Common Pitfalls in Database Code Testing You Must Avoid
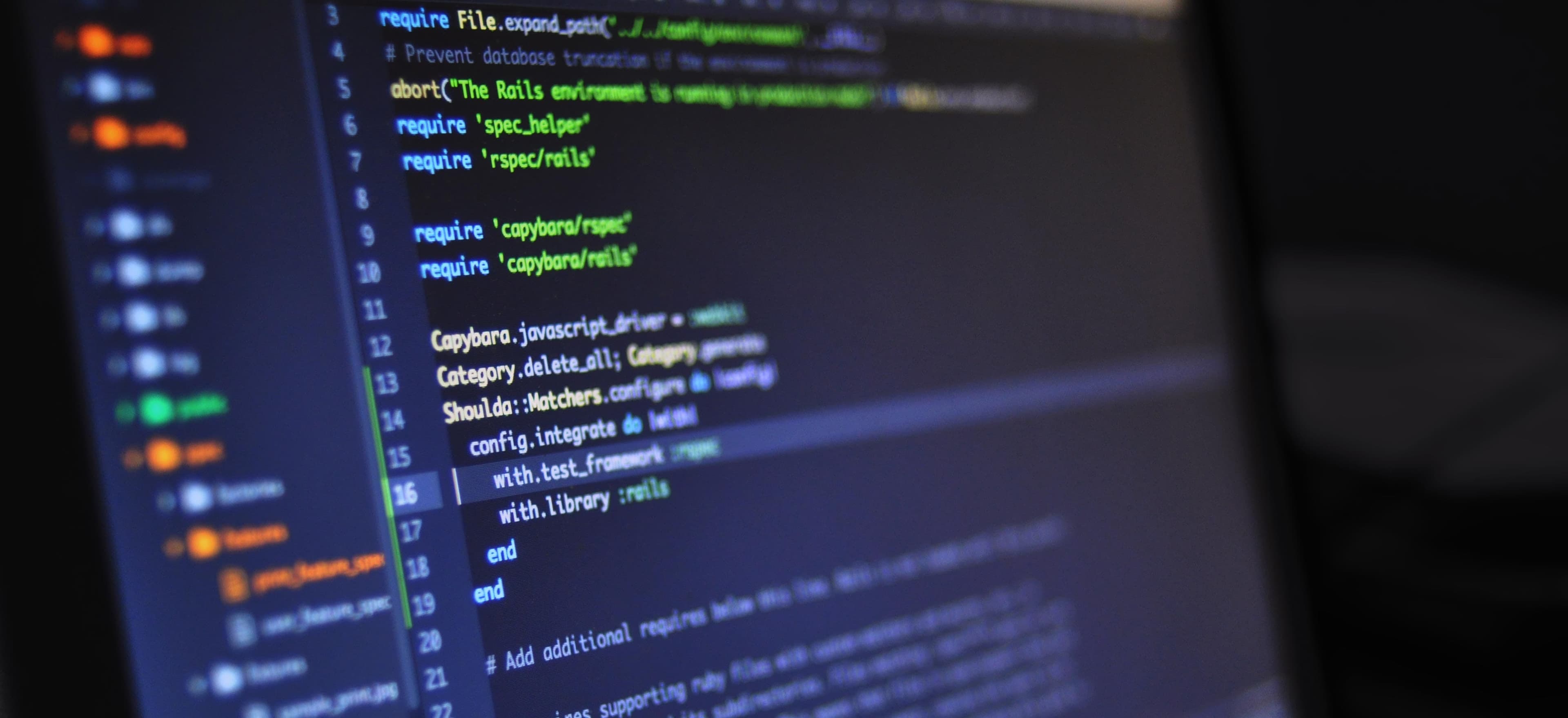
- Published on
Common Pitfalls in Database Code Testing You Must Avoid
Database code testing is a critical aspect of software development, especially in applications where data integrity and performance are paramount. However, this area is often fraught with pitfalls that can lead to significant issues down the line. In this post, we will discuss some common mistakes made during database code testing and provide best practices to help you avoid them.
1. Ignoring Test Data Management
One of the most common pitfalls in database code testing is neglecting test data management. Why does this matter? The quality of your tests depends on the data you use. If your test data is not representative of the production environment, your tests may pass without actually validating the functionality.
Best Practice: Use a Controlled Set of Test Data
- Create a controlled environment with relevant test data.
- Ensure that test data covers all edge cases.
For example, consider a user registration feature that relies on both valid and invalid email formats. Your test data must include various email formats to effectively validate your code.
@Test
public void testEmailValidation() {
String validEmail = "test@example.com";
String invalidEmail = "invalid-email.com";
assertTrue(EmailValidator.isValid(validEmail));
assertFalse(EmailValidator.isValid(invalidEmail));
}
2. Not Isolating Database Tests
Another often overlooked pitfall is the lack of isolation during database testing. If your tests are not independent, they can lead to flaky results, making it hard to pinpoint failures.
Best Practice: Use Transactions Wisely
Wrap each test in a transaction that can be rolled back after the test completes. This ensures that each test starts with a clean slate.
@Before
public void setUp() {
Connection connection = Database.getConnection();
connection.setAutoCommit(false);
this.connection = connection;
}
@After
public void tearDown() {
connection.rollback();
}
By doing this, you can ensure that no test leaves residual data that can affect subsequent tests. This practice promotes reliable testing and quick feedback loops.
3. Overlooking Performance Testing
Testing database code should not be limited to correctness. Performance testing is essential to ensure that queries run efficiently under load. Neglecting this aspect can lead to degraded application performance.
Best Practice: Incorporate Load Testing Early
Use tools such as Apache JMeter or Gatling to simulate user load on your database code.
Here’s a simple example of how you can set up a JMeter test plan to query for user data:
<ThreadGroup>
<num_threads>100</num_threads>
<ramp_time>5</ramp_time>
<loop_count>10</loop_count>
</ThreadGroup>
<HTTPSamplerProxy>
<domain>mysite.com</domain>
<port>80</port>
<path>/api/users</path>
<method>GET</method>
</HTTPSamplerProxy>
Incorporating load tests can help you identify performance bottlenecks before they affect user experience.
4. Skipping Integration Testing
Unit tests are essential, but they are not enough. Often, developers skip integration tests, assuming that unit tests will suffice. This approach can lead to situations where individual components work well but fail to interact correctly when integrated.
Best Practice: Always Include Integration Tests
Integration tests allow you to validate the behavior of multiple components working together. Here’s an example of an integration test for a service that interacts with the database:
@Test
public void testUserServiceIntegration() {
UserService userService = new UserService(new UserRepository());
User user = new User("test@example.com");
userService.registerUser(user);
assertNotNull(userService.getUserByEmail("test@example.com"));
}
This test checks whether the UserService can correctly register a user and then retrieve it. Always aim to set up integration tests alongside your unit tests.
5. Not Considering Database Migrations
Database schemas change. Ignoring database migrations in your tests can lead to stability issues. If your code is relying on a particular version of the database schema that fails to match production, the consequences can be severe.
Best Practice: Test Your Migrations
Always run migration scripts as part of your testing strategy. Make sure that your tests run against the newly migrated database schema:
@BeforeClass
public static void setUpDatabase() {
DatabaseMigration.runMigration();
}
By ensuring that all migrations are applied during testing, you can catch issues related to schema changes before they reach your production environment.
6. Failing to Use Mocking and Stubbing
It can be tempting to directly test against the database, but doing so may introduce inconsistencies and slow down your test execution. Mocking and stubbing can provide greater control over your tests.
Best Practice: Use Mocking Frameworks
Frameworks like Mockito can help create test doubles that simulate database interactions without hitting the actual database.
Here’s an example using Mockito to mock a UserRepository:
UserRepository mockRepo = Mockito.mock(UserRepository.class);
when(mockRepo.findByEmail("test@example.com")).thenReturn(new User("test@example.com"));
UserService userService = new UserService(mockRepo);
assertNotNull(userService.getUserByEmail("test@example.com"));
This practice helps you keep your tests fast and focused on the specific logic you're testing.
7. Not Automating Tests
Lastly, many teams still run tests manually, missing out on the benefits of automation. Manual testing can lead to inconsistencies and missed regressions.
Best Practice: Use Continuous Integration
Set up a Continuous Integration (CI) pipeline that automates the execution of database tests on every code change. Tools like Jenkins or GitHub Actions can help you integrate automated tests easily.
name: Run Tests
on: [push]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Run Tests
run: mvn test
Automated tests run on every commit, ensuring that new changes do not break existing functionality.
The Last Word
Database code testing is a vital component of software development, but it is fraught with pitfalls. By avoiding common mistakes—such as ignoring test data management, not isolating tests, and skipping performance testing—you can enhance your testing strategy. Emphasize automation, integration testing, and effective migrations to keep your database secure and your application performant.
For further reading on database testing, refer to Martin Fowler's article on Testing Database Applications, which covers additional testing techniques and principles.
By integrating these best practices into your workflow, you will build a robust database testing strategy that delivers dependable software ready for production. Happy coding!
Checkout our other articles