Mastering NoSQL with Hibernate OGM: Common Pitfalls to Avoid
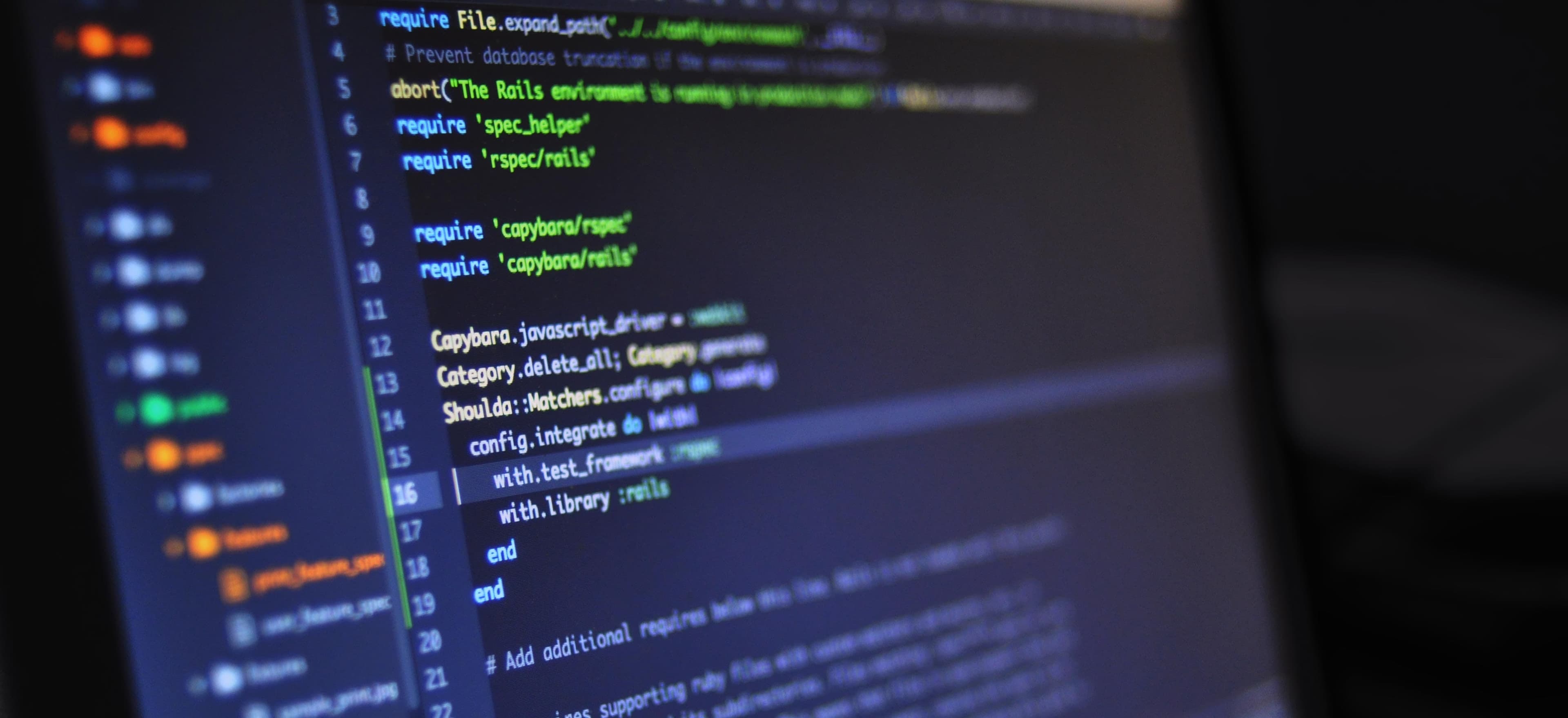
- Published on
Mastering NoSQL with Hibernate OGM: Common Pitfalls to Avoid
As developers strive for efficiency and speed in their applications, NoSQL databases have surged in popularity. Their ability to handle unstructured data makes them an appealing choice, especially for high-velocity data workloads. Hibernate OGM (Object/Grid Mapper) fills the gap by providing a familiar interface for Java developers transitioning from traditional relational databases to NoSQL solutions. However, it’s not without its pitfalls. In this blog post, we will explore some common pitfalls to avoid when using Hibernate OGM with NoSQL databases.
Understanding Hibernate OGM
Before diving into pitfalls, let’s clarify what Hibernate OGM is. It is a library within the Hibernate ORM ecosystem that allows developers to work seamlessly with various NoSQL databases like MongoDB, CouchDB, and Cassandra. This framework leverages the full power of object-oriented paradigms in Java while abstracting the underlying NoSQL complexities.
Common Pitfalls
1. Ignoring the Schema-less Nature of NoSQL
NoSQL databases such as MongoDB allow developers to store data without a fixed schema. This flexibility can be liberating, but it can also lead to chaotic data structures. If you attempt to apply strict relational design principles to a NoSQL database, you may end up with confusing and difficult-to-maintain data structures.
Avoid this pitfall by:
- Establishing Clear Data Models: Even though NoSQL is schema-less, define a clear data model for consistency across your application.
- Consolidating Collections: Group similar data into logical collections to maintain organization.
Example:
@Entity
public class User {
@Id
private String id;
private String name;
@Field
private List<String> hobbies; // NoSQL allows flexible arrays
}
In this example, the hobbies
field allows for flexibility. However, it is best to have a predefined approach, ensuring users have a reasonable set of hobbies listed rather than an ambiguous array.
2. Mismanaging Transactions
NoSQL databases often lack robust transaction support. While some NoSQL solutions provide eventual consistency, using them requires a deeper understanding of how transactions affect your data integrity.
Avoid this pitfall by:
- Recognizing When to Use Transactions: Use transactions only when the data integrity of your operation must be guaranteed.
- Focusing on Eventual Consistency: Be okay with the fact that not every operation may be immediately consistent.
Example:
public void updateUserHobbies(String userId, List<String> newHobbies) {
User user = session.find(User.class, userId);
user.setHobbies(newHobbies);
session.put(user); // In NoSQL use, this may not guarantee all-or-nothing.
}
Here, while updating hobbies, it may be more effective to treat the write operation carefully, ensuring that users are aware of the potential for these operations being seen only later.
3. Over-using Object-Relational Mapping
Hibernate OGM brings the power of object-relational mapping to NoSQL, but this does not mean you should apply all ORM features indiscriminately. Suppose you attempt to map every object directly from a class. In that case, it could lead to performance issues, especially with large data sets.
Avoid this pitfall by:
- Using Projections: Only retrieve the data you need instead of fetching entire objects.
Example:
Query<User> query = session.createQuery("SELECT u.name, u.hobbies FROM User u", User.class);
List<User> users = query.getResultList(); // Avoids loading the entire user object
Here, by fetching only the necessary fields, we enhance performance — particularly crucial when handling large datasets.
4. Neglecting Indexing
While NoSQL databases can handle large amounts of unstructured data, they require indexing to maintain performance and quick searchability. Ignoring proper indexing is a sure way to undermine your application’s performance.
Avoid this pitfall by:
- Creating Indexes Appropriately: Use indexing for frequently queried fields.
Example:
@Indexed
@Entity
public class Product {
@Id
private String id;
@Field
private String name;
@Field
private double price;
// Indexing on the price field for quick lookups
}
By indexing the price
field, we dramatically improve the efficiency of searches related to product prices — crucial for e-commerce applications.
5. Forgetting Community and Documentation Resources
The NoSQL landscape is vast and evolving. New improvements and best practices frequently emerge, and the Hibernate OGM documentation, along with community resources, provide invaluable insights.
Avoid this pitfall by:
- Staying Updated: Regularly check for updates on Hibernate OGM and NoSQL practices.
- Engaging with Community: Participate in forums and online discussions about challenges and solutions.
6. Overlooking Relationship Mapping
In NoSQL databases, relationships work differently than they do in SQL databases. While traditional SQL databases facilitate relationships through foreign keys, NoSQL databases might require denormalization or references that need careful handling.
Avoid this pitfall by:
- Choosing the Right Relationship Model: Decide whether embedded documents or references suit your needs better.
Example:
@Entity
public class Order {
@Id
private String id;
@Reference
private List<Product> products; // Reference to product entities rather than embedding
}
This example uses references to Product
, allowing for relationships but necessitating careful handling of related database records.
Final Considerations
Hibernate OGM provides Java developers with a powerful tool for leveraging NoSQL databases. However, avoiding common pitfalls requires diligence, awareness, and a thoughtful approach to data modeling.
Remember that while NoSQL affords tremendous flexibility, it also demands careful planning to use effectively. Take the time to establish clear schemas, manage transactions carefully, and make judicious choices regarding your data relationships.
For further reading on Hibernate OGM, discover the official documentation here or join communities on platforms like Stack Overflow to engage with other developers facing similar challenges.
Embrace NoSQL with confidence, and avoid the traps that can lead to performance bottlenecks and data chaos. Happy coding!
Checkout our other articles