Troubleshooting JAXB and Jersey 2 Integration Issues
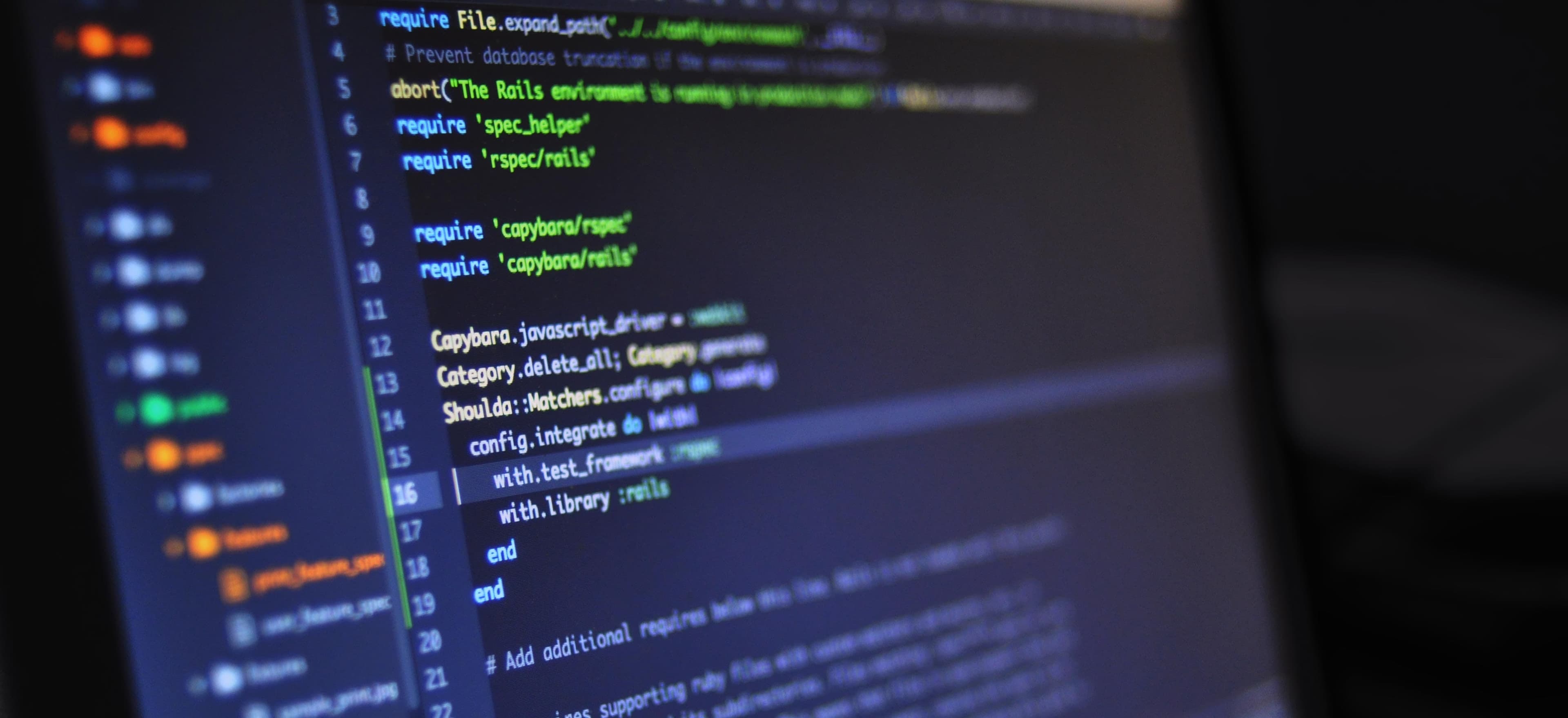
- Published on
Troubleshooting JAXB and Jersey 2 Integration Issues
In the realm of Java web services, integrating JAXB (Java Architecture for XML Binding) with Jersey 2 (the JAX-RS reference implementation) is a common necessity. While both are robust in their own right, developers often encounter integration issues. Understanding the nature of these problems can significantly enhance your web service development efficiency. This blog post delves into common integration challenges and offers practical solutions for troubleshooting them.
Understanding JAXB and Jersey
What is JAXB?
JAXB is a framework that allows Java developers to convert Java objects into XML and vice versa without extensive manual coding. By annotating Java classes, developers can define XML representations, simplifying data interchange between Java applications and XML-based services.
What is Jersey 2?
Jersey 2 is an implementation of JAX-RS (Java API for RESTful Web Services), which provides a set of APIs for creating RESTful web services in Java. It enables developers to expose resources as REST endpoints for seamless communication over HTTP.
Why Integrate JAXB with Jersey?
Integrating JAXB with Jersey allows automatic marshalling and unmarshalling of Java objects to and from XML format. This integration facilitates easier data exchange between client and server, enhancing the capability of RESTful web services.
Common Integration Issues
Here, we outline some frequent problems you may encounter when integrating JAXB with Jersey and the strategies to resolve them.
1. JAXB Context Not Found
One of the common issues is the inability to find the JAXB context for a particular Java object. This often manifests as runtime exceptions indicating that the class cannot be marshalled or unmarshalled.
Solution: Ensure Correct Annotations
You must annotate your Java classes correctly to ensure that JAXB knows how to process them.
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class User {
private String name;
private int age;
// Getters and setters
}
In this example, the @XmlRootElement
annotation informs JAXB that this class can be mapped to an XML element. Always verify that your classes have proper JAXB annotations.
2. Incorrect Media Type
Another common issue arises from using the wrong media type in your Jersey resource configuration. By default, Jersey may not recognize XML content types if they are not properly configured.
Solution: Set Correct Media Type
Ensure your resource returns the correct media type:
import javax.ws.rs.Produces;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
@Path("/user")
public class UserResource {
@GET
@Produces("application/xml") // Specify the media type
public User getUser() {
User user = new User();
user.setName("John Doe");
user.setAge(30);
return user;
}
}
By explicitly setting the @Produces
annotation, you inform Jersey that this endpoint returns XML content, aiding in the correct serialization of Java objects.
3. Missing JAXB Provider
Jersey applications must have JAXB capability registered. Failing to register the JAXB provider can lead to failures in processing XML responses.
Solution: Register the JAXB Feature
You can register the JAXB feature by adding the following code to your Jersey application configuration:
import org.glassfish.jersey.server.ResourceConfig;
public class MyApplication extends ResourceConfig {
public MyApplication() {
packages("com.example"); // Specify your package to scan
register(org.glassfish.jersey.jaxb.internal.JaxbFeature.class); // Register JAXB feature
}
}
This ensures that Jersey is aware of JAXB's functionality, enabling smooth communication between the two frameworks.
4. Schema Validation Errors
When working with XML, you might face schema validation issues. These typically arise when the XML generated does not conform to the expected schema.
Solution: Validate against XSD
To validate marshalling results against a specific XML Schema Definition (XSD), you can utilize JAXB's Schema Validation:
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Unmarshaller;
import javax.xml.validation.Schema;
import javax.xml.validation.SchemaFactory;
SchemaFactory schemaFactory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
Schema schema = schemaFactory.newSchema(new File("user.xsd")); // Load your XSD file
Unmarshaller unmarshaller = JAXBContext.newInstance(User.class).createUnmarshaller();
unmarshaller.setSchema(schema);
User user = (User) unmarshaller.unmarshal(new File("user.xml")); // Validate against XSD
This code snippet creates a JAXB unmarshaller that validates the XML input against the specified schema, ensuring your service consumes only well-formed data.
5. XML Namespace Issues
JAXB can produce XML output that includes namespaces, which might not match the expected format in your Jersey application.
Solution: Handle Namespaces Explicitly
You can manage namespaces in your JAXB-annotated classes by specifying them:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "User", namespace = "http://www.example.com/users")
public class User {
private String name;
private int age;
@XmlElement(namespace = "http://www.example.com/users")
public String getName() {
return name;
}
@XmlElement(namespace = "http://www.example.com/users")
public int getAge() {
return age;
}
}
By defining the namespace in both class and element annotations, you can prevent mismatches in expected XML output.
Wrapping Up
Integrating JAXB with Jersey 2 streamlines the process of developing robust RESTful web services in Java. By understanding the common issues and their solutions, you can troubleshoot integration problems effectively.
For a deeper understanding of JAXB, explore the official JAXB documentation. For Jersey, refer to the Jersey user guide.
Through diligent attention to detail in annotations, media types, JAXB configuration, and schema validation, you can build XML-based web services that are both reliable and effective. Should you come across any obstacles beyond those discussed, consider consulting community forums such as Stack Overflow or the Jersey mailing list for further assistance. Happy coding!
Checkout our other articles