Mastering JBox2D: Overcoming JavaFX Event Handling Challenges
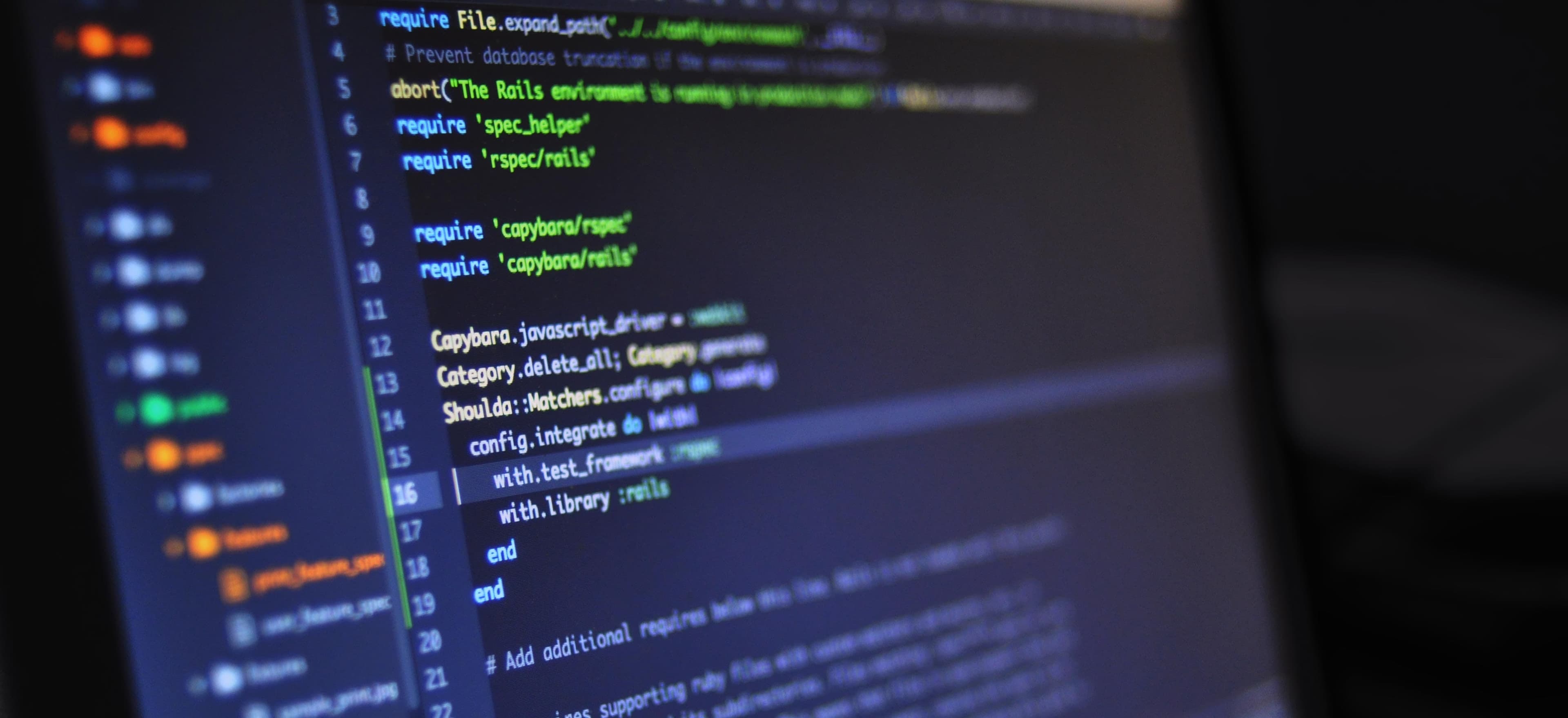
- Published on
Mastering JBox2D: Overcoming JavaFX Event Handling Challenges
When working with physics simulations in Java, JBox2D is a formidable library offering a robust suite of tools for 2D physics. However, integrating JBox2D with JavaFX introduces a unique set of challenges, particularly regarding event handling. In this post, we'll explore common scenarios and provide solutions for effective event handling, all while ensuring that our JavaFX application remains responsive and user-friendly.
Understanding the Basics of JBox2D
JBox2D is a Java port of the Box2D physics engine, originally built for C++. It allows developers to simulate rigid body physics, enabling them to create interactive experiences in 2D spaces. The library includes various components such as bodies, fixtures, joints, and more, all essential for establishing physical simulations.
To get started, it's important to familiarize yourself with the core concepts of JBox2D. Here's a quick structure of how you would generally initialize a simple physics world:
World world = new World(new Vector2(0, -9.81f), true);
In this snippet, we define a physics world with a gravity vector pointing down (simulating Earth’s gravity). The second parameter indicates whether to allow sleeping, which lets bodies come to rest when still.
Integrating JBox2D with JavaFX
JavaFX offers a rich set of UI functionalities that can be integrated with JBox2D physics simulation. The combination allows you to create interactive applications with two-dimensional physics, such as games or simulations. However, you might face challenges in event handling, which is crucial for user interactions.
Adding JavaFX Event Handlers
JavaFX provides a comprehensive event handling model. For example, we'll explore mouse clicks, keyboard events, and drag events. Here's how you can listen for mouse click events on a JavaFX Pane
.
pane.setOnMouseClicked(event -> {
// Handle click event
System.out.println("Mouse Clicked at: " + event.getX() + ", " + event.getY());
});
In the above snippet, the setOnMouseClicked
method binds a lambda expression that executes when the user clicks on the pane. The x and y coordinates of the click are accessible through the event object.
Challenge: Mapping JavaFX Events to JBox2D Bodies
One common issue arises when trying to map JavaFX events to JBox2D bodies, especially when your rendering and physics worlds are asynchronous. For example, you may want to select a JBox2D body when the user clicks on it. Here's an effective way to tackle this:
Getting Coordinates
First, translate the click coordinates from JavaFX to JBox2D world coordinates. This is crucial, as JavaFX uses pixels while JBox2D operates in real-world meters.
float mouseX = event.getX() / pixelsToMeters;
float mouseY = event.getY() / pixelsToMeters;
Finding the Nearest JBox2D Body
Once you have the coordinates, you can check which body, if any, was clicked. For this, JBox2D provides a ray-casting functionality.
RayCastCallback callback = new RayCastCallback() {
public float reportFixture(Fixture fixture, Vector2 point, Vector2 normal, float fraction) {
// You can choose to get body and act accordingly
Body body = fixture.getBody();
System.out.println("Hit body: " + body);
return fraction; // This allows the ray to continue until the first hit, or zero to ignore.
}
};
world.raycast(callback, new Vector2(mouseX, mouseY), new Vector2(mouseX, mouseY));
By implementing this RayCastCallback, you can determine which fixture was hit by the mouse click and obtain the associated body.
Challenge: Updating the Physics World
The physics simulation must be updated periodically – typically done in a game loop. JavaFX uses a Platform.runLater which can help keep the UI responsive while processing physics updates in a separate thread.
Here's a simple game loop setup using JavaFX Timeline:
Timeline timeline = new Timeline(new KeyFrame(Duration.millis(16), e -> {
world.step(1 / 60.0f, 6, 2); // Update physics world
render(); // Custom render method to update JavaFX nodes
}));
timeline.setCycleCount(Animation.INDEFINITE);
timeline.play();
In this loop, the physics world is stepped forward for a frame duration of approximately 16 milliseconds (which corresponds to 60 frames per second).
Rendering JBox2D Bodies in JavaFX
To visualize JBox2D bodies, we need to draw JavaFX shapes that correspond to JBox2D bodies. This typically involves mapping JBox2D coordinates to JavaFX coordinates.
Here’s a basic example of rendering:
void render() {
pane.getChildren().clear(); // Clear existing drawings
for (Body body : bodies) {
// Get position and angle of the body
Vector2 position = body.getPosition();
double angle = body.getAngle();
// Create a visual representation (Rectangle for simplicity here)
Rectangle rectangle = new Rectangle(position.x * metersToPixels, position.y * metersToPixels, width, height);
rectangle.setRotate(Math.toDegrees(angle));
pane.getChildren().add(rectangle);
}
}
In this method, we clear the pane and then create a new Rectangle for each JBox2D body, setting its position, dimensions, and rotation according to the body's properties.
Handling Multiple Events
In a more complex application, you might have multiple event interactions. For example, responding to both mouse and keyboard events while maintaining a smooth user experience can be tricky.
You can enhance your event handling using a centralized event manager or controller class that can manage these interactions more fluidly.
Best Practices for JavaFX and JBox2D
-
Thread Safety: Ensure that updates to the UI are done on the JavaFX Application Thread, while physics calculations can take place on a separate thread.
-
Event Filtering: If the application needs to handle multiple overlapping events, consider using event filters for greater control.
-
Optimization: Only update the rendering and events that are necessary. This way, you'll ensure the application runs at optimal performance.
-
Debugging: Use logging effectively to monitor the flow of the application, especially during event handling.
Lessons Learned
Integrating JBox2D into a JavaFX application offers tremendous potential for creating engaging simulations and games. Although there are challenges, especially regarding event handling and rendering, you can effectively navigate these issues with the outlined approaches.
In summary, understanding the interplay between JBox2D's physics world and JavaFX's event handling model is crucial for developing seamless applications. By using ray-casting for hit detection and a structured game loop for updates, you can achieve fluid interactions in your JavaFX applications.
For further reading on JBox2D, you might find the official documentation helpful: JBox2D Documentation.
Feel free to share your experiences with JBox2D and JavaFX, or any challenges you've encountered in the comments below. Happy coding!
Checkout our other articles