Mastering Activity State: Avoiding Common Storage Mistakes
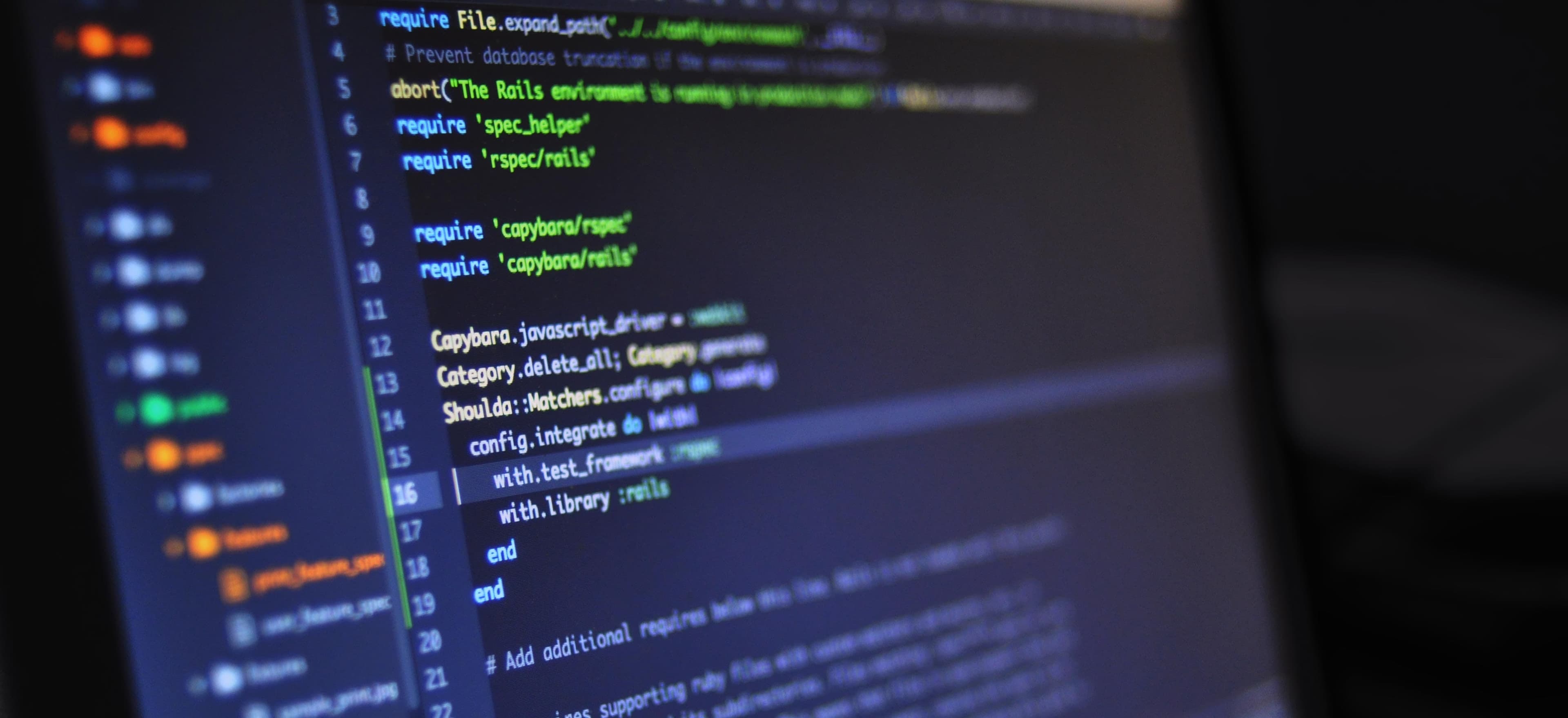
- Published on
Mastering Activity State: Avoiding Common Storage Mistakes in Java
When developing applications in Java, one of the most critical aspects is managing the activity state. Activities can be thought of as the various states an application can be in while executing. Each activity may need to save or restore some information based on its state. Inadequate management of these states can lead to data loss or application crashes.
In this blog post, we'll delve deep into the common mistakes developers make when handling activity states in Java and explore best practices to avoid them. Let’s get started!
Understanding Activity State
Before diving into the mistakes, it's essential to understand what activity state means in the context of Java applications. Typically, it refers to the information that describes the current condition and behavior of your application. This encompasses both UI state (like which screen is currently visible) and non-UI state (like user session information).
While Java manages some aspects of an application’s state automatically, developers must actively handle activity states to ensure user data isn't lost during orientation changes, backgrounding, or other lifecycle events.
A Quick Overview of Java Activity Lifecycle
Many developers are familiar with the concept of an Activity lifecycle, especially in Android development. The lifecycle states consider different phases: Active, Paused, Stopped, and Destroyed. Here is how you can visualize this:
- Active: The activity is in the foreground and interactive.
- Paused: The activity is partially visible, but it can be saved for a brief time.
- Stopped: The activity is not visible but can be resumed.
- Destroyed: The activity has finished and has been cleaned up from memory.
Managing state effectively through these transitions is key to a smooth user experience.
Common Mistakes in Activity State Management
1. Ignoring Lifecycle Events
One of the most frequent mistakes developers make is neglecting to handle lifecycle events correctly. For instance, failing to implement onSaveInstanceState()
can result in a loss of user inputs if the system kills your activity to reclaim memory.
@Override
protected void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString("username", usernameEditText.getText().toString());
}
Why is this important? This code snippet shows the implementation of onSaveInstanceState()
, which saves the state of an EditText into a Bundle. When the activity is recreated, this state can be utilized to restore the UI.
2. Overusing Static Variables
Another common mistake is using static variables to hold activity state. While it may seem convenient at first, it can lead to unintended consequences such as memory leaks.
public class MyActivity extends AppCompatActivity {
private static String savedState; // Bad Practice
}
Why is this dangerous? Static variables persist beyond the lifecycle of the activity and can cause memory leaks. Always consider using instance variables or the savedInstanceState
pattern instead.
3. Details of Serialization
Managing complex objects can be challenging due to Java's serialization limitations. Not every Java object can be serialized, and failing to recognize this can lead to runtime exceptions.
Example of a Serializable object:
import java.io.Serializable;
public class User implements Serializable {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and Setters
}
Why use Serializable? By implementing Serializable
, you're ensuring that your object can be written to and read from a byte stream, thus preserving the state data necessary for the activity.
4. Not Restoring State Properly
Failing to properly restore the state of your UI can lead to confusion and frustration for users. If the part of your activity responsible for reinitializing the user interface components does not account for all possible scenarios, you may end up with a confusing experience.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if (savedInstanceState != null) {
String username = savedInstanceState.getString("username");
usernameEditText.setText(username);
}
}
Why use this approach? In the onCreate()
method, we check if we're restoring the state. If savedInstanceState
is not null, we retrieve the username and restore it into the EditText. This practice enhances user experience significantly.
Best Practices for Activity State Management
Having identified the common pitfalls, it's time to look at some best practices that can help streamline activity state management in your Java applications.
1. Utilize onSaveInstanceState()
Consistently utilize onSaveInstanceState()
to store critical information before the activity is destroyed or goes into the background. This allows for easy restoration of the state in case the activity is recreated.
2. Use ViewModels for UI-related Data
If you're using Android Jetpack, view models are an excellent solution for managing UI-related data in a lifecycle-conscious way. They help in separating out UI data from business logic.
3. Embrace Parcelable over Serializable
For better performance, prefer using Parcelable over Serializable for transferring data between activities. Parcelable is specifically designed for Android and is faster and more efficient.
Example:
import android.os.Parcel;
import android.os.Parcelable;
public class User implements Parcelable {
private String name;
private int age;
protected User(Parcel in) {
name = in.readString();
age = in.readInt();
}
public static final Creator<User> CREATOR = new Creator<User>() {
@Override
public User createFromParcel(Parcel in) {
return new User(in);
}
@Override
public User[] newArray(int size) {
return new User[size];
}
};
@Override
public int describeContents() {
return 0;
}
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeString(name);
dest.writeInt(age);
}
}
Why use Parcelable? By implementing Parcelable, you enhance performance and provide a clean interface for data transfer between activities in Android.
4. Create a Robust State Restoration Strategy
Incorporate a pattern to consistently manage and restore state across various lifecycle events. This could involve managing significant data in a data repository or a dedicated manager class.
Closing Remarks
Managing activity states effectively is essential for creating resilient Java applications. By being mindful of the common storage mistakes and adhering to best practices, developers can significantly improve the user experience and ensure data consistency. Remember to leverage the built-in lifecycle management approaches provided by the Java ecosystem, enhancing both performance and reliability in your apps.
For a deeper dive into handling data in Android apps, consider exploring Android Lifecycle Docs for more insights.
In mastering activity state management, not only do you craft better applications, but you also become a more proficient Java developer. Happy coding!