Mastering URL Rewriting in Camel 2.11 HTTP Proxy Routes
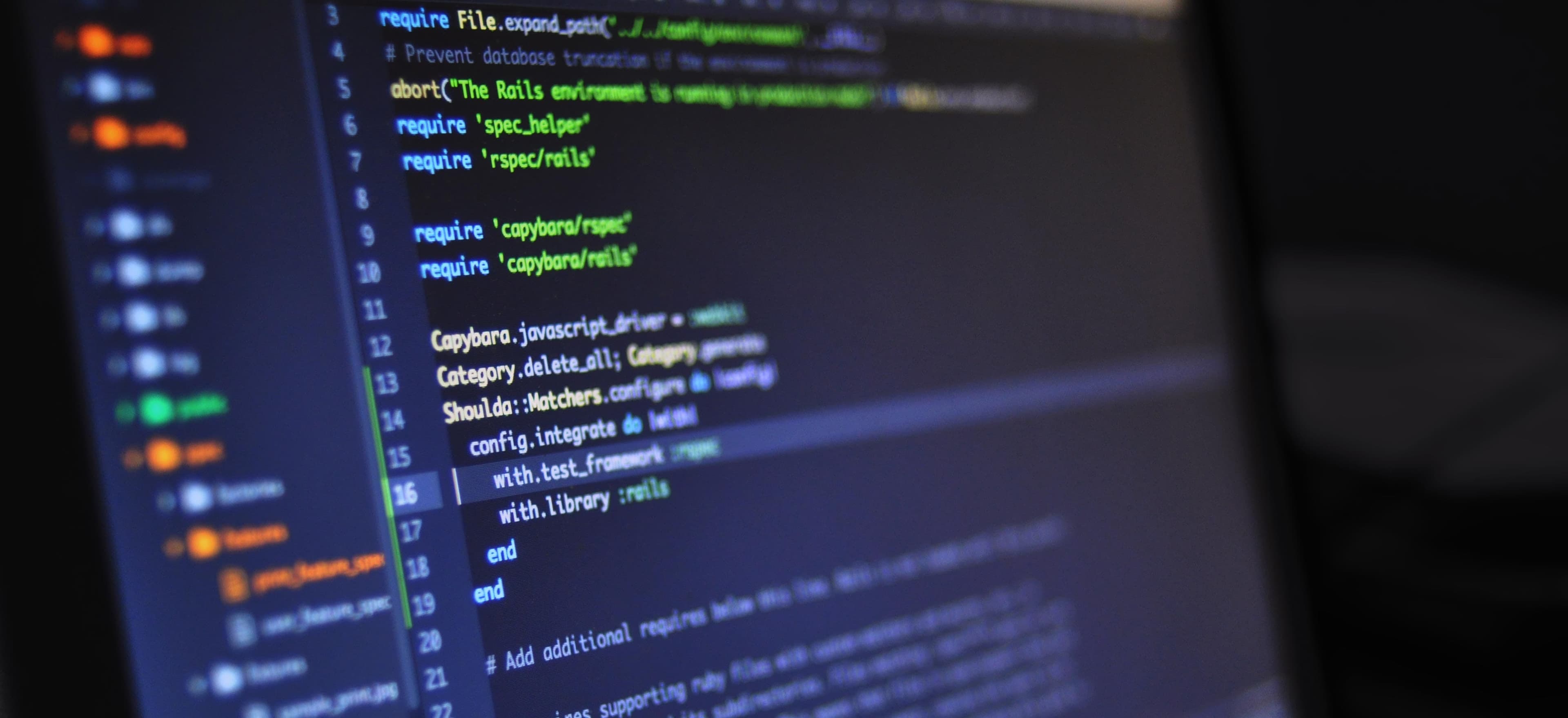
- Published on
Mastering URL Rewriting in Camel 2.11 HTTP Proxy Routes
In the realm of integration frameworks, Apache Camel stands out for its simplicity and effectiveness. As applications grow, the need for clean and manageable routes becomes ever more critical. One of the techniques to keep your HTTP routes organized and efficient is URL rewriting. In this blog post, we will delve deep into URL rewriting in Camel 2.11's HTTP Proxy Routes, exploring why it matters, how to implement it, and some real-world scenarios where it shines.
What is URL Rewriting?
URL rewriting is the process of modifying the incoming request URL before it is processed by a server or application. This technique is often employed to:
- Simplify URLs for users.
- Enhance security by hiding underlying application endpoints.
- Facilitate integration with legacy systems.
In Camel, this technique is crucial as it allows you to create routes that are flexible and maintainable.
Understanding Camel HTTP Proxy Routes
Before diving into URL rewriting, it's essential to understand how Camel HTTP Proxy Routes work. In Camel, a proxy route allows you to direct incoming HTTP requests to a specific backend service while optionally transforming the requests and responses.
A typical Camel HTTP Proxy Route might look something like this:
from("jetty:http://0.0.0.0:8080/myproxy")
.to("http://localhost:8081/mybackend");
In this example, incoming requests to http://yourdomain.com/myproxy
will be forwarded to http://localhost:8081/mybackend
.
The Need for URL Rewriting
Imagine a scenario where you want to expose a clean URL to the end-users but internally, the API structure changes or is complex. For instance, instead of users calling http://yourdomain.com/myproxy/user?id=123
, you'd prefer them to use http://yourdomain.com/myproxy/user/123
.
This is where URL rewriting comes into play.
Creating a URL Rewriting Proxy Route
Here's a simplified version of how to implement URL rewriting in Camel 2.11:
Step 1: Define Your Route
Start by defining your HTTP proxy route in a RouteBuilder
class. You will use a regular expression to rewrite the URL:
import org.apache.camel.builder.RouteBuilder;
public class RewriteRouteBuilder extends RouteBuilder {
@Override
public void configure() {
from("jetty:http://0.0.0.0:8080/myproxy/user")
.process(exchange -> {
String path = exchange.getIn().getHeader("CamelHttpUri", String.class);
String newPath = path.replaceAll("user\\?id=(\\d+)", "user/$1");
exchange.getIn().setHeader("CamelHttpUri", newPath);
})
.to("http://localhost:8081/mybackend");
}
}
Step 2: URL Rewriting Logic
In the code above:
- We set up an HTTP route that listens on
http://0.0.0.0:8080/myproxy/user
. - The incoming requests should include a query parameter
id
. - The processor rewrites the URL from the query-style (
user?id=123
) to a cleaner path-style (user/123
) using a regular expression.
Step 3: Test Your Route
After defining your route, it's crucial to test it to ensure it behaves as expected. You can test it using tools like Postman or curl.
Example curl command:
curl "http://localhost:8080/myproxy/user?id=123"
If everything is set up correctly, your backend service should receive the request as if it was sent to http://localhost:8081/mybackend/user/123
.
Error Handling
One of the most critical aspects of any integration scenario is fault tolerance and error handling. Camel offers a powerful mechanism for error handling, allowing us to gracefully manage any issues during the rewriting process.
onException(Exception.class)
.handled(true)
.process(exchange -> {
exchange.getIn().setBody("An error occurred during URL rewriting.");
exchange.getIn().setHeader("Content-Type", "text/plain");
});
In this example:
- We're capturing any exception that occurs in our route.
- We're marking it as handled so that it won't propagate further.
- We're also setting a friendly message in the response.
Redirecting Users
In some cases, if URL rewriting fails, you may wish to redirect users back to a static page or a different endpoint. To do this, extend the error handling to include redirection:
from("jetty:http://0.0.0.0:8080/myproxy/user")
.doTry()
.process(exchange -> {
String path = exchange.getIn().getHeader("CamelHttpUri", String.class);
String newPath = path.replaceAll("user\\?id=(\\d+)", "user/$1");
exchange.getIn().setHeader("CamelHttpUri", newPath);
})
.doCatch(Exception.class)
.process(exchange -> {
exchange.getIn().setHeader(Exchange.HTTP_RESPONSE_CODE, 302);
exchange.getIn().setHeader(Exchange.HTTP_LOCATION_HEADER, "http://yourdomain.com/myerrorpage");
});
In this block:
- We're using a try-catch block to separate our normal processing from error handling.
- In case of an exception, we set an HTTP response code of 302 to redirect users.
Advanced URL Rewriting Techniques
As your application scales, your URL rewriting logic may require greater complexity. Here are two advanced techniques that can help you maintain your routes more efficiently:
-
Use of Camel DSL: Leverage the DSL (Domain Specific Language) to create more readable and manageable routes. Camel's fluent syntax allows you to use constructs such as
.choice()
,.when()
, and.otherwise()
to refine your URL handling logic. -
External Configuration: Store your rewriting rules in an external configuration file (e.g., JSON or XML). This way, you can alter behavior without modifying the Java code. Implement a processor that reads rules from the file and processes URLs dynamically.
Closing Thoughts
Mastering URL rewriting in Apache Camel 2.11's HTTP Proxy Routes is a worthwhile investment. Not only does it enhance your application's professionalism, but it also creates a better user experience by providing clean and meaningful URLs.
Whether you are building microservices or integrating legacy systems, having a solid grasp of URL rewriting will certainly make your journey smoother.
Additional Resources
If you're interested in learning more about Camel and URL handling concepts, check out:
With these tools and techniques at your disposal, you are now ready to tackle URL rewriting with confidence in your Apache Camel projects. Happy coding!