Unlocking the Power: 5 JIT Optimization Pitfalls to Avoid
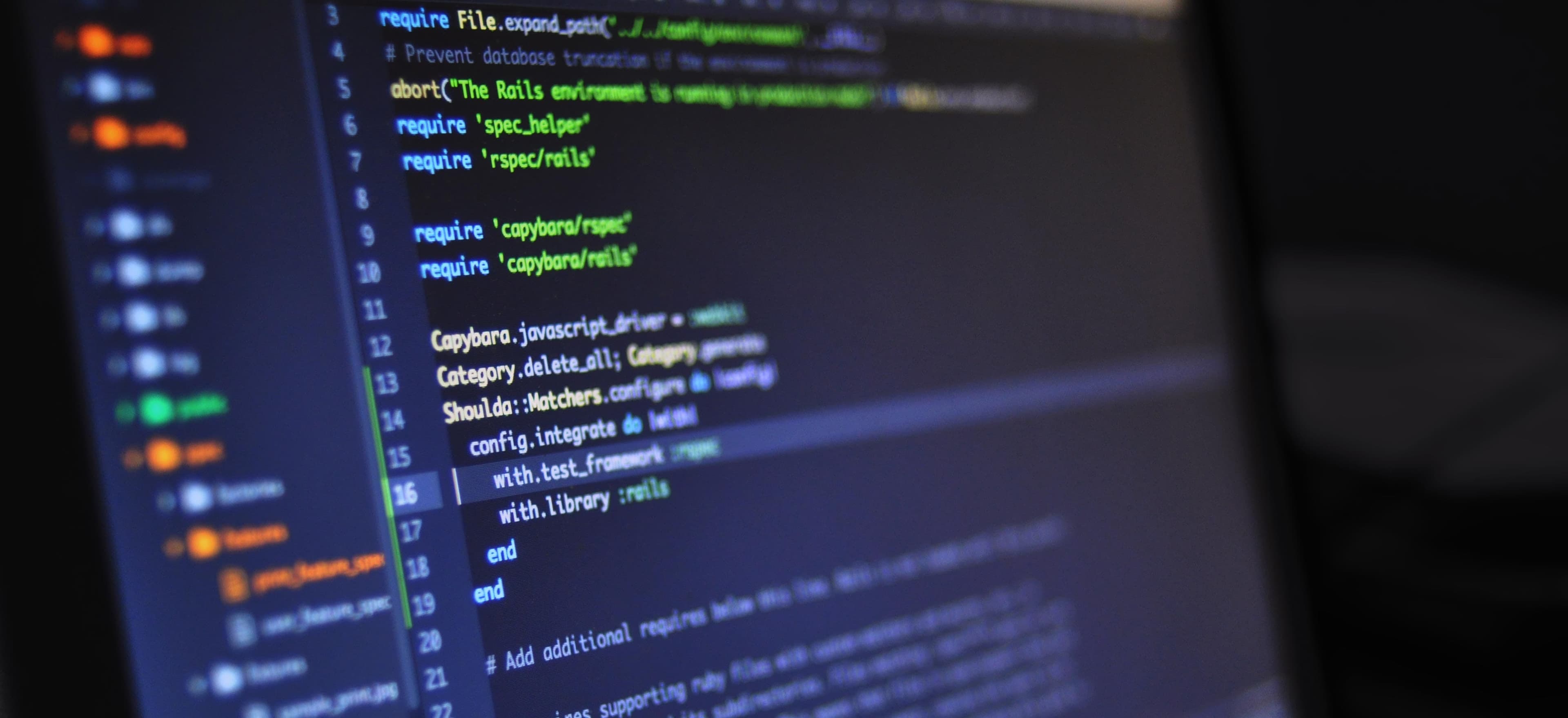
- Published on
Unlocking the Power: 5 JIT Optimization Pitfalls to Avoid
Just-In-Time (JIT) compilation has revolutionized the world of Java performance optimization. By converting bytecode to machine code at runtime, JIT enhances execution speed and offers dynamic adaptation based on real-time profiling. However, while JIT can lead to exceptional application performance, there are common pitfalls that developers may encounter. In this post, we will explore five JIT optimization pitfalls to avoid to ensure that your Java applications remain efficient and responsive.
Table of Contents
- Understanding JIT Compilation
- Pitfall #1: Over-Optimizing Code
- Pitfall #2: Ignoring Profile Data
- Pitfall #3: Misusing HotSpot Compiler Flags
- Pitfall #4: Not Utilizing Escape Analysis
- Pitfall #5: Failing to Test Performance Changes
- Conclusion
1. Understanding JIT Compilation
Before diving into the pitfalls, it's essential to grasp the basics of JIT compilation. JIT compilers are responsible for improving the performance of Java applications by compiling bytecode into native machine code at runtime. This process allows the JVM (Java Virtual Machine) to make informed optimizations based on the actual execution patterns and behaviors of the application.
Why JIT Matters
Java's JIT compilation is critical for performance. It bridges the gap between Java’s platform independence and the need for high-efficiency execution. For instance, consider the difference between interpreted code and compiled code. Compiled code runs faster because it is optimized for the specific architecture it is executed on. The JIT compiler effectively allows Java to combine the advantages of both worlds: portability and performance.
2. Pitfall #1: Over-Optimizing Code
One of the leading pitfalls in JIT optimization is the tendency to over-optimize code prematurely. Developers may attempt to micro-optimize every tiny detail, leading to degraded performance due to excessive complexity.
Example
public int computeSum(int limit) {
int sum = 0;
for (int i = 0; i < limit; i++) {
sum += i;
}
return sum;
}
Commentary
In the above example, there is no unnecessary complexity. The method computes the sum in a straightforward manner, making it easy for the JIT compiler to optimize at runtime. If you were to rewrite this method with excessive optimization strategies—such as breaking it down into several methods or using bit shifting for simple arithmetic—you might confuse the JIT compiler or inhibit its ability to optimize the code effectively.
Takeaway: Keep your methods clear and concentrate on algorithmic efficiency, not micro-optimizations.
3. Pitfall #2: Ignoring Profile Data
The JIT compiler relies heavily on profile data collected during execution. Ignoring this information can lead you to make suboptimal decisions during the development phase.
Example
Consider a scenario where a method shows signs of being frequently executed during testing:
public void frequentMethod() {
// Your logic here
}
If you notice that this method is running frequently, but fail to observe its impact on overall performance, you may miss opportunities to optimize critical parts of your application.
Commentary
By profiling your application with tools like Java Mission Control or VisualVM, you can gather essential data to identify hotspots—methods or functions that consume the most processing time. This will guide your optimization efforts where they are needed most.
Takeaway: Always collect and analyze profile data—this will ensure that your optimization efforts align with real performance bottlenecks.
4. Pitfall #3: Misusing HotSpot Compiler Flags
The JVM offers various HotSpot Compiler flags that allow you to customize the compilation process. However, each flag carries potential risks and implications.
Example
Let’s say you decide to use the -XX:CompileThreshold
flag to force the JIT compiler to compile methods after they've been executed a specific number of times. Setting this threshold too low may lead to the compilation of methods that are not performance-critical.
Commentary
Using compiler flags haphazardly can lead to diminishing returns on performance. Evaluate the needs of your application carefully before making changes. Consult the Official Oracle Documentation to understand the implications of each compiler flag.
Takeaway: Use compiler flags judiciously and test their impact thoroughly.
5. Pitfall #4: Not Utilizing Escape Analysis
Escape analysis is a sophisticated optimization technique that can significantly impact the performance of Java applications. By allowing the compiler to determine if an object can be allocated on the stack instead of the heap, escape analysis can reduce memory pressure and increase execution speed.
Example
Consider the following method that unnecessarily uses the heap:
public MyObject createObject() {
return new MyObject();
}
Commentary
If the JIT compiler determines that the MyObject
instance does not escape the method context (i.e., it is not returned or saved elsewhere), it can allocate this object on the stack, leading to faster access times and reduced garbage collection overhead.
Takeaway: Always write methods such that escape analysis can be applied effectively. Utilize final variables where possible to enhance the compiler’s ability to analyze object lifetimes.
6. Pitfall #5: Failing to Test Performance Changes
After making optimizations, developers often overlook the importance of testing performance changes. What may seem like an improvement could inadvertently introduce regressions.
Example
Say you refactored some frequently used code to improve readability:
public int calculateTotal(int[] values) {
return Arrays.stream(values).sum();
}
Commentary
Although using streams can enhance code clarity, it might not provide the same performance benefits as the traditional for-each loop concept due to overhead associated with stream creation.
Takeaway: Always perform comprehensive performance testing after changes. Use benchmarks to compare performance pre- and post-optimization.
Final Considerations
JIT compilation is a powerful asset for Java developers, offering substantial performance benefits when utilized correctly. However, it's crucial to remain mindful of the common pitfalls discussed above: avoid over-optimizing code, leverage profile data, use HotSpot flags wisely, utilize escape analysis properly, and always test performance impacts after changes. By doing so, you will not only improve your Java applications but also ensure that they are efficient and scalable.
For further reading, check out Understanding the Java Virtual Machine for an in-depth look at how Java achieves optimization through JIT compilation. Happy coding!