Solving JAXB Date Formatting Issues in Spring Applications
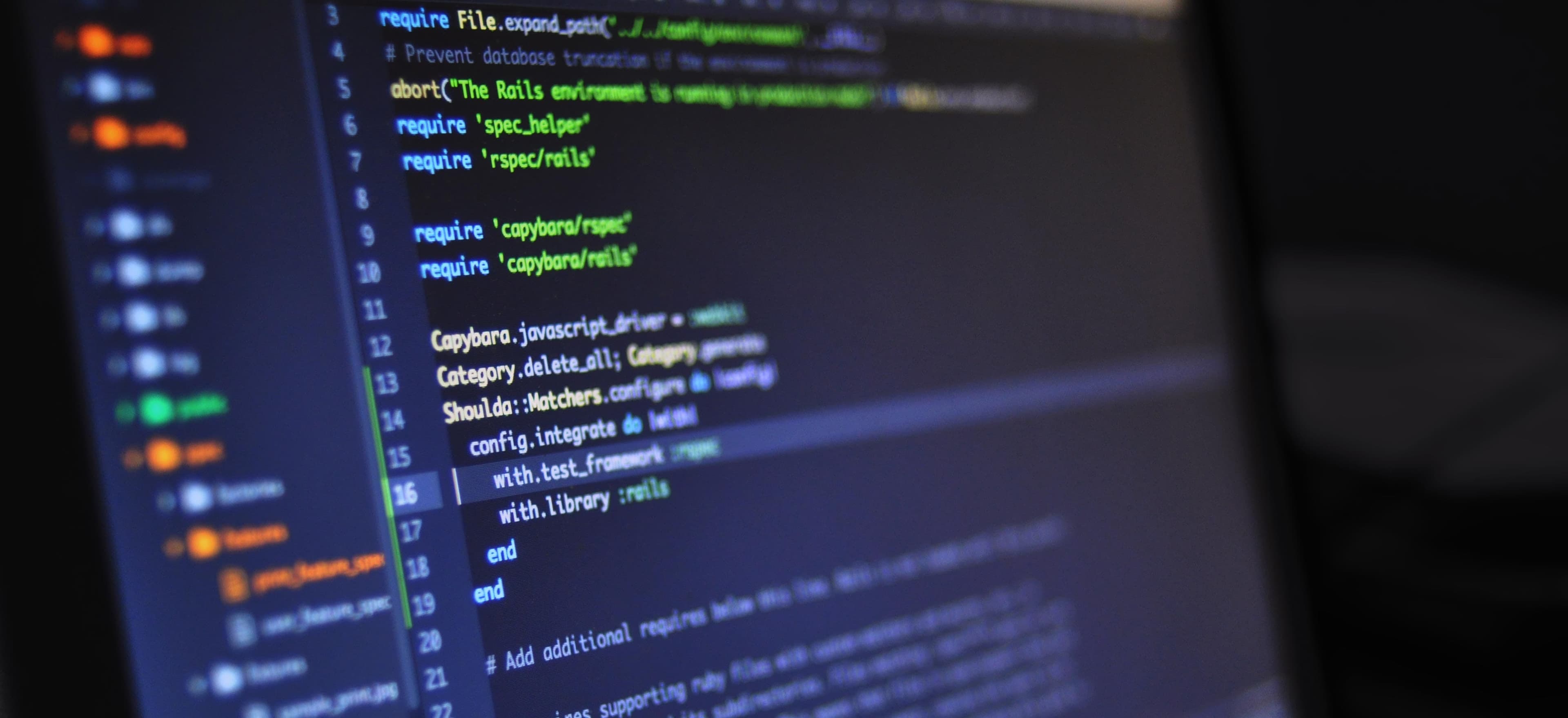
- Published on
Solving JAXB Date Formatting Issues in Spring Applications
When working with Spring applications, particularly those that involve XML data transfer, it's not uncommon to encounter issues with date formatting using JAXB (Java Architecture for XML Binding). JAXB provides a convenient way to convert Java objects into XML and vice versa. However, date formatting can sometimes lead to unexpected results, especially when dealing with time zones. This blog post aims to discuss the common pitfalls of JAXB date formatting in Spring applications and present best practices to overcome them.
Understanding JAXB and Its Date Handling
JAXB is a powerful tool that simplifies the process of working with XML in Java. It does so by enabling developers to map Java classes to XML schemas easily. While JAXB can handle many types of data seamlessly, date and time types can introduce additional complexity.
By default, JAXB uses the java.util.Date
class for date representations. However, this can lead to discrepancies in representation, especially when dealing with serialization and deserialization across different platforms.
Common Formatting Issues
-
Timezone Problems: XML data might not contain time zone information. The default behavior of JAXB is to serialize dates using the local time zone of the JVM, which may lead to confusion when the XML is consumed elsewhere.
-
Format Mismatches: Different systems might expect dates in different formats. For example, while ISO 8601 is widely accepted, some systems may require a specific format.
-
Null Handling: How null dates are represented can vary, posing a challenge for clients consuming the generated XML.
Configuring Date Formats with JAXB
To handle date formatting issues, we can use JAXB annotations to customize how dates are serialized and deserialized. This allows us to enforce a consistent date format across our Spring application. Below are some approaches you can adopt.
1. Using @XmlSchemaType
The @XmlSchemaType
annotation can specify the expected XML schema type for your date fields. Here’s how you can use it:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapter.XmlAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import java.util.Date;
@XmlRootElement
@XmlType
public class User {
private String name;
@XmlJavaTypeAdapter(DateAdapter.class)
private Date birthDate;
// Getters and Setters
}
Here, DateAdapter
is a custom adapter to handle the conversion of Date
to a specific string format.
2. Implementing a Custom Adapter
Creating a custom adapter allows for greater control over how dates are formatted. Utilize XmlAdapter
as shown below:
import javax.xml.bind.annotation.adapters.XmlAdapter;
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateAdapter extends XmlAdapter<String, Date> {
private final SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
@Override
public Date unmarshal(String dateString) throws Exception {
return dateFormat.parse(dateString);
}
@Override
public String marshal(Date date) throws Exception {
return dateFormat.format(date);
}
}
Explanation of the Adapter
- Unmarshalling: Converts the incoming string format into a
Date
object. - Marshalling: Transforms the
Date
object back into a string in the specified format.
By implementing this adapter, you ensure that date representations are consistent, and you mitigate issues related to time zones by focusing on a universally accepted format.
3. Handling Null Dates
Handling null dates can be as crucial as correctly formatting them. You may choose to represent null dates explicitly or skip them entirely during serialization. The adapter can be modified as follows:
@Override
public String marshal(Date date) throws Exception {
if (date == null) {
return null; // or return a specific string like "N/A"
}
return dateFormat.format(date);
}
Integrating with Spring
To integrate your JAXB setup seamlessly within a Spring application, define a Marshaller
bean to utilize your custom adapter:
import javax.jms.JMSException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import javax.xml.bind.JAXBContext;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class JAXBConfig {
@Bean
public Marshaller marshaller() throws Exception {
JAXBContext context = JAXBContext.newInstance(User.class);
Marshaller marshaller = context.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
return marshaller;
}
@Bean
public Unmarshaller unmarshaller() throws Exception {
JAXBContext context = JAXBContext.newInstance(User.class);
return context.createUnmarshaller();
}
}
Testing Serialization and Deserialization
Creating test cases is an essential step to ensure that your JAXB configuration works as expected. Below is a sample test using JUnit.
import org.junit.jupiter.api.Test;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import java.io.StringReader;
import java.io.StringWriter;
import java.util.Date;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class UserJaxbTest {
@Test
public void testMarshallingAndUnmarshalling() throws Exception {
User user = new User();
user.setName("John Doe");
user.setBirthDate(new Date());
// Marshalling
JAXBContext context = JAXBContext.newInstance(User.class);
Marshaller marshaller = context.createMarshaller();
StringWriter writer = new StringWriter();
marshaller.marshal(user, writer);
String xmlResult = writer.toString();
// Unmarshalling
Unmarshaller unmarshaller = context.createUnmarshaller();
User unmarshalledUser = (User) unmarshaller.unmarshal(new StringReader(xmlResult));
assertEquals(user.getName(), unmarshalledUser.getName());
assertEquals(user.getBirthDate(), unmarshalledUser.getBirthDate());
}
}
Key Points to Remember
- Consistency: Always ensure that the date format is consistent across your application.
- Testing: Write comprehensive tests to verify that your date handling is working as expected.
- Documentation: Keep your documentation up-to-date to help future developers understand date handling in your application.
Lessons Learned
JAXB can be a great asset when working with XML in Spring applications, but it comes with challenges, especially concerning date handling. By implementing custom adapters and utilizing JAXB annotations effectively, you can overcome these issues and ensure that your application handles dates in a reliable manner.
For more information on JAXB, check out the official documentation. If you encounter any specific issues not covered in this post, feel free to reach out in the comments or explore community forums for more insights.
Checkout our other articles