Struggling with First-Class Procedures in OO and Functional?
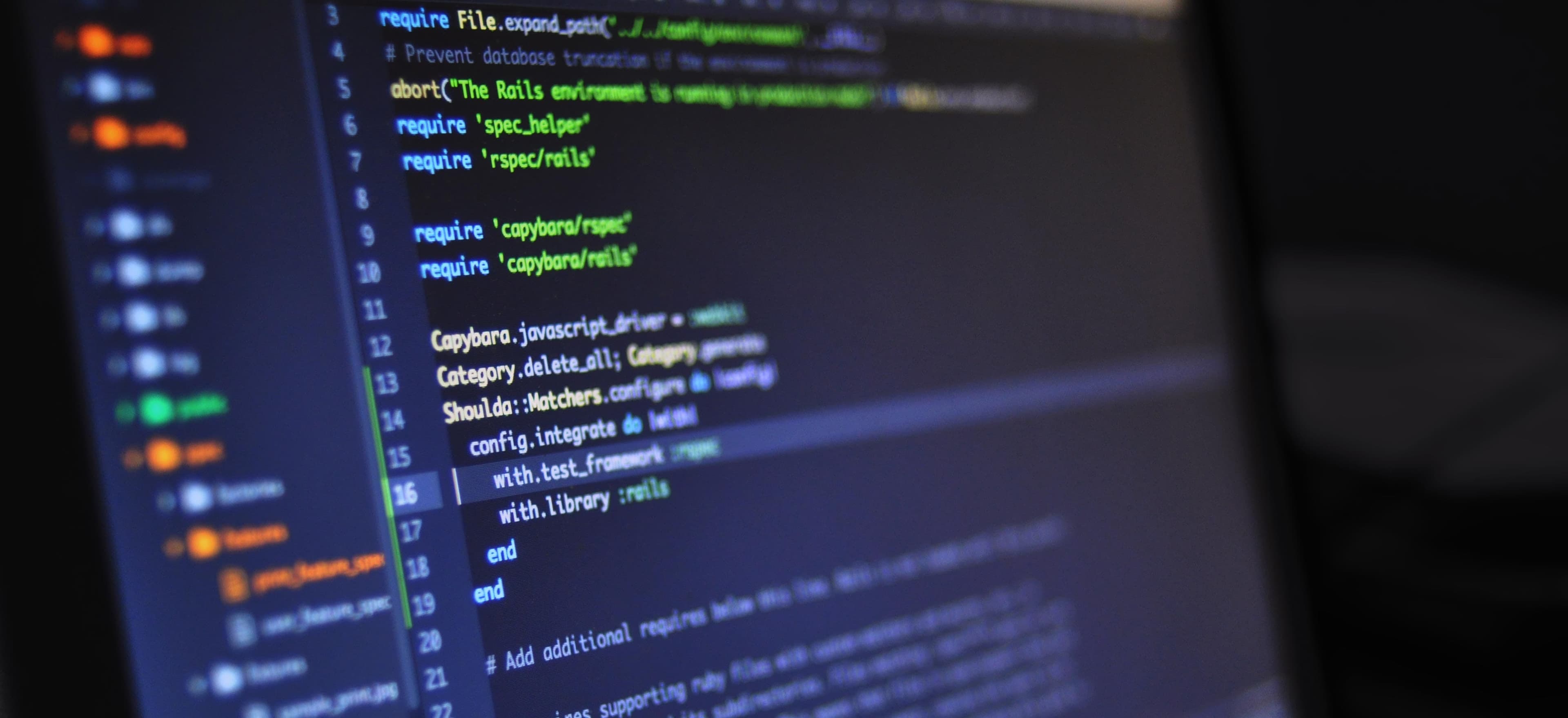
- Published on
Struggling with First-Class Procedures in Object-Oriented and Functional Programming?
First-class procedures are a fundamental concept in both object-oriented (OO) and functional programming paradigms. At its core, a first-class procedure can be treated like any other value: it can be passed as a parameter, returned from a function, and assigned to variables. Understanding this concept is crucial for leveraging the full power of programming languages that support these paradigms.
In this blog post, we'll dive deep into first-class procedures, explore their significance, and illustrate them with Java examples. We’ll also draw comparisons and understand how OO and functional programming approaches differ and overlap. Let’s get started!
What Are First-Class Procedures?
In programming, first-class entities are those that can be manipulated just like other data types. These entities have four essential traits:
- Creating: You can create procedures.
- Assigning: You can assign procedures to variables.
- Passing: You can pass procedures as arguments to other functions.
- Returning: You can return procedures from other functions.
In languages like Java, procedures are typically represented as methods within classes. However, through the introduction of functional interfaces and lambda expressions in Java 8, Java also supports first-class procedures to some extent.
First-Class Procedures in Functional Programming
Functional programming emphasizes pure functions and immutability. Procedures in functional languages (like JavaScript, Python, or Haskell) are inherently first-class.
Here is an example using JavaScript, a language that prioritizes functional programming principles:
const greet = (name) => `Hello, ${name}!`;
const callFunction = (func, value) => {
return func(value);
};
console.log(callFunction(greet, "World")); // Outputs: Hello, World!
Code Explanation:
- The
greet
function is defined using a concise arrow function syntax. - The
callFunction
takes another function (func
) and a value, showing that we can pass functions as arguments. - This flexibility makes functional programming powerful, allowing for higher-order functions that can operate on or return functions.
First-Class Procedures in Object-Oriented Programming
Java is primarily an object-oriented language, but since version 8, it has adopted some functional programming features. Let's look at an example of using lambda expressions as first-class procedures within the realm of Java.
Example 1: Functional Interfaces
In Java, a functional interface is an interface that has exactly one abstract method. This is a powerful way to represent first-class procedures.
@FunctionalInterface
interface Greeting {
String sayHello(String name);
}
public class Main {
public static void main(String[] args) {
Greeting greet = name -> "Hello, " + name + "!"; // Lambda expression
System.out.println(greet.sayHello("World")); // Outputs: Hello, World!
}
}
Code Explanation:
- The
Greeting
interface illustrates how we can define a functional interface. - The
sayHello
method is the single abstract method. - We implement the
Greeting
interface using a lambda expression, showcasing the syntax for anonymous function implementation in Java.
Example 2: Passing Functions
We can create methods that accept these functional interfaces, effectively passing procedures.
public class Main {
public static void main(String[] args) {
printGreeting("World", greet);
}
public static void printGreeting(String name, Greeting greeting) {
System.out.println(greeting.sayHello(name)); // Invoking the lambda
}
}
Code Explanation:
- The
printGreeting
method accepts aGreeting
type, demonstrating that we can pass procedures around just like other data types. - We invoke the
greet
lambda, effectively treating it as a first-class entity.
Benefits of First-Class Procedures
- Higher-order Functions: Functions that can take other functions as arguments or return functions as results are invaluable in abstraction.
- Code Reusability: Procedures can be reused in different contexts, maintaining the DRY (Don’t Repeat Yourself) principle.
- Cleaner Code: The ability to pass procedures can lead to cleaner and more understandable code, avoiding boilerplate that would be necessary otherwise.
Differences Between OO and Functional Approaches
While both paradigms support first-class procedures, their application and design philosophies differ:
- State Management: OO often manages state through object attributes, while functional programming strives for immutability and statelessness.
- Data vs. Behavior: OO revolves around data and the behavior associated with that data (methods), whereas functional programming treats procedures as primary with data being secondary.
Final Considerations
Understanding first-class procedures can elevate your programming skill set, allowing you to write code that is more modular, reusable, and clean. In Java, you can use functional interfaces and lambda expressions to effectively emulate first-class procedures from functional languages while still benefitting from OO principles.
With Java continually evolving, embracing these concepts becomes increasingly essential for any developer looking to enhance their craft. By exploring both paradigms, you can greatly improve your approach to problem-solving and code structuring.
For more insights into functional programming in Java, check out this official documentation.
In your explorations, don't hesitate to experiment with higher-order functions in your codebase. Observe how they affect readability and reusability. Understanding these principles and how they apply across different programming paradigms will undoubtedly sharpen your programming skills for years to come. Happy coding!
Checkout our other articles