Struggling to Convert Maps to MultiValueMap? Here's How!
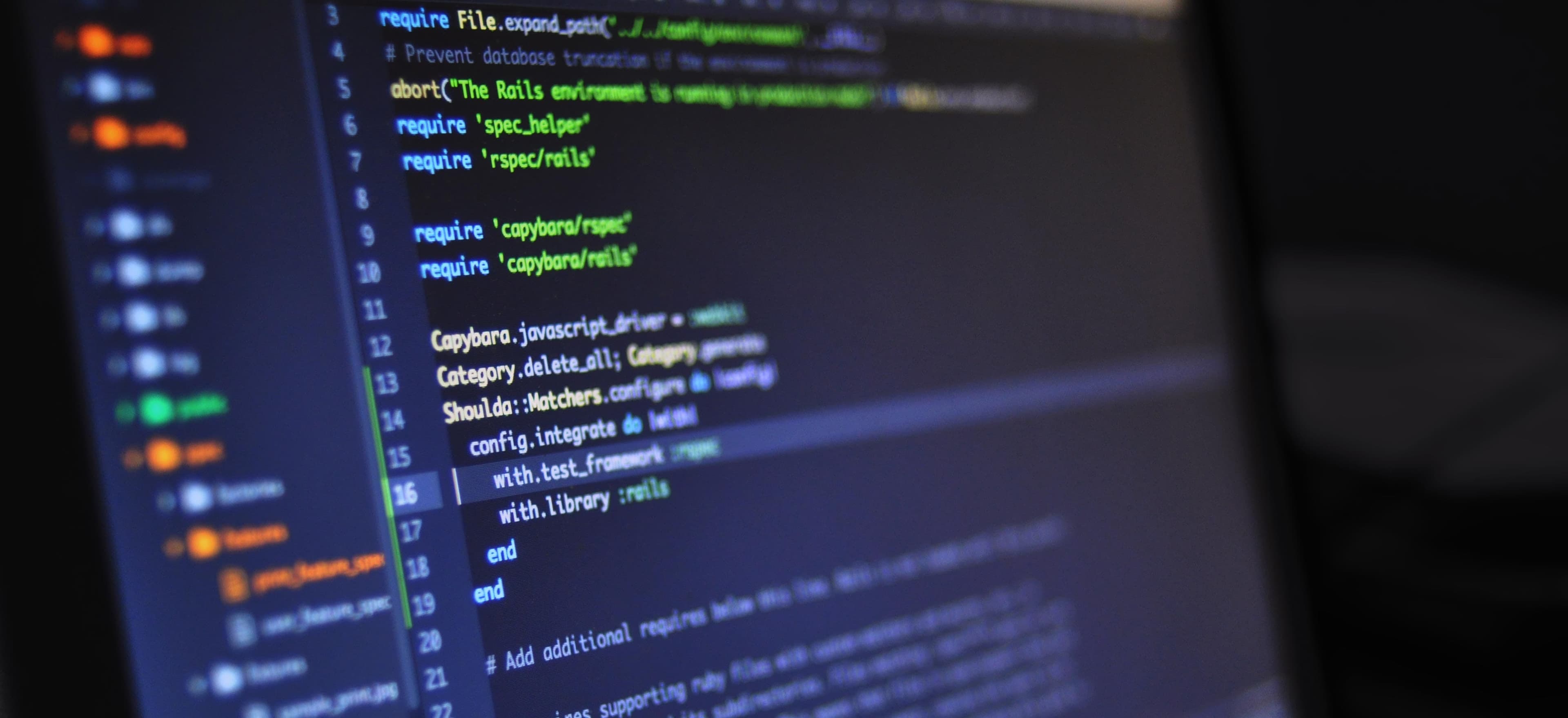
- Published on
Struggling to Convert Maps to MultiValueMap? Here's How!
In the world of Java development, it’s common to encounter various data structures that serve different purposes. One of these structures, the MultiValueMap
, can sometimes be a bit confusing to use, especially when you're transitioning from the familiar Map
interface. If you're struggling with this conversion, you’re not alone. In this blog post, we'll delve into what a MultiValueMap
is, why it can be beneficial, and how to effectively convert standard Java Map
collections to MultiValueMap
.
What Is a MultiValueMap?
Before we dive into the conversion, it’s crucial to understand what a MultiValueMap
is. In essence, a MultiValueMap
maps keys to multiple values. Unlike a standard Java Map<K, V>
, which associates a single value with a key, a MultiValueMap<K, V>
allows you to add multiple values for a single key.
Why Use a MultiValueMap?
-
Handling Duplicate Keys: If you're dealing with a scenario where a single key may need to map to multiple values (like form inputs, query parameters in HTTP requests, etc.), a
MultiValueMap
is ideal. -
Ease of Access: Retrieving values is straightforward. You can retrieve a list of values associated with a specific key without needing to handle lists or duplicates yourself.
-
Integration with Spring Framework: If you’re working in a Spring environment,
MultiValueMap
is part of Spring’sorg.springframework.util
package and integrates seamlessly with many Spring components.
Converting Map to MultiValueMap
If you find yourself with a standard Map
and need to convert it to a MultiValueMap
, the process isn’t too complicated. We’ll walk through some concrete examples to illustrate how this can be accomplished.
Example: Basic Conversion
Let’s assume we have a standard Java Map<String, String>
, representing some form of key-value pairs. Below is a simple example followed by the conversion to MultiValueMap
.
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import java.util.HashMap;
import java.util.Map;
public class MapToMultiValueMap {
public static void main(String[] args) {
// Create a standard Map
Map<String, String> simpleMap = new HashMap<>();
simpleMap.put("key1", "value1");
simpleMap.put("key2", "value2");
// Convert to MultiValueMap
MultiValueMap<String, String> multiValueMap = convertToMultiValueMap(simpleMap);
// Output the MultiValueMap
System.out.println(multiValueMap);
}
private static MultiValueMap<String, String> convertToMultiValueMap(Map<String, String> map) {
MultiValueMap<String, String> result = new LinkedMultiValueMap<>();
map.forEach(result::add);
return result;
}
}
Code Commentary
-
Creating the
Map
: In this example, we start by creating a simpleHashMap
and populate it with key-value pairs. -
Conversion Function: The
convertToMultiValueMap
method takes our standardMap
as an argument, initializes aLinkedMultiValueMap
, and utilizes theforEach
method for conversion. Theadd
method supports adding multiple values for a single key seamlessly.
Running the Code
When executed, this code will produce the following output:
{key1=[value1], key2=[value2]}
Here, you can see the converted MultiValueMap
now holds the same key-value pairs but with the capability to easily add more values later.
Example: Handling Duplicate Values
If your Map
has duplicate values and you want to maintain the integrity of the data, you might want to handle multi-value assignments explicitly.
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class AdvancedMapToMultiValueMap {
public static void main(String[] args) {
// Create a Map with duplicate values
Map<String, List<String>> mapWithDuplicates = new HashMap<>();
mapWithDuplicates.put("key1", List.of("value1", "value3"));
mapWithDuplicates.put("key2", List.of("value2", "value4"));
// Convert to MultiValueMap
MultiValueMap<String, String> multiValueMap = convertToMultiValueMapWithList(mapWithDuplicates);
// Output the MultiValueMap
System.out.println(multiValueMap);
}
private static MultiValueMap<String, String> convertToMultiValueMapWithList(Map<String, List<String>> map) {
MultiValueMap<String, String> result = new LinkedMultiValueMap<>();
map.forEach((key, values) -> values.forEach(value -> result.add(key, value)));
return result;
}
}
Explanation of Advanced Example
-
Initial Map Creation: This time, our sample
Map
usesList<String>
to hold multiple values under each key, allowing us to demonstrate handling duplicates when converting. -
Nested Conversion Logic: In the conversion function, we loop through each entry in the map. For every key, we iterate over the associated list of values and add each one to the
MultiValueMap
.
Results in Action
When you run the advanced version, the output looks like this:
{key1=[value1, value3], key2=[value2, value4]}
The MultiValueMap
now correctly reflects the relationship of multiple values for both keys.
Use Cases for MultiValueMap
MultiValueMap can be particularly beneficial in certain situations:
-
HTTP Requests: When handling HTTP requests, multi-value parameters are common. Using MultiValueMap allows you to manage form submissions conveniently.
-
Data Processing: In scenarios where datasets are processed, and you need to categorize data points under the same key, a MultiValueMap simplifies the storage and retrieval of the information.
A Final Look
In summary, converting a standard Map
to a MultiValueMap
in Java is a straightforward process that can enhance your data management capabilities. Whether you are handling forms, processing data, or simply need a flexible data structure, MultiValueMap
can provide the solution you're looking for.
For more information on Java collections, you can check the official documentation Java Collections Framework and on Spring's MultiValueMap
, read more on Spring Documentation.
Feel free to try the provided code snippets and experiment with your own data. Happy coding!