Overcoming Permissions Issues in Azure Blob for Maven
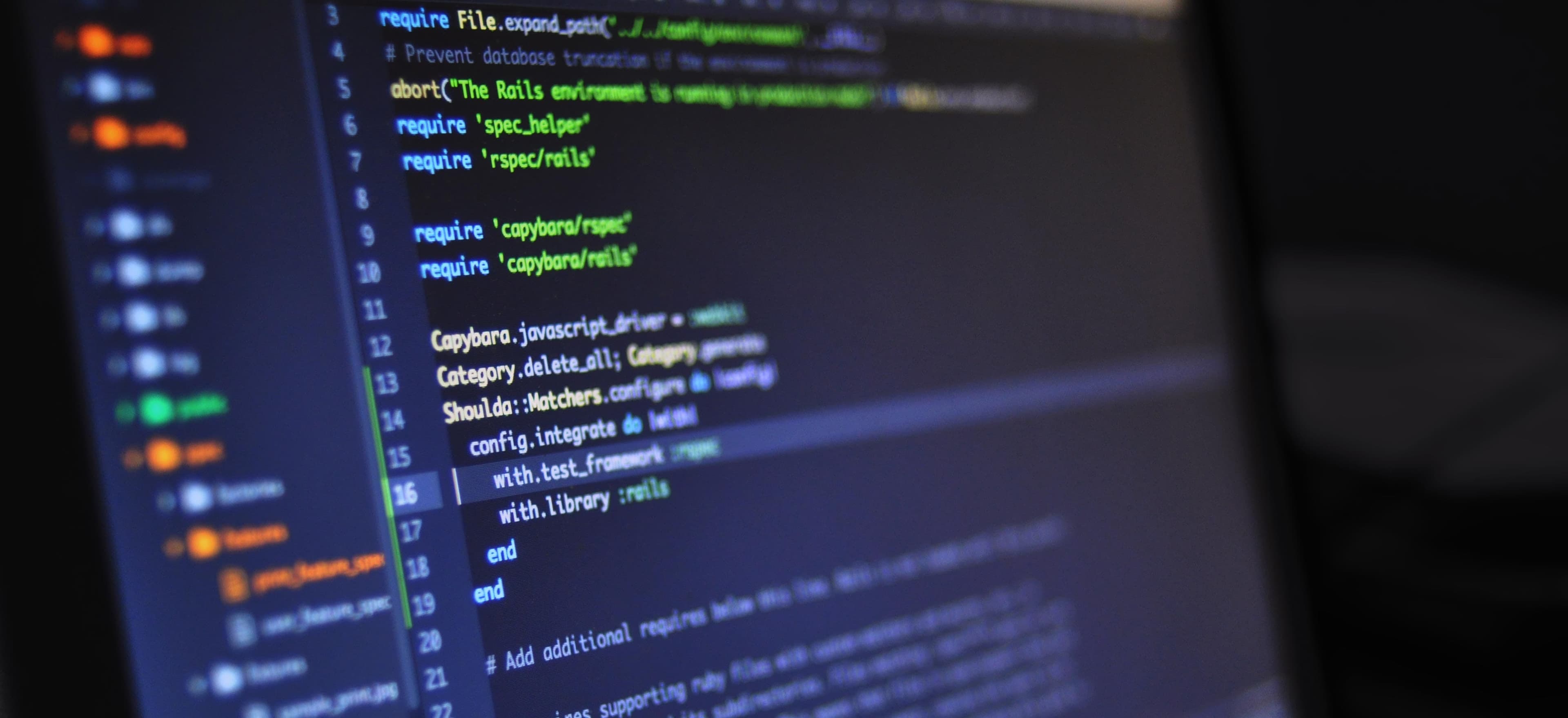
- Published on
Overcoming Permissions Issues in Azure Blob for Maven
When working with Azure Blob Storage, managing access and permissions is crucial, especially in a CI/CD pipeline where tools like Maven are involved. This blog will walk you through common permissions issues in Azure Blob, how they can affect your Maven builds, and how to resolve them.
Azure Blob Storage and Maven
Azure Blob Storage is a scalable solution for storing large amounts of unstructured data. This includes documents, media files, and other non-relational data types. Maven, a popular build automation tool primarily for Java projects, can easily integrate with Azure Blob Storage for tasks such as storing build artifacts or pulling dependencies.
However, mishandling permissions can lead to frustrating problems. Therefore, understanding permissions in Azure and their integration with Maven is essential.
Understanding Azure Blob Storage Permissions
Before diving into troubleshooting, it's important to understand the types of permissions available in Azure Blob Storage. Access can be granted at the account level or the container level through several means:
-
Shared Access Signatures (SAS): This allows limited access to Azure Storage resources without exposing the account key. SAS tokens are time-limited and can restrict access to specific operations.
-
Stored Access Policies: These provide more granular control over SAS tokens by managing permissions under a security policy.
-
Role-Based Access Control (RBAC): This is an Azure feature that provides fine-grained access to resources, allowing administrators to define roles and assign them to users, groups, and applications.
Common Permissions Issues
Working with these permission types, developers frequently encounter challenges such as:
- Insufficient permissions when trying to upload artifacts
- Denying access when attempting to access the blob storage
- Timeouts or unexpected failures during artifact retrieval
Diagnosing Permission Issues
The first step in resolving permissions issues with Azure Blob Storage in Maven is to identify what type of error you are encountering. Here are some common error messages and their implications:
-
403 Forbidden: This typically signals issues with your SAS token or insufficient permissions. You might be trying to access a resource that your token doesn't have permission for.
-
404 Not Found: This often indicates that the resource (i.e., a blob or container) does not exist; however, it can also suggest that the user does not have permission to view it.
-
Timeout errors: These can indicate network issues, but if they occur after numerous successful runs, permissions could be in question.
Use Azure Storage Explorer
A practical tool to test permissions is the Azure Storage Explorer. This user-friendly GUI allows you to connect to your Azure Blob Storage account, browse your blobs, and verify whether you can perform the operations you require.
Configuring Maven to Work with Azure Blob
Before resolving permissions, ensure that your Maven setup is correctly configured to access Azure Blob Storage. Below is a simple example of a pom.xml
file where you configure an Azure repository.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
<repositories>
<repository>
<id>my-azure-repo</id>
<url>https://<your-account-name>.blob.core.windows.net/<your-container-name>/</url>
<releases>
<enabled>true</enabled>
</releases>
</repository>
</repositories>
</project>
In this snippet, replace <your-account-name>
and <your-container-name>
with the appropriate values.
Why This Configuration Matters
This configuration allows Maven to fetch dependencies directly from Azure Blob Storage. However, if permissions are not set correctly, Maven won’t be able to access the repository, leading to build failures.
Resolving Permissions Issues
1. Check SAS Tokens
If you are using SAS tokens for access, ensure the token is properly configured to allow the necessary permissions. For instance, a valid SAS token for writing blobs should include permissions such as:
sp=w&st=2021-06-01T00:00:00Z&se=2021-06-01T01:00:00Z&spr=https&sv=2020-04-08&sr=c&sig=<signature>
Where sp=w
indicates write permissions.
2. Utilize Stored Access Policies
Using stored access policies allows you to have a set of permissions dynamically. To create a stored access policy in Azure, use the following Azure CLI command:
az storage container policy create --account-name <YourAccountName> --container-name <YourContainerName> --name <PolicyName> --permissions rwdl
Replace <YourAccountName>
, <YourContainerName>
, and <PolicyName>
as required.
3. Implement Role-Based Access Control (RBAC)
If your scenario permits it, consider using RBAC for assigning permissions. You can provide an Azure AD user, group, or service principal the necessary roles (like Contributor or Blob Data Contributor) to interact with your blob storage. Here’s how to assign a role using Azure CLI:
az role assignment create --assignee <YourAssignee> --role "Storage Blob Data Contributor" --scope /subscriptions/<YourSubscriptionId>/resourceGroups/<YourResourceGroup>/providers/Microsoft.Storage/storageAccounts/<YourAccountName>
4. Testing Permissions
After making changes to permissions, it’s good practice to test access. Again, Azure Storage Explorer or a simple curl command can be useful here. Use the following curl command to test access:
curl -X GET "https://<your-account-name>.blob.core.windows.net/<your-container-name>/<your-blob-name>?<sas-token>"
If you receive a valid response, your permissions are set correctly.
Closing Remarks
Permissions management is crucial when working with Azure Blob Storage through Maven. Understanding how permissions work, diagnosing common issues, and applying the correct settings are foundational for a seamless experience. By implementing the suggestions outlined in this blog, you should be able to overcome common permissions issues effectively.
Learning More
For further reading on Azure Blob Storage permissions, consider exploring:
- Azure Blob Storage Overview
- Manage access permissions for Storage Account
Don’t hesitate to share your experiences or challenges in the comments below! Happy coding!
Checkout our other articles