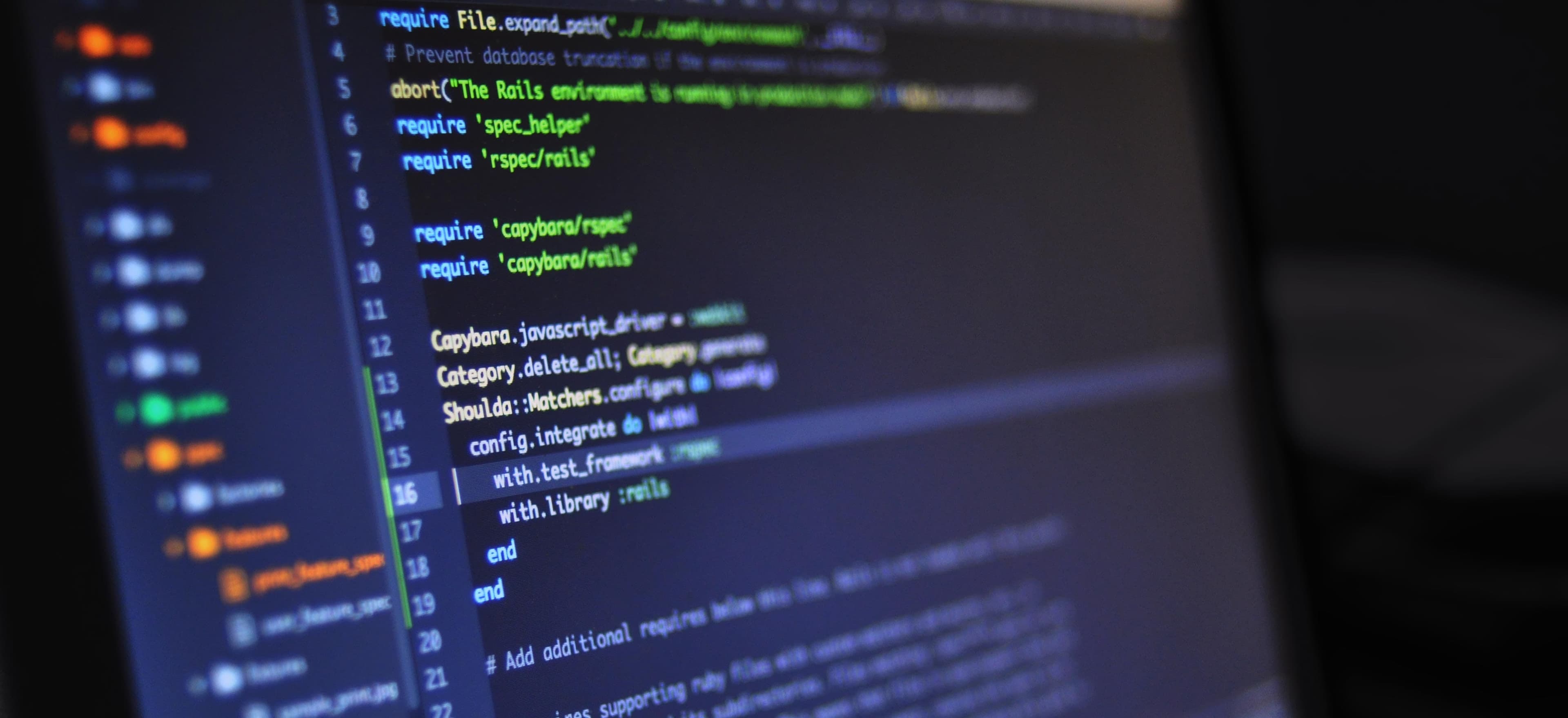
- Published on
Mastering Row-Level Security in jOOQ: A Step-by-Step Guide
In today's data-driven world, security is paramount. One of the growing concerns in application development is ensuring that users can only access the data they are authorized to view. Row-Level Security (RLS) is a powerful concept that restricts data access on a per-user basis without requiring extensive SQL logic in the application layer. When combined with jOOQ, a popular SQL generation library for Java, RLS can be implemented effectively to protect sensitive information.
In this guide, we'll explore the principles of Row-Level Security, understand its importance, and go through a step-by-step implementation in jOOQ.
What is Row-Level Security?
Row-Level Security enables fine-grained access control within the database. It allows system administrators and developers to create policies that determine who can see which rows of a table based on certain conditions, such as user roles or attributes.
For example, in a multi-tenant application, you might want users to only access data pertaining to their specific organization. RLS helps enforce this rule at the database level, reducing the risk of unauthorized data exposure.
Why Use jOOQ?
jOOQ (Java Object Oriented Querying) is known for its fluent API and type-safe SQL generation, making it easier to work with databases in Java. Some advantages of using jOOQ include:
- Type-Safety: Helps to catch SQL issues at compile time.
- Fluent API: Supports writing SQL-like code in a way that is more natural to Java developers.
- Vendor Agnostic: Works with multiple databases, making it versatile.
Setting Up jOOQ
To start utilizing jOOQ, you first need to add its dependencies to your project. If you are using Maven, add the following dependencies to your pom.xml
:
<dependencies>
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq</artifactId>
<version>3.16.0</version>
</dependency>
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq-meta</artifactId>
<version>3.16.0</version>
</dependency>
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq-codegen</artifactId>
<version>3.16.0</version>
</dependency>
</dependencies>
Run mvn clean install
to download the dependencies.
Step 1: Enable Row-Level Security in the Database
Row-Level Security is primarily a database feature. Let's enable it on a PostgreSQL database as an example.
- Create a Sample Table (for demonstration):
CREATE TABLE documents (
id SERIAL PRIMARY KEY,
content TEXT NOT NULL,
owner_id INT NOT NULL
);
- Enable RLS:
ALTER TABLE documents ENABLE ROW LEVEL SECURITY;
- Create a Policy:
CREATE POLICY user_policy ON documents
FOR SELECT
USING (owner_id = current_user_id());
In this example, we create a policy that allows users to see rows where the owner_id
matches the ID of the currently logged-in user.
Step 2: Connect jOOQ to the Database
Now we need to set up jOOQ to connect with the database. Here is a basic configuration example:
import org.jooq.*;
import org.jooq.impl.DSL;
import javax.sql.DataSource;
public class DatabaseConfig {
private static DSLContext create;
static {
DataSource dataSource = // initialize your data source
create = DSL.using(dataSource, SQLDialect.POSTGRES);
}
public static DSLContext getContext() {
return create;
}
}
This initializes a DSLContext
, which we will use for all our database interactions.
Step 3: Accessing Data with Row-Level Security
When accessing the secured data, jOOQ will respect the Row-Level Security policies defined in the database.
import static com.example.jooq.tables.Documents.DOCUMENTS;
public class DocumentService {
public List<DocumentRecord> fetchUserDocuments() {
DSLContext dsl = DatabaseConfig.getContext();
return dsl.selectFrom(DOCUMENTS)
.fetch();
}
}
Code Explanation:
- We import the generated
Documents
table reference from jOOQ. - The
fetchUserDocuments
method executes a simpleselect
query. - Due to the Row-Level Security policies, each user will only receive the document rows they are authorized to view based on their
owner_id
.
Step 4: Testing the Implementation
It's essential to test that Row-Level Security is correctly configured. Here’s one way to test it:
-
Setup Different Users: Create database users and insert sample data with differing
owner_id
values. -
Run Tests: For each user, attempt to fetch documents using your
fetchUserDocuments()
method. Each user should only see the documents they own.
import org.junit.jupiter.api.Test;
public class DocumentServiceTests {
@Test
void testUserDocuments() {
DocumentService service = new DocumentService();
// Assume the current user context is set to User A
List<DocumentRecord> userADocuments = service.fetchUserDocuments();
assertEquals(1, userADocuments.size()); // User A has one document
// Change user context to User B and test again
//...
}
}
My Closing Thoughts on the Matter
Row-Level Security in jOOQ allows developers to enforce data access policies directly within the database layer. By using a combination of policies in the database and jOOQ for the application code, you can implement a robust security model that reduces the risk of unauthorized access.
This guide provided a foundational understanding of RLS and its integration within jOOQ. For a deeper dive into jOOQ, you can explore their official documentation to further enhance your knowledge.
Additional Resources
By mastering Row-Level Security with jOOQ, you can create applications that are both powerful and secure, fulfilling the needs of modern data management while keeping sensitive information safe.