2", "false")));
option3.setSelected(Boolean.parseBoolean(properties.getProperty("option3", "false")));
} catch (IOException e) {
e.printStackTrace();
}
}
private void saveCheckboxStates() {
Properties properties = new Properties();
properties.setProperty("option1", String.valueOf(option1.isSelected()));
properties.setProperty("option2", String.valueOf(option2.isSelected()));
properties.setProperty("option3", String.valueOf(option3.isSelected()));
try (FileOutputStream output = new FileOutputStream("checkbox_states.properties")) {
properties.store(output, "Checkbox States");
} catch (IOException e) {
e.printStackTrace();
}
}
Code Explanation
loadPreviousState
: Loads checkbox states from a properties file.saveCheckboxStates
: Saves the current checkbox states to a properties file.
To Wrap Things Up
Managing checkbox states in Java modal forms can feel daunting, but by following structured approaches, it can become straightforward. The dual approach of utilizing a modal dialog and storing checkbox states enhances user experience and reliability.
For an additional resource focusing on Displaying Checked Checkbox Elements in Modal, you can get deeper insights into managing modals with checkbox selections.
Adopting best practices will further simplify the handling of user inputs, ensuring that your application is intuitive and user-friendly. Happy coding!
Checkout our other articles