Why the Law of Demeter Could Save Your Java Projects
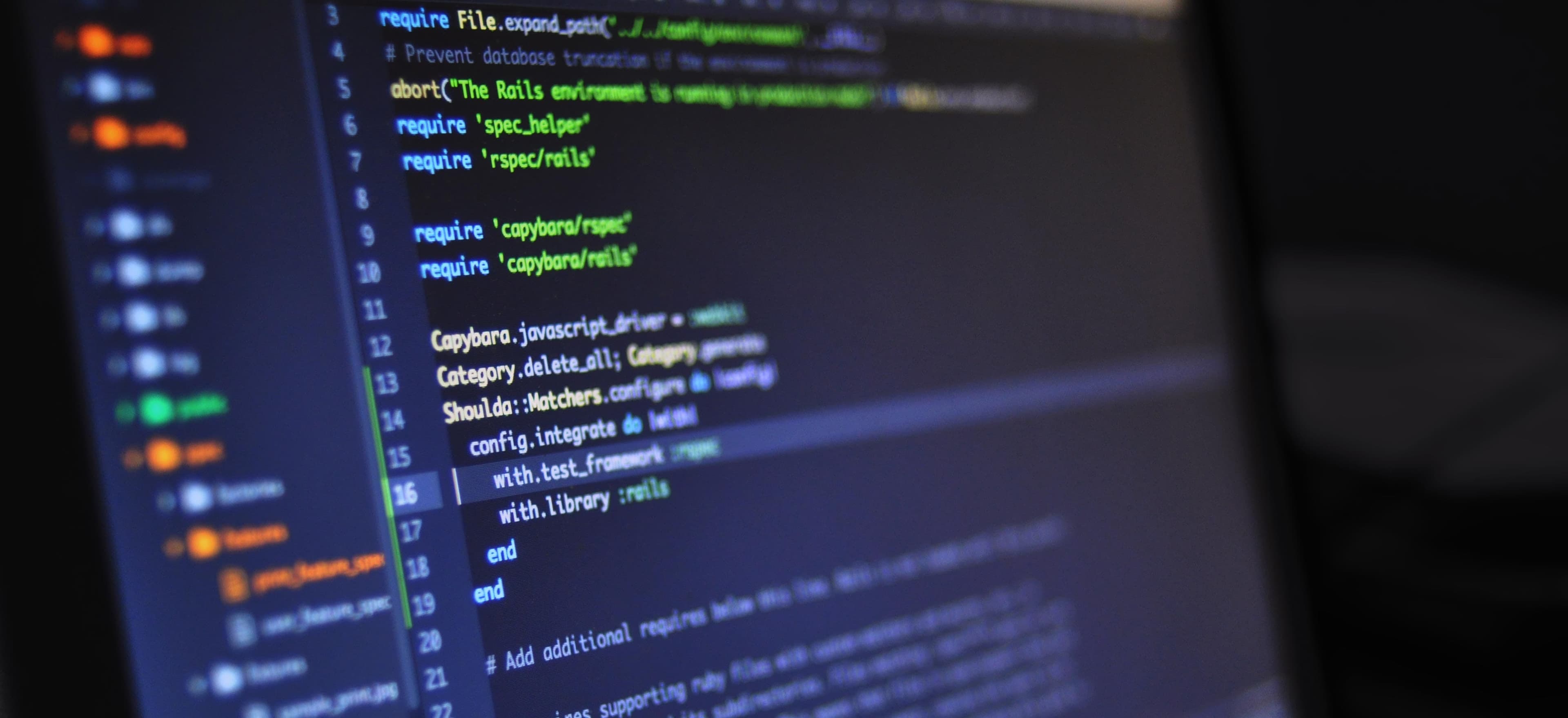
- Published on
Why the Law of Demeter Could Save Your Java Projects
Software engineering is filled with principles that aim to create more maintainable and robust code. One such principle is the Law of Demeter (LoD), often summarized as "only talk to your immediate friends." While this concept may sound simple, its application can drastically improve the design and resilience of your Java projects. In this post, we'll explore the Law of Demeter, its implications for object-oriented programming, and provide you with practical examples in Java that highlight its benefits.
Table of Contents
- What is the Law of Demeter?
- Why Use the Law of Demeter?
- The Law of Demeter in Java: Examples
- Benefits of Applying the Law of Demeter
- Conclusion
What is the Law of Demeter?
The Law of Demeter was formulated at Northeastern University in 1987, particularly for object-oriented programming. It is a design guideline that promotes the idea of loose coupling between components in a system.
Specifically, the principle states that:
- A method of an object should only call methods of:
- The object itself.
- Objects created within the method.
- Parameters passed to the method.
- Directly held components.
In a more straightforward way, you should not reach through an object to grab another object’s methods. This fosters a minimal reliance on the internal workings of other objects.
Why Use the Law of Demeter?
Using the Law of Demeter offers several advantages, including:
- Increased Maintainability: When your classes are loosely coupled, modifying one class does not heavily impact others.
- Improved Readability: Code becomes easier to understand when objects only communicate within a limited scope.
- Isolated Testing: Looser coupling allows for simpler unit testing as you can mock or stub dependencies easily.
The Law of Demeter in Java: Examples
Let’s delve into concrete examples to demonstrate the Law of Demeter in Java.
Example 1: Code That Violates the Law of Demeter
The following code snippet demonstrates a common violation:
class Driver {
private Car car;
public Driver(Car car) {
this.car = car;
}
public int getCarEngineTemperature() {
return car.getEngine().getTemperature();
}
}
class Car {
private Engine engine;
public Car(Engine engine) {
this.engine = engine;
}
public Engine getEngine() {
return engine;
}
}
class Engine {
private int temperature;
public int getTemperature() {
return temperature;
}
}
In this example, the Driver
class directly accesses the Engine
class's methods through the Car
class. This is a violation of the Law of Demeter because Driver
is reaching through Car
to fetch Engine
's state. Such design can lead to tight coupling and make the codebase more fragile.
Example 2: Refactored Code Complying with the Law of Demeter
Let’s refactor the earlier code to adhere to the Law of Demeter.
class Driver {
private Car car;
public Driver(Car car) {
this.car = car;
}
public int getCarEngineTemperature() {
return car.getEngineTemperature();
}
}
class Car {
private Engine engine;
public Car(Engine engine) {
this.engine = engine;
}
public int getEngineTemperature() {
return engine.getTemperature();
}
}
class Engine {
private int temperature;
public int getTemperature() {
return temperature;
}
}
In the refactored version, the Car
class exposes a method getEngineTemperature
which encapsulates the Engine
’s internal details. This enables the Driver
class to communicate with the Car
directly, significantly reducing its dependency on Engine
.
Commentary on the Code
Notice how we didn’t just fix the method call issue; we also conveyed contract-like interactions between classes. This encapsulation allows you to change the inner workings of the Car
or Engine
class without needing to modify the Driver
class. This is the essence of maintainability; less interdependence means fewer downstream issues when making changes.
Benefits of Applying the Law of Demeter
By adhering to the Law of Demeter, you pave the way for various benefits in your Java projects:
- Enhanced Code Readability: By limiting the visibility of the inner workings of classes, each class speaks only to its immediate dependencies. This simplifies understanding and reasoning about the code, which is vital in collaborative environments.
- Reduced Complexity: Keeping dependencies close and interactions limited minimizes inter-object complexities. This leads to a cleaner architecture that is easier for new developers to grasp.
- Easier Refactoring: As your application grows, refactoring becomes necessary. Loosely coupled classes make this task easier, enabling developers to swap out or update components without fearing widespread repercussions.
Exploring further into the documentation on the Law of Demeter could provide additional insights. You can check the Design Patterns and Patterns of Enterprise Application Architecture for more design guidelines.
A Final Look
The Law of Demeter is more than just an idea; it's a powerful guideline that can significantly enhance the quality of your Java applications. By fostering low coupling and high coherence, you not only improve the maintainability and readability of your code but also make your projects more adaptable to change.
As you embark on your development journey, consider the immediate interactions more than the intricate webs. Remember, if you follow the Law of Demeter, your code will relish in the joys of simplicity and clarity while standing resilient amid the evolving landscape of requirements.
For further reading, you might find Java's Object-Oriented Programming Principles insightful as it integrates well with the principles we discussed here.
By embracing the Law of Demeter, you're ensuring that your Java projects not only meet today's requirements but are also ready for the challenges of tomorrow. Happy coding!
Checkout our other articles