Common Pitfalls When Displaying Strings in Android ListView
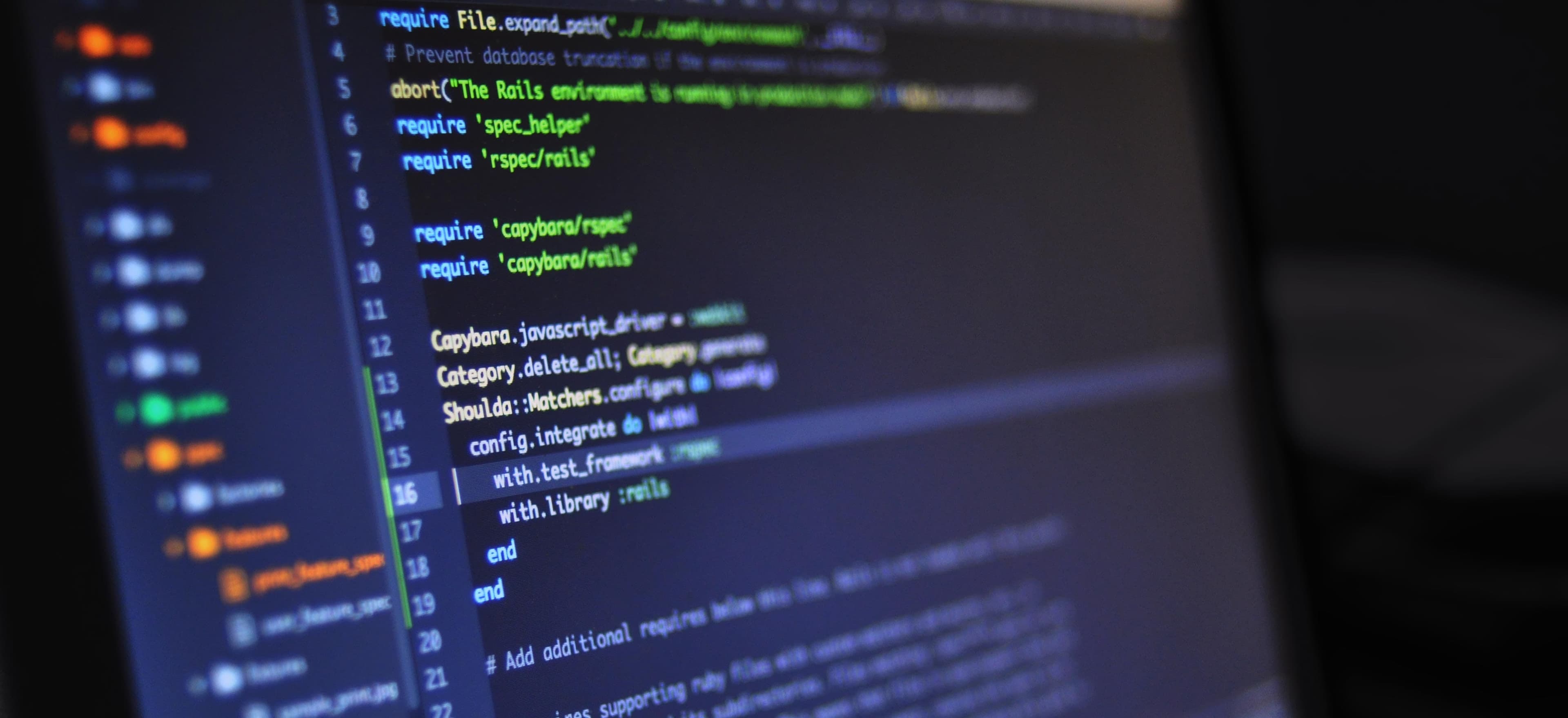
- Published on
Common Pitfalls When Displaying Strings in Android ListView
When developing Android applications, using a ListView for displaying strings is a common scenario. While it seems straightforward, several pitfalls can arise that may hinder performance, lead to crashes, or result in undesirable user experiences. In this blog post, we'll explore these common pitfalls and provide strategies for overcoming them.
Understanding ListView
Before we dive into the common pitfalls, it’s crucial to understand what ListView is. ListView is a widget that allows users to scroll through a collection of items in a list. It uses an adapter to bind data to the view.
The Importance of Efficient Data Binding
Needing a Replication of Functions
-
Using
ArrayAdapter
When a Custom Adapter is Required: WhileArrayAdapter
is great for simple implementations, it may not be sufficient for more complex layouts.Why it matters: Custom layouts in list items can lead to incorrect display and data binding issues. When using a simple adapter for complex data sets, users might end up seeing data not properly formatted.
Solution: Create a custom adapter to effectively manage your layout. Here is an example of how to implement a custom adapter.
Code Example: Custom Adapter
public class CustomListAdapter extends ArrayAdapter<String> {
private final Context context;
private final String[] values;
public CustomListAdapter(Context context, String[] values) {
super(context, R.layout.custom_list_item, values);
this.context = context;
this.values = values;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View rowView = inflater.inflate(R.layout.custom_list_item, parent, false);
TextView textView = rowView.findViewById(R.id.label);
textView.setText(values[position]);
return rowView;
}
}
Commentary:
- This custom adapter inflates a layout defined in
custom_list_item.xml
for each item, allowing for better control over how each string is displayed.
-
Inefficient View Recycling: One of the major pitfalls is failing to recycle views within the ListView. Every time a view is created, resource-intensive calls are made to the system.
Why it matters: This can lead to significant impact on performance and memory issues, especially in long lists.
Solution: Utilize
convertView
effectively, as demonstrated in the above code snippet. Only inflate new views whenconvertView
is null.
Code Example: View Recycling
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View rowView = convertView;
if (rowView == null) {
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
rowView = inflater.inflate(R.layout.custom_list_item, parent, false);
}
TextView textView = rowView.findViewById(R.id.label);
textView.setText(values[position]);
return rowView;
}
Commentary:
- With view recycling, when a ListView scrolls, it reuses existing views instead of creating new ones. This reduces the load on the system and maintains smooth user experience.
Common Mistakes When Managing Data
-
Modifying Data Directly: Appearing simple, directly modifying the underlying data set can create complications.
Why it matters: When the data set changes, the ListView does not automatically reflect these changes unless an appropriate notify method is called.
Solution: Always modify data through the adapter’s methods, such as adding or removing items from the adapter itself.
Code Example: Modifying Data in Adapter
public void addItem(String item) {
values.add(item);
notifyDataSetChanged(); // Notify ListView to refresh its data
}
Commentary:
- This ensures that whenever a new item is added, the ListView is notified to refresh its display, maintaining synchronization between data and UI.
-
Ignoring Item Clicks: Often, developers overlook implementing click listeners effectively.
Why it matters: This can lead to a lack of interactivity; if users can't click to view item details, it hampers the app's usability.
Solution: Implement click listeners within the adapter or in the activity to handle user interactions.
Code Example: Handling Clicks
@Override
public View getView(int position, View convertView, ViewGroup parent) {
// ... (conversion logic)
rowView.setOnClickListener(v -> {
// Handle click event here
Toast.makeText(context, "Clicked: " + values[position], Toast.LENGTH_SHORT).show();
});
return rowView;
}
Commentary:
- Assigning a click listener empowers the ListView items, allowing for greater user engagement and interaction.
Testing for Performance Issues
-
Long-running Background Tasks: Blocking the UI thread can cause lag in the ListView when performing operations like data loading.
Why it matters: Android highly prioritizes UI response. A laggy ListView equivalent can be a deal-breaker, leading to user frustration.
Solution: Use
AsyncTask
orThread
for long-running background tasks to keep the UI responsive.
Code Example: Using AsyncTask
new AsyncTask<Void, Void, List<String>>() {
@Override
protected List<String> doInBackground(Void... voids) {
// Simulate long running task
return fetchData(); // Fetch your data here
}
@Override
protected void onPostExecute(List<String> result) {
// Update the adapter with new data
adapter.addAll(result);
}
}.execute();
Commentary:
- AsyncTasks help you manage background operations without blocking UI updates, thereby enhancing user experience.
A Final Look
Navigating the world of ListViews in Android requires an awareness of potential pitfalls. From inefficient data binding to overlooking user interaction, these common traps can significantly impact performance and usability. By leveraging custom adapters, view recycling, and other best practices, you can create a responsive and attractive list interface in your Android application.
For more in-depth knowledge on efficient ListView management, check out Android's official documentation and the latest Kotlin features, which can enhance your development journey.
By keeping these points in mind, you can construct a fluid ListView experience that both you and your users will appreciate. Happy coding!
Checkout our other articles