Common Mistakes When Using iText for PDF Creation
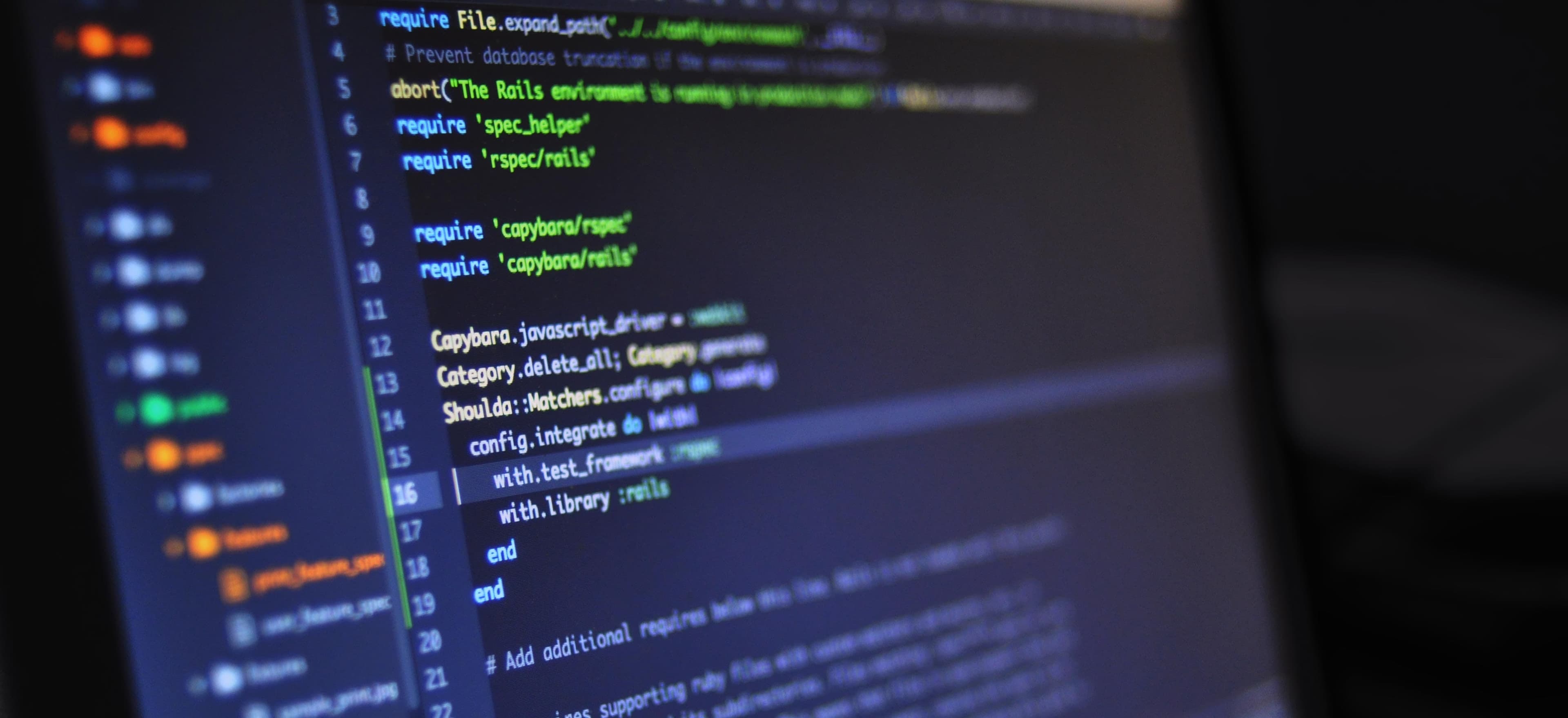
- Published on
Common Mistakes When Using iText for PDF Creation
Creating PDFs is a common requirement in software development, and iText has become one of the most popular libraries for this purpose in Java. While iText offers exceptional features and flexibility, developers often encounter pitfalls that can lead to inefficient code or unexpected results. In this blog post, we will discuss common mistakes made when using iText for PDF creation and how to avoid them.
1. Not Using the Right Version
One of the most common mistakes is not paying attention to which version of iText you're using. There are two main versions: iText 5 and iText 7. iText 5 is open-source but has limitations in licensing for commercial use, while iText 7 is fully commercial with more features and support.
Why This Matters
Using the wrong version can lead to complications in functionality and licensing. For example, many features present in iText 7 do not exist in iText 5, leading to incomplete implementations in applications.
Solution
Always consult the official iText documentation to choose the most appropriate version for your project needs.
// Example of using iText 7
PdfWriter writer = new PdfWriter("example.pdf");
PdfDocument pdf = new PdfDocument(writer);
Document document = new Document(pdf);
document.add(new Paragraph("Hello, World!"));
document.close();
2. Not Closing the Document Properly
Another elementary yet common mistake is forgetting to close the Document
object after completing the PDF creation. Failing to close it can lead to corruption in the PDF output or missing content.
Why This Matters
The Document
object ensures that all content is flushed to the file system. If it is not closed properly, the output may not be what you expect.
Solution
Always use the close()
method when you're done.
Document document = new Document(pdf);
document.add(new Paragraph("Hello, World!"));
// Closing the document
document.close(); // Make sure to close
3. Ignoring Exception Handling
Developers sometimes overlook implementing proper exception handling while using iText. PDF creation can result in various errors, and failing to handle these exceptions can crash the application.
Why This Matters
Exception handling provides a safety net, ensuring that your application can gracefully manage and log errors instead of failing unexpectedly.
Solution
Wrap your PDF creation code in try-catch blocks to capture potential exceptions.
try {
PdfWriter writer = new PdfWriter("example.pdf");
PdfDocument pdf = new PdfDocument(writer);
Document document = new Document(pdf);
document.add(new Paragraph("Hello, World!"));
document.close();
} catch (IOException e) {
System.err.println("Error while creating PDF: " + e.getMessage());
}
4. Forgetting to Set Document Properties
Document properties such as title, author, and subject are often neglected during PDF creation. These properties not only help in organizing files but also make them more searchable.
Why This Matters
Search engines and PDF readers often rely on document properties to display useful information about the PDF. Without these properties, users might find it hard to locate the document.
Solution
Use the PdfDocument
class to set these properties early in your PDF creation process.
PdfDocument pdf = new PdfDocument(writer);
pdf.getDocumentInfo().setTitle("My Document Title");
pdf.getDocumentInfo().setAuthor("Your Name");
5. Misunderstanding Font Handling
iText provides various options for fonts, but many developers don't fully understand how to embed fonts properly. Unsuitable font embedding can result in rendering issues or the absence of the expected font in the PDF.
Why This Matters
When a PDF is opened on a different system, if the font is not embedded, the viewer may substitute the font with a default one, altering the document's appearance.
Solution
Always embed the fonts you intend to use in your PDF.
PdfFont font = PdfFontFactory.createFont(FontConstants.HELVETICA, PdfEncodings.IDENTITY_H, true);
document.add(new Paragraph("Hello, World!").setFont(font)); // Embedding font
6. Not Using Page Sizes Correctly
Developers frequently assume that the default A4 size is suitable for all documents. However, different types of documents may require various page sizes.
Why This Matters
Incorrect page sizes can lead to layout issues. For example, a report may need a landscape orientation, whereas an invoice might work better in portrait.
Solution
Specify the page size explicitly when you create your document.
PageSize pageSize = PageSize.A4.rotate(); // Example of using landscape
PdfDocument pdf = new PdfDocument(new PdfWriter("example.pdf", new WriterProperties().setCompressionLevel(9)));
pdf.setDefaultPageSize(pageSize);
7. Inadequate Testing Across PDF Readers
After creating a PDF, developers sometimes limit their testing to a single PDF reader. This can lead to discrepancies in how the PDF is rendered in different applications.
Why This Matters
Each PDF reader may interpret PDF standards slightly differently, affecting layout, formatting, and interactivity.
Solution
Test your created PDFs in multiple readers, such as Adobe Acrobat, Foxit Reader, or even browser-based viewers.
Wrapping Up
While iText is a powerful tool for creating PDFs in Java, it’s essential to be aware of common mistakes that developers might encounter. By understanding these pitfalls and implementing the appropriate solutions, you can create robust, searchable, and visually appealing PDFs.
For further reading, check out the iText 7 Documentation, which offers extensive information on best practices and examples. Additionally, consider exploring iText’s Community, where you can ask questions and share your experiences with other iText users.
By being mindful of these common mistakes, you enhance your PDF creation workflow, ensuring both quality and reliability. Happy coding!