Overcoming File Mocking Challenges in JUnit for Spring Boot
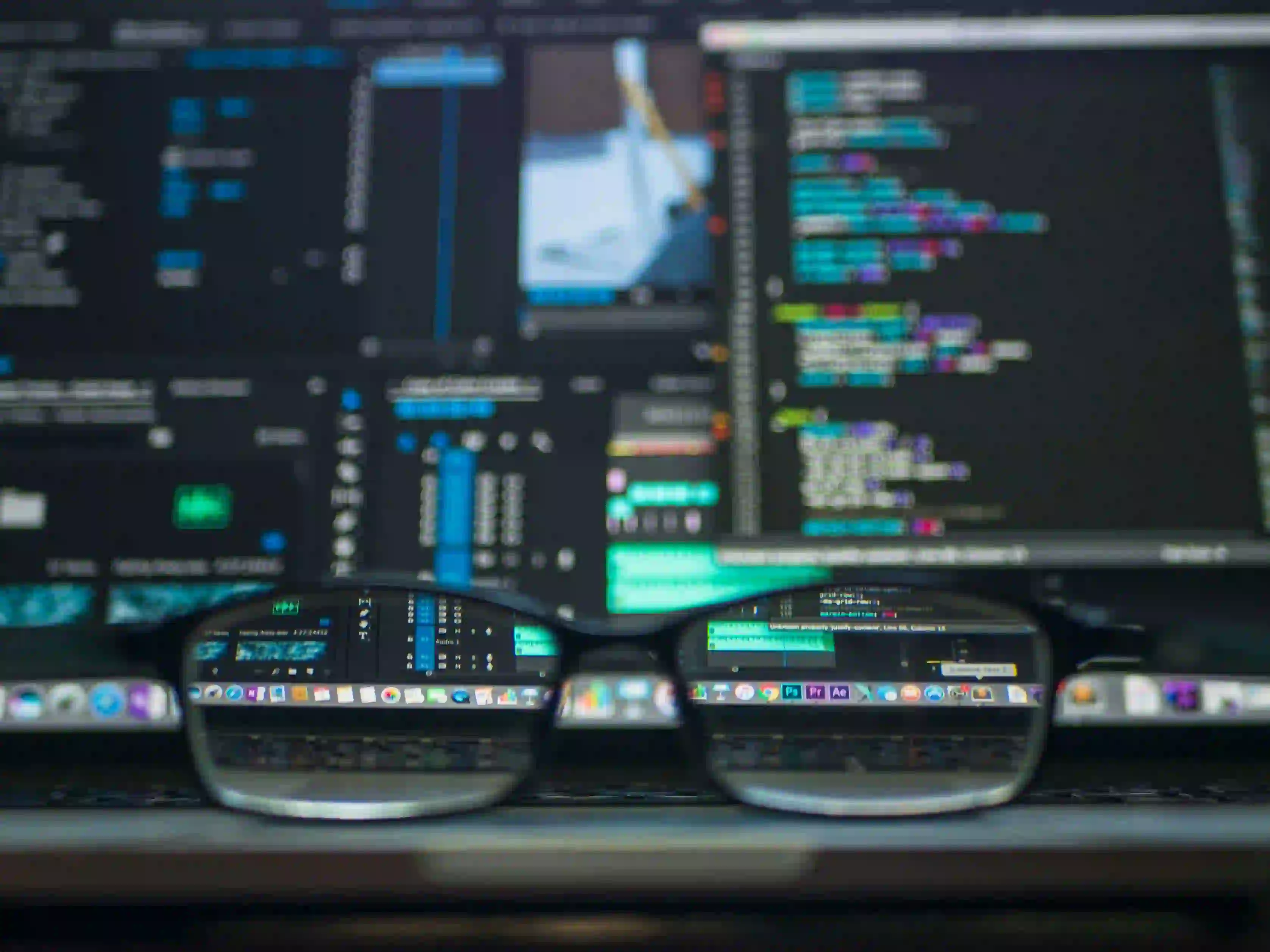
Overcoming File Mocking Challenges in JUnit for Spring Boot
When developing applications with Spring Boot, you may encounter situations where you need to test services that interact with files. These interactions can introduce complexity, especially when you want to ensure your tests remain robust and isolated. This blog post will guide you through overcoming file mocking challenges in JUnit for Spring Boot applications.
Understanding File Mocking
File mocking involves simulating file input and output operations in your tests. The goal is to avoid dependencies on the file system while ensuring that your unit tests remain fast and reliable. Mocking file interactions helps you achieve the following:
- Isolation: Tests are not affected by the actual file system or its contents.
- Speed: Tests run faster because they do not rely on I/O operations.
- Consistency: You can control the behavior of file interactions to test various scenarios.
When to Mock Files in Your Spring Boot Tests
Here are a few scenarios when you'll likely need to mock files in your JUnit tests:
- When reading configuration files.
- When processing uploaded files.
- When interacting with CSV, JSON, or XML files for data import or export.
Setting Up a Spring Boot Project
Before we dive into mocking file interactions, let’s set up a basic Spring Boot application. Create a new Spring Boot project and add the necessary dependencies in your pom.xml
file:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
Example Service
Let's create a simple service that reads from a file. This service will demonstrate how to handle file mocking in tests.
import org.springframework.stereotype.Service;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
@Service
public class FileService {
public String readFile(String filePath) throws IOException {
StringBuilder content = new StringBuilder();
try (BufferedReader reader = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = reader.readLine()) != null) {
content.append(line).append("\n");
}
}
return content.toString().trim();
}
}
Why This Code?
In this code snippet, the readFile
method opens a file, reads its content line-by-line, and returns it as a single String
. It uses Java's BufferedReader
and FileReader
classes for efficient file reading. This approach, however, introduces external dependencies that complicate testing.
Writing the Unit Test with Mocking
To effectively unit test the FileService
, we must mock the file interactions. We can use Mockito, a popular mocking framework, for this purpose. First, include Mockito in your dependencies:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<scope>test</scope>
</dependency>
Test Class Implementation
Here’s an example of how to test the FileService
using JUnit and Mockito:
import static org.mockito.Mockito.*;
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.StringReader;
class FileServiceTest {
@InjectMocks
FileService fileService;
@Mock
BufferedReader bufferedReader;
public FileServiceTest() {
MockitoAnnotations.openMocks(this);
}
@Test
void testReadFile() throws IOException {
when(bufferedReader.readLine()).thenReturn("line 1", "line 2", null);
String filePath = "dummy/file/path.txt"; // This path is irrelevant due to mocking
// Using a spy or working on BufferedReader will differ in terms of flexibility.
try (BufferedReader spyReader = new BufferedReader(new StringReader("line 1\nline 2"))) {
String result = fileService.readFile(filePath);
assertEquals("line 1\nline 2", result);
}
verify(bufferedReader, times(2)).readLine();
}
}
Why This Test?
-
Mocking BufferedReader: This approach mocks the behavior of the
BufferedReader
class. This way, we can simulate file content without accessing the file system. -
Use of
Mockito.when
: We specify the return values of calls to thereadLine
method. This abstraction allows us to test various scenarios, such as reading different lines or an empty file. -
Assertions and Verification: We check that the result matches the expected content and verify that
readLine
is called the expected number of times.
Alternative Approach: Using a File System Abstraction
While mocking works well, another approach is using a file system abstraction library, such as Jimfs, which allows you to create an in-memory file system for testing. Here’s an example of how to incorporate Jimfs:
Adding Jimfs Dependency
Make sure to add the following dependency in your pom.xml
:
<dependency>
<groupId>com.google.jimfs</groupId>
<artifactId>jimfs</artifactId>
<scope>test</scope>
</dependency>
Example with Jimfs
Here’s how you can rewrite the test using Jimfs:
import com.google.common.jimfs.Configuration;
import com.google.common.jimfs.Jimfs;
import org.junit.jupiter.api.Test;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import static org.junit.jupiter.api.Assertions.assertEquals;
class FileServiceJimfsTest {
@Test
void testReadFileWithJimfs() throws IOException {
Path path = Jimfs.newFileSystem(Configuration.unix()).getPath("/dummy/file/path.txt");
Files.write(path, "line 1\nline 2".getBytes());
FileService fileService = new FileService();
String result = fileService.readFile(path.toString());
assertEquals("line 1\nline 2", result);
}
}
Why Use Jimfs?
Using Jimfs allows you to create an in-memory file system for your tests. This method provides a more realistic environment for file reading operations without touching the actual filesystem. You get all the benefits of isolation, speed, and consistency while maintaining the behavior of real file I/O.
Key Takeaways
File mocking in JUnit tests is crucial when working with Spring Boot applications. By utilizing frameworks like Mockito or libraries like Jimfs, you can efficiently isolate tests and handle file interactions robustly. The approach you choose depends on your specific needs.
For more information on testing with JUnit and Mockito, consider the following resources:
By mastering these techniques, you can enhance the quality of your tests and ensure your Spring Boot applications are resilient and maintainable. Happy coding!