JOOQ vs JDBC: Navigating the Complexity of SQL Queries
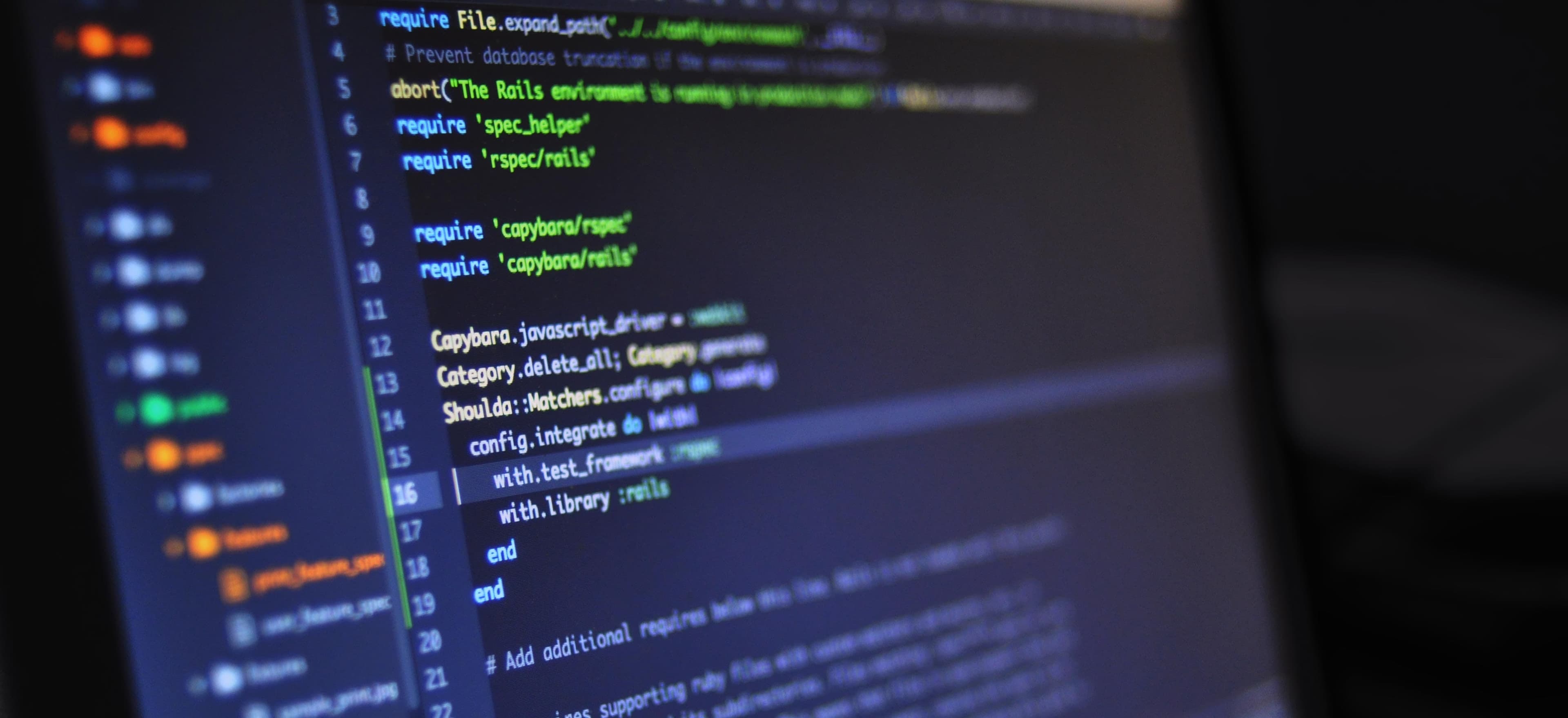
- Published on
JOOQ vs JDBC: Navigating the Complexity of SQL Queries
When it comes to interacting with databases in Java, developers often face the choice between JDBC (Java Database Connectivity) and modern frameworks like JOOQ (Java Object Oriented Querying). Both have their strengths and weaknesses, and the best choice can often depend on the specific requirements of your project. In this post, we will navigate this complexity, dissecting both methods of database interaction, examining their key features, and providing code samples for clarity.
Understanding JDBC
JDBC is a low-level API that allows Java applications to connect to various databases. Given its foundational nature, it provides the essential building blocks for database operations.
Key Features of JDBC:
- Low-Level Access: JDBC provides direct calls to execute SQL queries. This means you have complete control over your SQL, but it also means you must manage the complexity.
- Flexibility: You can use it with any database that supports JDBC, as long as you have the appropriate JDBC driver.
- Performance: With direct access to SQL, performance can be optimized for specific queries.
Example JDBC Usage
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class JdbcExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
try (Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement()) {
String sql = "SELECT * FROM users WHERE active = 1";
ResultSet resultSet = statement.executeQuery(sql);
while (resultSet.next()) {
System.out.println("User: " + resultSet.getString("name"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example:
- We establish a database connection using
DriverManager
. - We execute a simple SQL query to fetch active users.
- The result set is processed in a while loop, showcasing how to extract values.
While JDBC gives you granular control, it entails more boilerplate code. You must handle connections, exceptions, and result sets manually.
Enter JOOQ
JOOQ is a more modern approach that enhances how developers interact with SQL databases. It introduces a fluent API and allows for type-safe SQL query generation.
Key Features of JOOQ:
- Type Safety: JOOQ generates classes based on your database schema, ensuring compile-time checking of SQL queries.
- Fluent API: The syntax allows for readable and expressive code, making it easier to construct complex queries programmatically.
- SQL Integration: JOOQ translates Java code to SQL, meaning you can use SQL-like constructs directly in Java.
Example JOOQ Usage
Before using JOOQ, make sure to include the necessary dependency in your pom.xml
if you are using Maven:
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq</artifactId>
<version>3.15.0</version>
</dependency>
Now, let's see how we can rewrite our previous example using JOOQ:
import static org.jooq.impl.DSL.*;
import org.jooq.*;
import org.jooq.impl.DSLContext;
import java.sql.Connection;
import java.sql.DriverManager;
public class JooqExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
try (Connection connection = DriverManager.getConnection(url, user, password);
DSLContext create = DSL.using(connection, SQLDialect.MYSQL)) {
Result<Record> result = create.select()
.from("users")
.where(field("active").eq(1))
.fetch();
for (Record r : result) {
String name = r.get("name", String.class);
System.out.println("User: " + name);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Breakdown of the JOOQ Example
- We establish the connection similarly to JDBC.
- We use JOOQ's
DSLContext
to create a fluent query. - The SQL statement is more readable, and the compile-time safety ensures that mistakes are caught early.
Performance Considerations
Performance is always a hot topic when discussing database operations. JDBC might seem faster due to less abstraction, but the real benefits of JOOQ lie in developer productivity and fewer runtime errors.
However, both approaches can be optimized. For complex queries or bulk operations, JDBC may provide finer control over performance tuning, and using prepared statements can help prevent SQL injection attacks and improve performance.
Error Handling and Transactions
Error handling in JDBC requires manual management through try-catch blocks and ensuring that resources are properly released. JOOQ uses exceptions to inform developers of issues but automates resource management through its context.
Transactions can be managed in both with similar syntax, but JOOQ's fluent API makes it more intuitive:
JDBC Transaction Example
try {
connection.setAutoCommit(false);
// Execute multiple queries
statement.executeUpdate(query1);
statement.executeUpdate(query2);
connection.commit();
} catch (SQLException e) {
connection.rollback();
}
JOOQ Transaction Example
DSLContext create = DSL.using(connection, SQLDialect.MYSQL);
create.transaction(configuration -> {
DSLContext tx = DSL.using(configuration);
tx.execute(query1);
tx.execute(query2);
});
In the JOOQ example, the transactional scope is contained within a lambda, leading to cleaner code.
Key Takeaways
Choosing between JDBC and JOOQ often comes down to your project requirements, team experience, and personal preference. JDBC offers full control and is great for performance-focused development. However, JOOQ shines with its type-safe, fluent API and modern SQL integration.
In more complex applications, the maintainability and readability provided by JOOQ can significantly reduce development time and bugs, allowing developers to focus on building features rather than managing boilerplate code.
Further Reading
- Official JDBC Documentation
- JOOQ Documentation
- Java’s JDBC vs. JOOQ: A Comparison
In conclusion, assess your needs carefully before deciding. Both JDBC and JOOQ have their place in the Java ecosystem, and understanding their nuances will help you make informed architectural decisions.