Mastering JSON Deserialization: Super Type Tokens Explained
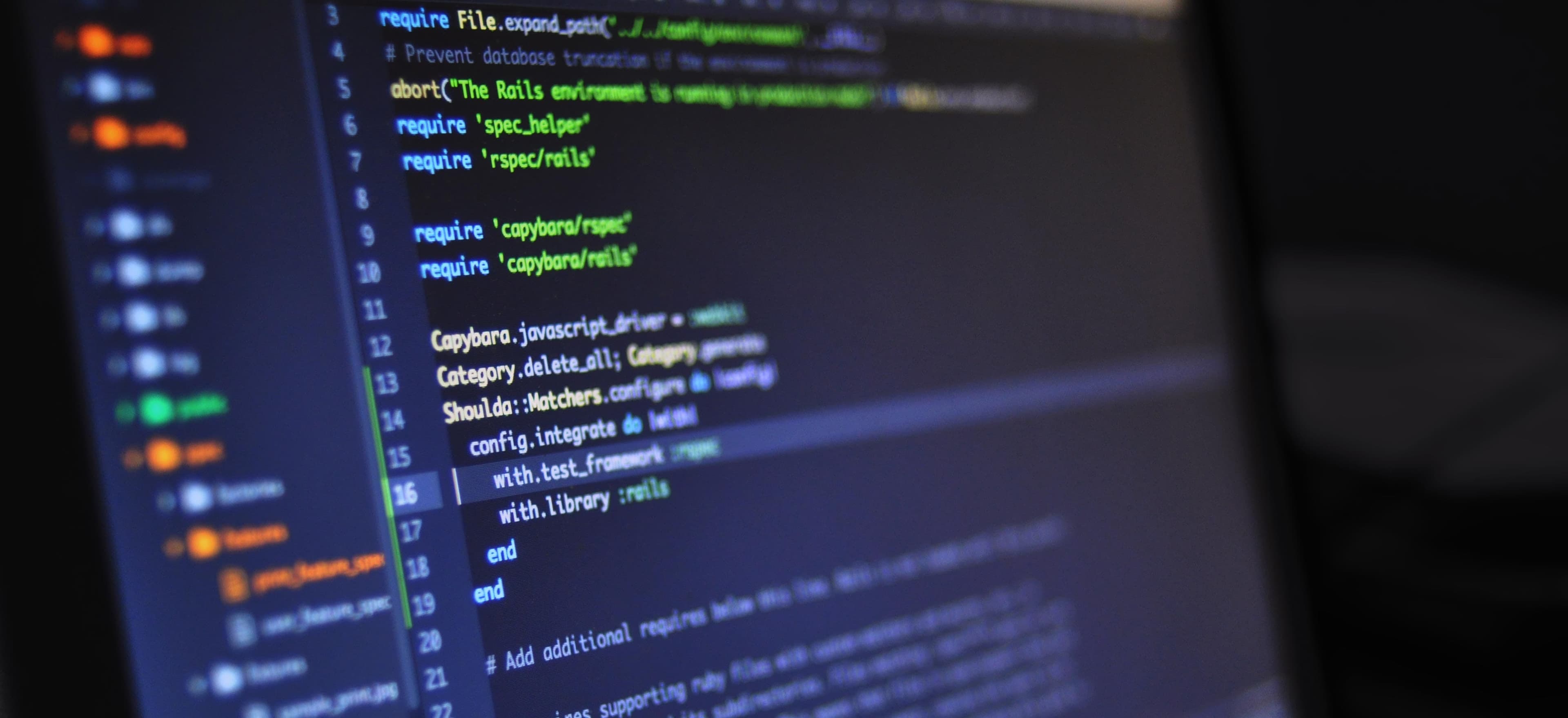
- Published on
Mastering JSON Deserialization: Super Type Tokens Explained
In the world of Java programming, one of the common requirements is the ability to work with JSON data. JSON, or JavaScript Object Notation, is a lightweight data interchange format that's easy for humans to read and write, and easy for machines to parse and generate.
When it comes to deserializing JSON in Java, things can get tricky, especially when you're dealing with generics. This is where super type tokens come into play. In this blog post, we will explore what super type tokens are, why they are important, and how you can use them effectively in your JSON deserialization processes.
Why JSON Deserialization?
JSON deserialization is the process of converting JSON data into Java objects. Whether you're communicating with web services or reading configuration files, being able to quickly and accurately deserialize JSON is imperative.
For example, consider a simple JSON object returned from a web API:
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
}
You might want to convert this into a Java object, like so:
public class User {
private int id;
private String name;
private String email;
// Getters and Setters
}
Simple, right? But what if the JSON data is more complex and involves collections or generics? Enter super type tokens.
Understanding Super Type Tokens
Super type tokens allow you to maintain type information when working with generics. This is particularly important during the deserialization of complex objects because Java's type erasure at runtime removes this information.
When to Use Super Type Tokens
Suppose we have a list of User
objects represented in JSON:
[
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
},
{
"id": 2,
"name": "Jane Smith",
"email": "jane@example.com"
}
]
To deserialize this JSON into a List<User>
, you'd need to maintain the generic type List<User>
. Let's see how to accomplish this using super type tokens.
Implementing Super Type Tokens
We'll use the popular Gson library for parsing JSON in Java. First, make sure to include Gson in your project. For Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.8</version>
</dependency>
Step 1: Create the User Class
Here's the User
class we've discussed. Ensure you have the appropriate getters and setters.
public class User {
private int id;
private String name;
private String email;
// Getters
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getEmail() {
return email;
}
// Setters
public void setId(int id) {
this.id = id;
}
public void setName(String name) {
this.name = name;
}
public void setEmail(String email) {
this.email = email;
}
}
Step 2: Define a Super Type Token
Next, you can define a super type token that represents the list of User
objects. This can be accomplished using TypeToken
from Gson.
Here's how:
import com.google.gson.Gson;
import com.google.gson.reflect.TypeToken;
import java.lang.reflect.Type;
import java.util.List;
public class JsonDeserializerExample {
public static void main(String[] args) {
// Sample JSON data
String jsonArray = "[{\"id\":1,\"name\":\"John Doe\",\"email\":\"john@example.com\"},{\"id\":2,\"name\":\"Jane Smith\",\"email\":\"jane@example.com\"}]";
// Create Gson instance
Gson gson = new Gson();
// Define a TypeToken for List<User>
Type userListType = new TypeToken<List<User>>() {}.getType();
// Deserialize JSON array to List<User>
List<User> users = gson.fromJson(jsonArray, userListType);
// Printing deserialized users
for (User user : users) {
System.out.println(user.getName() + " (" + user.getEmail() + ")");
}
}
}
Explanation of Code
- Gson Instance: We create an instance of
Gson
which will be used for deserialization. - TypeToken:
TypeToken<List<User>>() {}
is a way to create a subclass ofTypeToken
to capture the generic type information at runtime. - Deserialization: The
fromJson
method takes the JSON string and theType
provided by theTypeToken
instance to turn the JSON into the desired list type.
Benefits of Using Super Type Tokens
- Type Safety: By maintaining generics, you minimize the risk of runtime errors.
- Clarity: The purpose of the code becomes clearer. Anyone reading it understands that you're working with a specific type.
- Flexibility: You can easily expand the functionality to support more complex JSON structures without losing type information.
Handling More Complex Structures
If your JSON involves nested objects or several collections, you can still use super type tokens. Let's examine a more complex example where we have a JSON structure like this:
{
"users": [
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
},
{
"id": 2,
"name": "Jane Smith",
"email": "jane@example.com"
}
]
}
To deserialize this structure, you'll create parent classes and modify the deserialization process accordingly.
public class UserContainer {
private List<User> users;
public List<User> getUsers() {
return users;
}
public void setUsers(List<User> users) {
this.users = users;
}
}
Then change your deserialization code as follows:
String jsonObject = "{\"users\":[{\"id\":1,\"name\":\"John Doe\",\"email\":\"john@example.com\"},{\"id\":2,\"name\":\"Jane Smith\",\"email\":\"jane@example.com\"}]}";
Type userContainerType = new TypeToken<UserContainer>() {}.getType();
UserContainer userContainer = gson.fromJson(jsonObject, userContainerType);
List<User> userList = userContainer.getUsers();
for (User user : userList) {
System.out.println(user.getName() + " (" + user.getEmail() + ")");
}
Summary
Using super type tokens in JSON deserialization helps manage generics with clarity and safety. Utilizing libraries like Gson allows you to seamlessly convert JSON data into Java objects, making interactions with APIs and configuration files much simpler.
For further reading on JSON parsing in Java using Gson, check out the Gson Documentation or explore the Java Generics Tutorial for a deeper understanding of generics.
With a firm grasp of super type tokens, you can take advantage of Java's type system to ensure your applications remain robust and maintainable. Happy coding!
Checkout our other articles