Common Issues with Reusable MicroProfile Health Probes
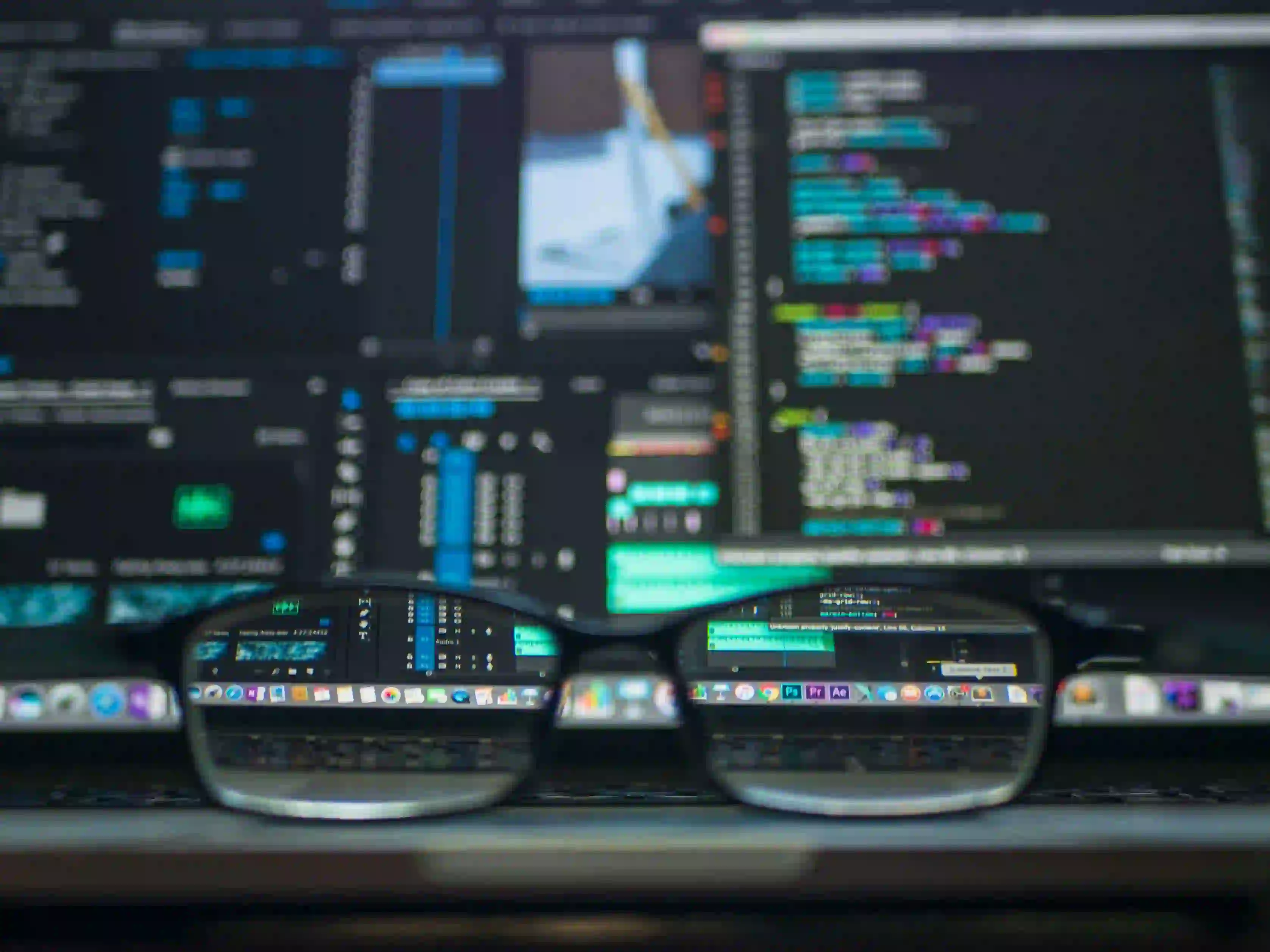
Common Issues with Reusable MicroProfile Health Probes
MicroProfile is an open and community-driven specification that optimizes Enterprise Java for microservices architecture. One of its key features is the Health Check API that allows you to create reusable health probes. These probes enhance your application's reliability by ensuring services are always up and running smoothly. However, like any technology, there can be challenges. In this blog post, we will explore some common issues with reusable MicroProfile health probes, providing solutions and best practices to circumvent these challenges.
Understanding MicroProfile Health Checks
Before diving into specific issues, let's briefly highlight what health checks are in the context of MicroProfile. The Health Check API provides a mechanism to define health probes in your microservices. This allows for monitoring by orchestrators and management tools. Implementing health checks is crucial for systems relying on continuous availability.
Key Components of MicroProfile Health Checks
- Health Check: Defines the health of a service.
- Health Check Response: A response object containing health status including status code and messages.
- Health Check Registry: Manages the lifecycle of registered health checks.
Common Issues with Reusable MicroProfile Health Probes
Here are some frequent challenges developers encounter when working with reusable MicroProfile health probes, along with practical solutions.
1. Configuration Management Issues
Problem:
Configuration values required for health checks often change across environments (development, staging, production). This can lead to incorrect health check responses.
Solution:
Utilize a configuration management strategy that externalizes these settings. For instance, you can use MicroProfile Config to externalize configurations that health checks might depend on.
import org.eclipse.microprofile.config.inject.ConfigProperty;
import org.eclipse.microprofile.health.HealthCheck;
import org.eclipse.microprofile.health.HealthCheckResponse;
import javax.enterprise.context.ApplicationScoped;
@ApplicationScoped
public class DatabaseHealthCheck implements HealthCheck {
@ConfigProperty(name = "db.url")
String dbUrl;
@Override
public HealthCheckResponse call() {
boolean isDatabaseUp = checkDatabase(dbUrl);
return HealthCheckResponse.named("Database Health Check")
.status(isDatabaseUp)
.build();
}
private boolean checkDatabase(String url) {
// Logic to check database connection
return true; // Simplified for demonstration
}
}
This code leverages MicroProfile Config to dynamically pull configuration values, ensuring that your application remains flexible and responsive to its environment.
2. Dependent Services Not Available
Problem:
When a health probe checks other services (e.g., database, third-party API), you might find that health checks start failing if any of these services are down.
Solution:
Implement a robust failure handling mechanism. For instance, classify your health checks into critical and non-critical checks.
@Override
public HealthCheckResponse call() {
if (!isServiceAvailable()) {
return HealthCheckResponse.named("Critical Service Health Check")
.down().withData("service", "External API is down").build();
}
return HealthCheckResponse.named("Critical Service Health Check").up().build();
}
By smartly categorizing service dependencies, you can avoid cascading failures in your application's overall health check status.
3. Inadequate Timeout Settings
Problem:
Health checks that take too long can lead to timeout errors. An overly long check can affect uptime, particularly in environments with slower network connections.
Solution:
Set proper timeout values for your health probes. When implementing an external API health check, for instance, this might look like:
public boolean checkExternalService() {
HttpClient client = HttpClient.newBuilder()
.connectTimeout(Duration.ofSeconds(2)) // Set a timeout of 2 seconds
.build();
// Logic to call external service
}
The above configuration safeguards your application from waiting too long and therefore helps maintain reliable health checks.
4. Caching Health Check Results
Problem:
If a health check is computationally expensive, repeated requests may lead to performance overhead.
Solution:
Cache the health check results for a specific duration. Utilize in-memory caching through frameworks like Caffeine or simple in-memory structures.
private HealthCheckResponse cacheResult;
@Override
public HealthCheckResponse call() {
if (cacheResult != null && !isExpired()) {
return cacheResult;
}
boolean serviceHealthy = validateServiceHealth();
cacheResult = HealthCheckResponse.named("Cached Health Check").status(serviceHealthy).build();
return cacheResult;
}
private boolean isExpired() {
// Implement expiration logic
return false; // Simplified for demonstration
}
This method speeds up health checks and reduces unnecessary load on your services by only recalculating the status when needed.
5. Lack of Contextual Information
Problem:
Sometimes, health checks might return a simple "up" or "down" status without additional information. This can make troubleshooting very difficult.
Solution:
Enhance your health check responses to include contextual data relevant to the checks.
@Override
public HealthCheckResponse call() {
boolean isServiceHealthy = checkSomeService();
HealthCheckResponse response = HealthCheckResponse.named("Enhanced Health Check")
.status(isServiceHealthy)
.withData("message", isServiceHealthy ? "Service is operational" : "Service is down")
.withData("timestamp", System.currentTimeMillis())
.build();
return response;
}
This richer response provides clarity about the current state of your service, thus aiding in incident resolution.
Wrapping Up
MicroProfile's Health Check API is a tremendously valuable tool for ensuring the reliability of microservices architectures. However, as the outlined common issues indicate, implementing health probes requires careful consideration and robust handling of potential pitfalls. By applying the best practices discussed, you can increase the reliability and visibility of your microservices, ultimately leading to improved performance and user experience.
For more detailed information on MicroProfile, refer to the MicroProfile Health Documentation to further enhance your implementation.
If you have faced other challenges not mentioned in this discussion, feel free to share them in the comments. Happy coding!