Mastering Higher-Order Array Functions: Common Pitfalls to Avoid
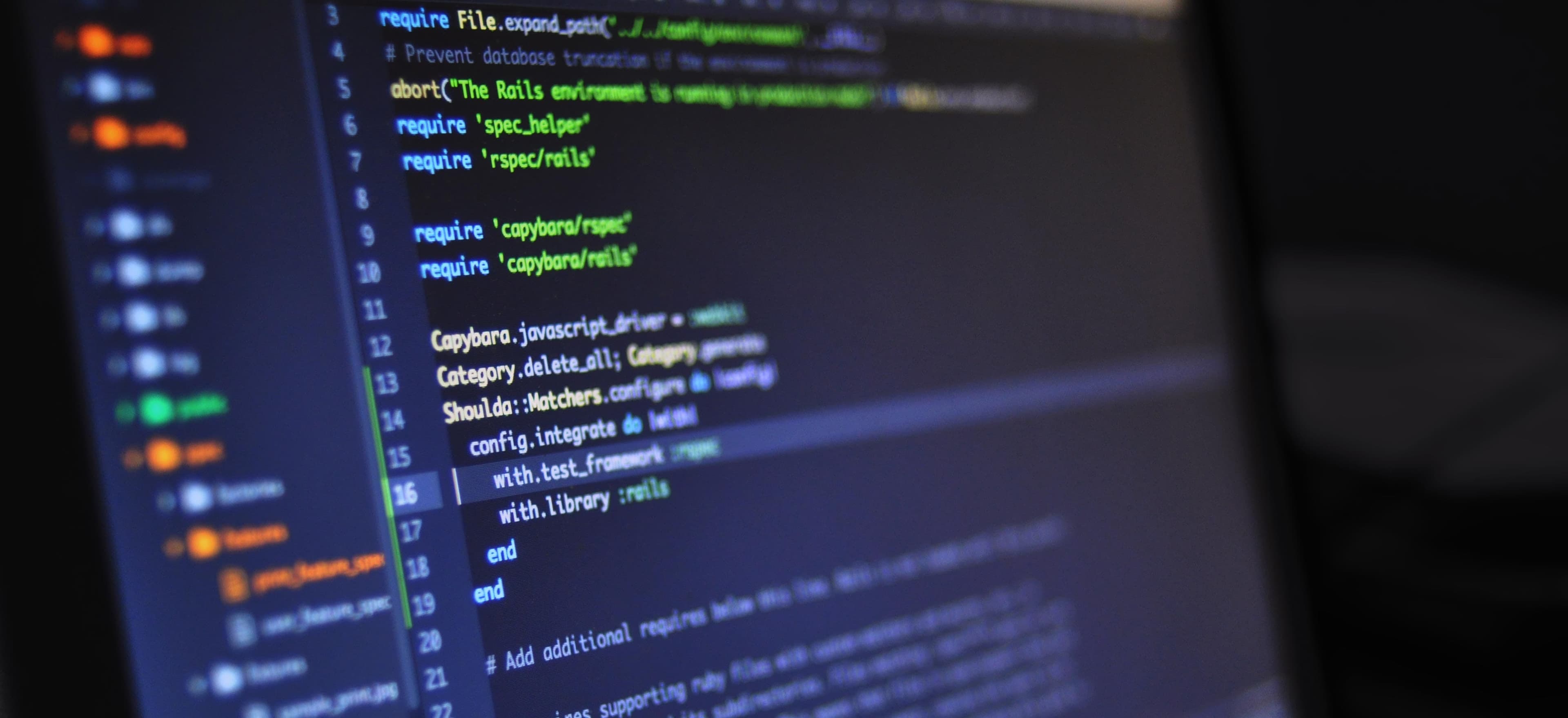
- Published on
Mastering Higher-Order Array Functions: Common Pitfalls to Avoid
JavaScript has transformed the way developers write code, especially with the introduction of higher-order functions. Among these, array functions such as map
, filter
, and reduce
have proven to be immensely powerful. These methods allow developers to manipulate arrays concisely while significantly improving code readability. However, despite their advantages, many developers encounter pitfalls when utilizing these functions. In this blog post, we will explore these higher-order array functions, examine common mistakes, and provide best practices to help you master them.
What Are Higher-Order Array Functions?
Higher-order functions are functions that either take other functions as arguments or return functions as their results. In JavaScript, array methods like map
, filter
, and reduce
are prime examples. These functions allow we to work with arrays in an expressive and declarative manner.
Example: Map Function
The map
function allows you to transform each element of an array based on a given function:
const numbers = [1, 2, 3, 4, 5];
const squares = numbers.map(num => num * num);
console.log(squares); // Output: [1, 4, 9, 16, 25]
In this code, we pass a function to map
, which takes each number and returns its square.
Common Pitfalls with Higher-Order Functions
Even experienced developers can fall into traps when working with higher-order functions. Below are some common pitfalls accompanied by solutions.
1. Ignoring Immutable Practices
A major selling point of higher-order functions is their ability to promote immutability. However, one common mistake is inadvertently modifying the original array.
Mistake Example
const numbers = [1, 2, 3, 4, 5];
const squares = numbers.map(num => {
numbers.push(num * num); // Mutating the original array
return num * num;
});
console.log(numbers); // Output: [1, 2, 3, 4, 5, 1, 4, 9, 16, 25]
The Correct Approach
To maintain immutability, always create a new array and avoid modifying the original:
const numbers = [1, 2, 3, 4, 5];
const squares = numbers.map(num => num * num);
console.log(numbers); // Output: [1, 2, 3, 4, 5]
console.log(squares); // Output: [1, 4, 9, 16, 25]
2. Misunderstanding Return Values
Many developers mistakenly assume the return value of higher-order functions. Contrary to what one might expect, map
will return a new array with transformed elements even if they are undefined
.
Mistake Example
const values = [1, 2, null, 4, 5];
const results = values.map(value => {
if(value == null) {
return; // Undefined is returned
}
return value * 2;
});
console.log(results); // Output: [2, 4, undefined, 8, 10]
The Correct Approach
Be explicit in your handling of cases where you do not want a return value.
const values = [1, 2, null, 4, 5];
const results = values.map(value => value == null ? 0 : value * 2); // Replace with a default value
console.log(results); // Output: [2, 4, 0, 8, 10]
3. Performance Issues with Heavy Computations
Performing heavy computations inside map
, filter
, or reduce
can lead to performance degradation. This is especially true if these functions are chained together multiple times.
Mistake Example
const largeArray = Array.from({length: 1000000}, (_, i) => i); // Create a large array
const result = largeArray
.map(num => {
// Simulating heavy computation
for(let i = 0; i < 1000000; i++) {}
return num * 2;
})
.filter(num => num > 1000);
The Correct Approach
If performance becomes an issue, consider processing the array in smaller chunks or simplifying the operations.
const largeArray = Array.from({length: 1000000}, (_, i) => i);
const filteredResult = [];
largeArray.forEach(num => {
if(num > 500) { // Added filter during iteration
filteredResult.push(num * 2);
}
});
// Use filter appropriately; reduce unnecessary computations
4. Not Using Arrow Functions
Arrow functions offer a more compact alternative to traditional function expressions. However, many developers still cling to the verbose function declaration.
Traditional Way
const numbers = [1, 2, 3, 4];
const squares = numbers.map(function(num) {
return num * num;
});
Using Arrow Function
const numbers = [1, 2, 3, 4];
const squares = numbers.map(num => num * num); // Simpler and cleaner
5. Overusing Reduce
The reduce
function can be overwhelmingly powerful but also confusing. Many developers are tempted to use it for every type of transformation, leading to unreadable code.
Mistake Example
const numbers = [1, 2, 3, 4];
const sum = numbers.reduce((acc, curr) => {
return acc + curr;
}, 0);
While the above is valid, using reduce
for simple summation may be overkill when forEach
or a simple sum loop suffices.
The Correct Approach
Use reduce
when you truly need to compile results into a single value or structure.
const sum = numbers.reduce((acc, curr) => acc + curr, 0);
console.log(sum); // Output: 10
Best Practices for Higher-Order Functions
-
Always Maintain Immutability: Treat your data as immutable; it prevents side effects and makes your code easier to understand.
-
Be Explicit with Return Values: Always handle
undefined
cases to avoid negative impacts on subsequent transformations. -
Consider Performance: If your array functions are becoming slow, rethink their implementation. Avoid using them for heavy calculations.
-
Favor Readability: Use shorter function forms like arrow functions for cleaner, more concise code.
-
Limit Reduce to Specific Cases: Use
reduce
judiciously to avoid decreasing the readability of your code.
A Final Look
Mastering higher-order functions in JavaScript is about understanding their proper usage and recognizing pitfalls to avoid. By adhering to best practices and maintaining a clear structure in your code, you can harness the full power of array methods while preventing common mistakes.
For further reading on JavaScript array methods, you can explore more at MDN Web Docs. Happy coding, and remember—practice makes perfect!