Resolving Ambiguous Method Call Errors in Mockito
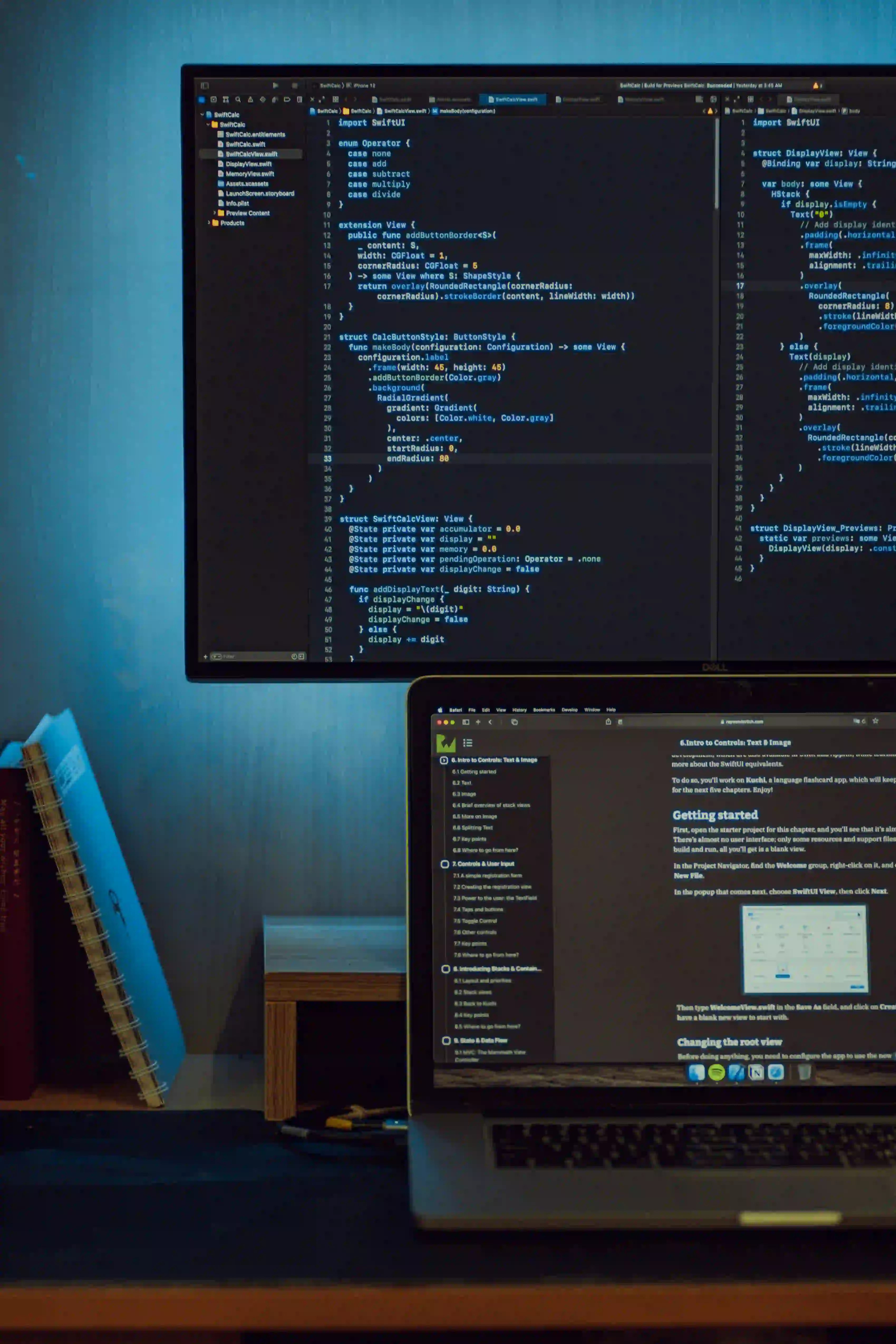
Resolving Ambiguous Method Call Errors in Mockito
When you're writing unit tests in Java, Mockito often comes to the rescue with its easy-to-use mocking framework. However, issues can arise when you encounter ambiguous method call errors. Understanding and resolving these errors can elevate your testing game significantly. This post aims to clarify how ambiguous method call errors occur in Mockito, why they matter, and most importantly, how to fix them effectively.
Understanding Ambiguous Method Call Errors
Ambiguous method call errors generally occur when Mockito is unable to distinguish between two or more overloaded methods in a mock object. Overloaded methods share the same name but differ in their parameter types or counts. This can easily lead to confusion, especially when the mocked methods are called without explicitly defining parameter types.
For instance, consider the following simple example:
public class UserService {
public String getUserDetails(int userId) {
// Fetch user details by ID
return "User details for userId: " + userId;
}
public String getUserDetails(String userName) {
// Fetch user details by name
return "User details for userName: " + userName;
}
}
In this UserService
class, we have two overloaded methods named getUserDetails
. If we try to mock and verify one of these methods without being explicit about which one we're referring to, we might run into an ambiguous call error.
Why Are These Errors Important?
Ambiguous method call errors are not just pesky little annoyances. They signify a deeper issue in your test code that could lead to unintended failures. When testing, clarity is crucial; pseudonymous method calls could lead to misleading test results or, worse, unnoticed regressions in your application.
Resolving Ambiguous Method Call Errors
Strategy 1: Use Specific Argument Matchers
One effective way to resolve ambiguous method calls is by utilizing argument matchers when setting up mocks. Argument matchers provide more context to Mockito about which method you want to mock. Here’s how you can do this:
import static org.mockito.Mockito.*;
public class UserServiceTest {
UserService userService = mock(UserService.class);
@Test
public void testGetUserDetailsWithId() {
when(userService.getUserDetails(argThat(arg -> arg instanceof Integer)))
.thenReturn("Mocked User details for ID");
String result = userService.getUserDetails(10);
assertEquals("Mocked User details for ID", result);
}
@Test
public void testGetUserDetailsWithName() {
when(userService.getUserDetails(argThat(arg -> arg instanceof String)))
.thenReturn("Mocked User details for name");
String result = userService.getUserDetails("John Doe");
assertEquals("Mocked User details for name", result);
}
}
Explanation:
- We create a mock of the
UserService
class. - In our test methods, we use
argThat
to provide specific criteria, allowing Mockito to resolve the correct overloaded method to mock. - By clearly indicating the type of argument being passed, you eliminate ambiguity.
Strategy 2: Explicitly Cast Arguments
Another straightforward way to prevent ambiguity is to downcast the arguments when you call the mocked method. This approach is less flexible compared to argument matchers, but it can be effective in simpler cases.
@Test
public void testGetUserDetailsOverloadedMethods() {
when(userService.getUserDetails((Integer) any())).thenReturn("Mocked User for ID");
when(userService.getUserDetails((String) any())).thenReturn("Mocked User for Name");
String resultById = userService.getUserDetails(1);
String resultByName = userService.getUserDetails("Jane Doe");
assertEquals("Mocked User for ID", resultById);
assertEquals("Mocked User for Name", resultByName);
}
Explanation:
- The
any()
method is cast to eitherInteger
orString
, directing Mockito to the proper method signature during the mock setup. - This leads to a clearer, unambiguous call to the appropriate method.
Strategy 3: Use Different Mocking Frameworks
While Mockito is among the most popular mocking frameworks, there are alternatives like EasyMock and JMock. Each framework has its strengths and may handle ambiguous calls differently. Some developers find that switching frameworks can simplify the mocking of complex scenarios.
That said, this should be a last resort after trying to fix ambiguous calls with the above strategies. Familiarizing yourself with multi-framework mocking strategies will also boost your versatility as a developer.
Strategy 4: Refactor Overloaded Methods
When overloaded method laws in a particular design lead to recurrent ambiguous call errors, consider refactoring the methods themselves. Introduce different names that can clarify the intent of each method:
public class UserService {
public String getUserDetailsById(int userId) {
return "User details for userId: " + userId;
}
public String getUserDetailsByName(String userName) {
return "User details for userName: " + userName;
}
}
This practice not only clarifies your code for other developers but also avoids ambiguity altogether when unit testing.
In Conclusion, Here is What Matters
In conclusion, dealing with ambiguous method call errors in Mockito is crucial for writing effective unit tests. By employing argument matchers, explicitly casting parameters, considering alternative mocking frameworks, or even refactoring your code, you can clear up any ambiguity surrounding your mock interactions.
For further reading on effective unit testing practices using Mockito, check out Mockito official documentation or Baeldung’s guide on Mockito. Understanding these techniques will not only help you resolve errors but also improve the overall quality of your Java application’s testing suite. Happy testing!