Overcoming Challenges with User Defined Types in Java DB
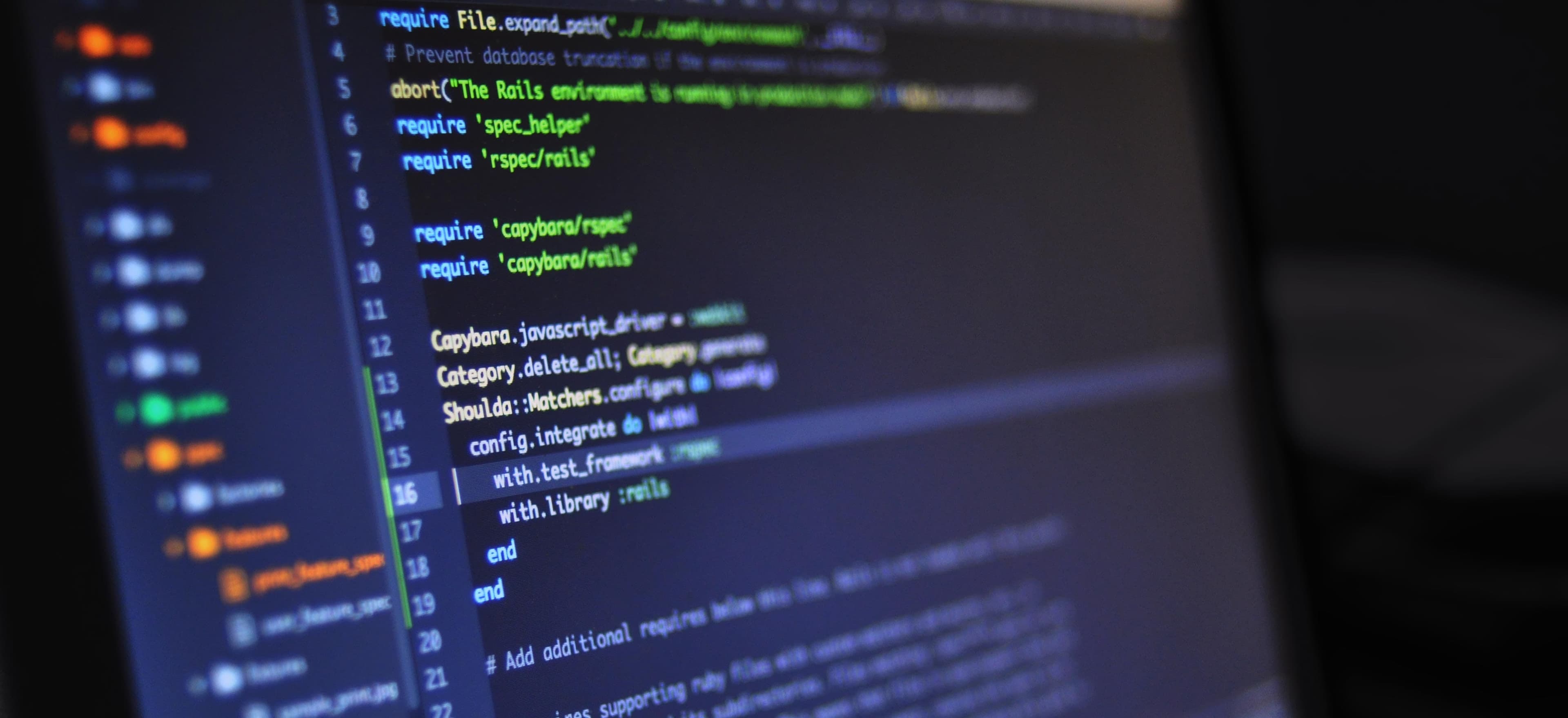
- Published on
Overcoming Challenges with User Defined Types in Java DB
Java is a versatile programming language known for its extensive capabilities in various applications, notably in database management. As developers create complex applications, the need for User Defined Types (UDTs) in Java DB rises. UDTs allow developers to define their data types, effectively modeling real-world entities. However, incorporating UDTs brings challenges that can complicate program maintenance and performance. In this post, we'll delve into how to effectively implement and overcome these challenges while optimizing your Java DB experience.
What Are User Defined Types?
User Defined Types (UDTs) allow developers to define custom data types, making data representation more tailored and efficient. For instance, if you were developing a banking application, creating a Currency
type instead of simply using double
for amounts allows you to encapsulate precision, currency codes, and even conversion logic within the type.
Benefits of Using UDTs
- Data Integrity: By encapsulating the logic within types, you can ensure that only valid data formats are processed.
- Reusability: Once created, a UDT can be reused across different tables and contexts.
- Simplicity: UDTs enhance code legibility and simplify complex data relationships.
Challenges with User Defined Types in Java DB
Despite their advantages, working with UDTs in Java DB presents some challenges:
1. Complexity in Definition
When defining UDTs, it often requires careful thinking and planning. A poorly defined UDT can lead to unwanted side effects.
2. Serialization and Deserialization Issues
Java DB requires UDTs to be serialized into a database-compatible format. This conversion can sometimes introduce bugs or incorrect data handling.
3. Performance Implications
Using UDTs can affect database performance. Correctly implementing these types needs insertion, retrieval, and indexing considerations.
Implementing User Defined Types in Java DB
Step 1: Defining a UDT
Let’s walk through the creation of a UDT for a Currency
type that represents monetary values.
Example Code Snippet
import java.math.BigDecimal;
import java.sql.Array;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class Currency {
private BigDecimal amount;
private String currencyCode;
public Currency(BigDecimal amount, String currencyCode) {
this.amount = amount;
this.currencyCode = currencyCode;
}
// Getters and Setters
}
Commentary
In this code, we create a simple Currency
class with an amount and a currency code. By using BigDecimal
, we can maintain precision, which is critical for currency calculations.
Step 2: Registering the UDT
Next, you need to inform your Java DB about the Currency
type. You can achieve this with the following SQL command:
CREATE TYPE Currency AS (amount DECIMAL, currencyCode VARCHAR(3));
This command outlines the attributes the Currency
UDT will exhibit.
Step 3: Inserting UDT Values
To insert a Currency
type into a database, you must convert your Java object to a compatible SQL object.
Example Code Snippet
public void insertCurrency(Connection conn, Currency currency) throws SQLException {
String sql = "INSERT INTO transactions (currency) VALUES (?)";
try (PreparedStatement ps = conn.prepareStatement(sql)) {
Array currencyArray = conn.createArrayOf("Currency", new Object[]{currency});
ps.setArray(1, currencyArray);
ps.executeUpdate();
}
}
Commentary
In this method, we create an Array
in SQL from our Currency
object. This is essential as Java DB needs a compatible object type to correctly store the UDT.
Step 4: Retrieving UDT Values
Retrieving UDT values can also be tricky due to serialization. You need to convert SQL representation back to your Java class.
Example Code Snippet
public Currency getCurrencyById(Connection conn, int id) throws SQLException {
String sql = "SELECT currency FROM transactions WHERE id = ?";
try (PreparedStatement ps = conn.prepareStatement(sql)) {
ps.setInt(1, id);
ResultSet rs = ps.executeQuery();
if (rs.next()) {
Array currencyArray = rs.getArray("currency");
Object[] currencyData = (Object[]) currencyArray.getArray();
return new Currency((BigDecimal)currencyData[0], (String)currencyData[1]);
}
}
return null; // Handle no result case appropriately
}
Commentary
This retrieval method fetches the currency stored in the database and converts it back into a Currency
object. Proper error checking is critical here as other complexities can arise from database constraints or incomplete data entries.
Best Practices for Working with UDTs
- Plan UDT Structure: Invest the time to plan your UDT's structure carefully to ensure it aligns with your application’s needs.
- Implement Validation Logic: Include validation checks within your UDT to ensure data integrity.
- Monitor Performance: Regularly analyze how UDTs affect application performance and optimize accordingly.
Closing Remarks
User Defined Types in Java DB are powerful tools for developers aiming to create robust applications. Understanding and overcoming the challenges associated with their implementation and management will greatly benefit your software engineering endeavors.
In summary, by effectively defining, registering, and managing UDTs, you can ensure data integrity, boost code reusability, and enhance application readability. Adhering to best practices while monitoring performance will position your Java DB applications for success.
For more insights on Java database management, check out Oracle’s Java Documentation and Java Tutorials. Happy coding!
Checkout our other articles